Experiment 2 basic application of Struts -- user login module based on Struts 2 Framework
1, Basic experiment -- Struts2 framework construction
(1) Experimental purpose
- Master the basic development steps and general configuration of struts 2 application;
- Observe the assignment relationship between form parameters and Action attribute, observe the execute() method of Action and its return value, and can be applied correctly;
- Observe the main elements and attributes in the configuration file struts.xml and apply them correctly;
- Understand the embodiment of MVC design pattern in Stuts2 framework, understand the main functions of Action, FilterDispatcher and struts.xml, and be able to apply them correctly.
(2) Basic knowledge and principles
-
Struts 2 is an MVC framework developed from WebWork framework;
-
FilterDispatcher is the core controller in struts 2. Requests from clients to servers will be filtered by FilterDispatcher; If the request needs to call an Action, the framework will find the Action class to be called according to the configuration file struts.xml;
-
Action class is a Java se class that conforms to certain naming standards and is used as a business controller; The execute() method in the action is used to call the business logic class of the Model layer and determine the page navigation according to the returned results;
-
If the request parameters submitted by the form need to be used in the Action class, the variable corresponding to the name of the form field must be declared in the Action class, and the getters/setters method must be provided for the variable;
-
The Action class can only be used after it is configured in struts.xml;
-
To compile and run the Web project based on struts 2 framework, you need to import 8 core jar packages of struts 2:
file name explain struts2-core-2.3.15.1.jar The core class library of Struts 2 Framework xwork-core-2.3.15.1.jar XWork class library, the foundation of Struts 2 Ognl-3.0.6.jar An expression language class library used by Struts 2 freemarker-2.3.19.jar The tag template of Struts 2 uses the class library javassist-3.11.0.GA.jar Code generation Toolkit commons-lang3-3.1.jar Apache language pack is an extension of java.lang package commons-io-2.0.1.jar Apache IO package commons-fileupload-1.3.jar Struts 2 file upload dependency package
(3) Experiment contents and steps
-
Sign in http://struts.apache.org/download.cgi Website, download the latest version of struts 2 (Full Distribution);
-
New project in IDEA
-
Add struts 2 related dependencies or import jar packages
<dependency> <groupId>org.apache.struts</groupId> <artifactId>struts2-core</artifactId> <version>2.3.37</version> </dependency>
-
Create a new login.jsp page as the view of user login.
<form method="post" action="login"> <table> <tr> <td>user name:</td> <td> <input name="loginUser.account" type="text"> </td> </tr> <tr> <td>password:</td> <td><input type="password" name="loginUser.password"></td> </tr> </table> <input type="submit" value="Submit" name="Submit"> </form>
-
Create loginSuccess.jsp and loginFail.jsp pages as views of login success or login failure respectively.
-
Create a new bean package and create UserBean.java to record the login user information:
package com.example.struts_prij1.bean; public class UserBean { private String account; private String password; public UserBean() { } public UserBean(String account, String password) { this.account = account; this.password = password; } public String getAccount() { return account; } public void setAccount(String account) { this.account = account; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
-
Create a new service package and create UserService.java to implement login logic
package com.example.struts_prij1.service; import com.example.struts_prij1.bean.UserBean; public class UserService { public boolean login(UserBean loginUser){ if(loginUser.getAccount().equals(loginUser.getPassword())){ return true; } return false; } }
-
Create the action package, create UserAction.java, call the login logic, and return different contents according to different login results
package com.example.struts_prij1.action; import com.example.struts_prij1.bean.UserBean; import com.example.struts_prij1.service.UserService; public class UserAction { private UserBean loginUser; public UserBean getLoginUser(){ return loginUser; } public void setLoginUser(UserBean loginUser){ this.loginUser = loginUser; } public String execute(){ UserService userService = new UserService(); if(userService.login(loginUser)){ return "success"; } return "fail"; } }
-
Create struts.xml file in src directory to configure Action and set page navigation
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="strutsBean" extends="struts-default"> <action name="login" class="com.example.action.UserAction"> <result name="success">/loginSuccess.jsp</result> <result name="fail">/loginFail.jsp</result> </action> </package> </struts>
