SpringBoot integrates Mybatis(CRUD implementation)
Preparatory tool: IDEA jdk1.8 Navicat for MySQL Postman
New Project
Selection dependency: mybatis Web Mysql JDBC
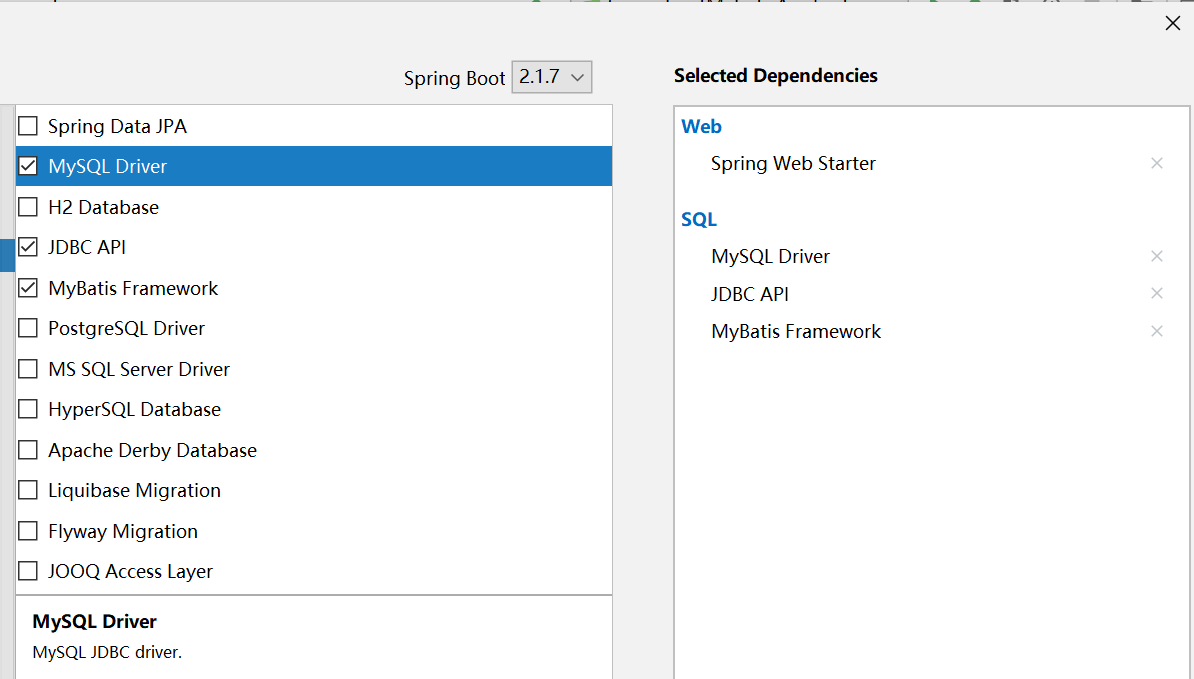
Project structure
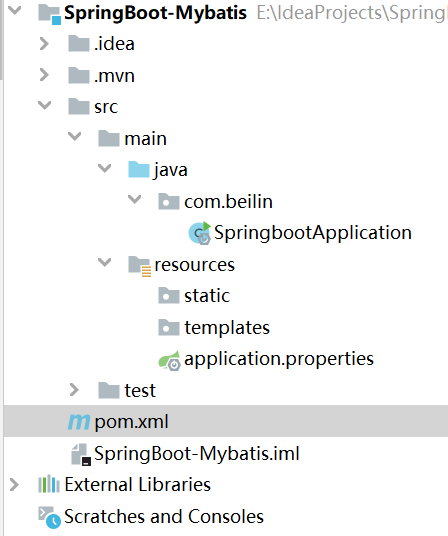
pom dependency:

