In fact, AOP_ The biggest difference between AspectJ programming and traditional AOP programming is that writing an Aspect supports multiple Advice and multiple pointcuts. And we write AOP_Aspctj does not need to inherit any interfaces, unlike traditional AOP.
- Pre notification is performed before running our proxy method.
- Advance notice has the following characteristics:
- Enhance the code before the target method is executed.
- AspectJ provides Advice without any excuses. You can write a lot of notification code to a class (aspect class)
- Pre notification definition method: no return value. You can pass in the parameter JoinPoint connection point
https://www.bilibili.com/video/BV1nz4y1d7uy
Introduction:
This set of Java video tutorials mainly explains the use and application of spring 4 in SSM framework. This set of Java video tutorials covers almost all the knowledge points that may be used in practical work. Lay a solid foundation for future study.
What is AOP?
AOP is aspect oriented programming and is based on dynamic agent. AOP is the standardization of dynamic agent, which defines the practice of dynamic agent according to steps and methods, so that developers can use dynamic agent in a unified way.
Aspect oriented programming: Aspect Oriented Programming
Aspect: aspect. The function added to the target class is aspect. The added log information and transactions are facets.
The biggest feature of the aspect is that non business methods have nothing to do with our business functions and can be used independently.
How to understand aspect oriented programming:
- It is necessary to find out the section when analyzing the project.
- Reasonably arrange the execution time of the aspect (whether it is executed before or after the target method).
- Reasonably arrange the execution position of the section (in which class and which method to add enhancements).
Terminology:
- Aspect: aspect, which means that the function to be enhanced for the business method is a pile of code, which completes a certain function. Aspect is non business function (log, transaction, statistics, parameter check, permission verification)
- JoinPoint: the connection point, where business methods and facets are connected, is the business method in a class.
- PointCut: PointCut refers to the combination of multiple join point methods. There are multiple methods, all of which need to add the function of section.
- Target object: which class do you want to add a method to
- Advice: notification, indicating the execution time of the aspect function (when the aspect is executed, before or after the method)
There are three key elements of a section:
- Function code of the section: what does the section do
- The execution position of the section is represented by pointcut
- The execution time of the aspect: before or after the target method, expressed by advice
Implementation of AOP: it is a specification and a standardization of dynamic agents.
Technical implementation framework of AOP:
1.Spring: Spring implements the AOP specification and mainly uses AOP in transaction processing. Spring's AOP implementation is rarely used in project development.
2.aspectJ: an open source framework dedicated to AOP. The aspectJ framework is integrated into the Spring framework, and the functions of the aspectJ framework can be used through Spring. The aspectJ framework implements AOP in two ways:
- Using xml configuration files
- Using annotations (we usually use annotations for AOP functions in the project). aspectJ has five annotations.
Learn how to use aspectJ framework:
1. The execution time of the aspect, that is, the advice notification. Annotations are used in the aspectJ framework.
- @Before:
- @AfterReturning
- @Around
- @AfterThrowing
- @Afte
2. Represents the position where the slice is executed, using a pointcut expression.
execution (access permission method return value method declaration (parameter) exception type)
Access permission and exception type can be omitted.
The object to be matched by the pointcut expression is the method name of the target method.
give an example:
execution(public **(..)): Specify the pointcut as any public method
execution(*set*(..)): Specify the pointcut as any method that starts with set
execution(*com.xyz.service.*.*(..)): Specify the pointcut as com xyz. Any method in any class in the service package
Using aspectJ framework to implement aop
The purpose of using aop is to add additional functions to existing classes and methods without changing the original code.
The basic steps to implement AOP using aspectJ:
- New maven project
- Add dependency: (1) Spring dependency (2) aspectJ dependency (3)Junit unit test
- Create target class: this class has interface and implementation class of interface. We want to add functionality to the methods in this class.
- Create a facet class (the code for adding functions is written in the facet class): ordinary class
- Add the @ Aspect annotation on the class
- Define facet methods in classes. Methods are the functional code to be executed. Add the notification annotation in aspectJ above the method, such as @ Before, and specify the pointcut expression.
5. To give objects to the container to create, Spring container manages these objects.
Create the Spring configuration file, declare the object in the configuration file, and hand over the object to the container for unified management. Declared objects can use annotations or < bean > tags of xml.
- Declare target object
- Declare facet class objects
- Declare the automatic proxy generator tag in the aspectJ framework.
Automatic proxy generator: used to complete the automatic creation of proxy objects.
6. Create a test class and get the target object from the Spring container (this object is actually a proxy object). Through the agent execution method, the function of AOP is enhanced.
