Spring framework - spring overview and IOC container
Spring framework overview
- Spring is a lightweight open source Java EE framework
- Spring framework can solve the complexity of enterprise application development.
- Spring has two core parts: IOC and AOP.
- IOC: control inversion, leaving the process of creating objects to Spring for management.
- AOP: aspect oriented, no source code modification, function enhancement.
- Spring features:
- Convenient decoupling and simplified development.
- AOP programming support.
- Facilitate program testing.
- Facilitate integration with other frameworks.
- Facilitate the operation of transactions.
- Reduce the difficulty of API development.
- Simple test, create objects through Spring
public class User {
public void add(){
System.out.println("add.................");
}
}
public class Test1 {
@Test
public void test1(){
//Read configuration file
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
//Gets the object created by the configuration
User user = (User) context.getBean("user");
//call
user.add();//add.................
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="user" class="com.oldking.User"></bean>
</beans>
2, IOC container
- What is IOC
- Control inversion, and the process of creating objects and calling between objects is managed by Spring.
- Purpose of using IOC: to reduce coupling.
- IOC underlying principle
- Xml parsing, factory mode, reflection
- Graphic IOC bottom layer
- Original mode: high code coupling
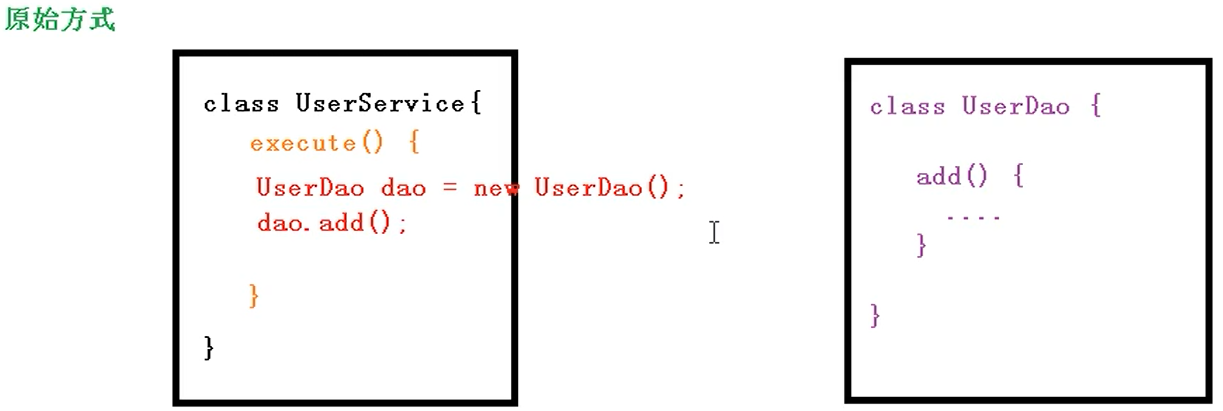
- Factory mode
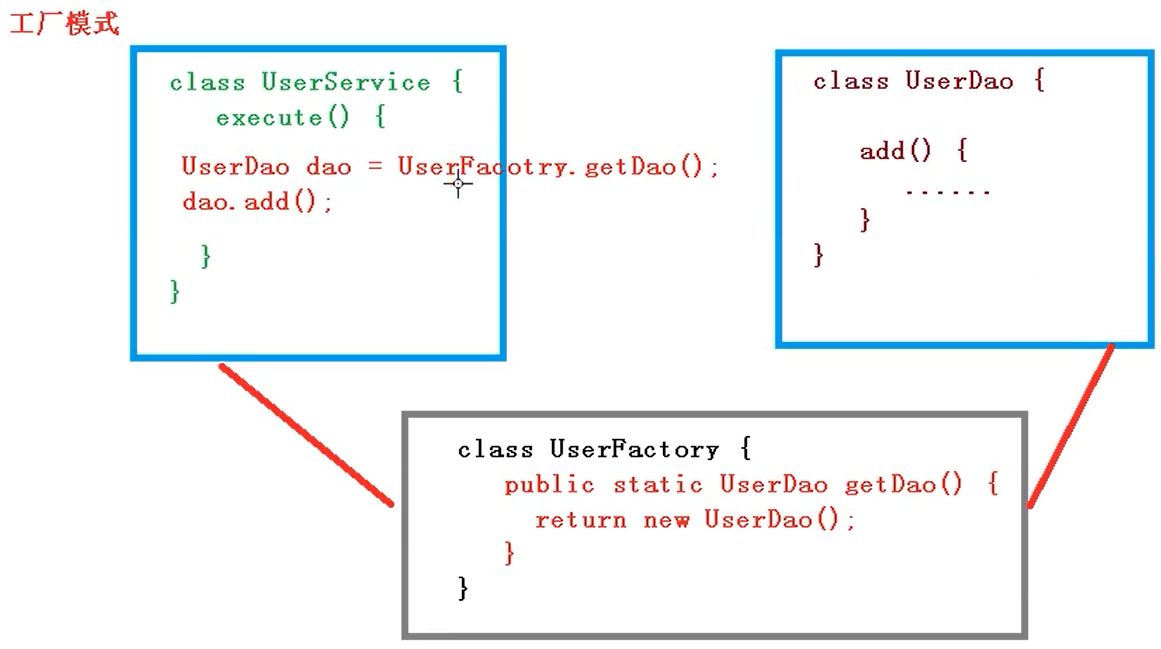
- Implementation process of control inversion (IOC)
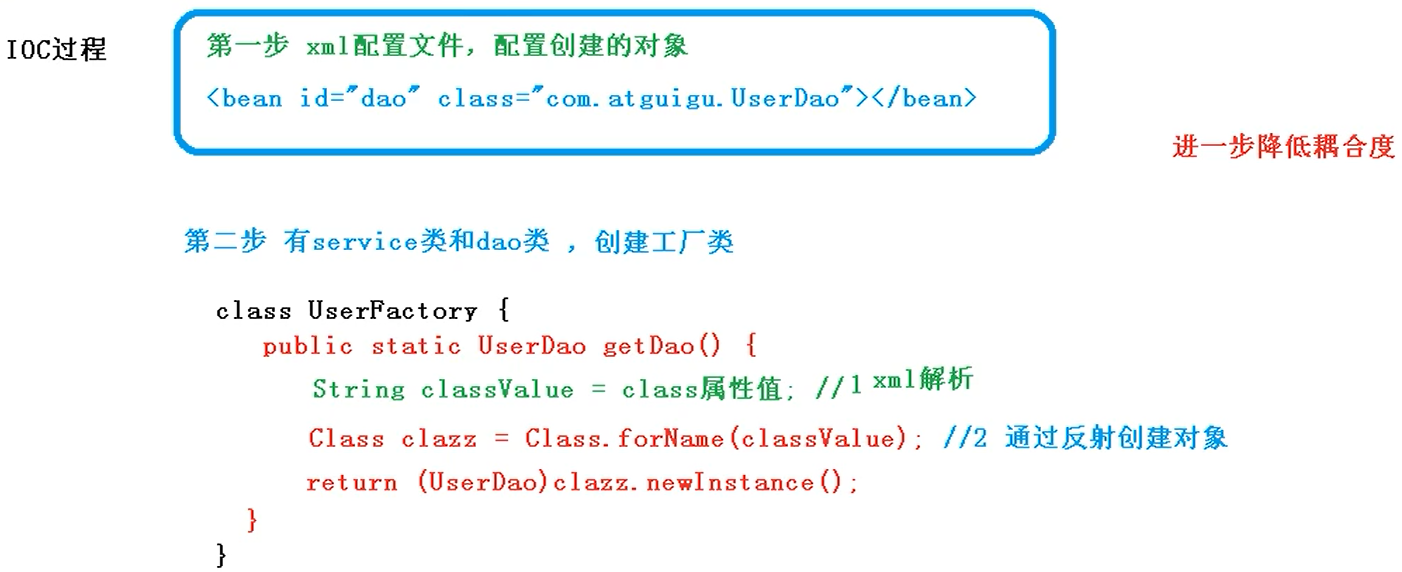
- IOC (Interface)
- The IOC idea is given to the IOC container. The bottom layer of the IOC container is the object factory.
