1, Java's own thread pool:
1.newCachedThreadPool 2.newFixedThreadPool 3.newSingleThreadExecutor
2, Look at an example
public class ThreadPoolDemo { public static void main(String[] args){ ExecutorService newCachedService1 = Executors.newCachedThreadPool(); ExecutorService newFixedService2 = Executors.newFixedThreadPool(10); ExecutorService newSingleService3 = Executors.newSingleThreadExecutor(); for (int i =1;i<=100;i++){ newCachedService1.execute(new MyTask(i)); } } static class MyTask implements Runnable { int i =0; public MyTask(int i) { this.i = i; } @Override public void run() { System.out.println(Thread.currentThread().getName()+"Programmers do the second"+i+"Items"); //Business logic try { Thread.sleep(3000L); } catch (InterruptedException e) { e.printStackTrace(); } } } }
1.newCachedService1.execute run result
pool-1-thread-1 Programmers do the first project pool-1-thread-2 The programmer does the second project pool-1-thread-3 The programmer does the third project pool-1-thread-4 Programmers do the fourth project pool-1-thread-5 The programmer does the fifth project pool-1-thread-6 The programmer does the sixth project pool-1-thread-7 Programmers do the seventh project pool-1-thread-8 Programmers do the eighth project pool-1-thread-9 Programmers do the ninth project pool-1-thread-10 Programmer to do the 10th project pool-1-thread-11 Programmers do the 11th project pool-1-thread-12 Programmers do the 12th project pool-1-thread-13 Programmers do the 13th project pool-1-thread-14 The programmer does the 14th project pool-1-thread-15 Programmers do the 15th project pool-1-thread-17 Programmers do the 17th project pool-1-thread-16 Programmers do the 16th project pool-1-thread-18 Programmers do the 18th project pool-1-thread-19 Programmers do the 19th project pool-1-thread-20 Programmers do the 20th project pool-1-thread-21 Programmers do the 21st project pool-1-thread-22 Programmers do the 22nd project pool-1-thread-24 Programmers do the 24th project pool-1-thread-23 Programmers do the 23rd project pool-1-thread-25 Programmers do the 25th project pool-1-thread-26 Programmers do the 26th project pool-1-thread-27 Programmers do the 27th project pool-1-thread-28 Programmers do the 28th project pool-1-thread-29 Programmers do the 29th project pool-1-thread-30 Programmers do the 30th project pool-1-thread-31 The programmer does the 31st project pool-1-thread-32 The programmer does the 32nd project pool-1-thread-33 The programmer does the 33rd project pool-1-thread-34 Programmers do the 34th project pool-1-thread-35 Programmers do the 35th project pool-1-thread-36 The programmer does the 36th project pool-1-thread-37 Programmers do the 37th project pool-1-thread-38 Programmers do the 38th project pool-1-thread-39 Programmers do the 39th project pool-1-thread-40 Programmers do the 40th project pool-1-thread-41 Programmers do the 41st project pool-1-thread-42 The programmer does the 42nd project pool-1-thread-43 The programmer does the 43rd project pool-1-thread-44 Programmers do the 44th project pool-1-thread-45 Programmers do the 45th project pool-1-thread-46 The programmer does the 46th project pool-1-thread-48 The programmer does the 48th project pool-1-thread-47 Programmers do the 47th project pool-1-thread-49 Programmers do the 49th project pool-1-thread-50 Programmers do the 50th project pool-1-thread-51 The programmer did the 51st project pool-1-thread-52 The programmer does the 52nd project pool-1-thread-53 The programmer does the 53rd project pool-1-thread-54 The programmer did the 54th project pool-1-thread-55 Programmers do the 55th project pool-1-thread-56 The programmer does the 56th project pool-1-thread-57 The programmer did the 57th project pool-1-thread-58 The programmer does the 58th project pool-1-thread-59 The programmer does the 59th project pool-1-thread-60 Programmers do the 60th project pool-1-thread-61 Programmers do the 61st project pool-1-thread-62 The programmer does the 62nd project pool-1-thread-63 The programmer does the 63rd project pool-1-thread-64 Programmers do the 64th project pool-1-thread-65 The programmer does the 65th project pool-1-thread-67 Programmers do the 67th project pool-1-thread-66 The programmer did the 66th project pool-1-thread-68 Programmers do the 68th project pool-1-thread-69 Programmers do the 69th project pool-1-thread-70 Programmers do the 70th project pool-1-thread-71 Programmers do the 71st project pool-1-thread-72 Programmers do the 72nd project pool-1-thread-73 The programmer did the 73rd project pool-1-thread-74 Programmers do the 74th project pool-1-thread-75 The programmer does the 75th project pool-1-thread-76 Programmers do the 76th project pool-1-thread-77 Programmers do the 77th project pool-1-thread-78 The programmer did the 78th project pool-1-thread-79 Programmers do the 79th project pool-1-thread-80 Programmers do the 80th project pool-1-thread-81 The programmer did the 81st project pool-1-thread-82 Programmers do the 82nd project pool-1-thread-83 The programmer did the 83rd project pool-1-thread-84 Programmers do the 84th project pool-1-thread-85 Programmers do the 85th project pool-1-thread-86 The programmer does the 86th project pool-1-thread-87 The programmer did the 87th project pool-1-thread-88 Programmers do the 88th project pool-1-thread-89 The programmer does the 89th project pool-1-thread-90 Programmers do the 90th project pool-1-thread-91 Programmers do the 91st project pool-1-thread-92 The programmer did the 92nd project pool-1-thread-93 The programmer does the 93rd project pool-1-thread-94 Programmers do the 94th project pool-1-thread-95 Programmers do the 95th project pool-1-thread-96 Programmers do the 96th project pool-1-thread-97 Programmers do the 97th project pool-1-thread-98 The programmer did the 98th project pool-1-thread-99 Programmers do the 99th project pool-1-thread-100 Programmers do the 100th project
2.newFixedService2.execute execution result
pool-2-thread-2 The programmer does the second project pool-2-thread-1 Programmers do the first project pool-2-thread-3 The programmer does the third project pool-2-thread-4 Programmers do the fourth project pool-2-thread-5 The programmer does the fifth project pool-2-thread-6 The programmer does the sixth project pool-2-thread-7 Programmers do the seventh project pool-2-thread-8 Programmers do the eighth project pool-2-thread-9 Programmers do the ninth project pool-2-thread-10 Programmers do the 10th project pool-2-thread-3 Programmers do the 11th project pool-2-thread-7 Programmers do the 12th project pool-2-thread-9 Programmers do the 13th project pool-2-thread-10 The programmer does the 14th project pool-2-thread-2 Programmers do the 16th project pool-2-thread-8 Programmers do the 18th project pool-2-thread-5 Programmers do the 15th project pool-2-thread-4 Programmers do the 19th project pool-2-thread-6 Programmers do the 20th project pool-2-thread-1 Programmers do the 17th project pool-2-thread-10 Programmers do the 21st project pool-2-thread-8 Programmers do the 23rd project pool-2-thread-5 Programmers do the 29th project pool-2-thread-3 Programmers do the 25th project pool-2-thread-9 Programmers do the 22nd project pool-2-thread-1 Programmers do the 30th project pool-2-thread-4 Programmers do the 28th project pool-2-thread-2 Programmers do the 27th project pool-2-thread-7 Programmers do the 26th project pool-2-thread-6 Programmers do the 24th project pool-2-thread-8 The programmer does the 32nd project pool-2-thread-10 The programmer does the 31st project pool-2-thread-3 The programmer does the 33rd project pool-2-thread-5 Programmers do the 34th project pool-2-thread-9 Programmers do the 35th project pool-2-thread-1 The programmer does the 36th project pool-2-thread-4 Programmers do the 37th project pool-2-thread-2 Programmers do the 38th project pool-2-thread-7 Programmers do the 39th project pool-2-thread-6 Programmers do the 40th project pool-2-thread-10 The programmer does the 42nd project pool-2-thread-8 Programmers do the 41st project pool-2-thread-3 Programmer to do the 43rd project pool-2-thread-5 Programmers do the 44th project pool-2-thread-9 Programmers do the 45th project pool-2-thread-1 The programmer does the 46th project pool-2-thread-4 Programmers do the 47th project pool-2-thread-7 Programmers do the 49th project pool-2-thread-2 The programmer does the 48th project pool-2-thread-6 Programmers do the 50th project pool-2-thread-3 The programmer did the 51st project pool-2-thread-1 The programmer does the 58th project pool-2-thread-6 Programmers do the 60th project pool-2-thread-5 The programmer did the 57th project pool-2-thread-9 The programmer does the 56th project pool-2-thread-10 Programmers do the 55th project pool-2-thread-4 The programmer does the 53rd project pool-2-thread-2 The programmer did the 54th project pool-2-thread-8 The programmer does the 52nd project pool-2-thread-7 The programmer does the 59th project pool-2-thread-3 Programmers do the 61st project pool-2-thread-5 Programmers do the 67th project pool-2-thread-10 Programmers do the 69th project pool-2-thread-1 The programmer did the 68th project pool-2-thread-4 Programmers do the 70th project pool-2-thread-7 The programmer did the 66th project pool-2-thread-8 The programmer does the 65th project pool-2-thread-9 The programmer does the 63rd project pool-2-thread-6 The programmer does the 62nd project pool-2-thread-2 Programmers do the 64th project pool-2-thread-3 Programmers do the 71st project pool-2-thread-1 Programmers do the 77th project pool-2-thread-6 Programmers do the 80th project pool-2-thread-9 Programmers do the 79th project pool-2-thread-7 The programmer did the 78th project pool-2-thread-5 Programmers do the 72nd project pool-2-thread-10 The programmer did the 73rd project pool-2-thread-2 Programmers do the 75th project pool-2-thread-8 Programmers do the 74th project pool-2-thread-4 Programmers do the 76th project pool-2-thread-7 The programmer did the 81st project pool-2-thread-9 Programmers do the 84th project pool-2-thread-5 The programmer did the 83rd project pool-2-thread-8 The programmer does the 89th project pool-2-thread-3 Programmers do the 82nd project pool-2-thread-4 Programmers do the 90th project pool-2-thread-2 Programmers do the 88th project pool-2-thread-10 The programmer did the 87th project pool-2-thread-1 The programmer does the 86th project pool-2-thread-6 Programmer to do the 85th project pool-2-thread-9 The programmer did the 92nd project pool-2-thread-4 Programmers do the 94th project pool-2-thread-10 Programmers do the 95th project pool-2-thread-6 The programmer does the 93rd project pool-2-thread-2 Programmers do the 91st project pool-2-thread-7 Programmers do the 100th project pool-2-thread-5 Programmers do the 99th project pool-2-thread-3 The programmer did the 98th project pool-2-thread-8 Programmers do the 97th project pool-2-thread-1 Programmers do the 96th project
3.newSingleService3.execute execution result
pool-3-thread-1 Programmers do the first project pool-3-thread-1 The programmer does the second project pool-3-thread-1 The programmer does the third project pool-3-thread-1 Programmers do the fourth project pool-3-thread-1 The programmer does the fifth project pool-3-thread-1 The programmer does the sixth project pool-3-thread-1 Programmers do the seventh project pool-3-thread-1 Programmers do the eighth project pool-3-thread-1 Programmers do the ninth project pool-3-thread-1 Programmers do the 10th project pool-3-thread-1 Programmers do the 11th project pool-3-thread-1 Programmers do the 12th project ......
3, Analysis
1. newCachedThreadPool implements the source code at the bottom:
public static ExecutorService newCachedThreadPool() { return new ThreadPoolExecutor(0, Integer.MAX_VALUE, 60L, TimeUnit.SECONDS, new SynchronousQueue<Runnable>()); }
public ThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue) { this(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue, Executors.defaultThreadFactory(), defaultHandler); }
1.1 for example, thread pool is an outsourcing company
For example, if the thread pool is an outsourcing company, the Task is the project to be contracted by the company, and the worker is the working programmer
corePoolSize is the number of core employees
maximumPoolSize is the number of non core elements
workQueue is a queue. Different queues can store different numbers of temporary tasks
Because there are no programmers in the company, the company needs to recruit temporarily after contracting the project, so the tasks need to be put in the queue first and wait to be assigned to which programmer. At present, there are 100 tasks. Now one task can only be put in the queue. There are no core employees in newCachedThreadPool, so only temporary workers can be recruited, while SynchronousQueue can only store one task, If you don't take this task away, you can't take the second task, so the first programmer takes the first task, then you can have the second task, the second programmer can take the second task, and then you can come to the third task
As can be seen from the running results of the example, each project is assigned a programmer, and one person only works on one project
1.2 now if thread sleep(3000L); Comment out
Execution result:
pool-1-thread-2 The programmer does the second project pool-1-thread-3 The programmer does the third project pool-1-thread-1 Programmers do the first project pool-1-thread-4 Programmers do the fourth project pool-1-thread-1 The programmer does the fifth project pool-1-thread-4 Programmers do the eighth project pool-1-thread-3 The programmer does the sixth project pool-1-thread-1 Programmers do the seventh project pool-1-thread-2 Programmers do the ninth project pool-1-thread-1 Programmers do the 11th project pool-1-thread-3 Programmers do the 12th project pool-1-thread-5 Programmers do the 10th project pool-1-thread-4 Programmers do the 13th project pool-1-thread-6 The programmer does the 14th project pool-1-thread-4 Programmers do the 15th project pool-1-thread-5 Programmers do the 16th project pool-1-thread-3 Programmers do the 17th project pool-1-thread-1 Programmers do the 18th project pool-1-thread-6 Programmers do the 19th project pool-1-thread-2 Programmers do the 20th project pool-1-thread-7 Programmers do the 21st project pool-1-thread-2 Programmers do the 22nd project pool-1-thread-6 Programmers do the 23rd project pool-1-thread-1 Programmers do the 24th project pool-1-thread-5 Programmers do the 27th project pool-1-thread-2 Programmers do the 28th project pool-1-thread-4 Programmers do the 29th project pool-1-thread-5 The programmer does the 32nd project pool-1-thread-5 Programmers do the 35th project pool-1-thread-8 Programmers do the 30th project pool-1-thread-6 Programmers do the 34th project pool-1-thread-4 Programmers do the 38th project pool-1-thread-9 The programmer does the 36th project pool-1-thread-8 Programmers do the 41st project pool-1-thread-4 The programmer does the 42nd project pool-1-thread-10 Programmers do the 39th project pool-1-thread-9 Programmers do the 45th project pool-1-thread-4 Programmers do the 44th project pool-1-thread-8 The programmer does the 46th project pool-1-thread-11 The programmer does the 43rd project pool-1-thread-12 Programmers do the 47th project pool-1-thread-11 The programmer does the 52nd project pool-1-thread-9 The programmer does the 48th project pool-1-thread-8 The programmer did the 54th project pool-1-thread-13 The programmer did the 51st project pool-1-thread-8 The programmer does the 59th project pool-1-thread-15 The programmer does the 58th project pool-1-thread-13 The programmer does the 62nd project pool-1-thread-14 Programmers do the 55th project pool-1-thread-15 The programmer does the 65th project pool-1-thread-11 The programmer did the 57th project pool-1-thread-14 The programmer did the 68th project pool-1-thread-17 The programmer does the 63rd project pool-1-thread-15 Programmers do the 67th project pool-1-thread-19 Programmers do the 69th project pool-1-thread-16 Programmers do the 60th project pool-1-thread-14 Programmers do the 75th project pool-1-thread-15 Programmers do the 72nd project pool-1-thread-18 The programmer did the 66th project pool-1-thread-17 Programmers do the 74th project pool-1-thread-15 The programmer did the 81st project pool-1-thread-18 The programmer did the 83rd project pool-1-thread-17 Programmers do the 84th project pool-1-thread-22 Programmers do the 79th project pool-1-thread-3 Programmers do the 26th project pool-1-thread-14 Programmers do the 77th project pool-1-thread-21 Programmers do the 76th project pool-1-thread-16 The programmer did the 78th project pool-1-thread-26 Programmers do the 90th project pool-1-thread-16 Programmers do the 94th project pool-1-thread-27 The programmer does the 93rd project pool-1-thread-20 Programmers do the 71st project pool-1-thread-26 Programmers do the 97th project pool-1-thread-27 The programmer did the 98th project pool-1-thread-21 Programmers do the 95th project pool-1-thread-3 The programmer did the 92nd project pool-1-thread-14 Programmers do the 91st project pool-1-thread-18 The programmer does the 89th project pool-1-thread-7 Programmers do the 25th project pool-1-thread-22 Programmers do the 88th project pool-1-thread-17 The programmer did the 87th project pool-1-thread-25 The programmer does the 86th project pool-1-thread-5 Programmers do the 37th project pool-1-thread-1 The programmer does the 33rd project pool-1-thread-2 The programmer does the 31st project pool-1-thread-6 Programmers do the 40th project pool-1-thread-4 Programmers do the 49th project pool-1-thread-24 Programmers do the 82nd project pool-1-thread-10 Programmers do the 50th project pool-1-thread-15 Programmer to do the 85th project pool-1-thread-12 The programmer does the 53rd project pool-1-thread-9 The programmer does the 56th project pool-1-thread-8 Programmers do the 61st project pool-1-thread-13 Programmers do the 64th project pool-1-thread-11 Programmers do the 70th project pool-1-thread-23 Programmers do the 80th project pool-1-thread-19 The programmer did the 73rd project pool-1-thread-29 Programmers do the 100th project pool-1-thread-16 Programmers do the 99th project pool-1-thread-28 Programmers do the 96th project
Result analysis:
Taking thread 2 as an example, we can see that thread 2 has completed projects 2, 9, 20, 22, 28 and 31. Why?
Because of thread reuse!
For example: the first programmer has done the first project, the second programmer has done the second project, and now comes the third project. It happens that the first programmer has completed the first project, and the company finds that he is relatively idle, so it gives him the third project, namely thread reuse: a thread receives multiple tasks! In this way, the company has 100 projects, so there is no need to recruit another 100 programmers
So: what thread to use depends on the length of business time!
2.newFixedThreadPool and newSingleThreadPool
2.1 newFixedThreadPool:
public static ExecutorService newFixedThreadPool(int nThreads) { return new ThreadPoolExecutor(nThreads, nThreads, 0L, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<Runnable>()); }
There are 10 core employees and no temporary employees
When the task comes, it can be assigned to 10 core employees. When the 11th task comes, it can only be put in the queue
LinkedBlockingQueue is an unbounded queue:
Here are 90 tasks. After 10 employees finish, take 10. That's why the operation result is a group of 10
2.2 newSingleThreadPool:
public static ExecutorService newSingleThreadExecutor() { return new FinalizableDelegatedExecutorService (new ThreadPoolExecutor(1, 1, 0L, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<Runnable>())); }
There is only one core employee of newSingleThreadPool. He can only do all the work alone, and the other 99 are in the queue, In the previous example , the newSingleThreadPool executes very fast, because 100000 tasks are executed every 1 millisecond, but the second example is different. Each task takes 3 seconds to complete
Therefore, what thread pool to use depends on the execution time of the project!
4, Summary
A good memory is better than a bad pen, and it's better to know than to do it.
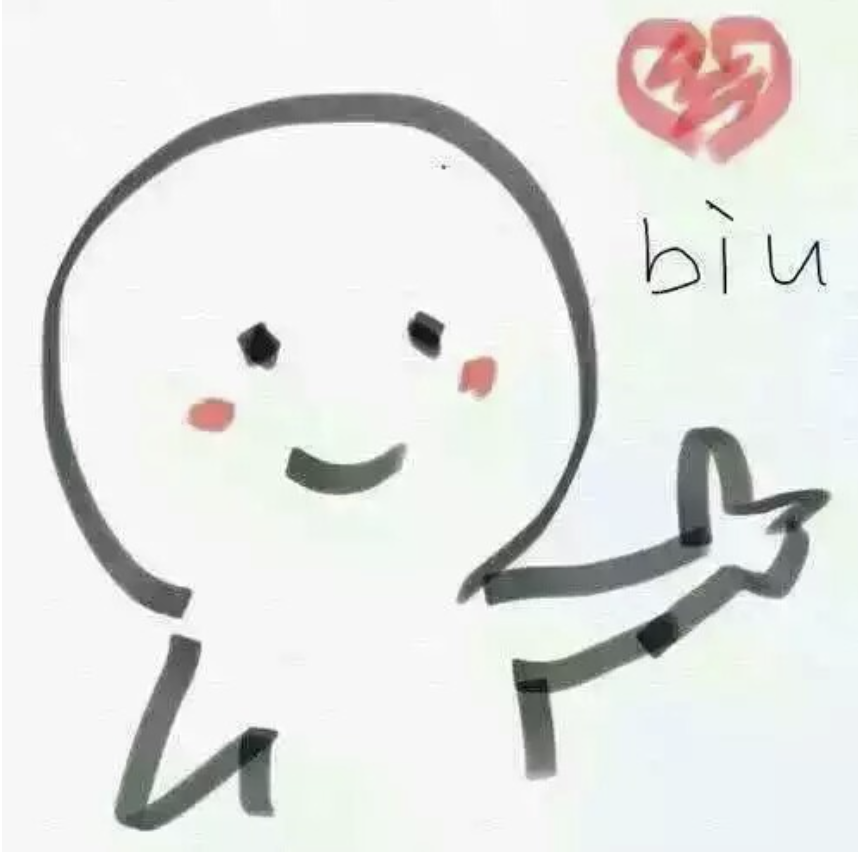