Article catalogue
- Ribbon integration Trilogy
- Modify the Ribbon's default load policy
- Ribbon's built-in load balancing algorithm
- Source code
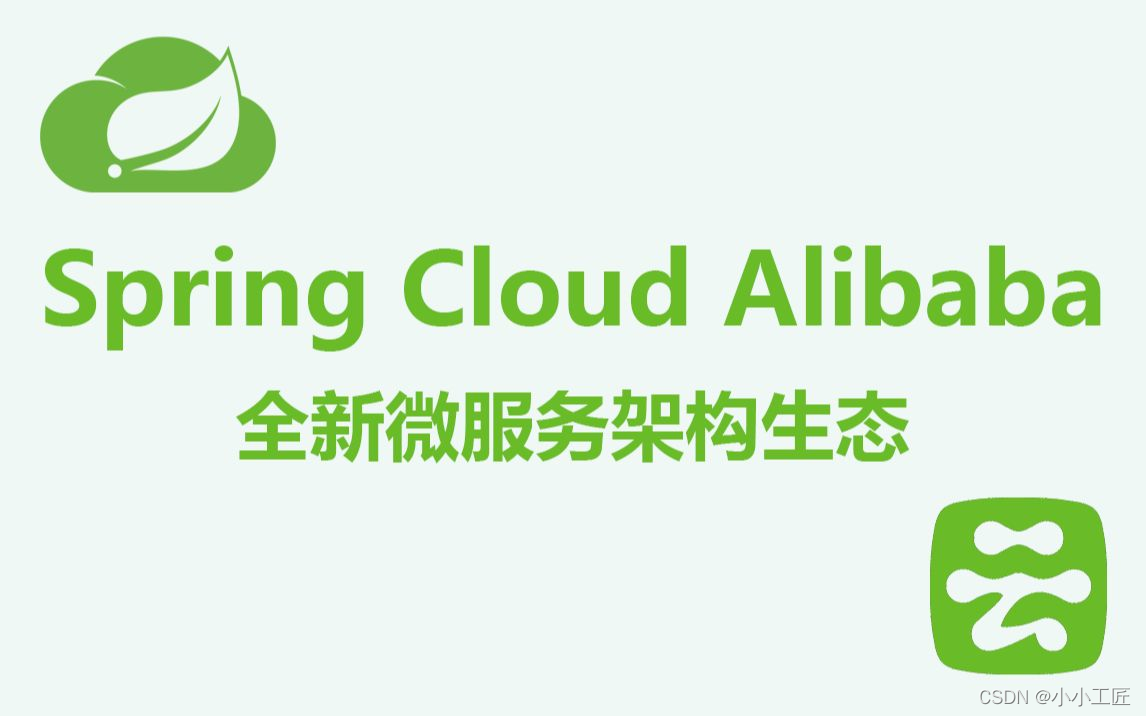
Ribbon integration Trilogy
Here we practice load balancing through the Ribbon component [the default load balancing algorithm is polling]

artisan-cloud-ribbon-order
step1 do dependency
<dependency> <groupId>com.alibaba.cloudgroupId> <artifactId>spring-cloud-alibaba-nacos-discoveryartifactId> dependency> <dependency> <groupId>org.springframework.cloudgroupId> <artifactId>spring-cloud-starter-netflix-ribbonartifactId> dependency>
Step 2 annotation (add @ LoadBalanced annotation on RestTemplate)
Step3 configuration file@Configuration public class WebConfig { @Bean @LoadBalanced public RestTemplate restTemplate(){ return new RestTemplate(); } }
Here is the configuration file of Nacos, and the configuration of Ribbon is not configured for the time being
spring: cloud: nacos: discovery: server-addr: 1.117.97.88:8848 application: name: artisan-order-center
artisan-cloud-ribbon-product
As a service provider, you only need to register with Nacos without integrating the Ribbon and start multiple load policies of the test Ribbon.
