SSM
Three components of Java Web: Servlet, Filter and Listener;
Review Java Web
filter process
Listener
Eight: ServletRequest (2), HttpSession (2), ServletContext (2)
2: Lifecycle listener, attribute change listener
4 (HttpSession): in addition to the above two, there are two additional ones (activate passivation listener and bind unbind listener)
Master listener:
Servertcontextlistener (life cycle): listen to the creation and destruction of ServletContext (listen to the start and stop of the server); The server starts to create a ServletContext object, the server stops and destroys the created ServletContext object;
ServletContext:
1. A web project corresponds to a ServletContext, which represents the information of the web project
2. It can also be used as the largest domain object to share data during the operation of the whole project
How to use the listener:
1. First implement the corresponding listener interface
2. Go to the web XML for configuration; Note: two listeners are interfaces that JavaBean s need to implement (HttpSessionActivationListener, HttpSessionBindingListener)
AJAX and JSON
JSON:(js object table method) is a lightweight data exchange format (compared with xml)
A complex js object
var student = { lastName: "Zhang Shan", age: 18, car: {pp: "baoma", price: "15555555555"}, infos: [{bookName: "Journey to the West", price: 19}, 18, true] };
If the server returns JS objects to the browser, it is convenient for the browser to use js parsing;
JSON
//json requires the same as js objects, except that the key must be a string //js objects can be declared in double quotes or not var student2 = { "lastName": "Zhang Shan", "age": 18 }; //JSON (built-in object of js) this is provided by js to convert js objects into JSON (which should be the string representation of js objects) strings var strjson= JSON.stringify(student2); alert(typeof strjson); alert(strjson);
Two important API s:
1. Convert js object to JSON
JSON.stringify(js object)
2. Convert JSON object to js' object
JSON.parse(JSON object);
AJAX
AJAX: synchronous Javascript And XML
AJAX: is a non refresh page and server interaction technology. (you can get the data of the server without refreshing the page)
Original interaction:
1. Send request
2. The server receives the request and calls the servlet corresponding to the queue for processing; Response information will be generated after servlet processing;
3. The browser receives the data responded by the server, empties the previous page and displays the new data; (the effect is page refresh)
Current interaction: (XmlHttpRequest object)
1. The XmlHttpRequest object helps us send the request
2, after receiving the request, the server calls the corresponding servlet to process. Response information will be generated after servlet processing;
3. The XmlHttpRequest object receives data (the browser cannot feel this data; the xml object receives this data)
Package for converting Object objects in fJava into Json objects
Code demonstration:
Map<String, Object> map = new HashMap<>(); map.put("lastName","zs"); map.put("age",18); //1. Convert to json Gson gson = new Gson(); String str = gson.toJson(map); response.getWriter().write(str);
Introduced dependencies: (just download from maven warehouse)
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.6</version> </dependency>
Changed our traditional cross reflection light hi
1. Send a request;
2. When the server receives a request, it often carries data to the page to process the request. request.setAtteribute(“map”,map); Forward to page
3. The browser receives the page data and uses el expression to obtain the data in the page;
It causes the whole page to be refreshed, resulting in a great burden on the server;
Only let the server return part of the data we need; Do not return to the entire page; xhr replaces the browser to receive the response and send the request; Use dom to add and delete to change the effect of the page;
Asynchronous non refresh page technology
Asynchronous: does not block the browser
Synchronization: it will block the browser; Because you need to wait until the server processes a complete request and completes the response before you can do anything else
What is ajax:
ajax is that the xhr object sends a request to the server, receives the response data, and changes the page effect by adding, deleting and modifying dom
The usage of get request and post request in Ajax is the same, except that one sends a get request and the other sends a post request. The difference is that the data is encapsulated in the request when the get request is made? Later, the post request encapsulates the data in the request body
Data encapsulated by post request
