Recently I learned the integrated development of SSM framework. Now I try to develop an employee management system by myself. I hope I can skillfully use SSM framework in the process of writing BUG.
The development of B/S end is adopted in this system, and the tools used are ideas, 019, navicat and mysql.
The framework used includes Spring, Spring MVC and mybaits.
Now let's start the first day of development.
First open IDEA - File - NEW - PROJECT - and select Maven
Then select webapp as the skeleton to create the project, and then define the project name yourself
Enter and wait for Maven to finish creating. The next step is to import the dependency of POM. My POM has come from the blog of teacher Qin Jiang. You can go to see his SSM integration, which is very helpful for novices.
<!--rely on junit Database driven connection pool servlet jsp mybaits mybaits-spring spring--> <dependencies> <!--Junit--> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <!--Database driven--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.47</version> </dependency> <!-- Database connection pool --> <dependency> <groupId>com.mchange</groupId> <artifactId>c3p0</artifactId> <version>0.9.5.2</version> </dependency> <!--Servlet - JSP --> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <!--Mybatis--> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.2</version> </dependency> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>2.0.2</version> </dependency> <!--Spring--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.1.9.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>5.1.9.RELEASE</version> </dependency> <!--Plug-in unit--> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.4</version> </dependency> </dependencies> <!--Static resource export problem--> <build> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>false</filtering> </resource> <resource> <directory>src/main/resources</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>false</filtering> </resource> </resources> </build> As long as these dependencies are imported, our basic functions, addition, deletion, modification and query can be completed.
Next we need to add WEB support
Right click your project and select how to Add Frameworks Support, and select WEB Application
And then you'll find that your directory is like thisThese preparations are ready to start preparing the database table. Here I create a users table field with Naticat as follows
This is the first table that I need to prepare for the function login function. Then we can start our first development work. Create several necessary packages first
pojo means bean, but also entity. They are the same (my understanding at this stage). The classes stored in pojo are entity classes corresponding to the database one by one
Then we write this entity class, which is generally the case
Note that I use a plug-in called lombok, which can help you implement getter and setter methods through annotations. If it is successful, just check the Structure in the lower right corner.
Then we start to connect to the DATABASE, and there's a DATABASE point card on the right
Here, I choose mysql8 as the database I use, and I need to add the region on the url to select 8
Then we configure the data source of mybaits. The file name is database.properties, which is used to configure four necessary JDBC items
Then create a new file called spring-dao.xml. The code is as follows
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"> <!-- Configuration integration mybatis --> <!-- 1.Associated database file --> <context:property-placeholder location="classpath:database.properties"/> <!-- 2.Database connection pool --> <!--Database connection pool dbcp Semi automatic operation cannot be connected automatically c3p0 Automatic operation (automatically load the configuration file and set it in the object) --> <bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"> <!-- Configure connection pool properties --> <property name="driverClass" value="${jdbc.driver}"/> <property name="jdbcUrl" value="${jdbc.url}"/> <property name="user" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> <!-- c3p0 Private properties of connection pool --> <property name="maxPoolSize" value="30"/> <property name="minPoolSize" value="10"/> <!-- Do not automatically close the connection commit --> <property name="autoCommitOnClose" value="false"/> <!-- Get connection timeout --> <property name="checkoutTimeout" value="10000"/> <!-- Number of retries when get connection failed --> <property name="acquireRetryAttempts" value="2"/> </bean> <!-- 3.To configure SqlSessionFactory object --> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!-- Inject database connection pool --> <property name="dataSource" ref="dataSource"/> <!-- To configure MyBaties Global profile:mybatis-config.xml --> <property name="configLocation" value="classpath:mybatis-config.xml"/> </bean> <!-- 4.Configuration scan Dao Interface package, dynamic implementation Dao Interface injection to spring Container --> <!--Interpretation: https://www.cnblogs.com/jpfss/p/7799806.html--> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <!-- injection sqlSessionFactory --> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/> <!-- Give the need to scan Dao Interface package --> <property name="basePackage" value="com.mo.dao"/> </bean> </beans>
Then create an interface and an xml in dao
UserMapper
package com.mo.dao; import com.mo.pojo.Users; public interface UserMapper { Users queryUsers(String username, String password); } UserMapper.xml ```java <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.mo.dao.UserMapper"> <select id="queryUsers" resultType="Users"> select * from users where username=#{username} and password = #{password} </select> </mapper> //Then create an interface and an implementation class in the service layer 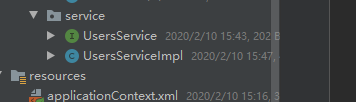 UsersService ```java package com.mo.service; import com.mo.pojo.Users; public interface UsersService { //Query whether this user exists Users queryUsers(String username,String passowrd); }
UsersServiceImpl
package com.mo.service; import com.mo.dao.UserMapper; import com.mo.pojo.Users; import org.springframework.beans.factory.annotation.Autowired; public class UsersServiceImpl implements UsersService { @Autowired private UserMapper userMapper; public Users queryUsers(String username, String passowrd) { Users users = userMapper.queryUsers(username, passowrd); System.out.println(users); return users; } }
Creating a new spring-service.xml
spring-service.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!-- scanning service Dependent bean --> <context:component-scan base-package="com.mo.service" /> <!--UsersServiceImpl Injection into IOC Container--> <bean id="UsersServiceImpl" class="com.mo.service.UsersServiceImpl"> <property name="userMapper" ref="userMapper"/> </bean> <!-- Configure transaction manager --> <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <!-- Inject database connection pool --> <property name="dataSource" ref="dataSource" /> </bean> </beans>
An error will be reported when filling in the property. As long as ALT + enter selects SET, it will automatically jump to the UsersService to SET a method.
