Article catalog
- 1, Response data and results view
- 2, Spring MVC file upload
- 3, Spring MVC exception handling
- 4, Spring MVC interceptor
1, Response data and results view
Dark horse programmer spring MVC Day2 part
1.1 return value classification
1.1.1 return string
import cn.gorit.entity.User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import javax.servlet.ServletException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.io.PrintWriter; @Controller @RequestMapping("/user") public class UserController { // Return string @RequestMapping("/testString") public String testString(Model model) { System.out.println("testString Yes"); User user = new User(); // Entity class User user.setUsername("to one's heart's content"); user.setPassword("123321"); user.setAge(18); model.addAttribute("user",user); return "success"; } } // After the previous processing by the view parser, you will jump to the success.jsp interface <h5>1, Return string</h5> full name: ${user.username}</br> password: ${user.password}</br> Age: ${user.age}
1.1.2 return void
/** * Returns null. If there is no return value, * testVoid.jsp will be requested by default, and the result is 404 * Request forwarding is a request * Redirection is two requests */ @RequestMapping("/testVoid") public void testVoid(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("testVoid Yes"); // 1. Prepare the procedure for request forwarding // request.getRequestDispatcher("/WEB-INF/pages/success.jsp").forward(request,response); // 2. Redirection // response.sendRedirect("../index.jsp"); // 3. Direct corresponding PrintWriter out = response.getWriter(); request.setCharacterEncoding("utf-8"); response.setCharacterEncoding("gbk"); // Solve Chinese garbled code response.setContentType("text/html;charset=gbk"); out.print("Hello"); out.flush(); out.close(); return; }
1.1.3 the return value is ModelAndView object
// Is consistent with the returned string @RequestMapping("/testModelAndView") public ModelAndView testModelAndView() { System.out.println("testModelAndView Yes"); //Spring provides ModelAndView mv = new ModelAndView(); User user = new User(); user.setUsername("breeze"); user.setPassword("456"); user.setAge(18); // Storing the user object in the mv object will also store the user object in the request object mv.addObject("user",user); // Which page to jump to mv.setViewName("success"); return mv; }
1.2 forwarding and redirection
/** * Forward or redirect using keywords * */ @RequestMapping("/testForwardOrRedirect") public String testForwardOrRedirect() { System.out.println("testForwardOrRedirect Yes"); // Forwarding of requests // return "forward:/WEB-INF/pages/success.jsp"; // Redirect (return to root) return "redirect:/index.jsp"; }
1.3 ajax response json string
- Add json parsing dependency in pom.xml
<dependency> <groupId>com.fasterxml.jackson.coregroupId> <artifactId>jackson-databindartifactId> <version>2.10.0version> dependency> <dependency> <groupId>com.fasterxml.jackson.coregroupId> <artifactId>jackson-coreartifactId> <version>2.10.0version> dependency> <dependency> <groupId>com.fasterxml.jackson.coregroupId> <artifactId>jackson-annotationsartifactId> <version>2.10.0version> dependency>
- Writing front-end ajax requests
<button id="btn">send out ajax</button> <script type="text/javascript"> // Page loading, binding click events $(function () { $("#btn").click(function () { // alert("Hello"); $.ajax({ url:"user/testAjax", type:"post", contentType:"application/json;charset=UTF-8", data:'{"username":"hehe","password":"122","age":30}', // Transfer json data dataType:"json", success:function (data) { // Data is the json data responded by the server, which is parsed alert(data); alert(data.username); alert(data.password); alert(data.age); } }) }) }) </script>
- ajax response (back-end processing)
// Simulate asynchronous request response @RequestMapping("/testAjax") public @ResponseBody User testAjax(@RequestBody User user) { System.out.println("testAjax Yes"); // The ajax request sent by the client is received, and the json string is passed. The back-end encapsulates the json string into the user object System.out.println(user); // Get the json string and process {"username":"hehe","password":"122","age:30} accordingly // Respond and simulate query database user.setUsername("hehe"); user.setAge(40); // Respond return user; }
2, Spring MVC file upload
2.1 file upload
- Prerequisites for file upload
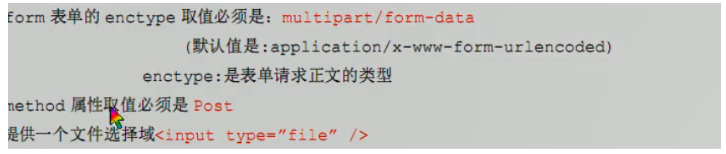
2.2 uploading files in traditional way
Upload dependent jar packages
<dependency> <groupId>commons-fileuploadgroupId> <artifactId>commons-fileuploadartifactId> <version>1.3.3version> dependency> <dependency> <groupId>commons-iogroupId> <artifactId>commons-ioartifactId> <version>2.5version> dependency>file
File upload front end code
<h3>File upload h3> <form action="user/fileupload1" method="post" enctype="multipart/form-data"> <input type="file" name="upload"/><br> <input type="submit" value="upload"> form>
Backend controller
@Controller @RequestMapping("/user") public class UserController { @RequestMapping("/fileupload1") public String fileUpload(HttpServletRequest request) throws Exception { System.out.println("File upload..."); // Use fileupload to complete file upload String path = request.getSession().getServletContext().getRealPath("/uploads/"); // Determine whether the path exists File file = new File(path); if (!file.exists()) { // Create this folder file.mkdirs(); } // Parse the request object to obtain the uploaded file item DiskFileItemFactory factory = new DiskFileItemFactory(); ServletFileUpload upload = new ServletFileUpload(factory); // Parse request List<FileItem> items = upload.parseRequest(request); // ergodic for (FileItem item: items) { // Judge whether the current item object is an uploaded file item if (item.isFormField()) { // Normal form items } else { // Upload file item // Gets the name of the uploaded file String fileName = item.getName(); // Set each file name to a unique value, uuid String uuid = UUID.randomUUID().toString().replace("-",""); fileName = uuid +"_"+ fileName; // Complete file upload item.write(new File(path,fileName)); // Delete temporary file item.delete(); } } return "success"; } }