get request encapsulates data
IOC and DI
IOC: (Inversion) Of Control;
Control: resource acquisition method;
Active: (you can create any resources you want)
BookServlet{ BookService bs= new BookService(); AirPlane ap=new AirPlane(); }
Passive: the acquisition of resources is not created by ourselves, but handed over to a container to create and set;
BookService{ BookService bs; public void test01(){ bs.checkout(); } }
Container: manage all components (functional classes)
Suppose that BookServlet is managed by container and BookService is also managed by container; The container can automatically detect which components (classes) need to use another write component (class); the container helps us create the BookService object and assign the BookService object to the past;
Container: the active new resource becomes the passive receiving resource·
(container) matchmaking agency: active acquisition becomes passive reception;
DI (Dependency Injection);
The container can know which component (class) needs another class (component) when it runs; The container injects the prepared BookService object in the container (assigning value to the attribute by reflection) into BookService in the form of reflection
HelloWorld; (register the objects in the container in various ways (similar to the registered members of the wedding Office)), which used to be their own new objects. Now all objects are created by the container; Register components in container
Process to be prepared in the future;
HelloWorld
1. Guide Package (4 jar packages: Spring context, spring core, spring beans, spring expression)
<!-- https://mvnrepository.com/artifact/org.springframework/spring-context --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.3.9</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>5.3.9</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-beans --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>5.3.8</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.logging.log4j/log4j-core --> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-core</artifactId> <version>2.14.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-expression --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-expression</artifactId> <version>5.3.9</version> </dependency>
2. Write configuration (ioc.xml)
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- Register one person Object, Spring Automatically creates a Person object--> <!-- One bean Tag can register a component (object, class) id: The unique identifier of this object class:The full class name of the registered component to write --> <bean id="person" class="com.pzx.spring.bean.Person"> <!-- property The tag assigns values to the properties of the object name Is used to specify the attribute name, value Is used to specify attribute values --> <property name="lastName" value="Zhang San"></property> <property name="age" value="18"></property> <property name="email" value="zs@guigu.com"></property> <property name="gender" value="male"></property> </bean> </beans>
3. Test (the current configuration file of ClassPathXmlApplicationContext is under ClassPath)
@Test public void test(){ //ApplicationContext: represents the ioc container //The xml configuration file of the current application is in the internal path //The current configuration file of ClassPathXmlApplicationContext is under ClassPath //Get the ioc container object according to the Spring configuration file ApplicationContext ioc = new ClassPathXmlApplicationContext("ioc.xml"); Person person = ioc.getBean("person", Person.class); System.out.println(person); } //Result Person{lastName = 'Zhang San', age=18, gender = 'male', email='zs@guigu.com '}
Little summary of HelloWorld
There are several small details: (objects are created through reflection)
1. ApplicationContext (interface of IOC container)
2. Register a component for the container; We also get the object of this component by id from the container?
- The creation of the component is done by the container for us
*When was the Person object created?
*The objects in the container are created after the container is created;
3. The same component is a single instance in the ioc container, and the container is created after startup
4. If there is no such component in the container, getting the component will report an exception
* org.springframework.beans.factory.NoSuchBeanDefinitionException: No bean named 'person3' available
5. When the ioc container creates this component object, (property) will use the setter method to assign values to the properties of the JavaBean
6. What determines the property name of a JavaBean? getter/setter method, the property name is to remove set, and the following string is lowercase