-
Edit the web.xml file of the Web application and add the struts 2 core Filter configuration
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.FilterDispatcher</filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
-
Deploy to Tomcat server
-
Modify the login.jsp code
<%-- Created by IntelliJ IDEA. User: YIYI Date: 2021/9/27 Time: 10:22 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="s" uri="/struts-tags"%> <html> <head> <title>Sign in</title> </head> <body> <s:form method="post" action="login.action"> <s:textfield name="loginUser.account" label="Please enter your account number"/> <s:password name="loginUser.password" label="Please enter your password"/> <s:submit value="Sign in"/> </s:form> </body> </html>
-
Operation results
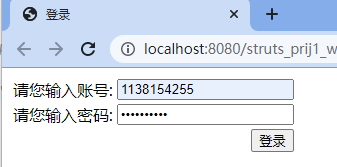 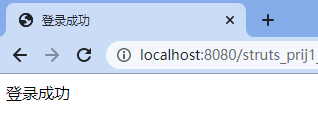
(4) Experimental summary
-
Before modifying the code, the code of login.jsp is obviously incorrect. You need to change the html tag to the struts 2 tag
<%@ taglib prefix="s" uri="/struts-tags"%>
-
Due to the great changes in struts 2.3 and 2.5, version 2.5 was installed at the beginning, and the struts.xml file was configured incorrectly, resulting in 404 errors;
-
Assignment relationship of Action attribute:
The value of the name attribute in jsp corresponds to the name of the data object in the Action class. If the data member of the data object is an object, the following format is used. Example: loginUser.name.first
The return value of the execute method in the Action class is a string, and the mapping is configured for the return value in struts.xml;
-
According to the container configuration, the server transfers the request to the FilterDispatcher for processing and enters the struts 2 process;
-
The struts framework will find the corresponding action class in the struts.xml configuration file, initialize the action, execute the set method, and then execute the execute method;
-
The framework matches the string returned by the execute method with the configuration file information, and then returns the result page.
-
Action has good code reusability and does not need to be coupled with Servlet API. At the same time, the returned string is used as the processing result, making the result processing more flexible.
<struts> <!-- <package> Element is used for package configuration Struts2 In the framework, packages are used to organize Action And interceptors, each packet is composed of zero or more interceptors and Action A collection of.--> <!-- name:Required attribute,Specifies the name of the package,This name is referenced by other packages Key--> <!-- namespace:optional attribute ,Namespace used to define the package--> <!-- extends:optional attribute ,Specifies that the package inherits from other packages,Its property value must be the value of another package name Attribute value,However, the property value is usually set to struts-default,In this way, the Action You have Struts Framework default interceptor and other functions--> <package name="strutsBean" extends="struts-default"> <!-- action The configuration specifies which needs to be initialized action Class--> <!-- result The processing result of the corresponding string is specified--> <action name="login" class="com.example.action.UserAction"> <result name="success">/loginSuccess.jsp</result> <result name="fail">/loginFail.jsp</result> </action> </package> </struts>
2, Improvement experiment -- struts 2 tag
(1) Experimental purpose
- Further familiar with the basic development steps and general configuration of struts 2 application;
- Further familiar with the application methods of Action and configuration file struts.xml;
- Master the basic usage of struts 2 tag;
- Be able to skillfully use the commonly used tags of struts 2, refer to the instruction documents of struts 2 Tags, and flexibly apply all kinds of tags;
(2) Basic knowledge and principles
- Using struts 2 Tags to express page logic can avoid using Java code in the view as much as possible, separate the logic from the display, and improve the maintainability of the view;
- The main TLD file of the struts 2 tag library is struts-tags.tld. In the struts 2-core-2.3.15.1.jar package, another Ajax related tag library TLD file is struts-dojo-tags.tld, which is in the struts 2-dojo-plugin-2.3.15.1.jar package;
- The steps for using the struts 2 tag are the same as using JSTL. You only need to use the taglib instruction in the JSP page to introduce the uri of the tld file in the tag library and specify the prefix, for example: <% @ taglib prefix = "s" uri = "/ struts tags"% >;
- According to the main functions of struts 2 Tags, they can be divided into UI tags for generating page elements, control class tags for realizing process control, data tags for controlling data and tags for supporting Ajax.
