Young GC simulating JVM
JVM parameter demonstration (based on JDK 1.8)
Run the code with the following JVM parameters:
# Initial Cenozoic size 5M -XX:NewSize=5242880 # Maximum Cenozoic size 5M -XX:MaxNewSize=5242880 # Initial heap size 10M -XX:InitialHeapSize=10485760 # Maximum heap size 10M -XX:MaxHeapSize=10485760 -XX:SurvivorRatio=8 # The threshold for large objects is 10MB -XX:PretenureSizeThreshold=10485760 -XX:+UseParNewGC -XX:+UseConcMarkSweepGC
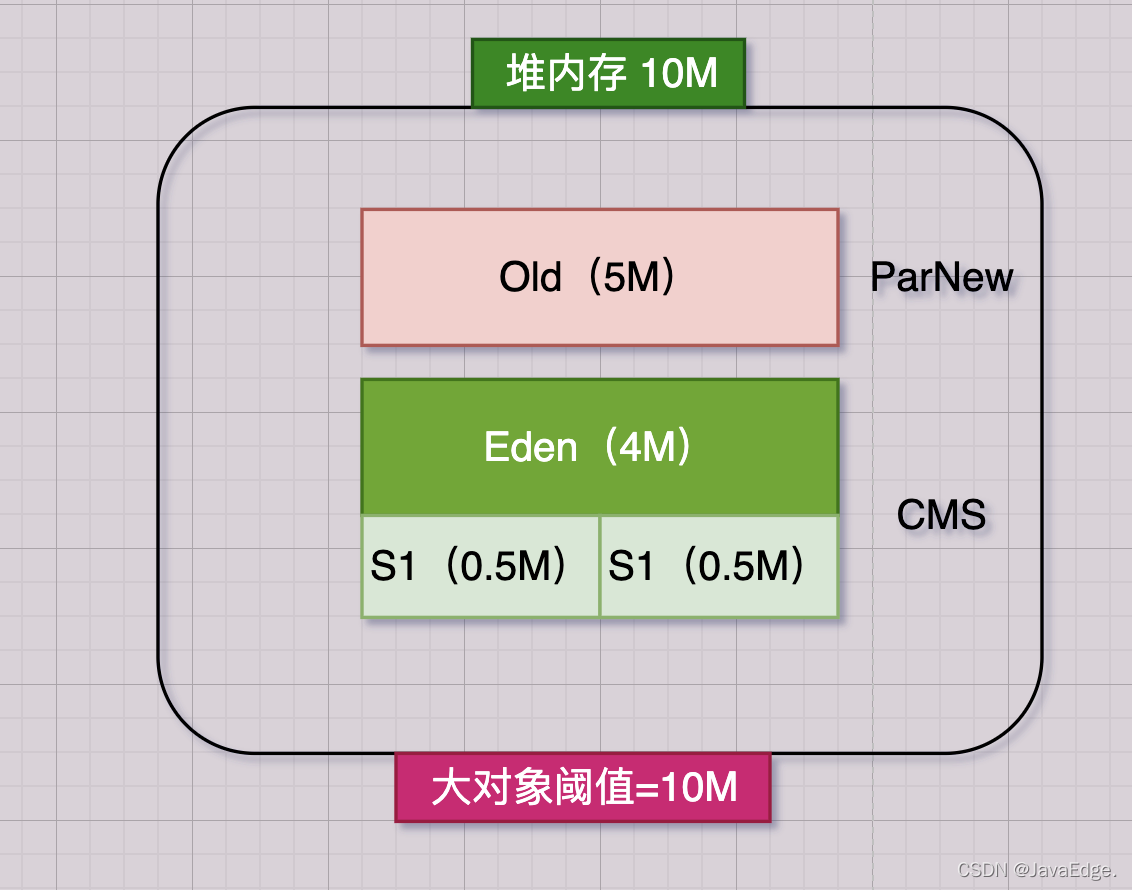
How to print JVM GC logs?
GC log print selection:
