outline
Introduction to Spring
II. Spring's IoC Actual Warfare
III. Summary of Common Annotations of IoC
IV. Project Source Code and Reference Download
5. Reference Articles
Introduction to Spring
1. What is Spring
Spring is a lightweight open source framework for layered Java SE/EE application full-stack. With IoC (Inverse Of Control) and AOP (Aspect Oriented Programming: Aspect Oriented Programming) as the core, Spring MVC, persistent Spring JDBC, business transaction management and other enterprise-level application technologies are provided. It can also integrate many famous open source applications in the world. Tripartite frameworks and class libraries have gradually become the most widely used open source framework for Java EE enterprise applications.
2. Spring Advantage
Convenient decoupling and simplified development
_Through the IoC container provided by Spring, the dependencies between objects can be controlled by Spring to avoid excessive program coupling caused by hard coding. Users no longer need to write code for such very low-level requirements as singleton schema classes, attribute file parsing, etc. They can focus more on the upper application.
AOP programming support
_Through Spring's AOP function, it is convenient to program face-to-face. Many functions that are not easy to implement with traditional OOP (object-oriented programming) can be easily handled by AOP.
Declarative transaction support
_can free us from tedious transaction management code, flexible transaction management through declarative way, improve the efficiency and quality of development.
Convenient program testing
_can use non-container-dependent programming for almost all testing work. Testing is no longer an expensive operation, but can be done at will.
Convenient integration of excellent frameworks
Spring can reduce the difficulty of using various frameworks and provide direct support for various excellent frameworks (Struts, Hibernate, Hessian, Quartz, etc.).
Reducing the difficulty of using Java EE API
_Spring has a thin packaging layer for Java EE APIs (such as JDBC, JavaMail, remote calls, etc.), which greatly reduces the difficulty of using these APIs.
3. spring's architecture
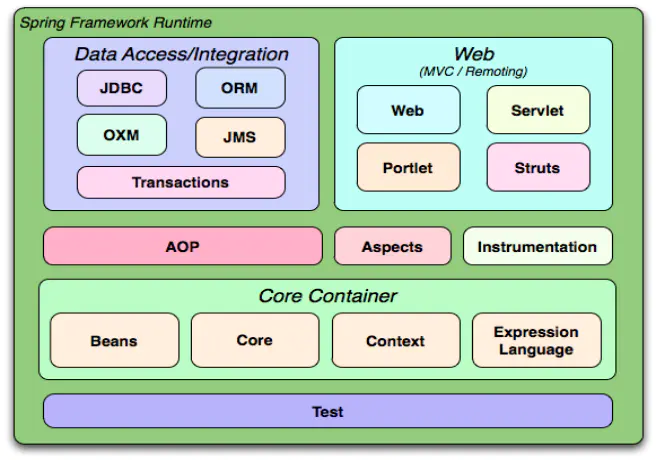
II. Spring's IOC Actual Warfare
Inversion of Control (IoC) is object-oriented programming A design principle that can be used to reduce the number of computer codes Coupling degree . One of the most common methods is called Dependency Injection (DI), and another is called Dependency Lookup. By controlling inversion, when an object is created, a reference to the object on which it depends is passed by an external entity that controls all objects in the system. In other words, dependencies are injected into objects.
There are two ways to implement IoC. One is configuration file (bean management), which includes using class parametric construction method (emphasis), using static factory to create and using instance factory to create. The second is annotation.