@RestController @Slf4j public class ProductInfoController { @Value("${server.port}") private Integer port; @Autowired private ProductInfoMapper productInfoMapper; @RequestMapping("/selectProductInfoById/{productNo}") public Object selectProductInfoById(@PathVariable("productNo") String productNo) { log.info("{} Requested",port); ProductInfo productInfo = productInfoMapper.selectProductInfoById(productNo); return productInfo; } }
verification

Three separate requests
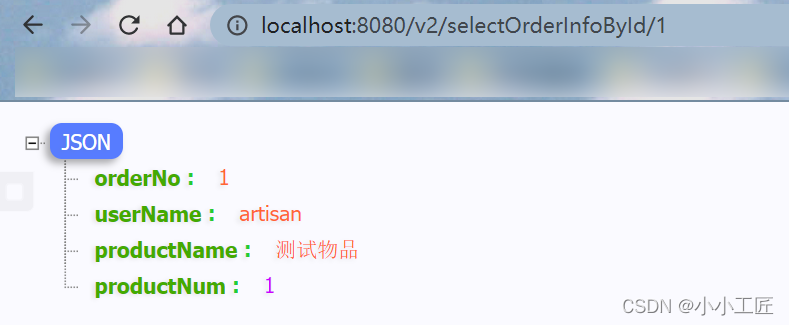
The log is as follows
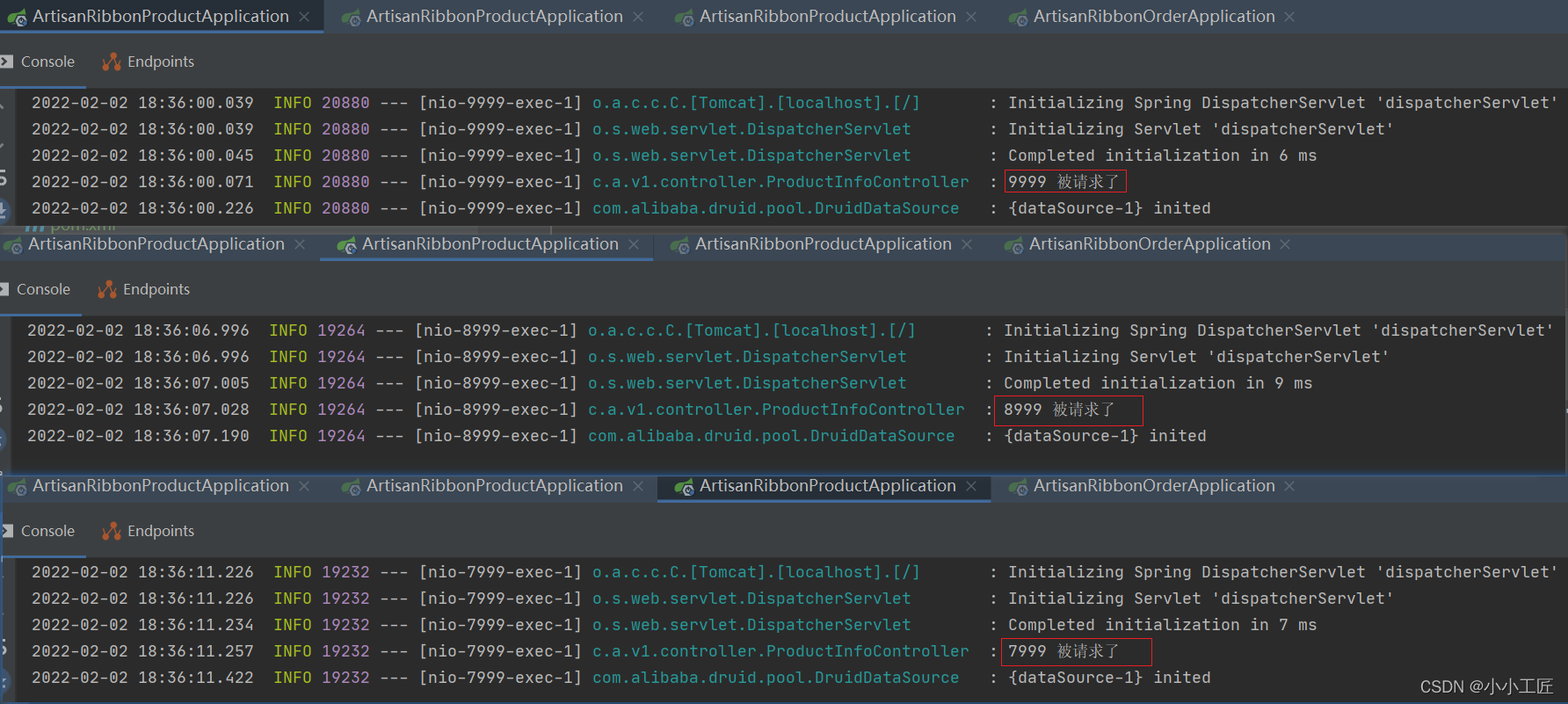
It can be guessed that the default policy is polling algorithm
Modify the Ribbon's default load policy
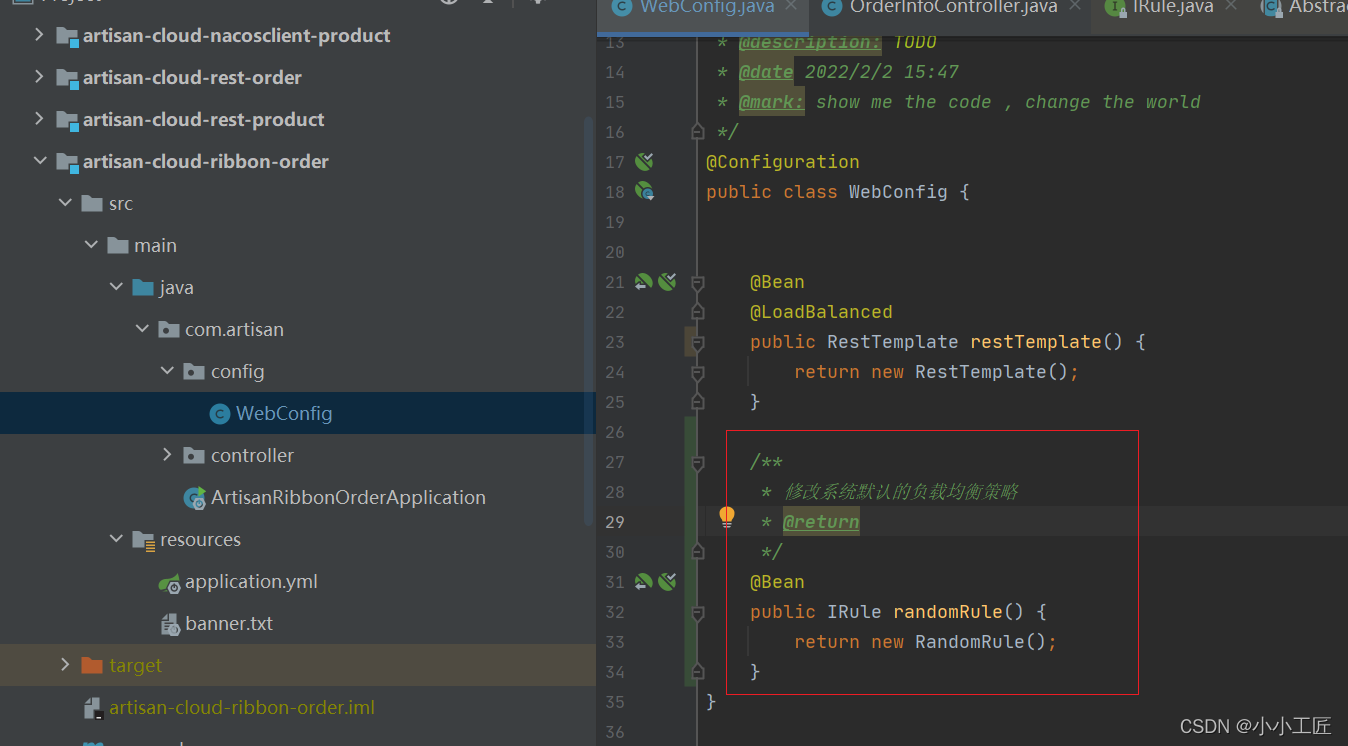
Request three times