-XX:+PrintGCDetils: Print details gc journal -XX:+PrintGCTimeStamps: This parameter can be printed out every time GC Time of occurrence -Xloggc:gc.log: This parameter can be set to gc The log is written to a disk file
After adding this parameter, the JVM parameters are as follows:
-XX:NewSize=5242880 -XX:MaxNewSize=5242880 -XX:InitialHeapSize=10485760 -XX:MaxHeapSize=10485760 -XX:SurvivorRatio=8 -XX:PretenureSizeThreshold=10485760 -XX:+UseParNewGC -XX:+UseConcMarkSweepGC -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log
example
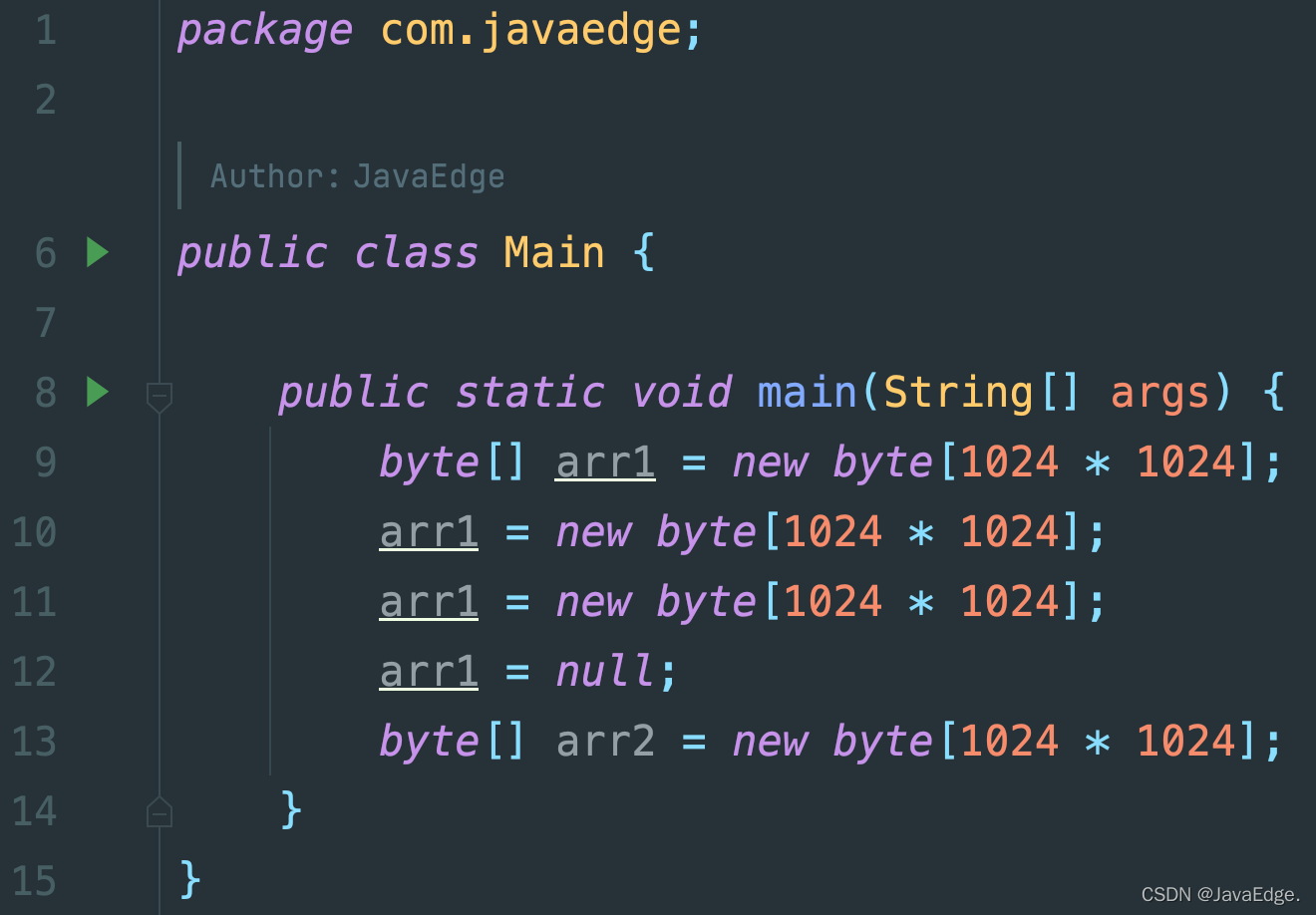