1. Create projects
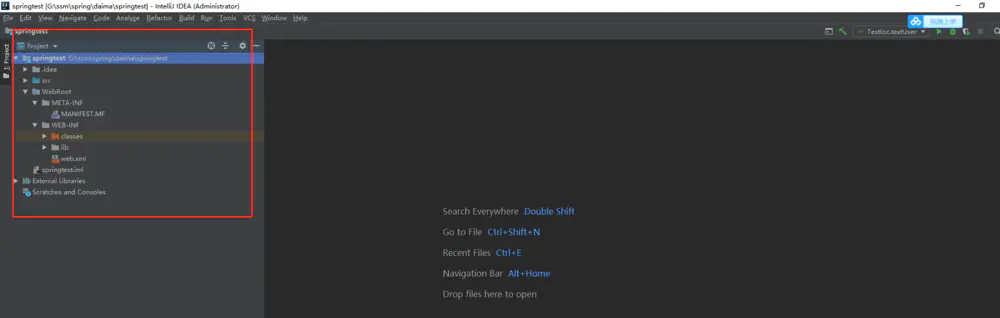
2. Adding jar packages (using maven in actual development)
Copy the jar package to the following folder
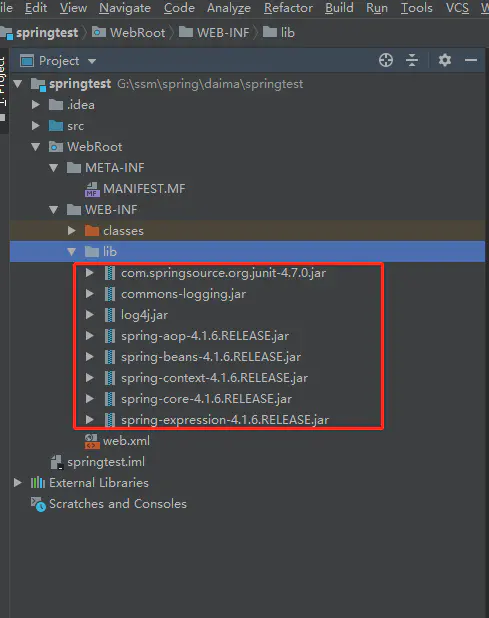
Set dependency
Specific ways can refer to the address in the blog: https://zyjustin9.iteye.com/blog/2172445
3. Implementation of Configuration File (bean Management)
Way 1: Use id configuration method -- commonly used (important)
Create the test class User.java
public class User {
public void add() {
System.out.println("add.......");
}
public void add(String haha)
{
System.out.println("add.."+haha);
}
}
Create configuration file myXml.xml under src
<?xml version="1.0" encoding="UTF-8"?> <! - These are all ways of writing death - >. <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd" > <!-- Originally, there was also an attribute name in the configuration file. Its function is the same as that of id. The value of id attribute can not contain special symbols (such as @#), but the name attribute can. However, the name attribute was used in earlier versions and is now replaced by id. The scope attribute, which has the following values in total, is mainly used for the first two, the latter two do not need to remember that we did not write the scope attribute in this configuration file, the default is singleton. Singleton: default, singleton prototype: Multiple cases: Request: Create objects and place them in the request domain Session: Create an object and place it in the session domain GlobalSession: Create objects and place them in the globalSession domain --> <! - The first method: ioc's configuration file id is the symbol of the class, class is the full path of the object class - > the <bean id="user" class="ioc1.User" scope="singleton"/> </beans>
Write test code
/**
* Three Methods of Implementing IOC by Test Configuration
*
* @author Wu Xiao Chang
*
*/
public class TestIoc {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("myXml.xml");
//Method 1: Use id configuration method -- commonly used
User user = (User) context.getBean("user");
System.out.println(user);
user.add("Fuck");
}
}
The results are as follows:
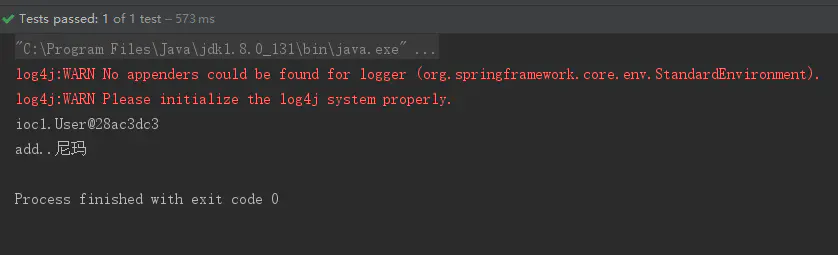
Mode 2: Static factories (just know)
Create test class User2.java
package ioc2;
public class User2 {
public void add() {
System.out.println("user2........");
}
}
Create the test factory class User2Factory.java
package ioc2;
public class User2Factory {
public static User2 getUser2()
{
return new User2();
}
}
Create configuration file myXml.xml under src
<?xml version="1.0" encoding="UTF-8"?> <! - These are all ways of writing death - >. <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd" > <!-- Originally, there was also an attribute name in the configuration file. Its function is the same as that of id. The value of id attribute can not contain special symbols (such as @#), but the name attribute can. However, the name attribute was used in earlier versions and is now replaced by id. The scope attribute, which has the following values in total, is mainly used for the first two, the latter two do not need to remember that we did not write the scope attribute in this configuration file, the default is singleton. Singleton: default, singleton prototype: Multiple cases: Request: Create objects and place them in the request domain session: Create objects and place them in session Inside the domain GlobalSession: Create objects and place them in the globalSession domain --> <! - The second method is to create objects using static factories - >. <bean id="user2" class="ioc2.User2Factory" factory-method="getUser2"/> </beans>
