The last article shared a learning website, Share a computer vision - deep learning website - highly recommended , a classmate learned the content of object counting inside.
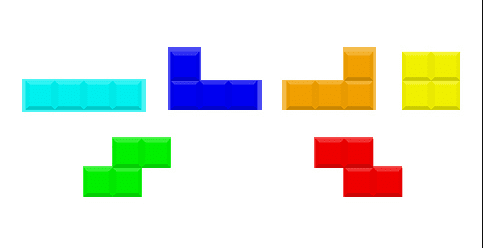
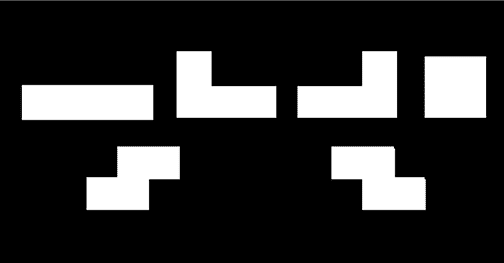
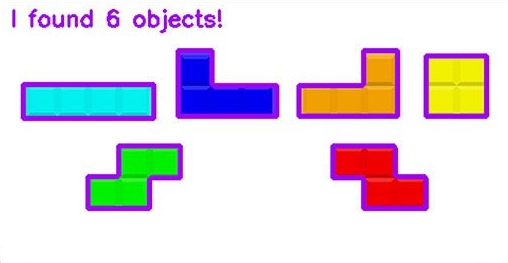
After learning, change to other picture tests and find that they can't.
Like the following.
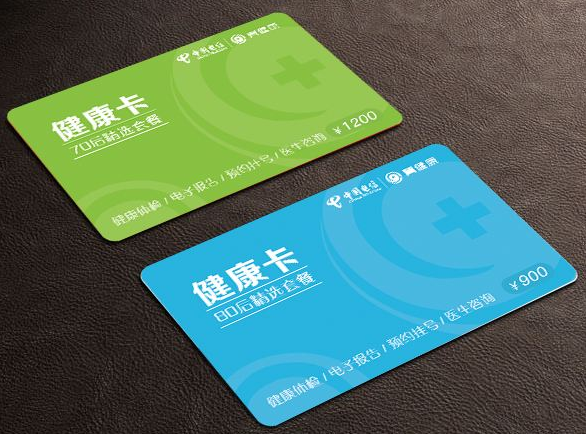
The reason why this problem can't be distinguished from the background is that the target can't be well distinguished from the background.
Generally, image preprocessing involves the following processes:
Convert to grayscale image.
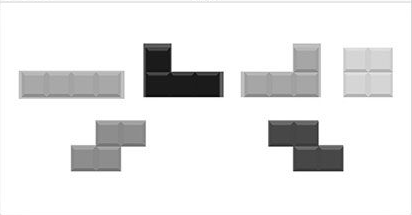
Detect object edge
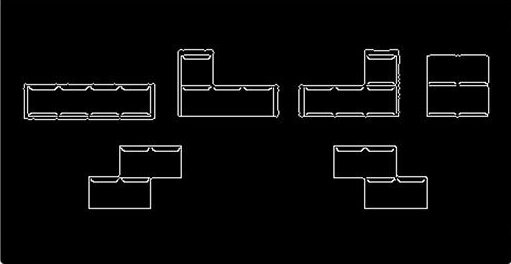
Threshold processing
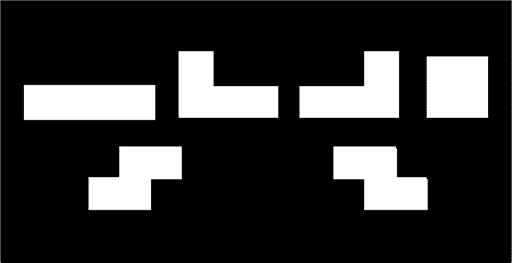
Solid color background is generally OK here. The target and background can be well distinguished.
Finally, the corresponding number of objects is calculated by finding the number of contours of the object.
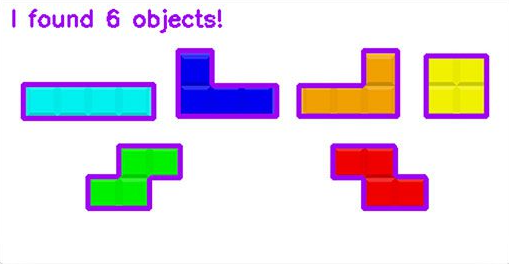
However, in the other picture above, the background is not uniform, so it is difficult to distinguish it well. Even after being distinguished by HSV, there are still some small noise data.