(3) Experiment contents and steps
-
Import the struts2-dojo-plugin-2.3.37.jar package into the lib directory and add dependencies
-
Create a new register.jsp page as the view of user registration. The page uses the UI tag of struts 2 to generate form elements, including user name, password, confirmation password, real name, gender, birthday, contact address, contact phone and e-mail:
<%-- Created by IntelliJ IDEA. User: yiyi Date: 2021/9/27 Time: 14:07 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="s" uri="/struts-tags"%> <%@ taglib prefix="sx" uri="/struts-dojo-tags"%> <html> <head> <s:head theme="xhtml"/> <sx:head parseContent="true" extraLocales="UTF-8"/> </head> <body> <s:form action="register.action" method="post" οnsubmit="return check()"> <s:textfield name="loginUser.account" label="enter one user name"/> <s:password name="loginUser.password" label="Please input a password"/> <s:password name="loginUser.repassword" label="Please enter the password again"/> <s:radio name="loginUser.sex" list="#{1: 'male', 0: 'female'} "label =" please enter gender "/ > <sx:datetimepicker name="loginUser.birthday" displayFormat="yyyy-MM-dd" label="Please enter birthday"/> <s:textfield name="loginUser.address" label="Please enter the contact address"/> <s:textfield name="loginUser.phone" label="Please enter your mobile phone number"/> <s:textfield name="loginUser.email" label="Please enter email address"/> <s:submit value="register"/> <s:reset value="Reset"/> </s:form> </body> </html>
-
Create a new regFail.jsp page to display "registration failed" as an attempt to register failed
-
Create a new regSuccess.jsp page. As a successful registration attempt, use the data tag and control tag of struts 2 to generate successful registration information, and save the login user information in the session scope.
<%-- Created by IntelliJ IDEA. User: yiyi Date: 2021/9/27 Time: 14:37 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="s" uri="/struts-tags" %> <html> <head> <title>login was successful</title> </head> <body> <s:property value="loginUser.name"/> <s:if test="%{loginUser.sex==\"1\"}"> <s:text name="sir, "/> </s:if> <s:else> <s:text name="ma'am, "/> </s:else> You have registered successfully! <s:set name="user" value="loginUser" scope="session"/> </body> </html>
-
Modify UserBean class
package com.example.bean; public class UserBean { private String account=""; private String password=""; private String repassword=""; private String name=""; private String sex=""; private String birthday=""; private String address=""; private String phone=""; private String email=""; public String getAccount() { return account; } public void setAccount(String account) { this.account = account; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getRepassword() { return repassword; } public void setRepassword(String repassword) { this.repassword = repassword; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public String getBirthday() { return birthday; } public void setBirthday(String birthday) { this.birthday = birthday; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
-
Modify UserService.java and add user registration logic. In order to simplify the registration logic, the conditions for successful registration are set as follows: the user name, password and confirmation password are the same, and they are not empty strings;
public boolean register(UserBean registerUser){ if(registerUser.getAccount().equals(registerUser.getPassword()) && registerUser.getPassword().equals(registerUser.getRepassword()) && !registerUser.getAccount().equals("")){ return true; } return false; }
-
Modify the execute() method in UserAction.java to call the registration logic and return different contents according to the registration results
public String execute(){ UserService userService = new UserService(); if(userService.register(loginUser)){ return "success"; } return "fail"; }
-
Modify struts.xml file, configure user registration and set page navigation
<action name="register" class="com.example.action.UserAction"> <result name="success">/regSuccess.jsp</result> <result name="fail">/regFail.jsp</result> </action>
-
Redeploy to Tomcat server and run.
(4) Experimental summary
There is no BUG in this experiment, but modifying the original execute method is not a reasonable operation. You should create another Action to handle the registration business instead of picking up sesame seeds and throwing watermelon.
3, Expanding experiment -- internationalization of struts 2
(1) Experimental purpose
- Further familiar with the basic usage of struts 2 tag;
- Can use struts 2 tag to realize internationalization;
- Understand the role and basic usage of the configuration file struts.properties and the internationalization resource file.
(2) Basic knowledge and principles
- By saving the characters of different language versions in the attribute file, the internationalization mechanism of struts 2 can realize the application of different language versions without modifying the program body;
- Select the content to be internationalized in the Web application, and instead of directly outputting the information in the page, output a key value through the struts 2 tag, which corresponds to different strings in different language environments; For example,: < s: textfield name = "loginUser.account" label = "please enter user name" / > the "please enter user name" in the code is the content that needs to be internationalized. Replace it with a key value as < s: textfield name = "loginUser.account" key = "login. Account. Label" / >;
- The contents to be internationalized are written into the internationalization resource file of struts 2 in the form of key value pair (key=value), such as "login. Account. Label = please enter user name"; The resource file name can be customized, but the suffix must be properties; The resource file should be placed in the class loading path of the Web application; Each language version needs to create a resource file;
- Configure the basic name of the resource file through the struts.properties configuration file of struts 2. If the basic name of the resource file is message, then message_zh_CN.properties is the corresponding Chinese resource file, message_en_US.properties is the corresponding American English resource file;
- The browser will automatically call the resource file of the corresponding language according to its default language version, so as to display different language effects on the page.