How are objects assigned in Eden
byte[] array1 = new byte[1024 * 1024];
This line will put a 1M array into JVM Eden, and press a main method stack frame into the virtual machine stack of main thread. There is a "arr1" variable inside the stack frame, which points to the 1M array of Eden:
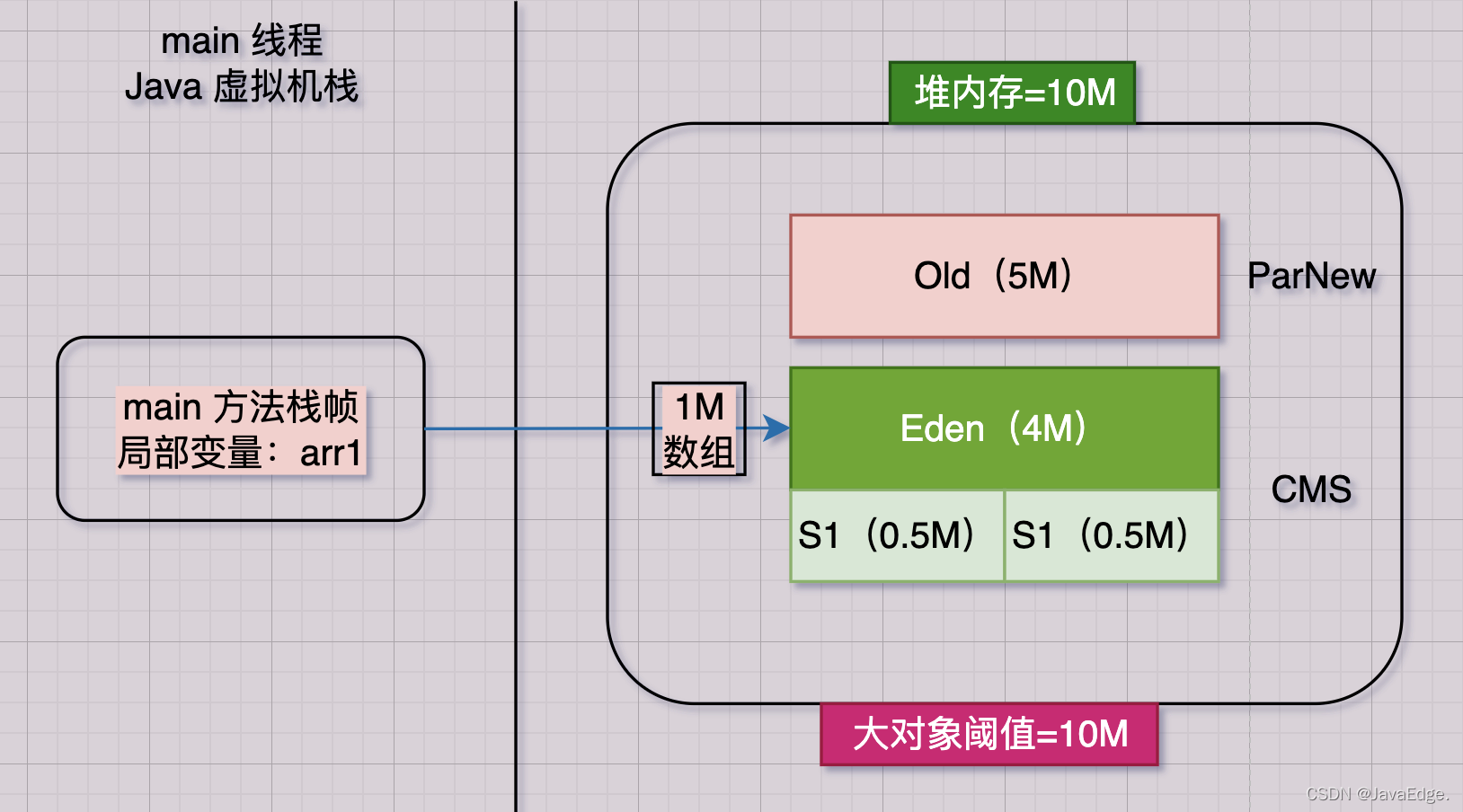
arr1 = new byte[1024 * 1024];
A second array is created in Eden with local variables pointing to it. Then the first array is unreferenced and becomes garbage:
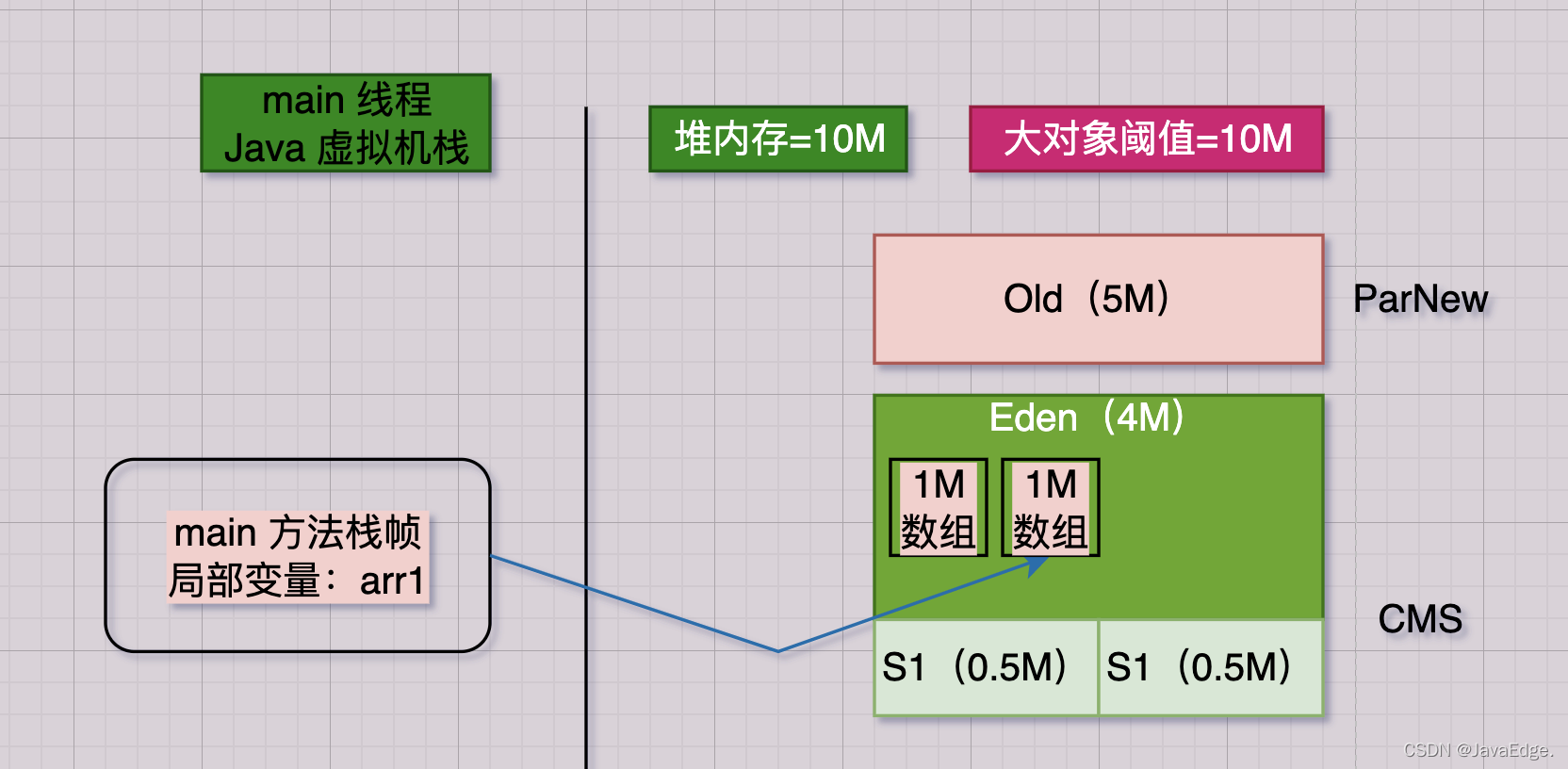
byte[] array1 = new byte[1024 * 1024];
