1. Introduction to interruption
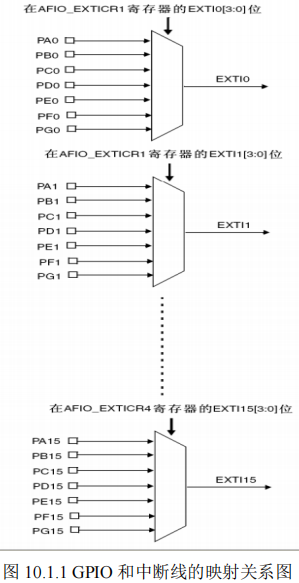
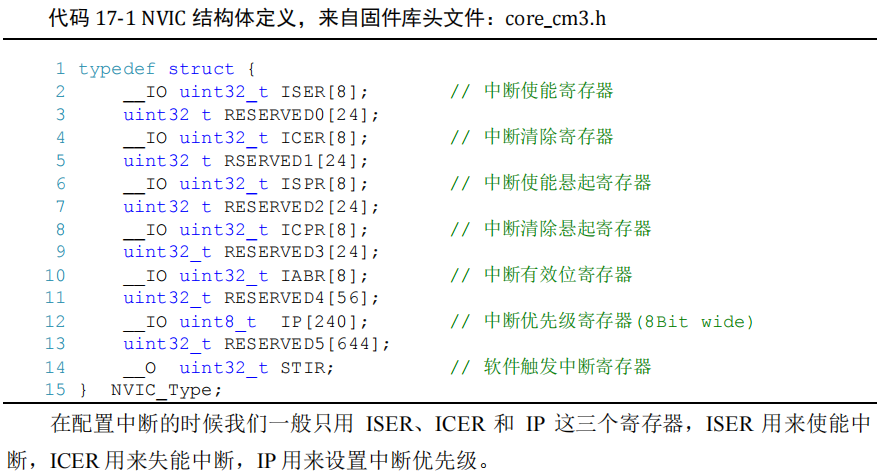
misc.h//NVIC setting system interrupt management

Sequence of interrupt programming
1 - enable interrupt request
static void EXTI_NVIC_Config() { NVIC_InitTypeDef NVIC_InitStruct; NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1); NVIC_InitStruct.NVIC_IRQChannel = EXTI0_IRQn; NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 1; NVIC_InitStruct.NVIC_IRQChannelSubPriority = 1; NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE; NVIC_Init(&NVIC_InitStruct); }
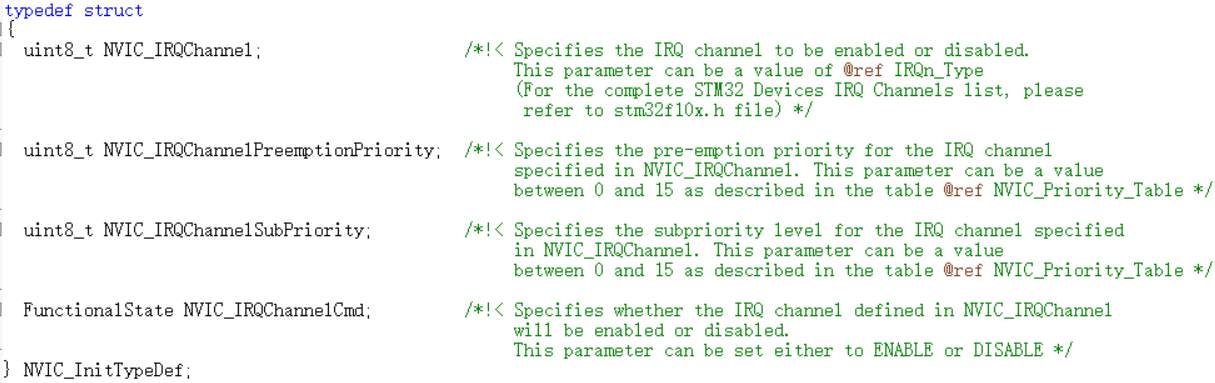
(For the complete STM32 Devices IRQ Channels list, please refer to stm32f10x.h file)
Want to know STM32 For this list, refer to stm32f10x.h (interrupt source)
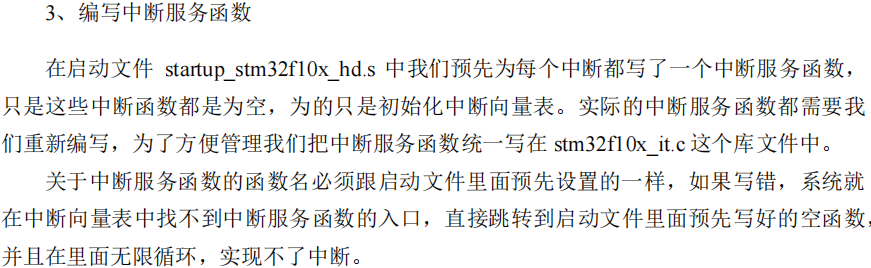
void EXTI0_IRQHandler(void) { if(EXTI_GetITStatus(EXTI_Line0) != RESET ) { LED_G_TOGGLE; } EXTI_ClearITPendingBit(EXTI_Line0); }
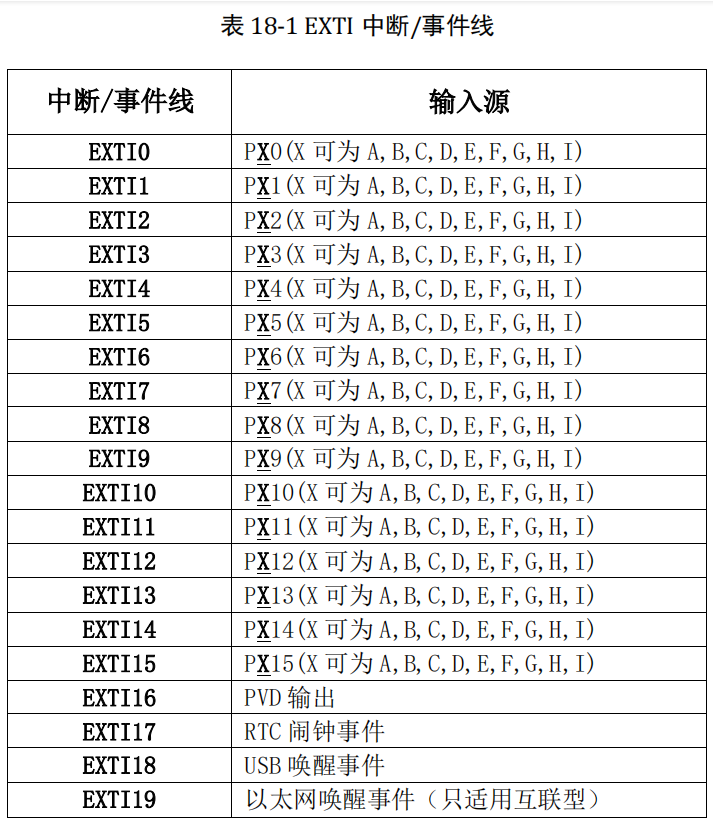
EXTI initialization structure
EXTI_InitTypeDef
typedef struct
{
uint32_t EXTI_Line; / / Used to generate interrupt / event lines
EXTIMode_TypeDef EXTI_Mode; / / Exti mode (interrupt / event)
EXTITrigger_TypeDef EXTI_Trigger; / / Trigger (up / down / up / down)