The test code is as follows:
package introduction;
import ioc1.User;
import ioc2.User2;
import ioc3.User3;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* Three Methods of Implementing IOC by Test Configuration
*
* @author Wu Xiao Chang
*
*/
public class TestIoc {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("myXml.xml");
//Method 2: Use the static factory approach -- just know it.
User2 user2 = (User2) context.getBean("user2");
System.out.println(user2);
}
}
The results are as follows:
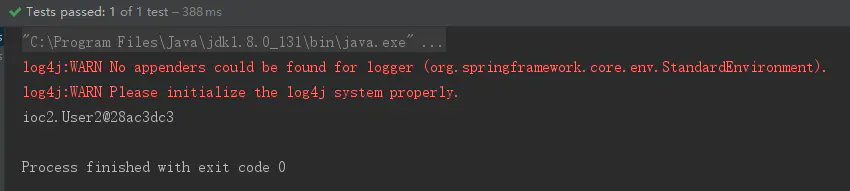
Way 3: Example factory (just understand)
Create test class User3.java
package ioc3;
public class User3 {
public void add() {
System.out.println("user3........");
}
}
Create the test factory class User3Factory.java
package ioc3;
public class User3Factory {
//Common method, returning the User3 object
public User3 getUser3()
{
return new User3();
}
}
Create configuration file myXml.xml under src
<?xml version="1.0" encoding="UTF-8"?> <! - These are all ways of writing death - >. <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd" > <!-- Originally, there was also an attribute name in the configuration file. Its function is the same as that of id. The value of id attribute can not contain special symbols (such as @#), but the name attribute can. However, the name attribute was used in earlier versions and is now replaced by id. The scope attribute, which has the following values in total, is mainly used for the first two, the latter two do not need to remember that we did not write the scope attribute in this configuration file, the default is singleton. Singleton: default, singleton prototype: Multiple cases: Request: Create objects and place them in the request domain session: Create objects and place them in session Inside the domain GlobalSession: Create objects and place them in the globalSession domain --> <! - The third method is to create objects using instance factories - >. <! - Objects to create factory classes <bean id="User3Factory" class="ioc3.User3Factory"></bean> <bean id="user3" factory-bean="User3Factory" factory-method="getUser3"></bean> </beans>
The test code is as follows:
package introduction;
import ioc3.User3;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* Three Methods of Implementing IOC by Test Configuration
*
* @author Wu Xiao Chang
*
*/
public class TestIoc {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("myXml.xml");
//Method 3: Create objects using instance factories -- just understand
User3 user3 = (User3) context.getBean("user3");
System.out.println(user3);
}
}
The results are as follows:
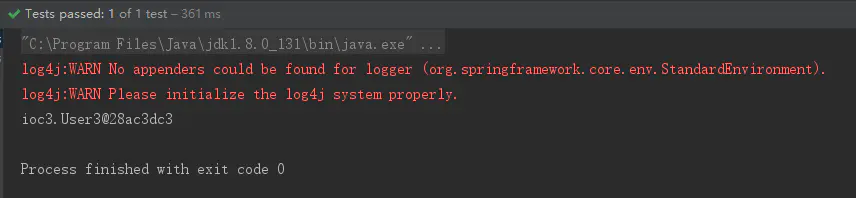
4. Implementation of Annotation
Way 1: Implementing Object Creation
Create test classes: UserBean1.java
/**
* Completing ioc by annotation
*
* @author Wu Xiao Chang
*
*/
//spring currently has four annotations, all of which have the same function and are used to create objects.