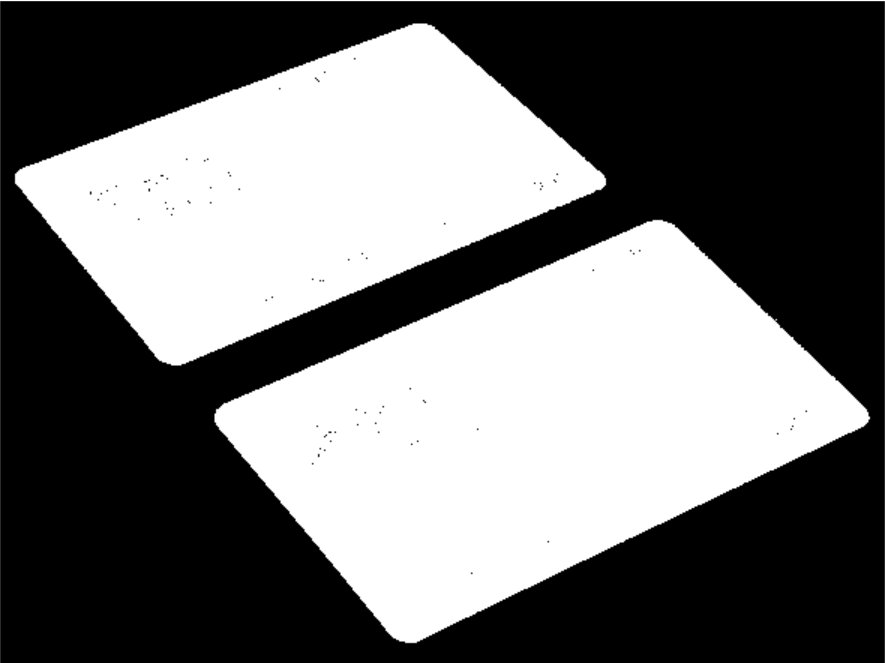
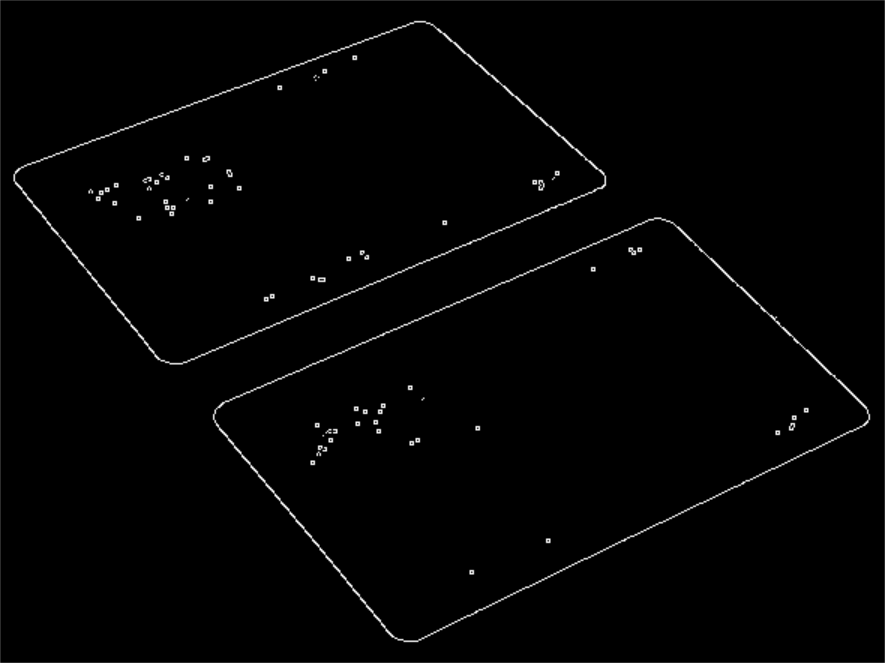
At this time, it can be removed after a round of expansion and corrosion operation.
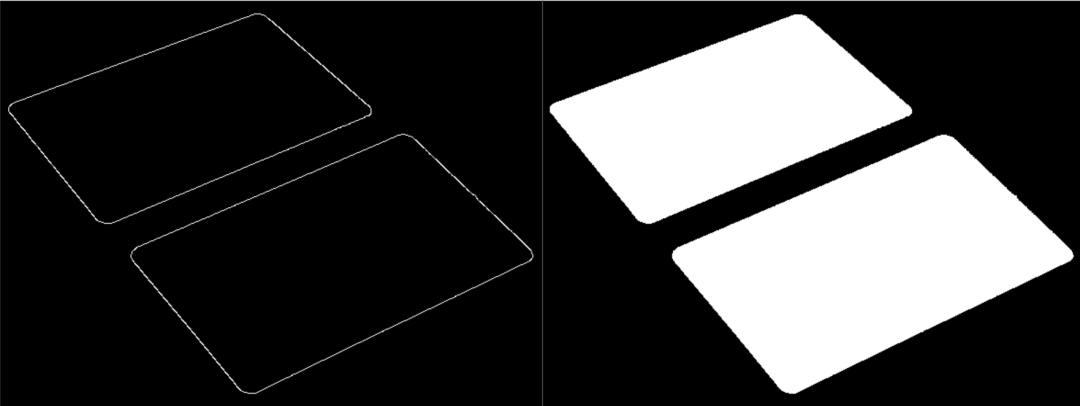
Full code:
Without machine learning, only relying on the traditional image processing method to achieve object recognition, we need to be very skilled in these basic operations of image processing, carry out various preprocessing on the pictures, and get a relatively simple background picture, which is convenient to distinguish the foreground from the background.import cv2 import numpy as np img = cv2.imread('card1.jpg') imgHSV = cv2.cvtColor(img,cv2.COLOR_BGR2HSV) lower = np.array([12,0,162]) upper = np.array([179,255,255]) # Gets the mask within the specified color range mask = cv2.inRange(imgHSV,lower,upper) # Perform bitwise and operation on the original image, and the mask area is reserved imgResult = cv2.bitwise_and(img,img,mask=mask) # Expansion and corrosion mask = cv2.dilate(mask, None, iterations=1) mask = cv2.erode(mask, None, iterations=1) # Edge detection edges = cv2.Canny(mask, 30, 70) # Find profile cnts = cv2.findContours(mask, cv2.RETR_EXTERNAL,cv2.CHAIN_APPROX_SIMPLE)[0] output = img.copy() # Draw an outline for c in cnts: cv2.drawContours(output, [c], -1, (240, 0, 159), 3) cv2.imshow("Contours", output) cv2.imshow('edges', edges) cv2.imshow("Mask", mask) cv2.waitKey(0)
I wrote it two years ago How to use python to identify verification code and license plate number? (the payment function was tested at that time, and I'll update the following content next time). The OCR software used at that time recognized it, but the picture can't be more complex. It needs to be preprocessed. Limited to the technical level at that time, it was not realized in a good way, and all kinds of image processing was troublesome.