FunctionalState EXTI_LineCmd; / / To enable or disable
}EXTI_InitTypeDef;
Select the GPIO line as the external interrupt
/** * @brief Selects the GPIO pin used as EXTI Line. * @param GPIO_PortSource: selects the GPIO port to be used as source for EXTI lines. * This parameter can be GPIO_PortSourceGPIOx where x can be (A..G). * @param GPIO_PinSource: specifies the EXTI line to be configured. * This parameter can be GPIO_PinSourcex where x can be (0..15). * @retval None */ void GPIO_EXTILineConfig(uint8_t GPIO_PortSource, uint8_t GPIO_PinSource) { uint32_t tmp = 0x00; /* Check the parameters */ assert_param(IS_GPIO_EXTI_PORT_SOURCE(GPIO_PortSource)); assert_param(IS_GPIO_PIN_SOURCE(GPIO_PinSource)); tmp = ((uint32_t)0x0F) << (0x04 * (GPIO_PinSource & (uint8_t)0x03)); AFIO->EXTICR[GPIO_PinSource >> 0x02] &= ~tmp; AFIO->EXTICR[GPIO_PinSource >> 0x02] |= (((uint32_t)GPIO_PortSource) << (0x04 * (GPIO_PinSource & (uint8_t)0x03))); }
exit.c:
#include "exti.h" static void EXTI_NVIC_Config() { NVIC_InitTypeDef NVIC_InitStruct; NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1); NVIC_InitStruct.NVIC_IRQChannel = EXTI0_IRQn; NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 1; NVIC_InitStruct.NVIC_IRQChannelSubPriority = 1; NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE; NVIC_Init(&NVIC_InitStruct); } void