Full code:
Idea Maven - select QuickStart POM for the template Add related dependencies to XML: (1) Spring dependency (2) aspectJ dependency (3)Junit unit test
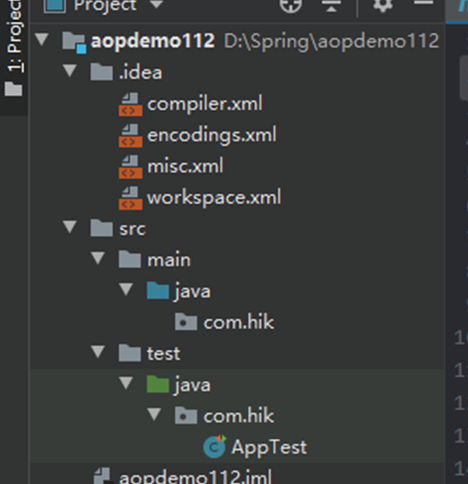
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <!--unit testing --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> <!--Spring rely on--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.2.5.RELEASE</version> </dependency> <!--aspectJ rely on--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>5.2.5.RELEASE</version> </dependency> </dependencies>
Maven view added dependencies:
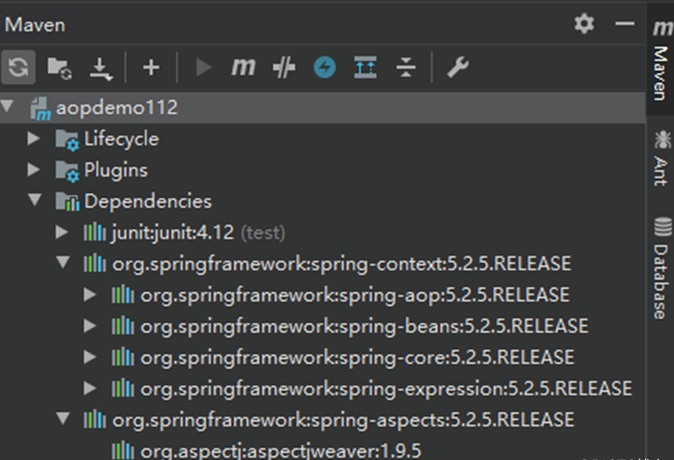
Com. The following classes and interfaces of hik package:
SomeService interface
package com.hik; public interface SomeSeivece { void doSome(String name, Integer age); }
Implementation class of SomeService interface SomeServiceImp
package com.hik; public class SomeSeiveceImpl implements SomeSeivece { @Override public void doSome(String name, Integer age) { //Add a function to the doSome() method to output the execution time of the method before the execution of doSome System.out.println("Target method doSome()implement"); } }
MyAspect class
package com.hik; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import java.util.Date; /* * @Aspect: Is an annotation in the framework of aspectJ. * Function: to indicate that the current class is a faceted class. A faceted class is a class that adds functions to business methods. In this class, there are faceted function codes * Where annotations are used: above the definition of the section class * */ @Aspect public class MyAspect { /* * Definition method: the method is to realize the section function. * Method definition requirements: * 1.Public method public * 2.Method has no return value * 3.The method name is custom * 4.Methods can have parameters or no parameters. If there are parameters, and the parameters are not user-defined, there are several parameter types available * */ /* * @Before: Pre notification annotation * This annotation has an attribute value. The attribute value is value: it is the pointcut expression execution (), indicating the execution position of the function in the aspect *@Befor Annotation location: add annotation on the method * characteristic: * 1.Execute before target method * 2.The execution result of the target method will not be changed * 3.Does not affect the operation of the target method * */ /*execution(Access modifier package name Class name Method name (parameter type 1, parameter type 2...)*/ @Before(value = "execution(public void com.hik.SomeSeiveceImpl.doSome(String,Integer))") public void myBefore(){ //Is the function code to be executed System.out.println("Pre notification, aspect function: output the execution time before the target method:" + new Date()); } }
Set the configuration file in the resource under the main directory: ApplicationContext xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd"> <!--Give the object to Spring Container, by Spring Containers create and manage objects uniformly--> <!--Declare target object--> <bean id="someService" class="com.hik.SomeSeiveceImpl"></bean> <!--Declare slice object--> <bean id="myAspect" class="com.hik.MyAspect"></bean> <!--Declaring automatic proxy generators: Using aspectJ Functions within the framework to create proxy objects for target objects. Creating a proxy object is implemented in memory and modifying the in memory structure of the target object. As a proxy object, the target object is the modified proxy object--> <!--This tag will find all the objects in the container, and then find the target and generate the proxy of the target according to the declaration information of the pointcut expression, Will put Spring The target object in generates a proxy object at once--> <aop:aspectj-autoproxy> </aop:aspectj-autoproxy>
Test class
package com.hik; import org.junit.Test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MyTest { @Test public void Test01(){ String config = "applicationcontext.xml"; ApplicationContext applicationContext = new ClassPathXmlApplicationContext(config); //Get target object from container SomeSeivece proxy = (SomeSeivece) applicationContext.getBean("someService"); //Through the proxy object execution method, the function is enhanced when the target method is executed proxy.doSome("Rita", 18); } }
Execution results:
Pre notification, aspect function: output execution time before the target method: Thu Jan 13 16:30:16 CST 2022
The target method doSome() executes
Process finished with exit code 0