* Be careful not to do it casually getter/setter,be-all getter/setter Are generated automatically
Principle of generic dependency injection
Convert an array to a collection
Arrays.asList(Array name)
AOP
How to define a class in Spring as an aspect class
Define a class in Spring as an aspect class
Add @ Aspect and @ Component annotations to the class
The function of @ Aspect is to declare this class as an Aspect class
The @ Component annotation is used to indicate that this class is injected into the IOC container
Spring AOP five notification notes
After adding these annotations to the following methods, you have to add execution() to the annotation
-
@Before: run the pre notification before the target method executes
-
@After: run post notification after target method execution is completed
-
@After returning: after the target method returns normally, a notification may be added to this annotation
@AfterReturning(value = "hahaMyPoint()" ,returning="result") @AfterReturning This annotation may also be added returning The value of is used to indicate the returned execution result
-
@After throwing: performs exception notification after the target method throws an exception
@AfterThrowing(value = "hahaMyPoint()",throwing="exception") This annotation may also need to be added throwing,Used to represent the returned exception information
Code demonstration
/** * Detail 4: we can get the detailed information of the target method when notifying the method to run; * 1)You only need to write one parameter on the parameter list of the notification method: * JoinPoint joinPoint:Encapsulates the details of the target method * * 2),Tell Spring which parameter is used to receive exceptions * * * 3),Exception exception: Specify which exceptions can be received by the notification method (generally written to the big aspect) * * It's kind of like an ajax request * ajax.post(url,function(abc){ * alert(abc); * }) * */ //Want to execute after the target method has an exception // @AfterThrowing(value = "execution(public int com.pzx2.impl.MyMathCalculator.*(int ,int ))",throwing="exception") @AfterThrowing(value = "hahaMyPoint()",throwing="exception") public static void logException(JoinPoint joinPoint , Exception exception) { Signature signature = joinPoint.getSignature(); String name = signature.getName(); System.out.println("[LogUtils-abnormal]["+name+"]An exception occurred in the method. The exception information is"+exception+ ":The test team has been notified for troubleshooting"); }
- @Around: surround (the most powerful) surround notification
Execution order of the four notification methods
try { @Before method.invoke(obj,args); @AfterReturning } catch (Exception e) { @AfterThrowing } finally { @After }
Several common ways of writing pointcut expressions
Fixed writing of pointcut expression Fixed format: execution(The access permission character returns the full signature of the value type method(Parameter type)) /** * The writing method of pointcut expression; * Fixed format: execution (full signature (parameter type) of the return value type method of the access authority character) * Wildcard: * * : 1),Match one or more characters: execution (public int com. Pzx2. Impl. Mymath *. * (int, int)) * 2),Match any parameter: the first parameter is of type int, and the second parameter is of any type; (a total of two types are matched) * execution(public int com.pzx2.impl.MyMathCalculator.*(int ,* )) * 3),Only one layer path can be matched * 4),The * of the permission position cannot represent any permission value. If the permission position is not written, it will have any access modifier in the table (public [optional]) * * * .. : 1),Match any number of parameters * execution(public int com.pzx2.impl.MyMathCalculator.*(..)) * 2)Match any multilayer path * execution(public int com.pzx2.impl..MyMathCalculator.*(..)) * * Remember two: * The most accurate way to write: execution(public int com.pzx2.impl.MyMathCalculator.add(int,int)) * The most ambiguous: execution(* *. * (..): Never write * * You can also use the following expression * "&&","||","!" * MyMathCalculator.add(int , double) * The pointcut expression must satisfy the following two expressions * execution(public int com.pzx..MyMath*.*(..)) && execution(* *.*(int,int)) *||: Satisfy an expression * * */
Extract the pointcut expression and reuse it (for references in the same class, you can directly use the method name plus the parameter list, instead of the full class name for references between the same classes)