Create the third array in Eden and let arr1 point to the third array. At this time, the first two arrays are unreferenced and become garbage:
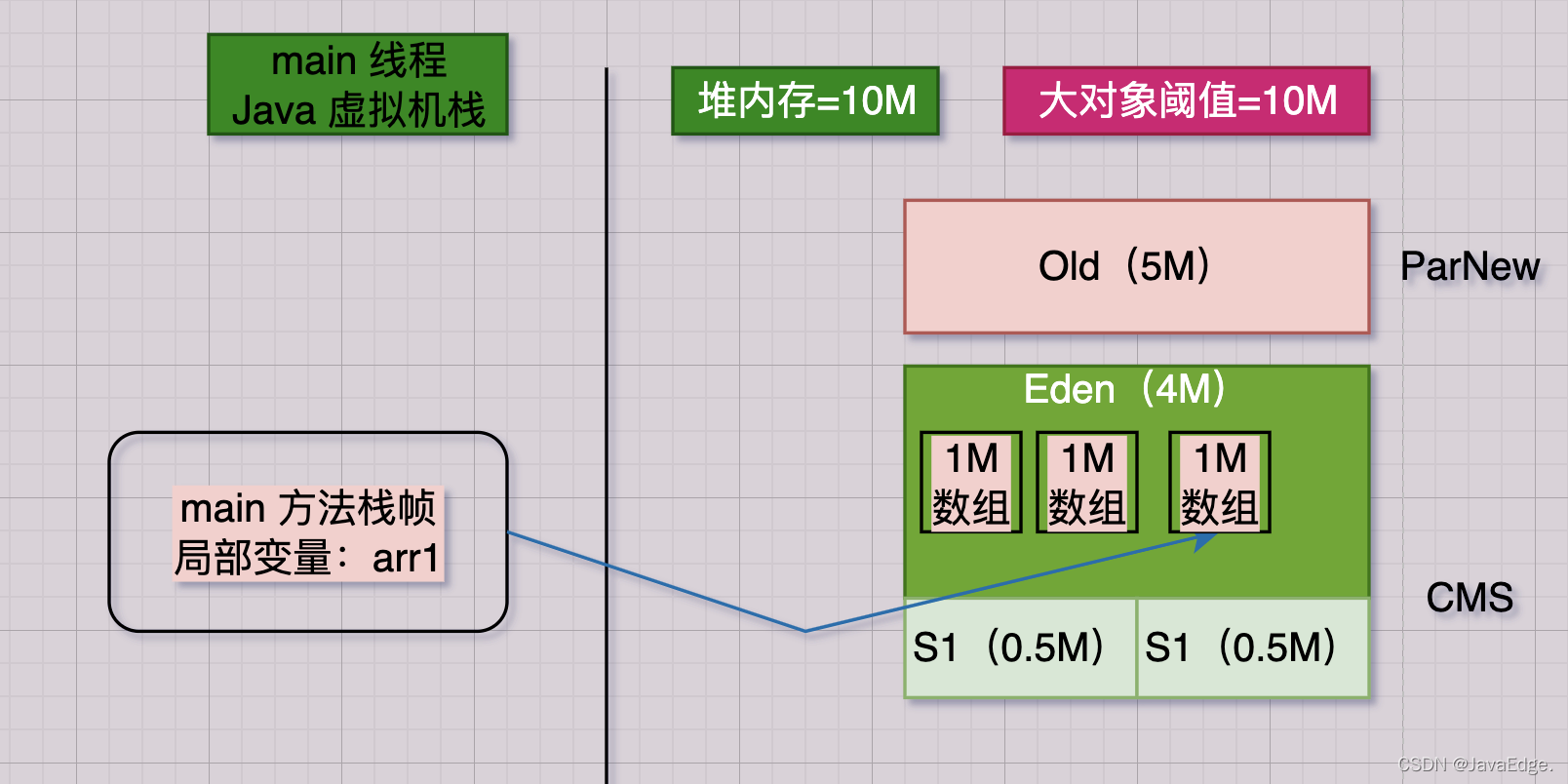
arr1 = null;
arr1 doesn't mean anything, causing all the 3 arrays created before to become garbage:
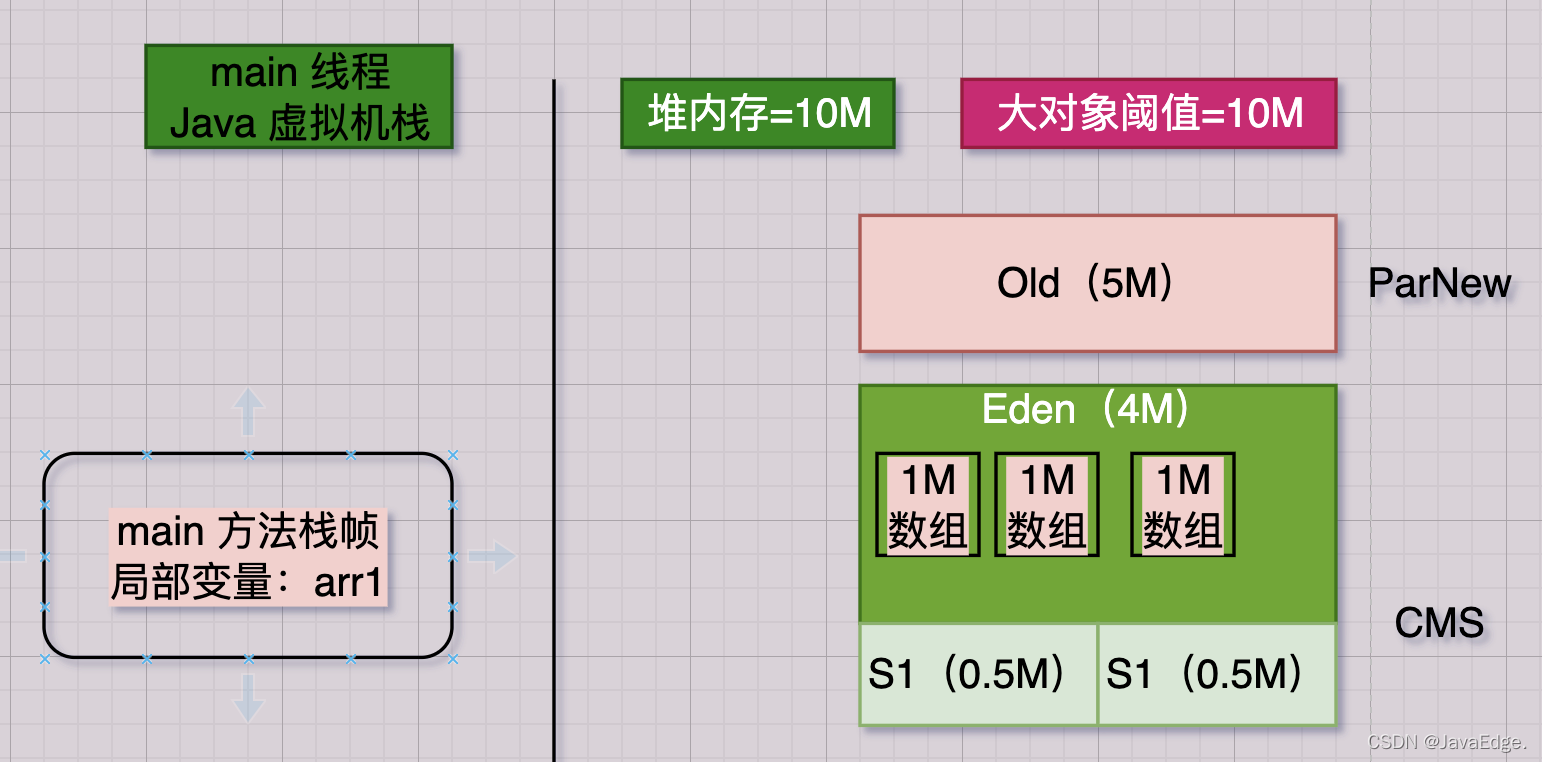
byte[] arr2 = new byte[2 * 1024 * 1024];
Allocate a 2MB array and try to put it in Eden. Can Eden put it in?
Obviously not. Eden is 4M in total. Three 1M arrays have been put into it, leaving only 1M, so Y-GC will be triggered at this time.