2.3 file upload provided by spring MVC
Upload principle
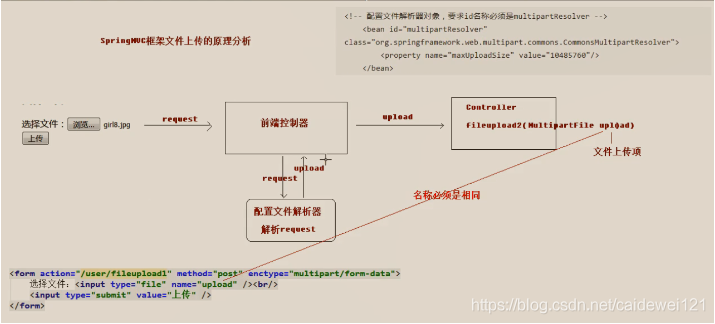
Front page
<h3>File upload SpringMVCh3> <form action="user/fileupload2" method="post" enctype="multipart/form-data"> <input type="file" name="upload"/><br> <input type="submit" value="upload"> form>
Springmvc.xml
Add a file parser
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <property name="maxUploadSize" value="10240" /> bean>
Written by controller
// Spring MVC file upload @RequestMapping("/fileupload2") public String fileupload2(HttpServletRequest request, MultipartFile upload) throws Exception { System.out.println("File upload..."); // Use fileupload to complete file upload String path = request.getSession().getServletContext().getRealPath("/uploads/"); // Determine whether the path exists File file = new File(path); if (!file.exists()) { // Create this folder file.mkdirs(); } // Upload file item // Gets the name of the uploaded file String fileName = upload.getOriginalFilename(); // Set each file name to a unique value, uuid String uuid = UUID.randomUUID().toString().replace("-",""); fileName = uuid +"_"+ fileName; // Complete file upload upload.transferTo(new File(path,fileName)); return "success"; }
2.4 cross server file upload
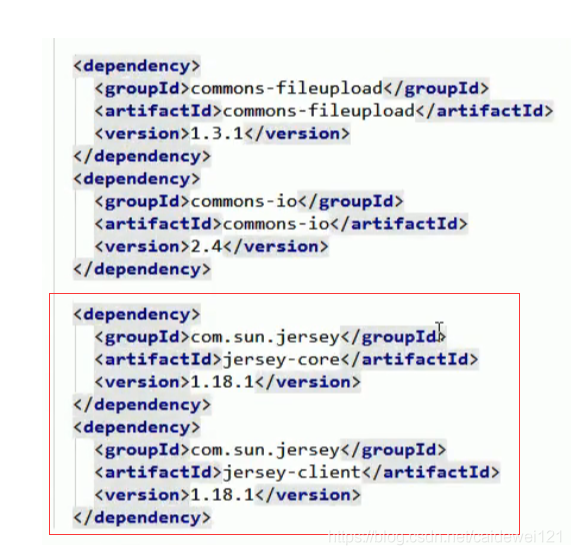
Add a jar package, open two Tomcat servers, and upload files across servers using different ports
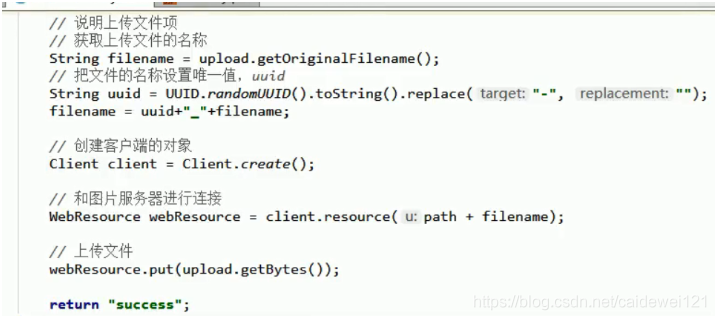
3, Spring MVC exception handling
3.1 exception handling ideas
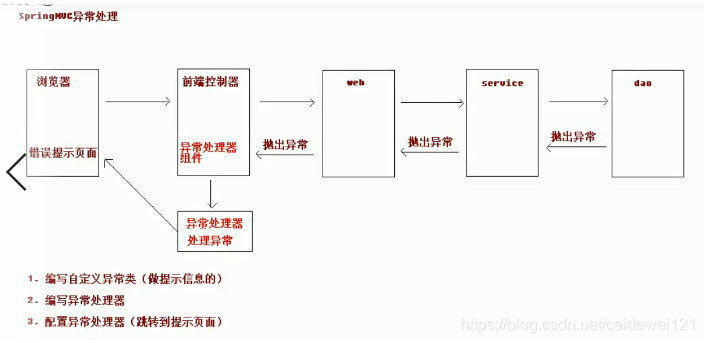
3.2 handling exceptions
I don't know why I always report 500 errors here
- Write a custom exception class (for prompt information)
- Write exception handler
- Configure exception handler (jump to display page)
Front jump page
<h3>exception handling h3> <a href="user/testException">exception handling a>
Exception handling controller writing
@Controller @RequestMapping("/user") public class UserController { // exception handling @RequestMapping("/testException") public String testException() throws SysException { System.out.println("testException. . . "); try { // Simulation anomaly int a = 10/0; } catch (Exception e) { // Print exception information e.printStackTrace(); // Throw custom exception information throw new SysException("An error occurred for all users queried"); } return "success"; } }
Custom exception handler
package cn.gorit.exception; import org.springframework.web.servlet.HandlerExceptionResolver; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Exception handler * */ public class SysExceptionResolver implements HandlerExceptionResolver { /** * Business logic for handling exceptions * */ @Override public ModelAndView resolveException(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) { // Get exception object SysException e = null; if (ex instanceof SysException) { e = (SysException)ex; } else { e = new SysException("The system is being maintained"); } // Create ModelAndView object ModelAndView mv = new ModelAndView(); mv.addObject("errorMsg", e.getMessage()); mv.setViewName("error"); // To jump to the page, remember to write an error.jsp under WEB-INF/pages return mv; } }