EXTI_Key_Config(void) { GPIO_InitTypeDef GPIO_InitStruct; EXTI_InitTypeDef EXTI_InitStruct; //Configure interrupt priority EXTI_NVIC_Config(); //Initialize GPIO RCC_APB2PeriphClockCmd(KEY_UP_GPIO_CLK, ENABLE); GPIO_InitStruct.GPIO_Pin =KEY_UP_GPIO_PIN; GPIO_InitStruct.GPIO_Mode =GPIO_Mode_IN_FLOATING; GPIO_InitStruct.GPIO_Speed=GPIO_Speed_50MHz; GPIO_Init(KEY_UP_GPIO_PORT, &GPIO_InitStruct); //Initialize EXTI RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE); GPIO_EXTILineConfig(GPIO_PortSourceGPIOA, GPIO_PinSource0); EXTI_InitStruct.EXTI_Line = EXTI_Line0; EXTI_InitStruct.EXTI_Mode = EXTI_Mode_Interrupt; EXTI_InitStruct.EXTI_Trigger = EXTI_Trigger_Rising; EXTI_InitStruct.EXTI_LineCmd = ENABLE; EXTI_Init(&EXTI_InitStruct); }
Just call it in the main function
EXTI_Key_Config(void);
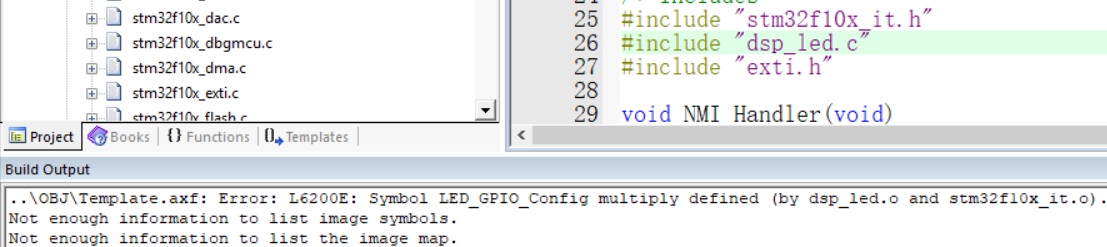
..\OBJ\Template.axf: Error: L6200E: Symbol LED_GPIO_Config multiply defined (by dsp_led.o and stm32f10x_it.o).
Not enough information to list image symbols.
Not enough information to list the image map.
It is found that the header file #include "dsp_led.c" is written incorrectly!!!
exti\exti.h(9): warning: #1295-D: Deprecated declaration EXTI_NVIC_Config - give arg types
Reason: static void EXTI_NVIC_Config()