Run the program with the specified JVM parameters
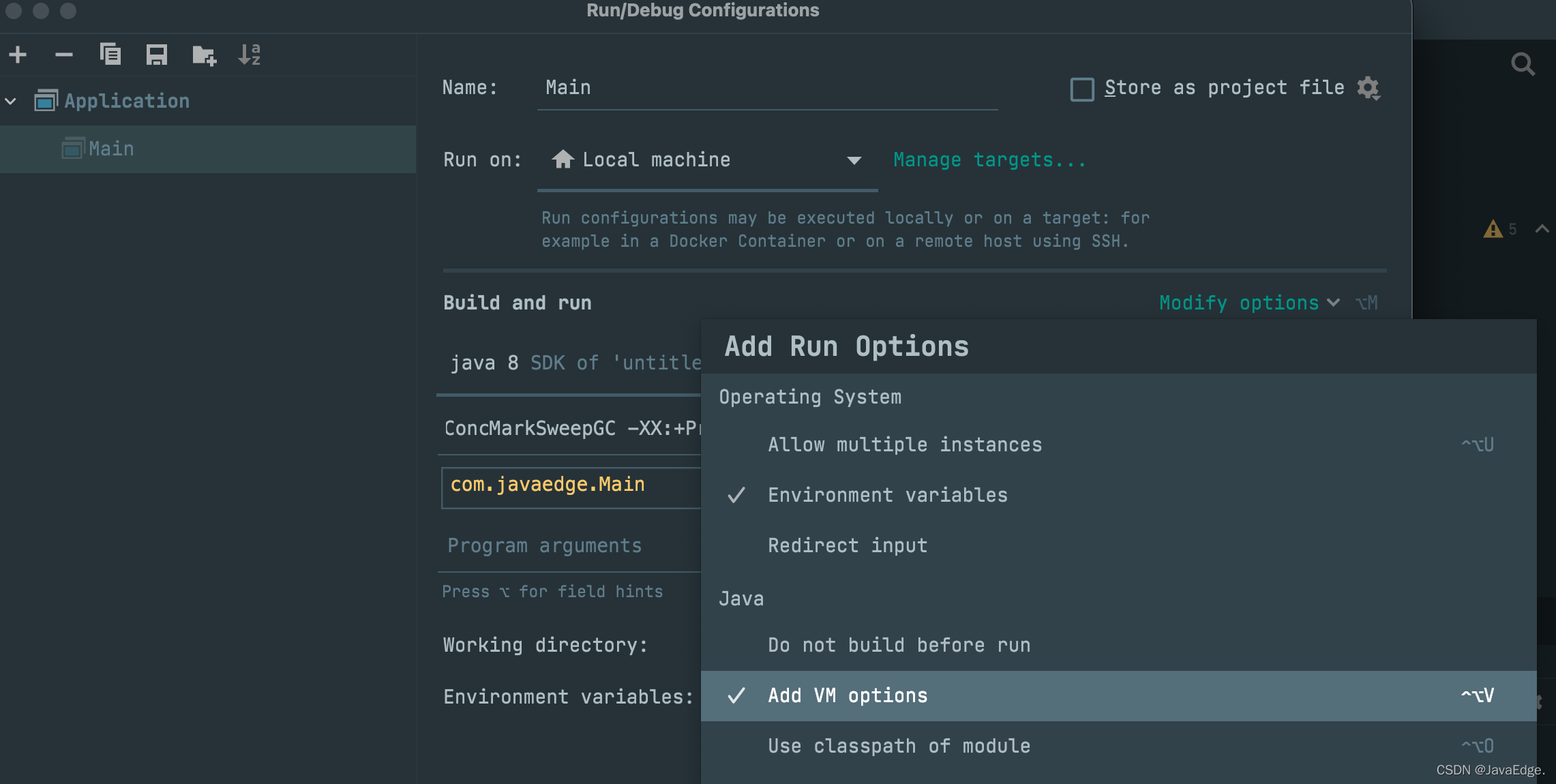
Then you can run it. After running, gc Log file, that is, the gc log of this program run:
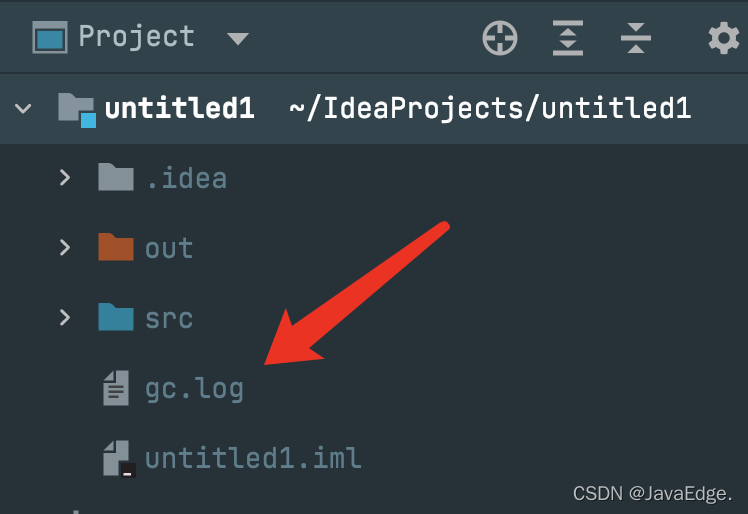
Open gc Log file, we will see the gc log as follows:
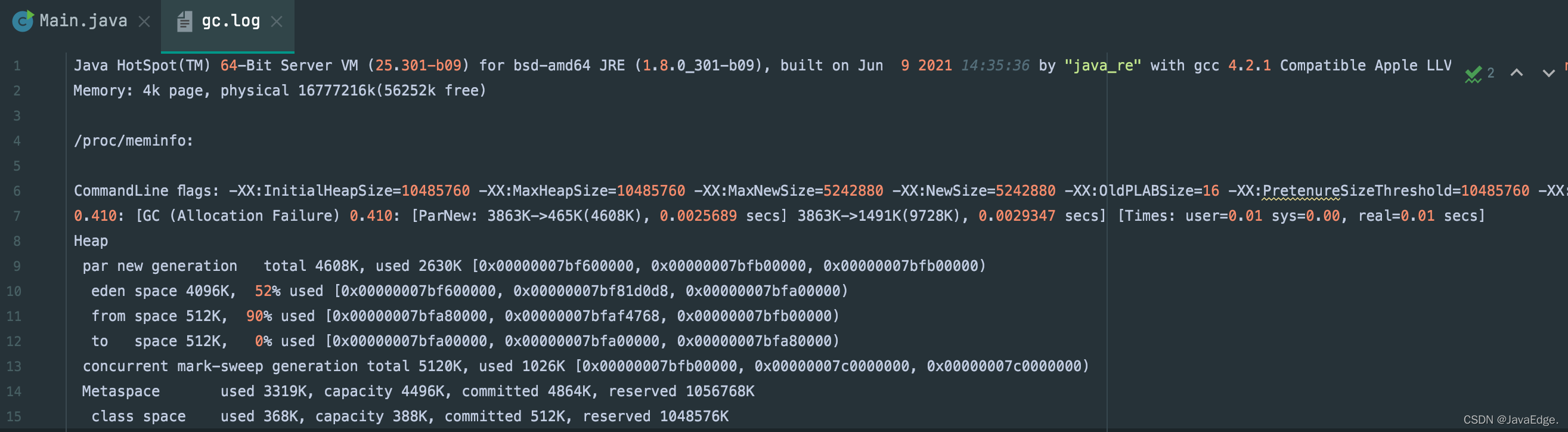
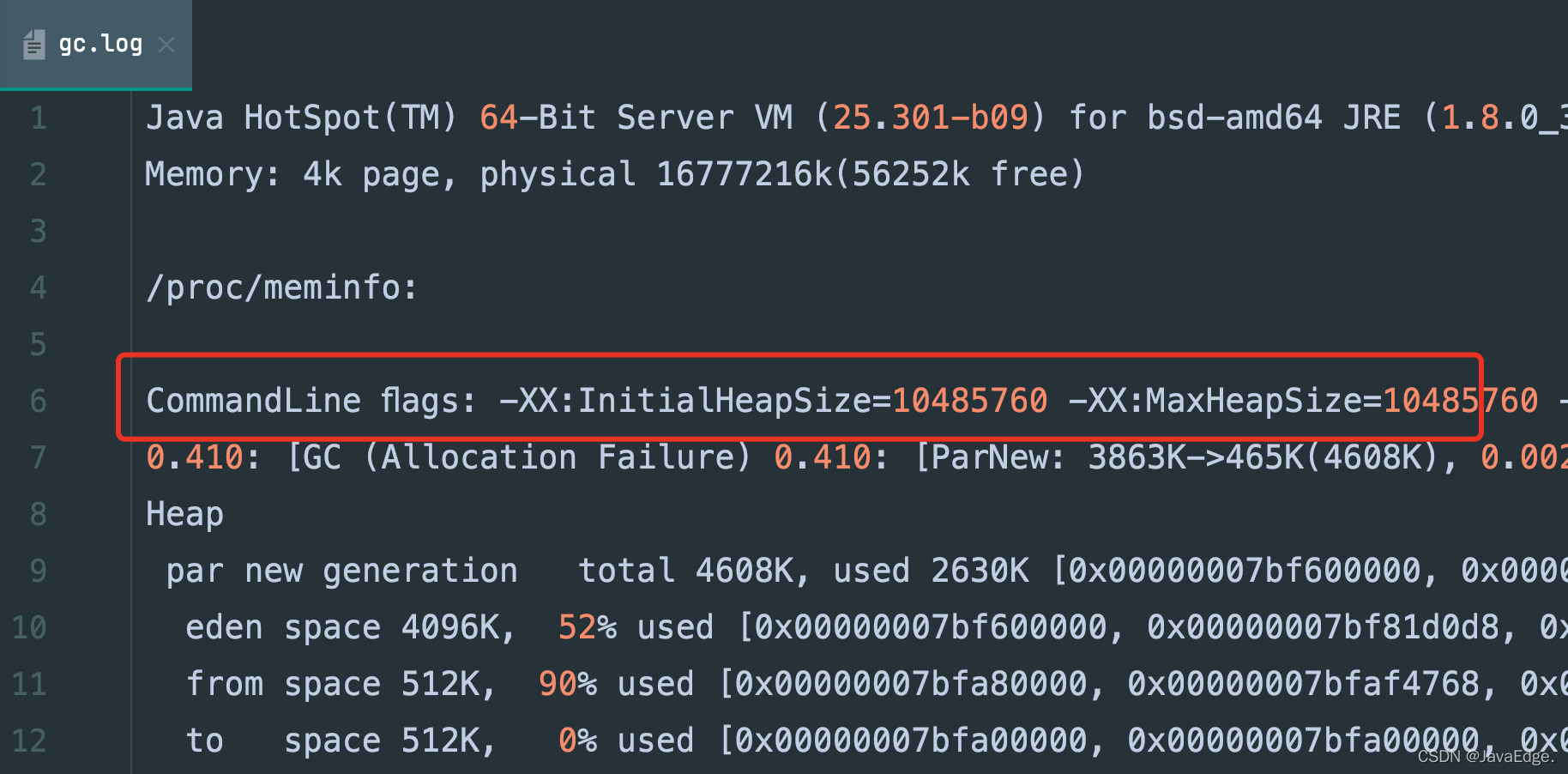
The JVM parameters of this running program include:
- We set it manually
- Default parameter settings Add a parameter to the JVM to print gc logs, and you can see the default parameter configuration of the JVM here
A journey of GC
0.410: [GC (Allocation Failure) 0.410: [ParNew: 3863K->465K(4608K), 0.0025689 secs] 3863K->1491K(9728K), 0.0029347 secs] [Times: user=0.01 sys=0.00, real=0.01 secs]
0.269: [ParNew: 4030K->512K(4608K), 0.0015734 secs] 4030K->574K(9728K), 0.0017518 secs]
[Times: user=0.00 sys=0.00, real=0.00 secs]
Outline the implementation of this GC:
- GC (Allocation Failure), literally, why does a GC happen? Because a 2M array needs to be allocated, but Eden is short of memory, so "Allocation Failure", memory allocation fails, so Y-GC must be triggered once.
When will this GC happen?
Look at a number, "0.410": how many seconds after your system runs, the GC occurs. Here is the GC occurring 410ms after the system runs.
ParNew: 3863K->465K(4608K), 0.0025689 secs
Y-GC is triggered here, so the specified ParNew is used to execute GC.
3863K->465K(4608K)
The available space of the new generation is 4608KB, about 4.5MB. Eden is 4M. Only one of the two survivors can be put into the living object, and the other must remain idle. Therefore, the available space of the young generation is Eden+1 Survivor=4.5MB.