- Spring provides two ways to implement IOC container: (two interfaces)
- BeanFactory: the basic implementation of IOC container, which is the internal use interface of Spring and is not provided for developers to use.
The object will not be created when the configuration file is loaded, but only when the object is obtained (used). - ApplicationContext: the sub interface of BeanFactory interface, which provides more and more powerful functions. Used by developers
Objects are created when the configuration file is loaded.
- Implementation class of ApplicationContext
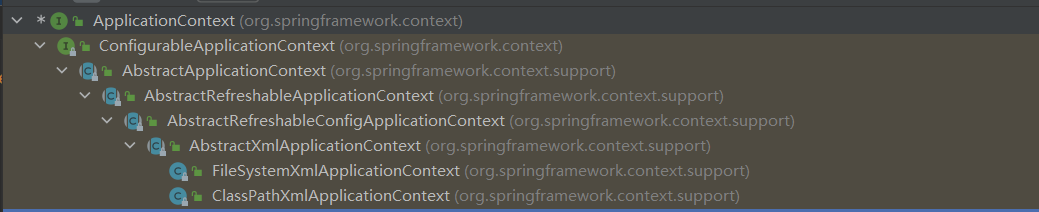
- IOC management Bean
- What is Bean Management: Bean management refers to two operations.
- Spring creates objects.
- Spring injection properties.
- There are two ways to manage beans
- Based on xml configuration file.
- The way in which comments are given.
- DI: dependency injection, i.e. injection attribute. It is the implementation of IOC.
- IOC manages beans based on xml configuration files
- Creating objects based on xml
<bean id="user" class="com.oldking.User"></bean>
- In the Spring configuration file, use the Bean tag and add the corresponding attribute in the tag to realize the creation of dusion
- Introduction to bean tag attributes:
id: the unique identifier of the bean
Class: the path of the corresponding entity class - When creating an object, the parameterless construction method is executed by default to complete the creation of the object.
- Attribute injection based on xml
- Injection through set method
- Create a class and define its properties and corresponding set methods.
public class Student {
/*
* Create attribute
*/
private String name;
private Integer age;
/*
* Create the set method corresponding to the attribute
*/
public void setName(String name) {
this.name = name;
}
public void setAge(Integer age) {
this.age = age;
}
}
- Configure object creation and attribute injection in Spring configuration file.
<!--create object-->
<bean id="student" class="com.oldking.Student">
<!--set Method injection properties
name: Attribute name
value: Attribute value
-->
<property name="age" value="20"></property>
<property name="name" value="OldKing"></property>
</bean>
- test
@Test
public void test1(){
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
Student student = (Student) context.getBean("student");
System.out.println(student.toString());
}
- Injection through a parametric constructor
- Provide constructor with parameters
public class Student {
/*
* Create attribute
*/
private String name;
private Integer age;
public Student(String name, Integer age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
- Configure in the Spring configuration file
The name attribute in the constructor Arg tag can also be replaced by the index attribute. Index is used to specify the index of the formal parameter list. For example, index=0 represents the first parameter in the formal parameter list.<!--create object-->
<bean id="student" class="com.oldking.Student">
<!--Parametric constructor injection-->
<constructor-arg name="name" value="Oldking"></constructor-arg>
<constructor-arg name="age" value="22"></constructor-arg>
</bean>
- P namespace injection
<!--Create objects and pass P Namespace injection-->
<bean id="student" class="com.oldking.Student" p:name="OldKing" p:age="25"/>
- Inject null
<!--create object-->
<bean id="student" class="com.oldking.Student">
<property name="name">
<!--Inject null-->
<null></null>
</property>
</bean>
- Inject special symbols
<!--create object-->
<bean id="student" class="com.oldking.Student">
<!--Attribute values contain special symbols
1,Translate special characters
2,use XML of CDATA expression
-->
<!--<property name="name" value="<123>"></property>-->
<property name="name">
<value>
<![CDATA[<123>]]>
</value>
</property>
</bean>
- Injecting external bean s based on xml
public class Student {
/*
* Create attribute
*/
private String name;
private Integer age;
private Address address;
public void setName(String name) {
this.name = name;
}
public void setAge(Integer age) {