//@Component @Controller @Service @Repository
@Component(value="userBean1")//This corresponds to <bean id="user" class="/>
public class UserBean1 {
public void add() {
System.out.println("UserBean1....add");
}
}
Create configuration file bean.xml under src
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!-- bean definitions here --> <!-- Open the annotation to scan the package name written by base-package. If the class is in more than one package, the way to write it is as follows ioc_bean1,ioc_bean2,ioc_bean3... Or cn is used to represent all packages starting with cn, cn.ioc is used to represent all packages starting with cn.ioc. --> <! - Scan the package for annotations on classes, methods and attributes - > <context:component-scan base-package="ioc_bean1"></context:component-scan> <! - Scan only the annotations above the attributes - > <!--<context:annotation-config></context:annotation-config> --> </beans>
The test code is as follows
package introduction;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import ioc_bean1.UserBean1;
import ioc_bean1.UserService;;
/**
* Test bean s using IOC
*
* @author Wu Xiao Chang
*
*/
public class TestBean {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("bean.xml");
//Test bean configuration to create objects
UserBean1 userBean1 = (UserBean1) context.getBean("userBean1");
System.out.println(userBean1);
userBean1.add();
}
}
The results are as follows.
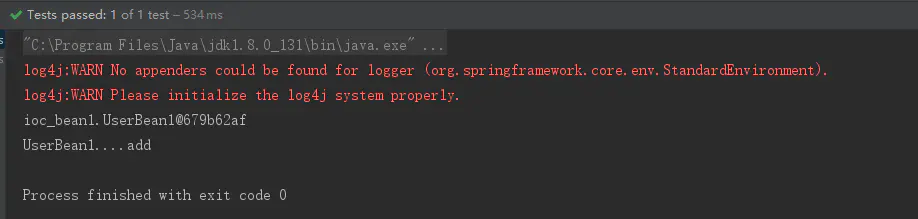
Mode 2: bean configuration injects object attributes
Create a test class: UserDao.java
import org.springframework.stereotype.Component;
//This step is equivalent to creating a UserDao object
@Component(value="userDao")
public class UserDao {
public void add() {
System.out.println("UserDao....add");
}
}
Write the test class UserService.java
package ioc_bean1;
import javax.annotation.Resource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
//@ Service(value="userService") is equivalent to @Service("userService")
//This step is equivalent to creating a UserService object
@Service(value="userService")
public class UserService {
//Get the UserDao object using the annotation method without using the set method, directly to the UserDao object using annotations, complete the object injection.