3863K - > 465K: perform a GC on the younger generation. 3863K was used before GC, but only 465K objects survived after GC.
Before GC, we only put three 1M arrays in Eden, a total of 3MB, that is, 3072K. Why is 3863K shown here?
- Although the custom created array itself is 1MB, in order to store the array, the JVM will also include some other information, so the actual memory occupied by each array is greater than 1MB
- There may be some objects you can't see in Eden
So before GC, three arrays + other unknown objects = 3863K memory. Before GC, we only put three 1M arrays in Eden, a total of 3MB, that is, 3072K. Why is 3863K shown here?
- Although the custom created array itself is 1MB, in order to store the array, the JVM will also include some other information, so the actual memory occupied by each array is greater than 1MB
- There may be some objects you can't see in Eden
So before GC, three arrays + other unknown objects = 3863K memory.
0.0025689 secs: This GC takes time. From here, it takes about 2.5ms. Only 3MB objects are recycled.
3863K->1491K(9728K), 0.0029347 secs
The memory of the entire Java heap. The total available memory space of the whole Java heap is 9728K (9.5M) = 4.5MB for the younger generation + 5M for the older generation.
- Before GC, the entire Java heap memory used 3863K
- 1491K of Java heap memory was used after GC
[Times: user=0.01 sys=0.00, real=0.01 secs]
The minimum unit of time consumed by this gc is two digits after the decimal point, and the unit is s.