this.age = age;
}
public void setAddress(Address address) {
this.address = address;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", address=" + address +
'}';
}
}
public class Address {
private String country;
private String province;
private String city;
private String district;
private String msg;
public void setCountry(String country) {
this.country = country;
}
public void setProvince(String province) {
this.province = province;
}
public void setCity(String city) {
this.city = city;
}
public void setDistrict(String district) {
this.district = district;
}
public void setMsg(String msg) {
this.msg = msg;
}
@Override
public String toString() {
return "Address{" +
"country='" + country + '\'' +
", province='" + province + '\'' +
", city='" + city + '\'' +
", district='" + district + '\'' +
", msg='" + msg + '\'' +
'}';
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="student" class="com.oldking.Student">
<property name="name" value="OldKing"></property>
<property name="age" value="25"></property>
<!--use ref Attribute receive external bean-->
<property name="address" ref="address"></property>
</bean>
<bean name="address" class="com.oldking.Address">
<property name="country" value="China"></property>
<property name="province" value="Shandong"></property>
<property name="city" value="Jinan"></property>
<property name="district" value="High tech Zone"></property>
<property name="msg" value="123456"></property>
</bean>
</beans>
- Injecting internal bean s and cascading assignment based on xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="student" class="com.oldking.Student">
<property name="name" value="OldKing"></property>
<property name="age" value="25"></property>
<!--Injection inside bean-->
<property name="address">
<bean name="address" class="com.oldking.Address">
<property name="country" value="China"></property>
<property name="province" value="Shandong"></property>
<property name="city" value="Jinan"></property>
<property name="district" value="High tech Zone"></property>
<property name="msg" value="123456"></property>
</bean>
</property>
</bean>
</beans>
- Inject array, List and Map types based on xml
public class Stu {
private String[] courses;
private List<String> list;
private Set<String> set;
private Map<String, String> map;
public void setCourses(String[] courses) {
this.courses = courses;
}
public void setList(List<String> list) {
this.list = list;
}
public void setMap(Map<String, String> map) {
this.map = map;
}
public void setSet(Set<String> set) {
this.set = set;
}
@Override
public String toString() {
return "Stu{" +
"courses=" + Arrays.toString(courses) +
", list=" + list +
", set=" + set +
", map=" + map +
'}';
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class="com.oldking.Test.Stu" id="stu">
<!--Injection array-->
<property name="courses">
<array>
<value>English</value>
<value>language</value>
<value>mathematics</value>
</array>
</property>
<!--injection List-->
<property name="list">
<list>
<value>123</value>
<value>456</value>
<value>789</value>
</list>
</property>
<!--injection Set-->
<property name="set">
<set>
<value>a</value>
<value>b</value>
<value>c</value>
<value>d</value>
<value>e</value>
</set>
</property>
<!--injection Map-->
<property name="map">
<map>
<entry key="k1" value="v1"></entry>
<entry key="k2" value="v2"></entry>
<entry key="k3" value="v3"></entry>
</map>
</property>
</bean>
</beans>
- IOC container management factory bean (FactoryBean)
- There are two kinds of Spring beans, one is an ordinary Bean and the other is a workshop Bean.
Ordinary bean: the bean type defined in the configuration file is the returned type.
Factory Bean: the type defined in the configuration file can be different from the return type. - code
public class Stu implements FactoryBean {
@Override
public Object getObject() throws Exception {
return new Object();
}
@Override
public Class<?> getObjectType() {
return null;
}
}
<bean class="com.oldking.Test.Stu" id="stu"></bean>
- Scope of Bean
- In Spring, beans are singleton objects by default.
@Test
public void test1(){
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
Object o = context.getBean("stu");
Object o1 = context.getBean("stu");
System.out.println(o.toString());//com.oldking.Student@6a1aab78
System.out.println(o1.toString());//com.oldking.Student@6a1aab78
}
- How to set single instance and multiple instances
The scope attribute is used for configuration in the bean tag. It has two commonly used attribute values: prototype multi instance and singleton single instance (default). <bean class="com.oldking.Student" id="stu" scope="prototype">
<property name="address">
<bean class="com.oldking.Address">
<property name="msg" value="123"></property>
<property name="province" value="456"></property>
</bean>
</property>
</bean>
@Test
public void test1(){
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
Object o = context.getBean("stu");
Object o1 = context.getBean("stu");
System.out.println(o.toString());//com.oldking.Student@eafc191
System.out.println(o1.toString());//com.oldking.Student@612fc6eb
}
- The difference between prototype and singleton
- singleton is a single instance. prototype is multi instance.
- When the value of scope is set to singleton, the object will be created when the spring configuration file is loaded.
When the value of scope is set to prototype, the object will be created only when the getBean method is called.
- Bean life cycle
- Create a Bean instance through the constructor (parameterless construction).
- Set values for the properties of the bean instance and references to other beans (call the set method).
- Call the initialization method of Bean (the method that needs configuration initialization).
- Bean is ready to use (object creation completed).
- When the container is closed, call the Bean's destruction method (the method that needs to be configured for destruction).
public class Order {
private String oName;
public void setoName(String oName) {
this.oName = oName;
System.out.println("by Bean Set values for the properties of the instance and for other bean Reference (call) of Set Method)");
}
public Order() {
System.out.println("Create by constructor Bean Instance (parameterless construction)");
}
public void init(){
/*bean Configure the init method attribute in the tag as the method name*/
System.out.println("call Bean Initialization method of (method requiring configuration initialization)");
}
public void destroy(){
/*bean Configure the destroy method attribute in the tag to this method name*/
System.out.println("Called when the container is closed Bean Destruction method of (method requiring configuration destruction)");
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="order" class="com.oldking.bean.Order" init-method="init" destroy-method="destroy">
<property name="oName" value="phone"></property>
</bean>
</beans>
@Test
public void test1(){
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
Order o = (Order) context.getBean("order");
System.out.println("Bean Ready to use (object creation completed)");
System.out.println(o);
((ClassPathXmlApplicationContext)context).close();
/*
* After running, the following contents are output in sequence
* Create Bean instance through constructor (parameterless construction)
* Set values for the properties of bean instances and references to other beans (call the set method)
* Call the initialization method of Bean (the method that needs configuration initialization)
* Bean Ready to use (object creation completed)
* com.oldking.bean.Order@e720b71
* When the container is closed, call the Bean's destruction method (the method that needs to be configured for destruction)
*
* */
}
- Post processor of Bean
- Before the third step of the bean's life cycle, the bean instance will be passed to the postProcessBeforeInitialization method of the bean post processor.
- After the third step of the bean's life cycle, the bean instance is passed to the postprocessor's postProcessAfterInitialization method.
- Code demonstration
public class MyBeanProcessor implements BeanPostProcessor {
@Override
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
System.out.println("Execute before initialization");
return bean;
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
System.out.println("Execute after initialization");
return bean;
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="order" class="com.oldking.bean.Order" init-method="init" destroy-method="destroy">
<property name="oName" value="phone"></property>
</bean>
<!--Configure post processor-->
<bean class="com.oldking.bean.MyBeanProcessor" id="bean"></bean>
</beans>
@Test
public void test1(){
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
Order o = (Order) context.getBean("order");
System.out.println("Bean Ready to use (object creation completed)");
System.out.println(o);
((ClassPathXmlApplicationContext)context).close();
/*
* Create Bean instance through constructor (parameterless construction)
* Set values for the properties of bean instances and references to other beans (call the set method)
* Execute before initialization
* Call the initialization method of Bean (the method that needs configuration initialization)
* Execute after initialization
* Bean Ready to use (object creation completed)
* com.oldking.bean.Order@74ad1f1f
* When the container is closed, call the Bean's destruction method (the method that needs to be configured for destruction)
*
* */
}
- xml Automatic Assembly
- What is automatic assembly
spring automatically injects the matching attribute values according to the specified assembly rules (attribute name or attribute type). - How to configure
bean tag attribute autowire, configure automatic assembly.
autowire has two attribute values:
- byName: injected according to the attribute name. The Id value of the injected bean is required to be the same as the class attribute name.
