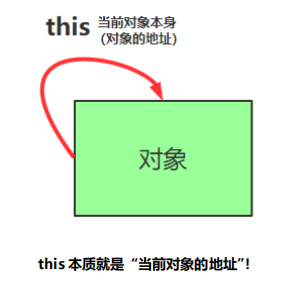
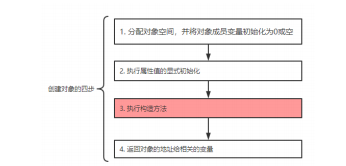
public class TestThis { int a, b, c; TestThis() { System.out.println("About to initialize a Hello object"); } TestThis(int a, int b) { // TestThis(); // In this way, the constructor cannot be called! this(); // Call the parameterless constructor and must be on the first line! a = a;// This refers to local variables rather than member variables // This distinguishes between member variables and local variables This situation accounts for the majority of this usage! this.a = a; this.b = b; } TestThis(int a, int b, int c) { this(a, b); // Call the constructor with parameters and must be on the first line! this.c = c; } void sing() { } void eat() { this.sing(); // Call sing() in this class; System.out.println("Your mother called you home for dinner!"); } public static void main(String[ ] args) { TestThis hi = new TestThis(2, 3); hi.eat(); } }
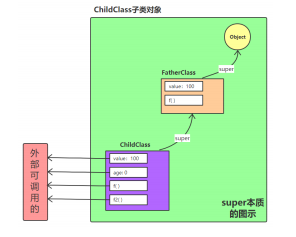
public class TestSuper01 { public static void main(String[ ] args) { new ChildClass().f(); } } class FatherClass { public int value; public void f(){ value = 100; System.out.println ("FatherClass.value="+value); } } class ChildClass extends FatherClass { public int value; public int age; public void f() { super.f(); //Call the normal method of the parent class value = 200; System.out.println("ChildClass.value="+value); System.out.println(value); System.out.println(super.value); //Call the member variable of the parent class } public void f2() { System.out.println(age); } }
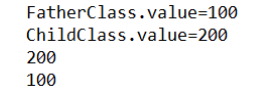
The difference between the two
1. Attribute differences:
This accesses the attribute in this class. If this class does not have this attribute, continue to find it from the parent class. super accesses properties in the parent class.
2. Differences in methods:
This accesses the method in this class. If this class does not have this method, continue to find it from the parent class. super accesses methods in the parent class.
3. Structural differences:
This call to this class construction must be placed in the first line of the construction method. When super calls the parent class construction, it must be placed in the first line of the child class construction method.
4. Other differences:
this represents the current object. super cannot represent the current object
A,this. Variables and super variable
this. The variable of the current object called by the variable;
super. Variables directly call variables in the parent class.
B. This and super methods
This (parameter) calls (forwards) the constructor in the current class;
Super (parameter) is used to confirm which constructor in the parent class to use.
Note:
1) When initializing a subclass with a parent class, the constructor of the parent class will also be executed, which takes precedence over the constructor of the subclass; Because the first line in the constructor of each subclass has a default implicit statement super();
2) this() and super() can only be written on the first line of the constructor;
3) this() and super() cannot exist in the same constructor. First, both this () and super () must be written on the first line of the constructor; Second, this () statement calls another constructor of the current class, and there must be a constructor of the parent class in this other constructor. Using super () to call the constructor of the parent class again is equivalent to calling the constructor of the parent class twice, and the compiler will not pass;
4) This and super cannot be used for static modified variables, methods and code blocks; Because this and super refer to objects (instances).