Graphical GC execution process
ParNew executes GC and recycles the three custom created arrays. At this time, because they are not referenced, they will become garbage:
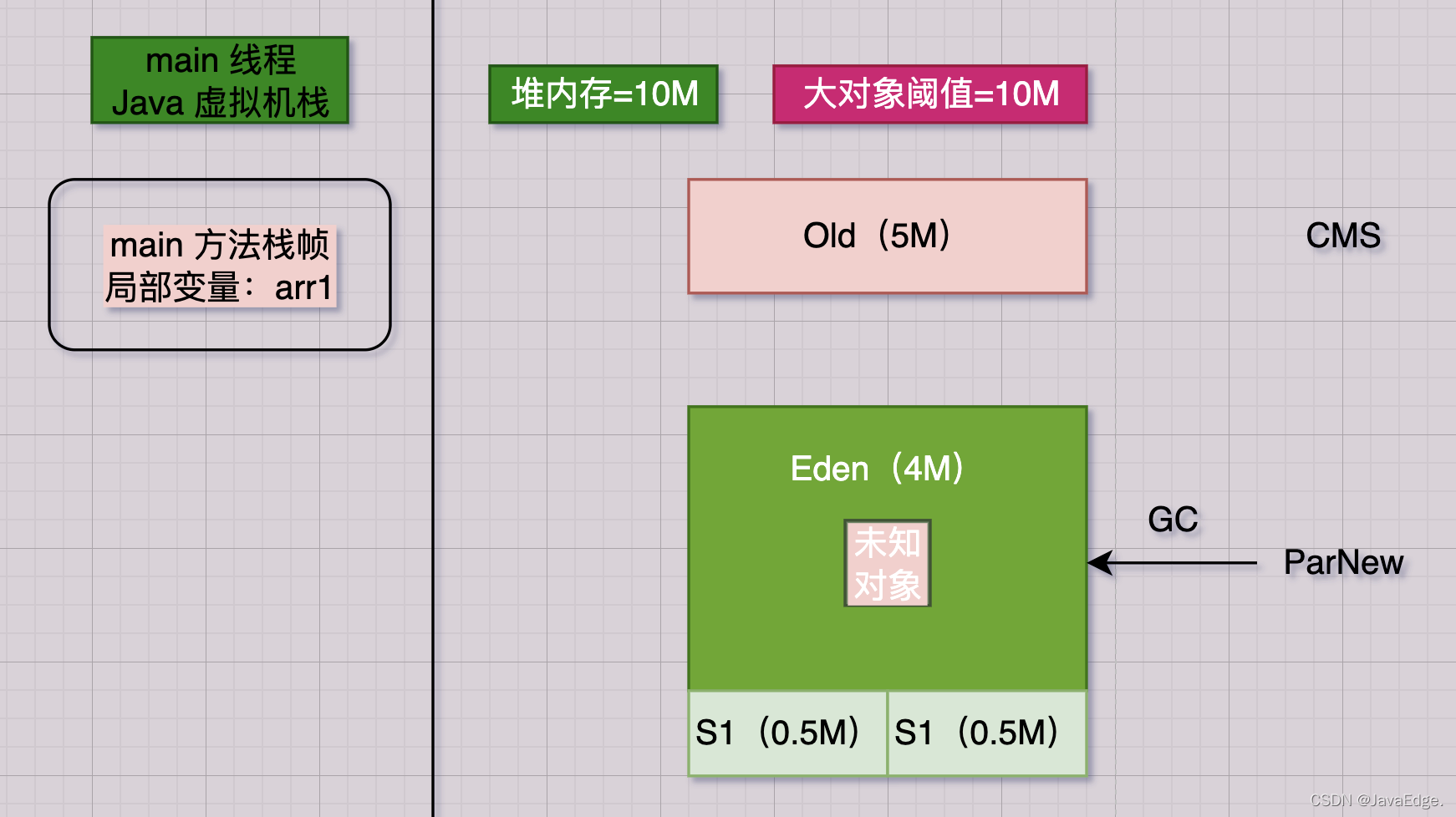
ParNew: 4030K->512K(4608K), 0.0015734 secs
After gc recycling, the memory usage is reduced from 4030K to 512K. That is, 512KB objects in the gc log survived and were transferred from Eden area to Survivor1 area:
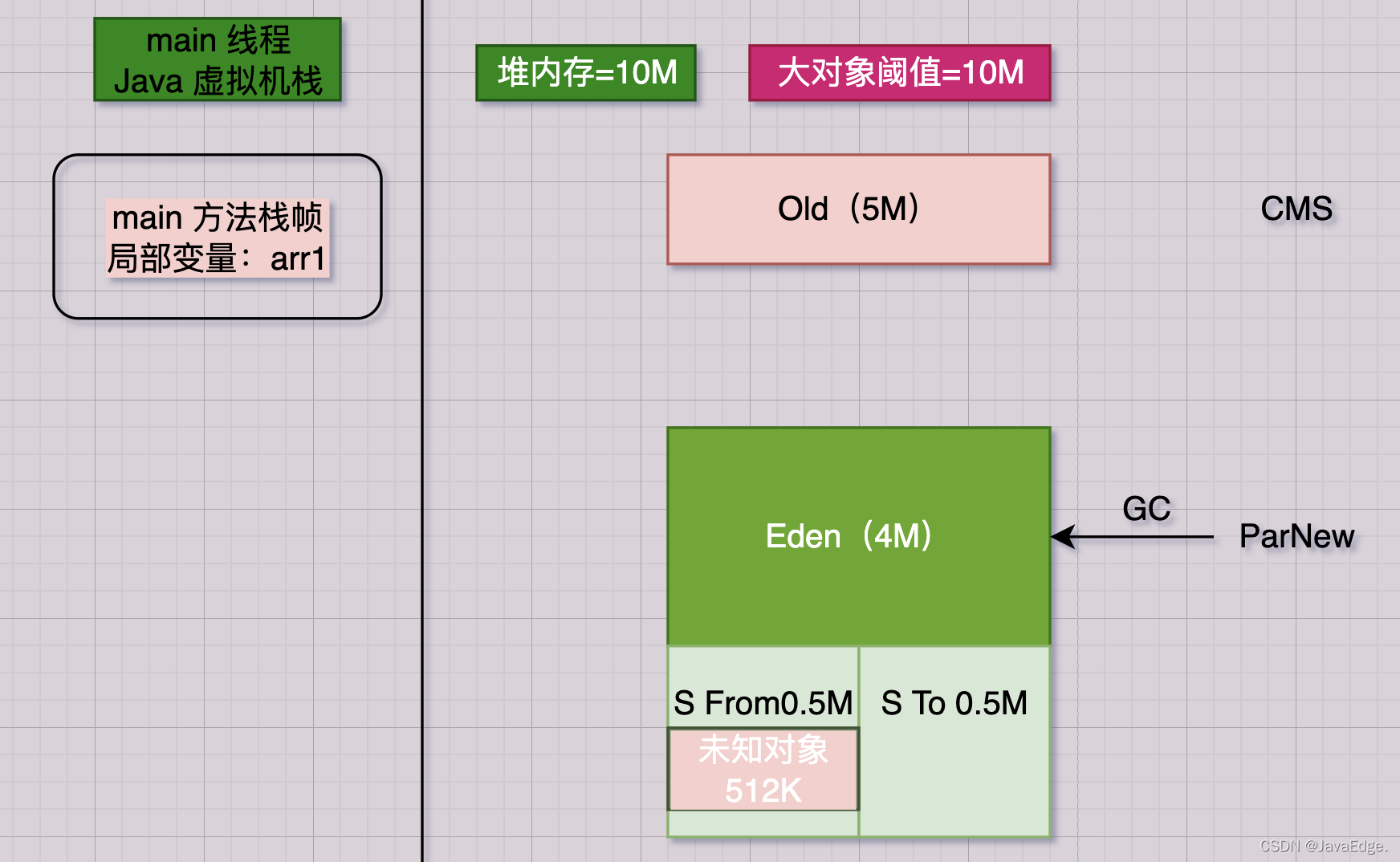
Heap memory after GC
Heap par new generation total 4608K, used 2601K [0x00000000ff600000, 0x00000000ffb00000, 0x00000000ffb00000) eden space 4096K, 51% used [0x00000000ff600000, 0x00000000ff80a558, 0x00000000ffa00000) from space 512K, 100% used [0x00000000ffa80000, 0x00000000ffb00000, 0x00000000ffb00000) to space 512K, 0% used [0x00000000ffa00000, 0x00000000ffa00000, 0x00000000ffa80000) concurrent mark-sweep generation total 5120K, used 62K [0x00000000ffb00000, 0x0000000100000000, 0x0000000100000000) Metaspace used 2782K, capacity 4486K, committed 4864K, reserved 1056768K class space used 300K, capacity 386K, committed 512K, reserved 1048576K
The current heap memory usage printed when the JVM exits