package com.mo.service; import com.mo.dao.UserMapper; import com.mo.pojo.Users; import org.springframework.beans.factory.annotation.Autowired; public class UsersServiceImpl implements UsersService { @Autowired private UserMapper userMapper; public Users queryUsers(String username, String passowrd) { Users users = userMapper.queryUsers(username, passowrd); System.out.println(users); return users; } public void setUserMapper(UserMapper userMapper) { this.userMapper= userMapper; } }
After that, no error will be reported, and then spring service will be sent. xml in spring
Then write a spring MVC. xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd"> <!-- To configure SpringMVC --> <!-- 1.open SpringMVC Annotation driven --> <mvc:annotation-driven/> <!-- 2.Static resource default servlet To configure--> <mvc:default-servlet-handler/> <!-- 3.To configure jsp display ViewResolver view resolver --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="viewClass" value="org.springframework.web.servlet.view.JstlView"/> <property name="prefix" value="/WEB-INF/jsp/"/> <property name="suffix" value=".jsp"/> </bean> <!-- 4.scanning web Dependent bean --> <context:component-scan base-package="com.mo.controller"/> </beans>
Create a class in the Controller
package com.mo.controller; import com.mo.pojo.Users; import com.mo.service.UsersService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller @RequestMapping("/User") public class UserController { @Autowired @Qualifier("UsersServiceImpl") private UsersService usersService; public String queryUsers(String username,String password){ Users users = usersService.queryUsers(username, password); System.out.println(users); return "UserInformation"; } }
Then create spring MVC. xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd"> <!-- To configure SpringMVC --> <!-- 1.open SpringMVC Annotation driven --> <mvc:annotation-driven/> <!-- 2.Static resource default servlet To configure--> <mvc:default-servlet-handler/> <!-- 3.To configure jsp display ViewResolver view resolver --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="viewClass" value="org.springframework.web.servlet.view.JstlView"/> <property name="prefix" value="/WEB-INF/jsp/"/> <property name="suffix" value=".jsp"/> </bean> <!-- 4.scanning web Dependent bean --> <context:component-scan base-package="com.mo.controller"/> </beans>
Finally deploy your project in tomcat
During this period, I only had one problem: there was an error when I started TONcat
java.lang.ClassNotFoundException: org.springframework.web.filter.CharacterEncodingFilter later found that there was a lack of lib, that is, our package was not imported, so we had to do this
In IDEA, click File > project structure > artifacts > right-click the project name in the Output Layout on the right, and select Put into Output Root.
After execution, add the lib directory in WEB-INF, which is the jar package referenced by the project. Click OK.
After that, the project can be started normally
Then index. jsp content writing
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<label >Account number:</label> <input type="text" class="form-control" name="username" required /> <label >Password:</label> <input type="password" class="form-control" name="password" required /> <input type="submit" class="form-control" value="Sign in">
The link inside is the connection of bootstrap, so we can use some of its styles
Start tomcat
After that, we input the account password. One is what you have in your database and the other is what you don't have
I input a wrong account password first, and we can still enter the display interface
But the day after tomorrow, we show that the account password is null
Prove that there is no such data in our database. When we input a correct account code, we can still enter it
But what our backstage shows is
It is proved here that our database has this information, so we can make some decisions on this null
package com.mo.controller; import com.mo.pojo.Users; import com.mo.service.UsersService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller @RequestMapping("/User") public class UserController { @Autowired @Qualifier("UsersServiceImpl") private UsersService usersService; //Login function @RequestMapping("/Login") public String queryUsers(String username,String password){ Users users = usersService.queryUsers(username, password); System.out.println(users); if(users == null){ System.out.println("Account password error"); return "Login"; } return "UserInformation"; } }
I add null judgment to the login function. At this time, we are going to enter the wrong account password again
The system prompts that the account password is wrong, and the page returns to the login page
And when we output the correct account password
We have successfully entered the display page
This is a simple login function made by myself. There are many defects. I hope you can give me more advice