- byType: injected according to the attribute type, but only by one instance of the corresponding attribute type.
- Demonstrate the automatic assembly process
public class Dept {
}
public class Emp {
private Dept dept;
public void setDept(Dept dept) {
this.dept = dept;
}
@Override
public String toString() {
return "Emp{" +
"dept=" + dept +
'}';
}
}
<bean id="emp" class="com.oldking.beanautowire.Emp" autowire="byName"></bean>
<bean id="dept" class="com.oldking.beanautowire.Dept"></bean>
- xml configuration external property file (such as database connection pool configuration)
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd\
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!--Import external files-->
<context:property-placeholder location="jdbc.properties"/>
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${prop.driverClass}"></property>
<property name="url" value="${prop.url}"></property>
<property name="username" value="${prop.userName}"></property>
<property name="password" value="${prop.pwd}"></property>
</bean>
</beans>
External profile:prop.driverClass=com.mysql.cj.jdbc.Driver
prop.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&zeroDateTimeBehavior=convertToNull&useSSL=true&serverTimezone=GMT%2B8
prop.userName=root
prop.pwd=root
- Annotation based management of bean s
- Annotations for Bean management in Spring
- @Component: general annotation
- @Service: for business layer
- @Controller: for Web tier
- @Repository: for persistence layer
The above four annotation functions are the same and can be used to create Bean instances.
- Create objects based on annotations
- Introduce related dependencies

- Turn on component scanning
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!--Turn on component scanning-->
<context:component-scan base-package="com.oldking"></context:component-scan>
</beans>
- Create a class and add annotations to create objects on the class.
@Controller //< bean id = class name initial lowercase >
public class Test1 {
private String name;
public void show(){
System.out.println("123456");
}
}
- Configuration related to component scanning
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!--<!–Turn on component scanning–>
<!–use-default-filters="false"Indicates that the default is not used now filter,Use custom–>
<context:component-scan base-package="com.oldking" use-default-filters="false">
<!–definition filter,Specify what to scan–>
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
</context:component-scan>-->
<!--Turn on component scanning-->
<context:component-scan base-package="com.oldking">
<!--definition filter,Specify what to scan-->
<context:exclude-filter type="annotation" expression="org.springframework.stereotype.Service"/>
</context:component-scan>
</beans>
- Attribute injection based on annotation
- @AutoWired: auto assemble according to attribute type
- @Qualifier: inject according to attribute name
- @Resource: can be injected according to type or name
- @value: inject common type attribute
Code demonstration@Component
public class Address {
private String city;
public void show(){
System.out.println("I won't tell you the address..........");
}
}
@Service
public class Student {
// @Autowired injects by type
// @Qualifier(value = "address") is injected according to the name and needs to be used with @ Autowired
// @Resource injects annotations into JavaX according to the type, which is not recommended
// @Resource(name = "address") is injected according to the name
@Autowired
private Address address;
@Value(value = "OldKing") //Inject common attributes
private String name;
public void study(){
System.out.println("study......." + name);
address.show();
}
}
- Fully annotated development
Create a Spring configuration class to replace the xml configuration@Configuration
@ComponentScan(basePackages = {"com.oldking"})
public class SpringConfig {
}
@Test
public void test1(){
ApplicationContext context = new AnnotationConfigApplicationContext(SpringConfig.class);
Student test1 = (Student) context.getBean("student", Student.class);
System.out.println(test1);
test1.study();
}
Keywords:
Spring
Added by dragonusthei on Fri, 28 Jan 2022 18:04:33 +0200