package cn.gorit.exception; /** * Custom exception class * */ public class SysException extends Exception{ // Storage prompt new private String message; public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public SysException(String message) { this.message = message; } }
springmvc.xml configuration
<bean id="sysExceptionResolver" class="cn.gorit.exception.SysExceptionResolver">bean>
4, Spring MVC interceptor
4.1 interceptor function
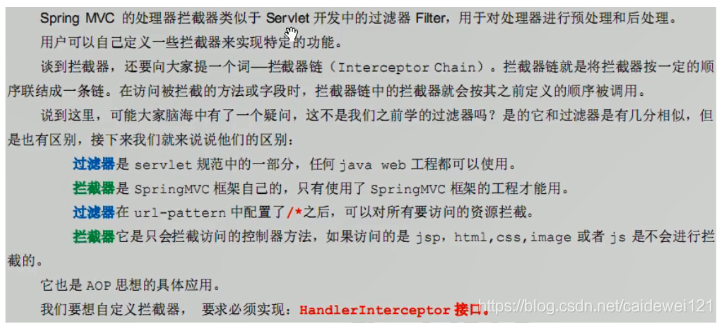
4.2 writing interceptors
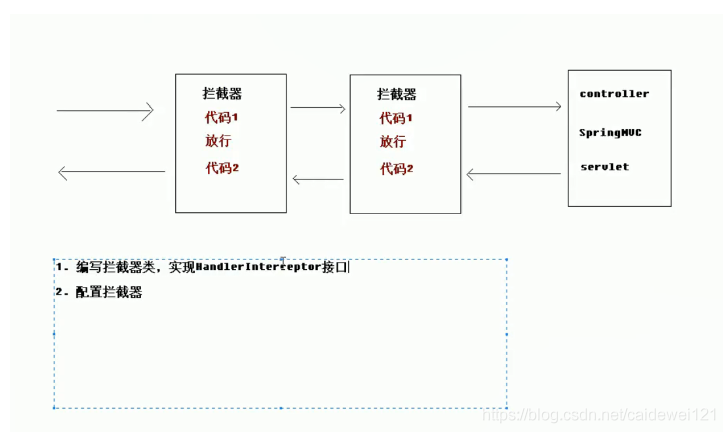
4.3 interceptor preparation
- Front end interface jump
<h3>Interceptor h3> <a href="test/testIntercepter">Interceptor a>
- Written by back-end controller
package cn.gorit.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller @RequestMapping("/test") public class TestController { /** * Interceptor * */ @RequestMapping("/testIntercepter") public String testIntercepter() { System.out.println("testIntercepter. . . "); return "success"; } }
- Interceptor class writing
package cn.gorit.interceptor; import org.springframework.web.servlet.HandlerInterceptor; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * custom interceptor * */ public class MyInterceptor implements HandlerInterceptor { /** * Pretreatment * return true Indicates release, and executes the next interceptor. If not, executes the controller method * */ @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out.println("The interceptor executed"); return true; } /** * Post processing method, after the controller method is executed and before the success.jsp is executed * */ @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { System.out.println("The interceptor executed,succes.jsp Before loading"); } /** * success.jsp If executed, the method will execute * */ @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { System.out.println("The interceptor executed success.jsp after"); } }
- Interceptor springmvc.xml configuration
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:component-scan base-package="cn.gorit">context:component-scan> <bean id="internalResourceViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/pages/">property> <property name="suffix" value=".jsp">property> bean> <mvc:resources mapping="/js/**" location="/js/">mvc:resources> <mvc:resources mapping="/css/**" location="/csss/">mvc:resources> <mvc:resources mapping="/images/**" location="/images/">mvc:resources> <mvc:interceptors> <mvc:interceptor> <mvc:mapping path="/**"/> <bean class="cn.gorit.interceptor.MyInterceptor">bean> mvc:interceptor> mvc:interceptors> <mvc:annotation-driven/> beans>
Operation effect
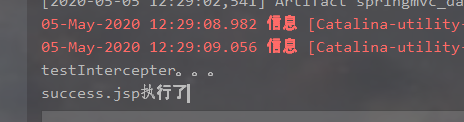