Ribbon's built-in load balancing algorithm
Class relationship (AbstractLoadBalancerRule abstract class of IRule interface)
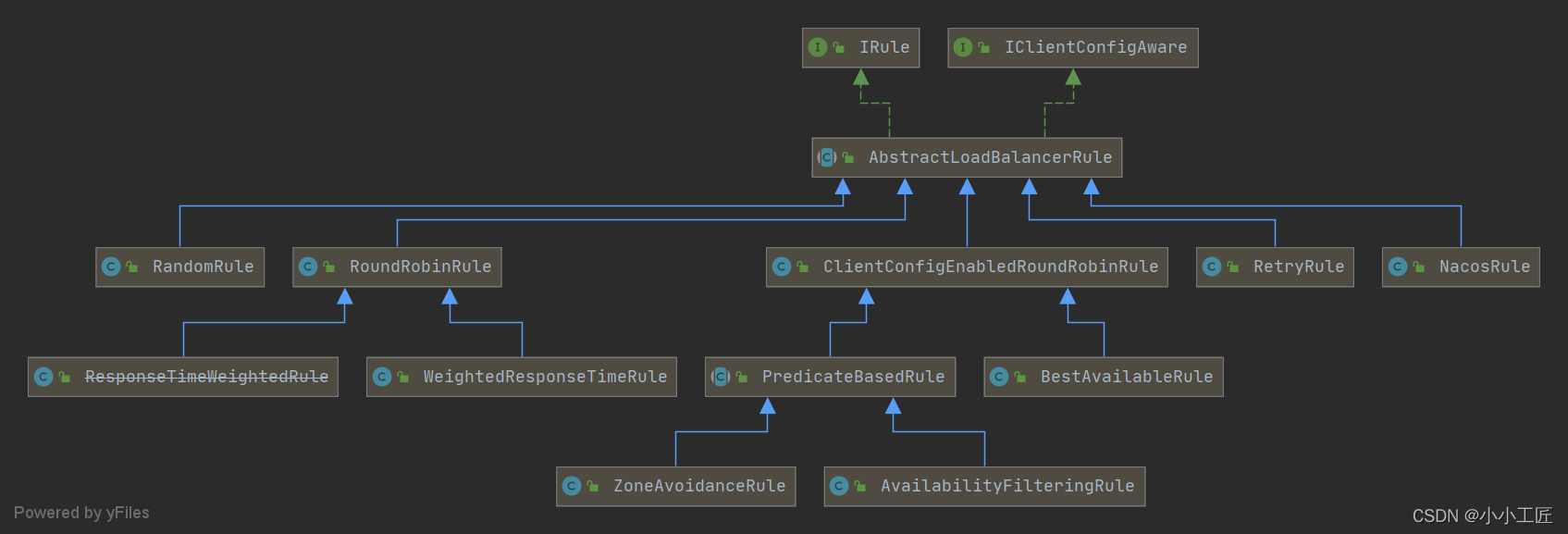
It can be seen that the policy design pattern is adopted, and the public ones are written in the abstract class
Load balancing algorithm
RandomRule
Randomly select a Server
RetryRule
For the on-board retry mechanism of the selected load balancing policy, if the server is not selected successfully within a configured time period, it has been trying to select an available server by using the subRule method
RoundRobinRule
Poll selection, poll index, and select the Server corresponding to the index
AvailabilityFilteringRule
Filter out the back-end servers marked as circuit tripped that have failed to connect all the time, and filter out those high concurrency back-end servers, or use an availability predicate to include the logic of filtering servers. In fact, it is to check the running status of each server recorded in status
BestAvailableRule
Select a Server with the smallest concurrent request and inspect the servers one by one. If the Server is tripped, skip it.
WeightedResponseTimeRule
According to the response time weighting, the longer the response time, the smaller the weight, and the lower the possibility of being selected;
ZoneAvoidanceRule (default)
Judge the performance of the Zone where the Server is located and the availability of the Server. Select the Server. If there is no Zone, the class is polling.