Looking at it again today, I think I should be able to achieve it well. Just get letters and numbers through the above methods. Recognition needs to train a model or find a large number of numbers and letter pictures, label them, match them one by one, and find the one with the highest matching degree.
Of course, there are many ready-made machine learning libraries. After mastering the process, it is relatively simple to set a template. Here is how to identify numbers.
# Import picture library import matplotlib.pyplot as plt import cv2 # Import dataset, classifier, data segmentation method from sklearn import datasets, svm from sklearn.model_selection import train_test_split import numpy as np # Load dataset digits = datasets.load_digits() # Change the data of 8 * 8 into 64 * 1 n_samples = len(digits.images) data = digits.images.reshape((n_samples, -1)) # Create classifier clf = svm.SVC(gamma=0.001) # Split data set, 50% of training data and 50% of test data X_train, X_test, y_train, y_test = train_test_split( data, digits.target, test_size=0.5, shuffle=False ) # print(X_test) # Training model clf.fit(X_train, y_train) # Predictive test set print(X_test[:1]) predicted = clf.predict(X_test[:1]) print(predicted[:4]) # Predict a new picture img = cv2.imread('1.jpg') img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) img[img>0]=255 img = cv2.resize(img,(8,8)) data = img.reshape(64,) r= clf.predict(data) print(r)
Today's question: Find the edge of the picture (you can't use ready-made algorithms.)
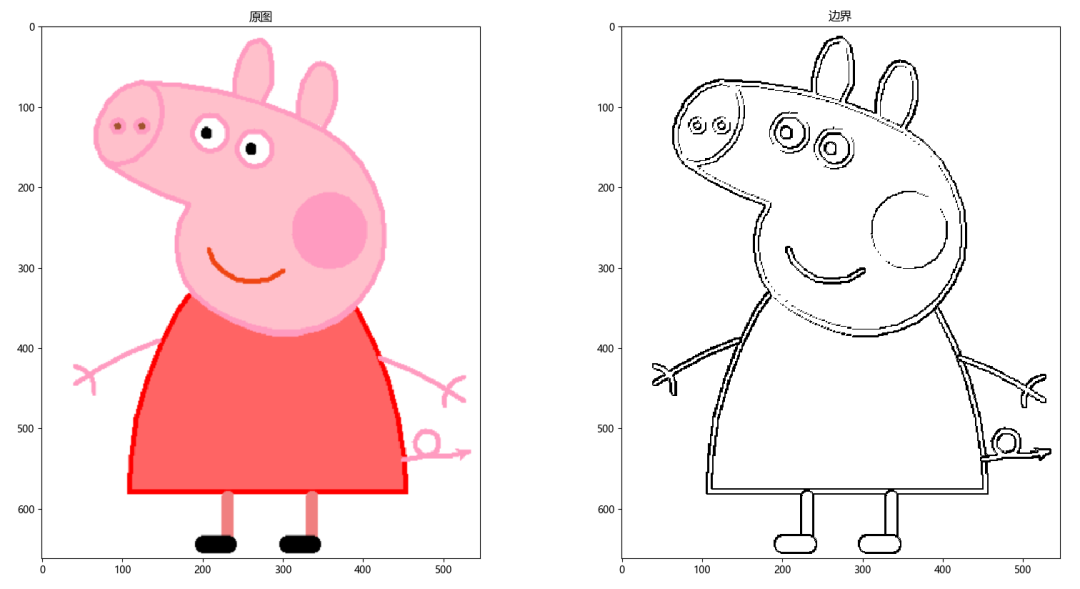