1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
3 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
4 <modelVersion>4.0.0</modelVersion>
5 <parent>
6 <groupId>org.springframework.boot</groupId>
7 <artifactId>spring-boot-starter-parent</artifactId>
8 <version>2.1.7.RELEASE</version>
9 <relativePath/> <!-- lookup parent from repository -->
10 </parent>
11 <groupId>com.beilin</groupId>
12 <artifactId>SpringBoot-Mybatis</artifactId>
13 <version>0.0.1-SNAPSHOT</version>
14 <name>SpringBoot-Mybatis</name>
15 <description>Demo project for Spring Boot</description>
16
17 <properties>
18 <java.version>1.8</java.version>
19 </properties>
20 <dependencies>
21 <dependency>
22 <groupId>org.springframework.boot</groupId>
23 <artifactId>spring-boot-starter-jdbc</artifactId>
24 </dependency>
25 <dependency>
26 <groupId>org.springframework.boot</groupId>
27 <artifactId>spring-boot-starter-web</artifactId>
28 </dependency>
29
30 <!--Import mybatis rely on-->
31 <dependency>
32 <groupId>org.mybatis.spring.boot</groupId>
33 <artifactId>mybatis-spring-boot-starter</artifactId>
34 <version>2.1.0</version>
35 </dependency>
36
37 <!--Import mysql rely on-->
38 <dependency>
39 <groupId>mysql</groupId>
40 <artifactId>mysql-connector-java</artifactId>
41 <version>5.1.47</version>
42 </dependency>
43
44 <dependency>
45 <groupId>org.springframework.boot</groupId>
46 <artifactId>spring-boot-starter-test</artifactId>
47 <scope>test</scope>
48 </dependency>
49 </dependencies>
50
51 <build>
52 <plugins>
53 <plugin>
54 <groupId>org.springframework.boot</groupId>
55 <artifactId>spring-boot-maven-plugin</artifactId>
56 </plugin>
57 </plugins>
58 </build>
59
60 </project>
pom.xml
2. Creating user tables
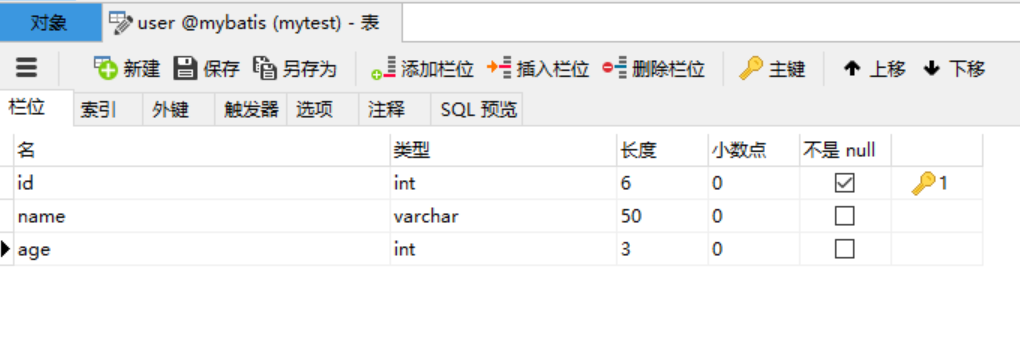
III. Project allocation
1. Create control package, mapper package and entity package under com.beilin; create mapping folder under resources folder (xml file used to store mapper mapping)
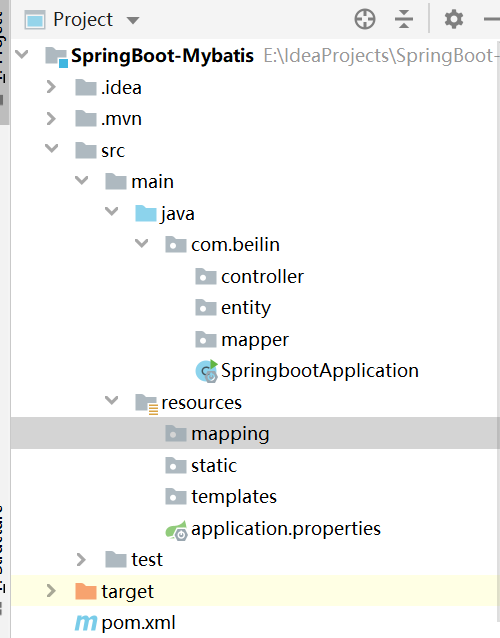
2. Add properties configuration file: application.properties

1 #Configure mybatis
2
3 #Configure the xml Mapping path
4 mybatis.mapper-locations=classpath:mapping/*.xml
5 #Configure entity category names
6 mybatis.type-aliases-package=com.beilin.entity
7 #Naming method of open hump
8 mybatis.configuration.map-underscore-to-camel-case=true
9
10 #Configure Mysql connection
11 spring.datasource.url=jdbc:mysql://localhost:3306/mybatis?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC
12 spring.datasource.username=root
13 spring.datasource.password=123456
14 spring.datasource.driver-class-name=com.mysql.jdbc.Driver
application.properties
3. Add @MapperScan annotation to Springboot startup class

1 package com.beilin;
2
3 import org.mybatis.spring.annotation.MapperScan;
4 import org.springframework.boot.SpringApplication;
5 import org.springframework.boot.autoconfigure.SpringBootApplication;
6
7 @MapperScan(value = "com.beilin.mapper")
8 @SpringBootApplication
9 public class SpringbootApplication {
10
11 public static void main(String[] args) {
12 SpringApplication.run(SpringbootApplication.class, args);
13 }
14
15 }
SpringbootApplication
4. Code Implementation
1. Entity class User

1 package com.beilin.entity;
2
3 public class User {
4 /**
5 * name:Student entity
6 */
7
8 //Primary key id
9 private int id;
10 //Full name
11 private String name;
12 //Age
13 private int age;
14
15 // Get and Set Method
16 public int getId() {
17 return id;
18 }
19
20 public void setId(int id) {
21 this.id = id;
22 }
23
24 public String getName() {
25 return name;
26 }
27
28 public void setName(String name) {
29 this.name = name;
30 }
31
32 public int getAge() {
33 return age;
34 }
35
36 public void setAge(int age) {
37 this.age = age;
38 }
39 }
User.java
2. Data manipulation layer UserMapper