/** Extract reusable pointcut expressions; * 1,Arbitrarily declare an empty method that returns void without implementation * 2,Mark @ Pointcut on the method */ @Pointcut("execution(* com.pzx2.impl..MyMathCalculator.*(..))") public void hahaMyPoint(){ }
Surround notification code demo
/** * @Around :Surround notification is the most powerful notification method in Spring * @Around: Surround dynamic agent; * * try{ * //Before advice * method.invoke(obj,args); * //Return notification * }catch(Exception e){ * //Exception notification * }finally{ * //Post notification * } * * Four in one is circular notice * There is a parameter in the surround notification: ProceedingJoinPoint pjp * * Surround notification: it is executed prior to ordinary notification, and the execution order is; * [Common method execution] * { * try{ * Surround front * Surround execution: target method execution * Surround return * }catch(){ * Surround exception * }finally{ * Surround rear * } * * * * * } * [General post] * [Normal method return / method exception] * * * New order; * Note: This is the running sequence of spring 4. Wrap around the front -- > normal method front -- > target method execution -- > wrap around the normal return / exception -- > wrap around the rear -- > normal post -- > normal return or exception * Spring5 In different order * * * */ @Around("hahaMyPoint()") public Object myAround(ProceedingJoinPoint pjp) { Object proceed=null; Signature signature = pjp.getSignature(); String name = signature.getName(); try { Object[] args = pjp.getArgs(); //The method of using reflection to advance the target is method invoke(obj,args); //Before System.out.println("[[surround front][" + name + "[method start]"); proceed = pjp.proceed(args); //@AfterReturning System.out.println("[[return notice]["+name+"Method return, return value"+proceed+"]"); } catch (Throwable throwable) { //@AfterThrowing System.out.println("[[exception in surround method][" + name + "]The method has an exception. The reason for the exception is:" + throwable); //In order to let the outside world know the reason, this anomaly must be thrown out throw new RuntimeException(throwable); } finally { // @After() System.out.println("[[post notification][" + name + "]Method end"); } //After the reflection call, the return value must also be returned return proceed; }
The method execution order when the normal method is executed with the wrap method
Execution order of spring 5
Surround pre – > normal method pre – > target method execution – > normal return / exception – > normal post – > surround normal return / exception – > surround post
Multi slice execution sequence
xml format configuration notification method
<!-- need AOP Namespace --> <aop:config> <!-- Declare a global pointcut expression --> <aop:pointcut id="globalPoint" expression="execution(* com.xml.impl.*.*(..))"/> <!-- Specify cut plane @Aspect --> <aop:aspect ref="logUtils"> <!-- Configure which method is pre notification;method Specify method name logStart@Before("Pointcut expression") --> <!-- This expression can only be used in the current aspect --> <aop:pointcut id="mypoint" expression="execution(* com.xml.impl.*.*(..))"/> <aop:before method="logStart" pointcut-ref="mypoint"/> <aop:after-returning method="logReturn" returning="result" pointcut-ref="mypoint"/> <aop:after-throwing method="logException" throwing="exception" pointcut-ref="mypoint"></aop:after-throwing> <aop:after method="logEnd" pointcut-ref="mypoint"></aop:after> <aop:around method="myAround" pointcut-ref="mypoint"></aop:around> </aop:aspect> <aop:aspect ref="validateApsect"> <aop:before method="logStart" pointcut-ref="globalPoint"></aop:before> <aop:after-returning method="logReturn" returning="result" pointcut-ref="globalPoint"></aop:after-returning> <aop:after-throwing method="logException" throwing="exception" pointcut-ref="globalPoint"></aop:after-throwing> <aop:after method="logEnd" pointcut-ref="globalPoint"></aop:after> </aop:aspect> </aop:config>
The higher version of tomcat breaks error 405 when spring MVC uses DELETE and PUT requests
Solution: add
<%@ page contentType="text/html;charset=UTF-8" language="java" isErrorPage="true" %> In fact, the main thing is to add isErrorPage="true" ,tell tomcat Errors may be reported
ModelAttribute principle
Running process of spring MVC
When integrating Spring MVC and Spring, Spring services cannot automatically assemble the Controller of Spring MVC because Spring is used as the parent container and Spring MVC as the child container by default. The child container can take the things of the parent container, and the parent container cannot take the child container
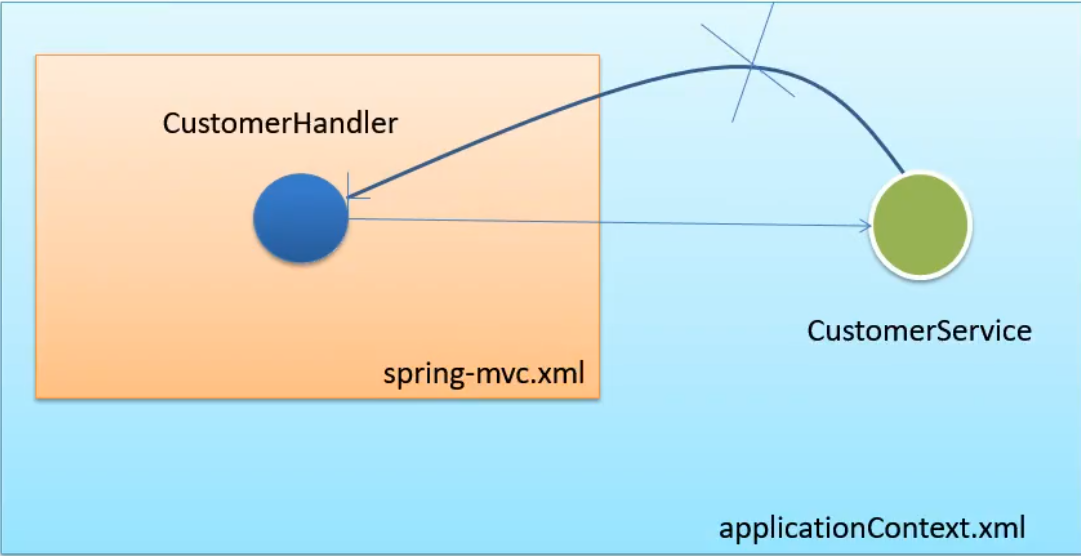
Simple record of knowledge points in mybatis
https://199604.com/709