//That is, @Autowire is used to inject attributes
@Autowired
private UserDao userDao;
//This approach achieves the same effect as the above one. The following are commonly used
//The value of name should be consistent with the value of the UserDao annotation
// @Resource(name="userDao")
// private UserDao userDao;
public void add() {
System.out.println("UserService...add....");
userDao.add();
}
}
Configuration and mode are consistent under src
The test code is as follows:
package introduction;
import ioc_bean1.UserBean1;
import ioc_bean1.UserService;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
;
/**
* Test bean s using IOC
*
* @author Wu Xiao Chang
*
*/
public class TestBean {
@Test
public void testUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("bean.xml");
//Test bean configuration injects object attributes
UserService userService = (UserService) context.getBean("userService");
userService.add();
}
}
The results are as follows.
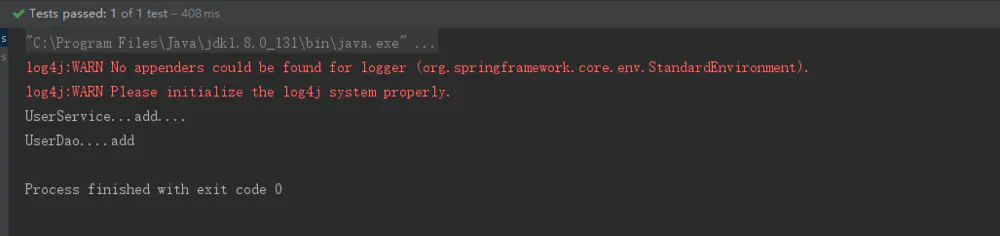
Reminder:
_The above annotation method needs to add @Component(value="userDao" on the use class) and @Autowire on the object variable to achieve.
Mode 3: Configuration files and annotations are mixed
Create the test class BookDao.java
package ioc_bean2;
public class BookDao {
public void book() {
System.out.println("BookDao....book");
}
}
Create the test class OrderDao.java
package ioc_bean2;
public class OrderDao {
public void buy() {
System.out.println("OrderDao....buy");
}
}
Create a test service BookService.java in which object properties are injected through annotations
package ioc_bean2;
import javax.annotation.Resource;
public class BookService {
//Get BookDao and OrderDao objects
@Resource(name="bookDao")
private BookDao bookDao;
@Resource(name="orderDao")
private OrderDao orderDao;
public void add() {
System.out.println("service");
bookDao.book();
orderDao.buy();
}
}
The configuration files under src are as follows
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!-- bean definitions here -->
<!--
//Open the annotation to scan the package name written by base-package. If the class is in more than one package, the way to write it is as follows
ioc_bean1,ioc_bean2,ioc_bean3...
//Or cn is used to represent all packages starting with cn, cn.ioc is used to represent all packages starting with cn.ioc.
-->
<!-- Scan the package for annotations on classes, methods, attributes -->
<context:component-scan base-package="ioc_bean2"></context:component-scan>
<!-- Configuration objects -->
<bean id="bookService" class="ioc_bean2.BookService"></bean>
<bean id="bookDao" class="ioc_bean2.BookDao"></bean>
<bean id="orderDao" class="ioc_bean2.OrderDao"></bean>
</beans>
The test code is as follows
package ioc_bean2;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import ioc1.User;
import ioc2.User2;
import ioc3.User3;
public class TestMixBean {
@Test
public void testService() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("bean2.xml");
BookService bookService = (BookService) context.getBean("bookService");
bookService.add();
}
}
The results are as follows.