1 package com.beilin.mapper;
2
3 import com.beilin.entity.User;
4
5 import java.util.List;
6
7 public interface UserMapper {
8
9 //insert
10 public void insert(User user);
11
12 //according to id delete
13 public void delete(Integer id);
14
15 //according to user Of id modify
16 public void update(User user);
17
18 //according to id query
19 public User getById(Integer id);
20
21 //All queries
22 public List<User> list();
23
24 }
UserMapper.java
3.UserMapper mapping file

1 <?xml version="1.0" encoding="UTF-8"?>
2 <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
3 <mapper namespace="com.beilin.mapper.UserMapper">
4
5 <!-- Insert a user -->
6 <insert id="insert" parameterType="user">
7 insert into user(name,age) values(#{name},#{age})
8 </insert>
9
10 <!-- according to id Delete students -->
11 <delete id="delete" parameterType="int">
12 delete from user where id=#{id}
13 </delete>
14
15 <!-- according to id Modifying Student Information -->
16 <update id="update" parameterType="user">
17 update user set name=#{name},age=#{age} where id=#{id}
18 </update>
19
20 <!-- according to id query -->
21 <select id="getById" parameterType="int" resultType="user">
22 select * from user where id=#{id}
23 </select>
24
25 <!-- Query all -->
26 <select id="list" parameterType="int" resultType="user">
27 select * from user
28 </select>
29
30 </mapper>
UserMapper.xml
4. Control layer User Controller

1 package com.beilin.controller;
2
3 import com.beilin.entity.User;
4 import com.beilin.mapper.UserMapper;
5 import org.springframework.beans.factory.annotation.Autowired;
6 import org.springframework.web.bind.annotation.*;
7
8 import java.util.List;
9
10 @RestController
11 public class UserController {
12
13 @Autowired
14 private UserMapper userMapper;
15
16 //insert user
17 @RequestMapping("/user")
18 public void insert( User user) {
19 userMapper.insert(user);
20 }
21
22 //according to id delete
23 @RequestMapping("/user1/{id}")
24 public void delete(@PathVariable("id") Integer id) {
25 userMapper.delete(id);
26 }
27 //modify
28 @RequestMapping("/user2/{id}")
29 public void update(User user,@PathVariable("id") Integer id) {
30 userMapper.update(user);
31 }
32
33 //according to id Query students
34 @RequestMapping("/user3/{id}")
35 public User getById(@PathVariable("id") Integer id) {
36 User user = userMapper.getById(id);
37 return user;
38 }
39
40 //All queries
41 @RequestMapping("/users")
42 public List<User> list(){
43 List<User> users = userMapper.list();
44 return users;
45 }
46
47 }
UserController.java
Testing using PostMan or directly in the browser
Problems encountered in the process:
Error reporting: org.apache.ibatis.binding.BindingException: Invalid bound statement (not found)
Interpretation: That is to say, your Mapper interface, injected by SpringBoot, can not normally use mapper.xml sql;
First, check whether your code is wrong, sql statement is correct, and on this basis, compare the following solutions.
There are several possible scenarios here:
- The interface has been scanned, but the proxy object has not been found. Even if you try to inject, you inject an incorrect object (probably null).
- The interface has been scanned, the proxy object has been found and injected into the interface, but when a specific method is called, it cannot be used (other methods may be normal).
The results of the two kinds of errors are the same. Here are several methods of checking:
1. Is the mapper interface and mapper.xml in the same package? Is the name the same (only with different suffixes)?
For example, the interface name is UserMapper.java; the corresponding XML should be UserMapper.xml.
2. Does the namespace of mapper. XML match the package name of the mapper interface?
For example, if the package name of your interface is com.beilin.mapper and the interface name is UserMapper.java, then the namespace of your mapper.xml should be com.beilin.mapper.UserMapper.
3. The method name of the interface is the same as the id of an sql tag in xml.
For example, the interface method List < User > GetById (); then, there must be one entry in the corresponding xml that is < select id="GetById" parameterType = "int" resultType = "user"> ** </select>.
4. If the return value List set in the interface is not known to be the same as other sets, then the configuration in xml should use resultMap as far as possible (to ensure the correct configuration of resultMap) and not use resultType.
5. Finally, after compiling, look at the directory where the interface is located. It is likely that no corresponding XML file is produced, because maven is not compiled by default, so you need to add a paragraph in your pom.xml <build></build>.

1 <resources>
2 <resource>
3 <directory>src/main/java</directory>
4 <includes>
5 <include>**/*.xml</include>
6 </includes>
7 <filtering>true</filtering>
8 </resource>
9 </resources>
resources
Keywords:
Windows
xml
Mybatis
Spring
Java
Added by vweston on Thu, 05 Sep 2019 08:14:06 +0300