The following table sorts out the accessible methods in the JpaRepository interface (inheriting the CrudRepository interface and pagingandsortingreposition interface) for simple queries. (1) First, sort out according to the function, which is divided into five categories: save, delete, find single, find multiple and others. (2) Then gray out the methods that are not recommended. Most of these methods are defined in the CrudRepository interface and pagingandsortingreposition interface. Later, alternative methods are defined in the JpaRepository interface, which is more convenient to use. For example, when finding multiple objects, it is easier to return List than Iterable.
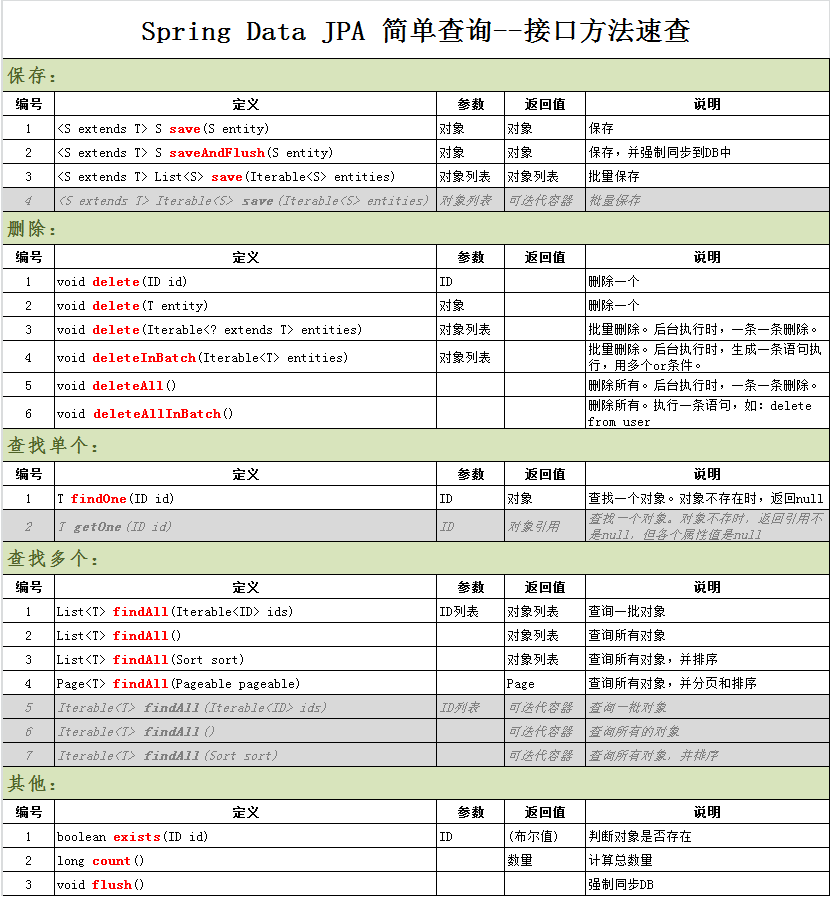
2, Detailed explanation of five interfaces
1. CrudRepository interface.
Where T is the entity class to operate, and ID is the type of entity class primary key. The interface provides 11 common operation methods.
@NoRepositoryBean public interface CrudRepository<T, ID extends Serializable> extends Repository<T, ID> { <S extends T> S save(S entity);//preservation <S extends T> Iterable<S> save(Iterable<S> entities);//Batch save T findOne(ID id);//Query an object by id. Returns the object itself. When the object does not exist, null is returned Iterable<T> findAll();//Query all objects Iterable<T> findAll(Iterable<ID> ids);//Query all objects according to the id list boolean exists(ID id);//Judge whether the object exists according to the id long count();//Total number of calculation objects void delete(ID id);//Delete by id void delete(T entity);//Delete an object void delete(Iterable<? extends T> entities);//Batch deletion, collection objects (when executed in the background, delete one by one) void deleteAll();//Delete all (one by one during background execution) }
2. PagingAndSortingRepository interface.
This interface inherits the CrudRepository interface and provides two methods to realize the functions of paging and sorting.
@NoRepositoryBean public interface PagingAndSortingRepository<T, ID extends Serializable> extends CrudRepository<T, ID> { Iterable<T> findAll(Sort sort);// Sort only Page<T> findAll(Pageable pageable);// Paging and sorting }
3. JpaRepository interface.
This interface inherits the PagingAndSortingRepository interface.
At the same time, it also inherits the QueryByExampleExecutor interface, which is an interface for querying with "instance", which will be described in detail later.
@NoRepositoryBean public interface JpaRepository<T, ID extends Serializable> extends PagingAndSortingRepository<T, ID>, QueryByExampleExecutor<T> { List<T> findAll(); //Query all objects and return List List<T> findAll(Sort sort); //Query all objects, sort and return List List<T> findAll(Iterable<ID> ids); //Query all objects according to the id List and return List void flush(); //Force cache synchronization with database <S extends T> List<S> save(Iterable<S> entities); //Batch save and return object List <S extends T> S saveAndFlush(S entity); //Save and force database synchronization void deleteInBatch(Iterable<T> entities); //Delete collection objects in batch (when executing in the background, generate a statement for execution, and use multiple or conditions) void deleteAllInBatch();//Delete all (execute a statement, such as delete from user) T getOne(ID id); //Query an object by id and return the reference of the object (different from findOne). When the object does not exist, the returned reference is not null, but each property value is null @Override <S extends T> List<S> findAll(Example<S> example); //Query by instance @Override <S extends T> List<S> findAll(Example<S> example, Sort sort);//Query and sort according to the instance. }
Notes:
(1) Compared with the CrudRepository interface, several query, and batch saving methods return a List, which is more convenient to use.
(2) InBatch deletion is added. During actual execution, an sql statement is generated in the background, which is more efficient. In comparison, the deletion methods of the CrudRepository interface are deleted one by one, even deleteAll is deleted one by one.
(3) The getOne() method is added. Remember that this method returns an object reference. When the queried object does not exist, its value is not Null.
4. JpaSpecificationExecutor interface This interface provides support for JPA Criteria query (dynamic query). This interface is very useful. It doesn't stick to the source code.
reference resources:
http://www.cnblogs.com/derry9005/p/6282571.html
http://www.cnblogs.com/dreamroute/p/5173896.html
5. Repository interface This interface is the most basic interface. It's just a symbolic interface without defining any methods. What's the use of this interface? Since Spring data JPA provides this interface, it naturally has its use. For example, we don't want to provide some methods externally. For example, we only want to provide adding and modifying methods instead of deleting methods, so the previous interfaces can't do it. At this time, we can inherit this interface, Then copy the corresponding methods in the CrudRepository interface to the Repository interface.