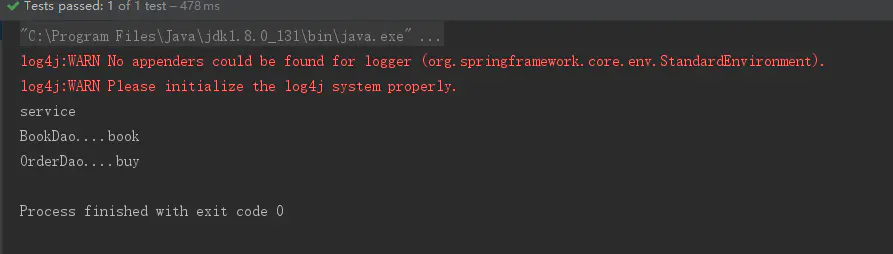
Mode 4: Attribute injection with parameters
Create arrays, collections, properties property injection test class Person.java
package property;
import java.util.List;
import java.util.Map;
import java.util.Properties;
/**
* Test array, collection, property injection
*
* @author Wu Xiao Chang
*
*/
public class Person {
private String pName;
private String[] arrs;
private List<String> list;
private Map<String, String> map;
private Properties properties;
public void setpName(String pName) {
this.pName = pName;
}
public void setArrs(String[] arrs) {
this.arrs = arrs;
}
public void setList(List<String> list) {
this.list = list;
}
public void setMap(Map<String, String> map) {
this.map = map;
}
public void setProperties(Properties properties) {
this.properties = properties;
}
public void test1() {
System.out.println("pName--"+pName);
System.out.println("arrs--"+arrs);
System.out.println("list--"+list);
System.out.println("map--"+map);
System.out.println("properties--"+properties);
}
}
Create a constructor to inject the property test class PropertyDemo1.java
package property;
/**
* Injecting attributes through constructive methods
*
* @author Wu Xiao Chang
*
*/
public class PropertyDemo1 {
private String userName;
public PropertyDemo1(String userName) {
this.userName = userName;
}
public void test1() {
System.out.println("demo1......"+userName);
}
}
Create set method to inject property test class PropertyDemo2.java
package property;
/**
* Injecting attributes through set method
*
* @author Wu Xiao Chang
*
*/
public class PropertyDemo2 {
private String bookName;
//Set method This method must conform to set/get method naming rules
public void setBookName(String bookName) {
this.bookName = bookName;
}
public void demoBookName()
{
System.out.println("book..."+bookName);
}
}
Object property injection test class UserDao.java
package property;
/**
* Object attribute injection
*
* @author Wu Xiao Chang
*
*/
public class UserDao {
public void add() {
System.out.println("UserDao.....dao");
}
}
Object property injection test class UserService.java
package property;
/**
* Object attribute injection
*
* @author Wu Xiao Chang
*
*/
public class UserService {
//Define UserDao attributes
private UserDao dao;
//Define set method
public void setDao(UserDao dao) {
this.dao = dao;
}
public void add() {
System.out.println("UserService...service");
//Using dao objects
dao.add();
}
}
Configuration file myXml.xml under src
<?xml version="1.0" encoding="UTF-8"?>
<!-- These are all ways of writing death. -->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd" >
<!--
There was another attribute in the configuration file. name,Its role and id Same, id Attribute values cannot contain special symbols (for example@#But the name attribute is OK, but the name attribute was used in earlier versions and is now replaced with id.
scope Attributes, there are the following values in total, but the main purpose is to use the first two, the latter two do not need to remember, we did not write in this configuration file. scope Property, which is used by default singleton
singleton: Default value, singleton
prototype: In many cases:
request: Create objects and place them in request Inside the domain
session: Create objects and place them in session Inside Domain
globalSession: Create objects and place them in globalSession Inside Domain
-->
<!-- Constructing injection attributes with parameters -->
<bean class="property.PropertyDemo1" id="demo">
<!-- Express to userName Attribute injection value For Xiao Wang's Value -->
<constructor-arg value="Xiao Wang" name="userName"> </constructor-arg>
</bean>
<!-- Use set Method injection attributes -->
<!--This step is equivalent to creating property.PropertyDemo2 Class objects-->
<bean class="property.PropertyDemo2" id="demo2">
<!-- Express to userName Attribute injection value For Xiao Wang's Value -->
<property value="Yi Jin Jing" name="bookName"> </property>
</bean>
<!-- Attributes of injected object type -->
<!-- To configure UserService and UserDao Object -->
<bean id="userDao" class="property.UserDao"></bean>
<bean id="userService" class="property.UserService">
