In the past, there were many configuration files in the traditional ssm framework. After reading the documents for several days, I made a mess of the logical relationship of xml. At that time, I completely followed the version requirements of the demo on the Internet (jdk and tomcat), so in the end, I failed to run with various problems.
Today, I tried to integrate with the springboot framework. I can't believe I succeeded after several failures!!
Now let's record the process:
Add POM dependency:
The dao layer depends on the following two mysql connector s and the starter of mybatis (this is a good thing)
Start writing code:
Here we have a simple data table:
Our purpose is to send a request with id value with get. The server returns all the information of the librarian according to the id value and displays it directly on the page in json,
Controller: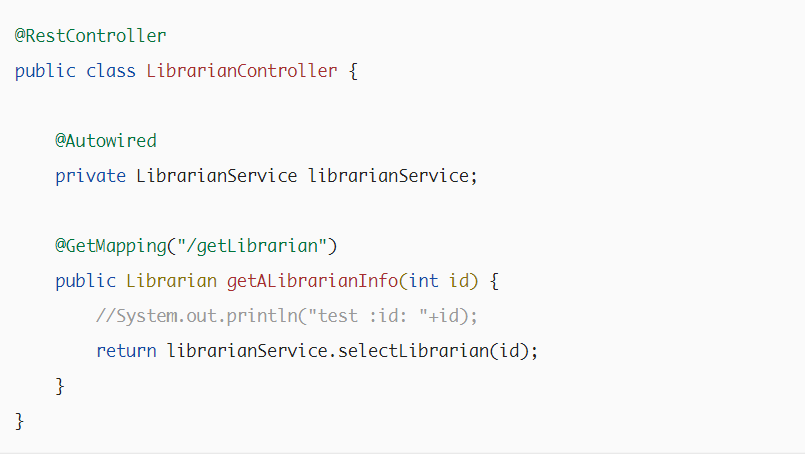
RestController is a combination of two annotations responsebody+Controller. It is generally used to directly transfer json data. Why can you send an object directly? This is because the jackson module is built into the springboot, and you can see the jar package in this regard with maven's dependence (I wrote it in accordance with the online tutorial and used gson to handle it, which is invincible compared to this)
Then we see the injected sevice, and the following is the service
Service: 
It's an interface
ServiceImpl: 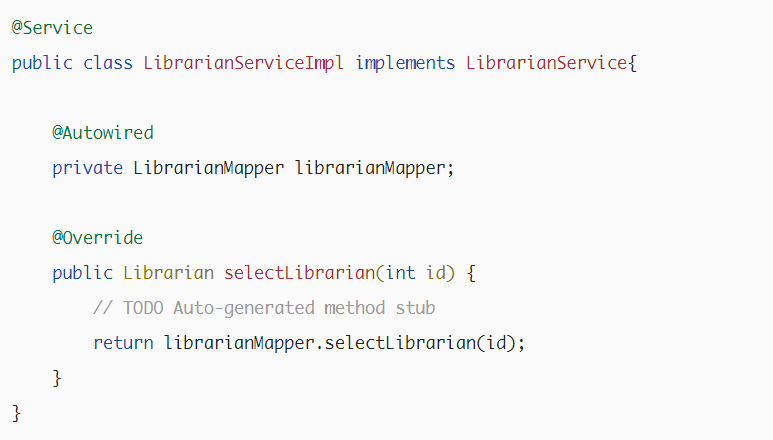
Remember to add Service comments here before spring generates bean s and injects them into the controller.
Then I see a mapper injected here
Dao: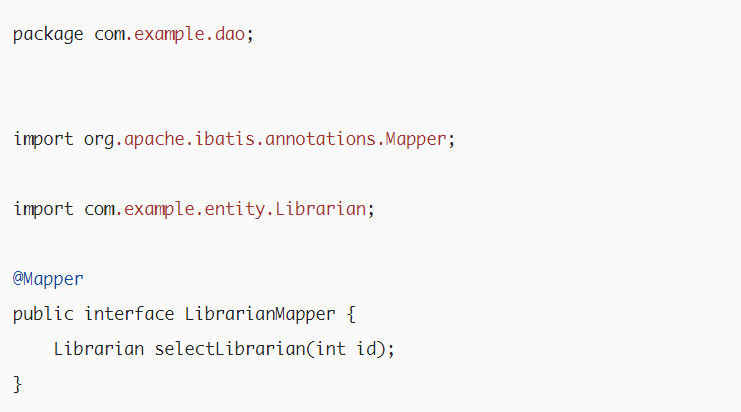
Mapper added here is the comment of MyBatis. The purpose is to enable spring to dynamically generate the implementation of this interface according to xml and this interface. If you add a Repository, spring generates a bean and automatically injects it into the relevant references of the service.
Then there is Mapper's xml file (my MyBatis related sql uses xml)
mapper.xml:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.example.dao.LibrarianMapper"> <!-- Can you use it according to your own needs --> <resultMap type="Librarian" id="LibrarianMap"> <id column="id" property="id" jdbcType="INTEGER" /> <result column="userName" property="useName" jdbcType="VARCHAR" /> <result column="password" property="password" jdbcType="VARCHAR" /> <result column="age" property="age" jdbcType="INTEGER" /> <result column="position" property="position" jdbcType="VARCHAR" /> </resultMap> <select id="selectLibrarian" parameterType="INTEGER" resultMap="LibrarianMap"> select * from t_librarian where 1=1 and id = #{id,jdbcType=INTEGER} </select> </mapper>
The namespace in the third line is very important. It specifies which dao (mapper) interface this xml corresponds to
resultMap is the correspondence between a pojo and a database table (only a libraian is written here, and the full path is not written because it is set, which will be described below)
The id of the select tag corresponds to the method name in the mapper interface, and the parameterType is the parameter type passed in
Simple configuration and setup:
OK, now let's talk about the settings. How do you know where the spring of Controller, Service and MyBatis annotation Mapper is? Don't you have to set up a scan like mvc?
Yes, we have made simple settings in the startup class:
package com.example.main; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; /** * Specify the package to be scanned, and all classes marked @ Component under the specified package will be automatically scanned and registered as bean s, * Of course, it includes the sub annotations @ Service,@Repository,@Controller under @ Component. * @author 85060 * */ @SpringBootApplication(scanBasePackages={"com.example.*"}) @MapperScan("com.example.dao") public class SpringBootDemoApplication { public static void main(String[] args) { SpringApplication.run(SpringBootDemoApplication.class, args); } }
The SpringBootApplication is actually a combination of several annotations, so you can directly write the settings of the scanning package here, and then the MapperScan is the setting annotation provided by MyBatis.
Then there are some configuration files:
spring.thymeleaf.cache=false spring.devtools.restart.enabled=true spring.devtools.restart.additional-paths=src/main/java spring.datasource.driver-class-name=com.mysql.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/db_library spring.datasource.username=root spring.datasource.password=4008 mybatis.type-aliases-package=com.example.entity mybatis.mapperLocations=classpath:mappers/*.xml
It is mainly related to the beginning of the fourth line. The last two lines are to set the basic package (package alias), which is why only one libraian can be written in mapper.xml.
The last important line is to tell the system where to find the mapper.xml file.
Last Rendering: