Article catalog
- Gorit takes you to learn the whole stack - Advanced JavaScript application (the first play)
- 1, Array article
- 2, JS regular expression
- 2.1 introduction to regular expressions
- 2.2 two ways to create regular expressions
- 2.3 simple pattern matching
- 2.4 special character matching
- 2.4. 1 '\ \' matches escape character
- 2.4. 2 '^' ab initio matching
- 2.4. End of 3 '$' matching input
- 2.4. 4 '*' matches zero or more
- 2.4. 5 '+' matches one or more times
- 2.4. 6 ‘?' Match 0 or 1 times
- 2.4. 7 ‘.' Matches any character except line feed
- 2.4. 8 '|' either of the two options can be matched
- 2.4.9 '{x}' specifies the number of occurrences
- 2.5 how to use regular expressions
- 2.6 cases
- 3, Original link
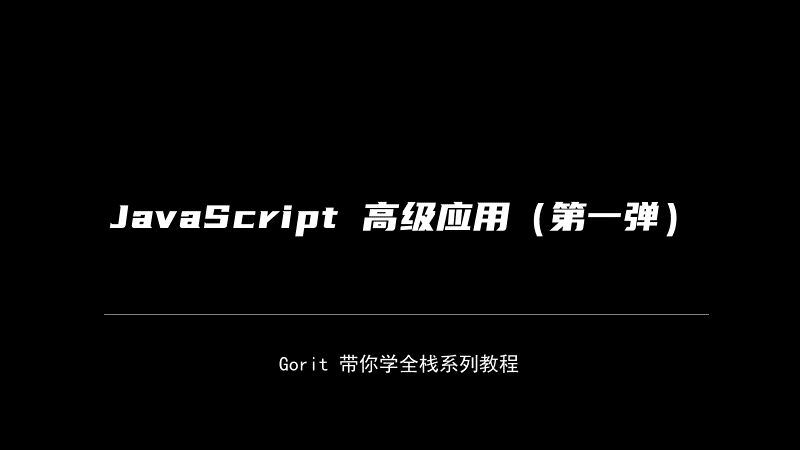
Author: Gorit
Date: December 5, 2021
Blog post published in 2021: 26 / 30
Gorit takes you to learn the whole stack - Advanced JavaScript application (the first play)
1, Array article
Objective: to explain the API s that are not common in development but are easy to use
1.1 expansion expression
Expand each item of the array and return a new array
let arr = [1,2,3]; console.log(...arr); // [1, 2 ,3] // Used to copy arrays let res = [...arr]; res.push(4); res // [1,2,3,4] arr // [1,2,3]
1.2 return a new array
In addition to the numeric for loop and forEach loop, what other useful loop processing schemes are available
1.2.1 map
Use map to perform a specific operation on each element, and then return the new array after the operation
eg:
let arr = [1, 2, 3]; let res = arr.map(item => item + 1); res // [2,3,4]
1.2.2 filter
Filter out the qualified elements according to the conditions and return a new array
eg:
let arr [1,2,3,4,5,6]; // Gets the even number in the array let res = arr.filter(item => item % 2 === 0); res // [2,4,6]
1.2.3 concat
Merge two or more arrays without changing the original array
concat() concat(value0) concat(value0, value1) concat(value0, value1, ... , valueN)
const array1 = ['a', 'b', 'c']; const array2 = ['d', 'e', 'f']; const array3 = array1.concat(array2); console.log(array3); // expected output: Array ["a", "b", "c", "d", "e", "f"]
practical application
Define a number locally, then load a data from other places, and merge the two data after processing
1.3 index related issues
1.4 return boolean value
1.5 return a final result
reduce
This built-in function is special. It processes each value through a callback. Each processing will save the results of the last processing and continue processing with the next element
Realize the continuous operation of a number
Implement an accumulator
let res = 0; for (const item of [1,2,3,4,5]) { res+=item; } console.log(res);
Use reduce to achieve the same function
let arr = [1,2,3,4,5]; // Implement an accumulator const reducer = (preVal, curVal) => preVal + curVal; console.log(arr.reduce(reducer)); /** * preVal + curVal (curVal) It starts from the array subscript (preVal) and starts from the result of the last processing For the first execution, since there is no initial value assigned, preVal starts from 0 0 + 1 => 1 The second time, continue to use the results of the first time 1 + 2 = 3 3 + 3 = 6; 6 + 4 = 10; 10 + 5 = 15; **/ console.log(arr.reduce(reducer, 6)); /** * There is an initial value * 6 + 1 = 7 * 7 + 2 = 9 * 9 + 3 = 12 * 12 + 4 = 16 * 16 + 5 = 21 **/
1.6 references
2, JS regular expression
Regular expressions are often used to deal with "String Problems", and many programming languages basically support regular expressions.
In order to ensure the learning difficulty and improve it in turn, I reorganized the content of MDN and explained it with clear examples
2.1 introduction to regular expressions
2.2 two ways to create regular expressions
// Create a regular expression let re = /ab + c/; // Mode 1: this mode has better performance let reg = new RegExp("ab + c"); // Method 2: xxx let res = reg.test("ab + c"); console.log(res); // true
PS: use spaces carefully in regular expressions. Spaces also represent a match
Where else do you use regular expressions?
A: regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used for RegExp's exec and test methods, and String's match, matchAll, replace, search, and split methods.
2.3 simple pattern matching
We will match whatever character we specify
let word = /abc/; // The string contains' abc '. Yes,' abc ', No
2.4 special character matching
2.4. 1 '\' matches escape character
Function: the backslash before a special character indicates that the next character is not a special character
Escape character | meaning | equivalence | Example |
---|---|---|---|
\d | Match any number between 0 and 9 | [0-9] | /\d/.test("a12 ") => 1 |
\D | Match a non numeric character | [^0-9] | |
\f | Match a page feed (U+000C) | ||
\n | Match a newline character (U+000A) | ||
\r | Match a carriage return (U+000D) | ||
\s | Matches a blank character, including spaces, tabs, page breaks, and line breaks \s does not match "\ u180e" | Hardly | |
\S | Match a non white space character | /S\w*/.test("foo bar") => foor | |
\t | Match a horizontal tab (U+0009) | ||
\v | Match a vertical tab (U+000B) | ||
\w | With a single word character (letter, number or underscore). Equivalent to [A-Za-z0-9_] | /\w/.test("banana") => 'b' /\w/.test("$3.28") => 3 | |
\W | Matches a non single word character. Equivalent to [^ A-Za-z0-9_] | /\W/.test("50%") => % | |
\n (less) | In a regular expression, it returns the matching substring of the last nth substring (the number of captures is counted in left parentheses). | ||
\0 | Match the NULL (U+0000) character, do not follow it with other decimals, because \ 0 is an octal escape sequence. | ||
[external link picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-lMyLMp1b-1639491830407)(D: \ technical notes \ img\image-20211207234934005.png)]
2.4. 2 '^' ab initio matching
Function: match the beginning of input. If the multiline flag is set to true, the position immediately following the newline character is also matched. (it doesn't make much sense to speak alone)
/** * Match from A */ let eg = /^A/; console.log(`an A is not match`.search(eg)); // -1 console.log(`An apple is matched`.search(eg)); // 0
2.4. End of 3 '$' matching input
Function: match the end of input. If the multiline flag is set to true, the position before the newline character is also matched. (ibid.)
/** * Match from t to end */ let eg1 = /t$/; console.log("eat is not matched".search(eg1)); // -1 console.log("matched is eat".search((eg1))); // 13 last string subscript
2.4. 4 '*' matches zero or more
Action: matches the previous expression 0 or more times. Equivalent to {0,}.
let eg1 = /ab*c/; console.log(eg1.test("ac", eg1.test("abbc"))); // true true
2.4. 5 '+' matches one or more times
Function: match the previous expression 1 or more times. Equivalent to {1,}.
let eg2 = /ab+c/; console.log("/ab+c/:" ,eg2.test("ac"), eg2.test("abc"), eg2.test("abbc")); // false true true
2.4. 6 ‘?’ Match 0 or 1 times
Function: matches the previous expression 0 or 1 times. Equivalent to {0,1}
let eg3 = /a?bc?/ console.log("/a?bc?: ", eg3.test("abc"), eg3.test("abb"), eg3.test("bcc")); // true true true
2.4. 7 ‘.’ Matches any character except line feed
Function: (decimal point) matches any single character except line feed by default.
let eg4 = /.n/; console.log(eg4.test('na , an apple on the tree')); // true (na will not be matched, an, on will be matched
2.4. 8 '|' either of the two options can be matched
Function: x | y, match 'x' or 'y'.
/** * One of them can hit */ let eg2 = /green|yellow/; console.log(eg2.exec("green apple is matched")); // ['green',index: 0,input: 'green apple is matched',groups: undefined] console.log(eg2.exec("red apple is not matched")); // null
2.4.9 '{x}' specifies the number of occurrences
Function: {n}, n is a positive integer that matches the previous character exactly n times.
/** * Specify the number of occurrences (2) */ let eg3 = /a{2}/; console.log("candy is not matched".search(eg3)); // -1, less than 2 times, unable to match console.log("caaandy".search(eg3)); // Output subscript 1 (matches the first occurrence)
2.5 how to use regular expressions
How to use regular expressions
Regular expressions can be used for RegExp exec and test (en-US) Methods and String of match (en-US),replace,search (en-US) and split (en-US) method. These methods are JavaScript manual It is explained in detail in.
2.5. 1. Methods used in regular expression matching
[the external chain picture transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-ZZt5u2zH-1639491830411)(D: \ technical notes \ img\image-20211208235800398.png)]
2.5. 2 Advanced Search
Regular expressions have six optional parameters (flags) that allow global and case insensitive searches, etc. These parameters can be used alone or together in any order, and are included in regular expression instances.
// grammar let re = /pattern/flags; let re = new RegExp("pattern", "flags");
Think of a topic and use regular expressions to match each word item
let reg = /\w+/g; let word = "an apple a day, keep the doctor away"; // Use regular expressions to match each word console.log(word.match(reg)); // Matching will also have day, [disadvantages] console.log(word.split(" "));
2.6 cases
PS: it may be difficult to write by yourself, but the impression is enough to review it in time
2.6. 1 check 11 digit mobile phone number
Without considering special situations, it can meet most scenarios
let reg = /\d{11}/; // This is not the easiest to think of, but the real mobile phone number is much more complex than this let reg1 = /^(13[0-9]|14[5|7]|15[0|1|2|3|5|6|7|8|9]|18[0|1|2|3|5|6|7|8|9])\d{8}$/;
2.6. 2 check email address
let reg = /^\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$/;
2.6. 3 verify domain name
What's up? Take my personal website as an example https://www.gorit.cn
- https / http is the domain name protocol of a website. SSL is enabled for those with's'. It is understood that the website is secure
- Www.spirit.com Cn is our domain name, spirit Cn is the root domain name, but generally speaking, www.spirit Cn and spirit Cn can visit my personal website. Like blog gorit.cn, prefixed domain names belong to secondary domain names, and so on
let reg = /http|https:\/\/[a-zA-Z0-9][-a-zA-Z0-9]{0,62}(/.[a-zA-Z0-9][-a-zA-Z0-9]{0,62})+/.?/;