<!-- injection dao Objects do not write now value Attribute, because above is the string, now is the object, using ref Attribute, dao To configure bean Label id value-->
<property name="dao" ref="userDao"></property>
</bean>
<!-- Injecting complex type attributes->Including arrays, collections, etc. -->
<bean id="person" class="property.Person">
<!-- array -->
<property name="arrs">
<list>
<value>Xiao Wang</value>
<value>pony</value>
<value>Xiao Song</value>
</list>
</property>
<!-- list aggregate -->
<property name="list">
<list>
<value>Little Austria</value>
<value>Small gold</value>
<value>Xiao Pu</value>
</list>
</property>
<!-- map aggregate -->
<property name="map">
<map>
<entry key="aa" value="Lucy"></entry>
<entry key="bb" value="Mary"></entry>
<entry key="cc" value="Tom"></entry>
</map>
</property>
<!-- properties -->
<property name="properties">
<props>
<prop key="driverclass">com.mysql.jdbc.Driver</prop>
<prop key="username">root</prop>
</props>
</property>
</bean>
</beans>
The test code is as follows
package property;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* Test attribute injection
*
* @author Wu Xiao Chang
*
*/
public class TestIoc {
@Test
public void textUser() {
//Load the spring configuration file to create objects based on content
ApplicationContext context = new ClassPathXmlApplicationContext("myXml.xml");
//Testing Method for Constructing Injection Attributes
//Get the object created by the configuration
PropertyDemo1 demo1 = (PropertyDemo1)context.getBean("demo");
demo1.test1();
//Test set method injects attribute values
PropertyDemo2 demo2 = (PropertyDemo2)context.getBean("demo2");
demo2.demoBookName();
//Testing injects object attributes using set method
UserService userService = (UserService) context.getBean("userService");
userService.add();
//Injecting complex attribute objects using set method
Person person = (Person) context.getBean("person");
person.test1();
}
}
The results are as follows.
log4j:WARN No appenders could be found for logger (org.springframework.core.env.StandardEnvironment).
log4j:WARN Please initialize the log4j system properly.
demo1......Xiao Wang
book...Yi Jin Jing
UserService...service
UserDao.....dao
pName--null
arrs--[Ljava.lang.String;@28ac3dc3
list--[Little Austria, Small gold, Xiao Pu]
map--{aa=Lucy, bb=Mary, cc=Tom}
properties--{driverclass=com.mysql.jdbc.Driver, username=root}
Process finished with exit code 0
III. Summary of Common Annotations of IoC
- Create objects (equivalent to: <bean id="" class=">)
(1)@Component
Role: Let spring manage resources. It is equivalent to configuring a bean in xml.
Attribute: value: specifies the id of the bean. If the value attribute is not specified, the id of the default bean is the class name of the current class. First letter lowercase.
(2)@Controller @Service @Repository
_Their three annotations are all for one derivative annotation, and their roles and attributes are identical.
They merely provide a clearer semantics.
@Controller: Annotations commonly used at the presentation level.
@Service: Annotations commonly used at the business level.
@Repository: Annotations commonly used for persistence layers.
Details: If there is only one attribute to assign in the commentary and the name is value, value can not be written in assignment.
- Used to inject data (equivalent to: <property name=""ref="> <property name=""value=">)
(1)@Autowired
_Function: Automatic injection according to type. When injecting attributes with annotations, set methods can be omitted. It can only inject other bean types. When multiple types are matched, the object variable name to be injected is used as the id of the bean, and the injection can also be found in the spring container. If you can't find it, make an error.
(2)@Resource
_effect: inject directly according to the id of the bean. It can only inject other bean types.
Attribute: name: specifies the id of the bean.
(3)@Value
_Function: Injecting basic data types and String data
_Attribute: value: Used to specify a value
IV. Project Source Code and Reference Download
Links: https://pan.baidu.com/s/1RDBXQcBchtA9i-bg8Z1r4Q
Extraction code: io8n