ParNew is responsible for 4608K (4.5MB) of available memory in the Cenozoic, and currently uses 2630K (2.5MB). At this time, before the JVM exits, why does the Cenozoic account for 2.5M? After GC, another 2M array is allocated through the following code:
byte[] arr2 = new byte[2 * 1024 * 1024];
Therefore, at this time, there must be a 2M array = 2048K in Eden. After the last GC, 512K unknown objects survived in From Survivor, then:
2048KB + 512KB = 2560KBEach array will occupy some extra memory to store some metadata of its own object. It can be considered that the extra 70K is the additional memory space used by the array object.
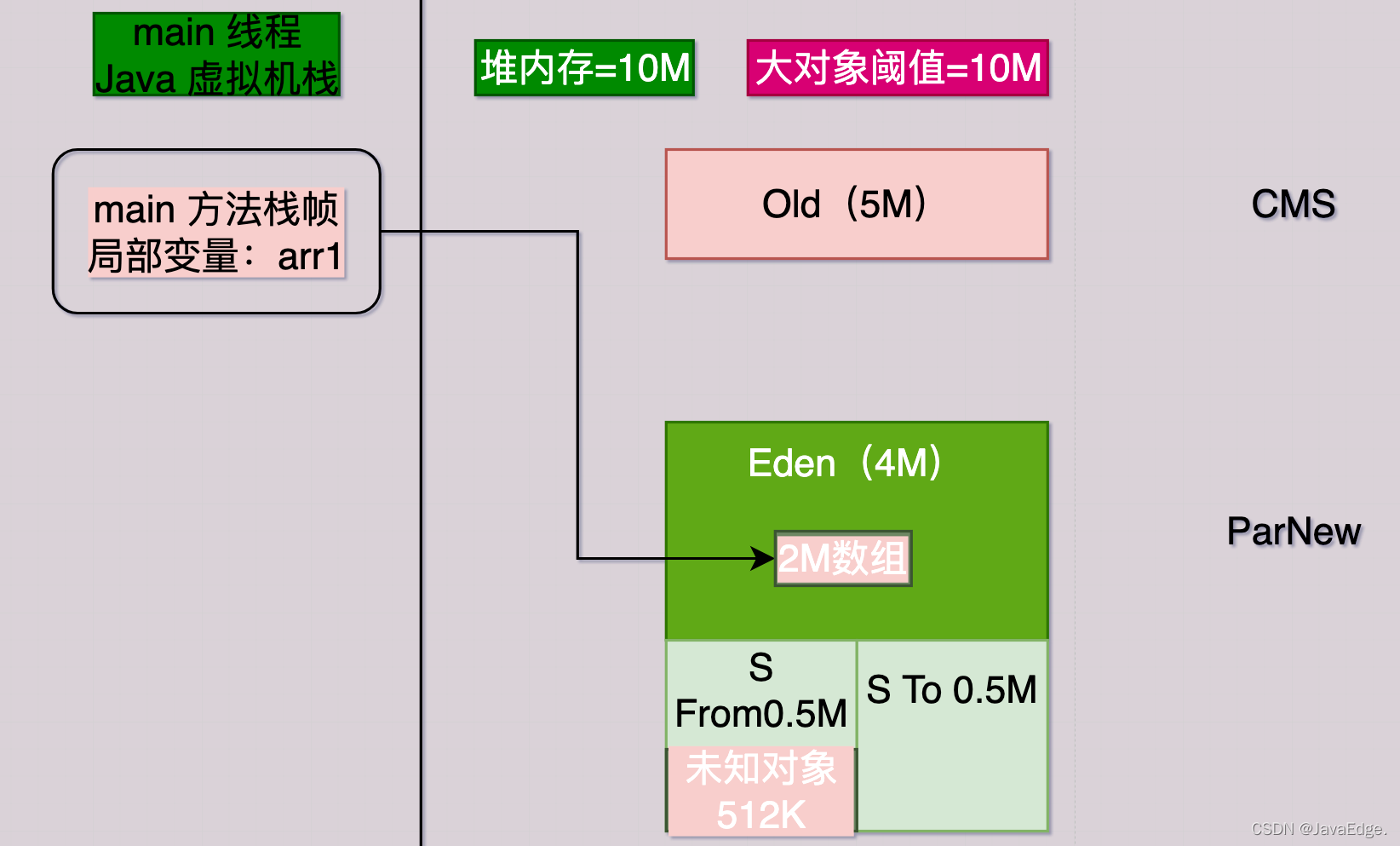
Eden log
Eden now uses 52% of 4M memory because of the 2M array. Then, in the From Survivor area, 512K is 100% utilization, which is occupied by 512K unknown objects that survived after the previous GC.
CMS, Metaspace metadata space and Class space
Concurrent mark sweep garbage collector, that is, the old memory space managed by CMS is 5M in total, and 62K is used at this time. Metaspace metadata space and Class space store some Class information, constant pool and other things. At this time, their total capacity and memory use.