(3) Experiment contents and steps
-
Create a cn.edu.zjut.local package in the src directory and place all resource files in it, such as message_zh_CN.properties,message_en_US.properties, etc;
#message_en_US.properties login.account.lable=Please input your account login.password.lable=Please input your password login.submit.button=submit
#message_zh_CN.properties login.account.lable=enter one user name login.password.lable=Please input a password login.submit.button=Sign in
-
Re encode the resource files to avoid garbled code;
#message_zh_CN.properties login.account.lable=enter one user name login.password.lable=Please input a password login.submit.button=Sign in
-
Create the struts.properties file in the src directory and load the resource file through it:
struts.custom.i18n.resources=cn.edu.zjut.local.message struts.i18n.encoding=GBK
-
Modify login.jsp, loginsuccess.jsp and loginFail.jsp pages to realize internationalization through struts 2 tag;
<!--login.jsp--> <s:textfield name="loginUser.account" key="login.account.lable"/> <s:password name="loginUser.password" key="login.password.lable"/> <s:submit name="submit" key="login.submit.button"/>
-
Redeploy to Tomcat server
-
Set the browser's preferred language option, visit the login.jsp page, observe and record the running results;
Visit in Chinese
Change browser language to English
Visit in English
-
Modify user registration module
#message_en_US.properties login.account.lable=Please input your account login.password.lable=Please input your password login.submit.button=submit register.account.lable=Please input your account register.password.lable=Please input your password register.repassword.lable=Please input your password again register.sex.lable=Please input your sex register.birthday.lable=Please input your birthday register.address.lable=Please input your address register.phone.lable=Please input your phone register.email.lable=Please input your email register.submit.button=Sign up register.reset.button=Reset
#message_zh_CN.properties login.account.lable=enter one user name login.password.lable=Please input a password login.submit.button=Sign in register.account.lable=enter one user name register.password.lable=Please input a password register.repassword.lable=Please enter the password again register.sex.lable=Please enter gender register.birthday.lable=Please enter birthday register.address.lable=Please enter the contact address register.phone.lable=Please enter your mobile phone number register.email.lable=Please enter email address register.submit.button=register register.reset.button=Reset
Create UserRegisterAction.java class to handle registration business
package com.example.action; import com.example.bean.UserBean; import com.example.service.UserService; public class UserRegisterAction { private UserBean loginUser; public UserBean getLoginUser(){ return loginUser; } public void setLoginUser(UserBean loginUser){ this.loginUser = loginUser; } public String execute(){ UserService userService = new UserService(); if(userService.register(loginUser)){ return "success"; } return "fail"; } }
Modify struts2.xml configuration
<struts> <!-- <package> Element is used for package configuration Struts2 In the framework, packages are used to organize Action And interceptors, each packet is composed of zero or more interceptors and Action A collection of.--> <!-- name:Required attribute,Specifies the name of the package,This name is referenced by other packages Key--> <!-- namespace:optional attribute ,Namespace used to define the package--> <!-- extends:optional attribute ,Specifies that the package inherits from other packages,Its property value must be the value of another package name Attribute value,However, the property value is usually set to struts-default,In this way, the Action You have Struts Framework default interceptor and other functions--> <package name="strutsBean" extends="struts-default"> <!-- action The configuration specifies which needs to be initialized action Class--> <!-- result The processing result of the corresponding string is specified--> <action name="login" class="com.example.action.UserAction"> <result name="success">/loginSuccess.jsp</result> <result name="fail">/loginFail.jsp</result> </action> <action name="register" class="com.example.action.UserRegisterAction"> <result name="success">/regSuccess.jsp</result> <result name="fail">/regFail.jsp</result> </action> </package> </struts>
Operation results
(4) Experimental summary
Internationalization through struts 2 tag can make a website add a properties resource file to complete pages in different languages, and there is no coupling between languages, so it is very convenient to modify.
Through this experiment, I am familiar with the way of internationalization of struts 2 and have a further understanding of struts 2 framework.