Introduction to this section:
For example, what this section brings to you is the comparison between TouchListener and OnTouchEvent, as well as multi-touch knowledge points! TouchListener is based on listening, while OnTouchEvent is based on callback! Here are two simple examples to deepen your understanding!
1. TouchListener Based on Monitoring
Code example:
Achievement effect diagram:
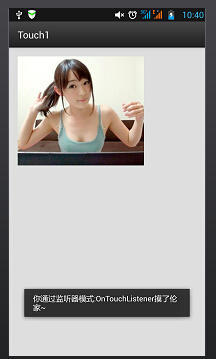
Implementation code: main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MyActivity"> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imgtouch" android:background="@drawable/touch"/> </RelativeLayout>
MainAcitivity.java
public class MyActivity extends ActionBarActivity { private ImageView imgtouch; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_my); imgtouch = (ImageView)findViewById(R.id.imgtouch); imgtouch.setOnTouchListener(new View.OnTouchListener() { @Override public boolean onTouch(View v, MotionEvent event) { Toast.makeText(getApplicationContext(),"You go through the monitor mode:OnTouchListener Touch the Lun family~",Toast.LENGTH_LONG).show(); return true; } }); } }
OnTouchListener related methods and attributes:
OnTouch (View v, Motion Event Event Event Event): The parameters are the components that trigger the touch event in turn. The touch event encapsulates the details of the trigger event, including the type of event, the trigger time and so on. For example, event.getX(),event.getY()
We can also use event.getAction() to judge the action type of touch.
event.getAction == MotionEvent.ACTION_DOWN: Press the event
event.getAction == MotionEvent.ACTION_MOVE: Mobile Event
event.getAction == MotionEvent.ACTION_UP: Pop-up event
2. On TouchEvent () Method Based on Callback
It's also a touch event, but onTouchEvent is more about customized views, which are rewritten in all view classes, and this touch event is based on callbacks, that is, if the value we return is false, then the event will continue to spread outward and be processed by an external container or Activity! Of course, Gesture is also involved, which we will follow. In fact, onTouchEvent and onTouchListener are similar, but the processing mechanism is not used, the former is callback, the latter is monitoring mode!
Code example: Define a simple view, draw a small blue circle, you can follow the fingers to move.
Implementation code: MyView.java
public class MyView extends View{ public float X = 50; public float Y = 50; //Create brush Paint paint = new Paint(); public MyView(Context context,AttributeSet set) { super(context,set); } @Override public void onDraw(Canvas canvas) { super.onDraw(canvas); paint.setColor(Color.BLUE); canvas.drawCircle(X,Y,30,paint); } @Override public boolean onTouchEvent(MotionEvent event) { this.X = event.getX(); this.Y = event.getY(); //Notify component to redraw this.invalidate(); return true; } }
main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MyActivity"> <example.jay.com.touch2.MyView android:layout_width="wrap_content" android:layout_height="wrap_content" /> </RelativeLayout>
Achievement effect diagram:
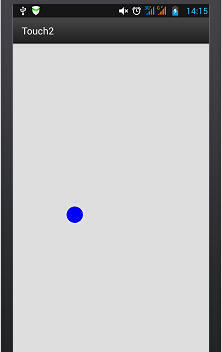
Move with finger touch~
3. Multi-touch
Principles:
The so-called multi-touch is the operation of multiple fingers on the screen, the most used estimate is the zoom function, for example, many picture browsers support zoom! In theory, the Android system itself can handle up to 256 finger touches, depending on the support of mobile phone hardware; however, mobile phones that support multi-touch generally support 2-4 points, of course, some more! We find that MotionEvent is used in the first two points, MotionEvent represents a touch event; we can judge which operation is based on event. getAction () & MotionEvent. ACTION_MASK. In addition to the three single-point operations described above, there are two multi-point special operations:
MotionEvent.ACTION_POINTER_DOWN: Triggered when a point on the screen has been pressed and then another point is pressed.
MotionEvent.**ACTION_POINTER_UP: Triggered when multiple points on the screen are pressed and one of them is loosened (that is, when the non-last point is loosened).
The simple process is probably like this:
- When a finger touches the screen - > trigger ACTION_DOWN event
- Then another finger touches the screen - > trigger ACTION_POINTER_DOWN event, if there are other finger touches, continue to trigger
- One finger left the screen - > trigger ACTION_POINTER_UP event, continue to have a finger left, continue to trigger
- When the last finger leaves the screen - > trigger ACTION_UP event
- And during the whole process, ACTION_MOVE events will be triggered continuously.
We can get the location of different touch points by event.getX(int) or event.getY(int). For example, event.getX(0) can get the X coordinate of the first contact point, event.getX(1) can get the X coordinate of the second contact point, and so on. In addition, we can also call getPoCount () method of MotionEvent object to determine how many fingers are touching at present.~
Code example:
Well, let's write an example of the most common single-finger drag and double-finger zoom pictures.
Achievement effect diagram:
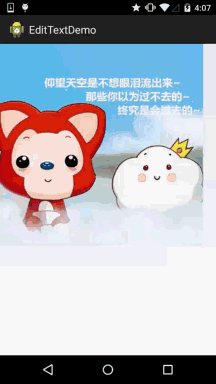
Implementation code:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" > <ImageView android:id="@+id/img_test" android:layout_width="match_parent" android:layout_height="match_parent" android:scaleType="matrix" android:src="@drawable/pic1" /> </RelativeLayout>
MainActivity.java
public class MainActivity extends Activity implements OnTouchListener { private ImageView img_test; // Zoom control private Matrix matrix = new Matrix(); private Matrix savedMatrix = new Matrix(); // Representation of different states: private static final int NONE = 0; private static final int DRAG = 1; private static final int ZOOM = 2; private int mode = NONE; // Define the first pressing point, the focus of the two contact points, and the distance between the two fingers of the accident: private PointF startPoint = new PointF(); private PointF midPoint = new PointF(); private float oriDis = 1f; /* * (non-Javadoc) * * @see android.app.Activity#onCreate(android.os.Bundle) */ @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); img_test = (ImageView) this.findViewById(R.id.img_test); img_test.setOnTouchListener(this); } @Override public boolean onTouch(View v, MotionEvent event) { ImageView view = (ImageView) v; switch (event.getAction() & MotionEvent.ACTION_MASK) { // Single finger case MotionEvent.ACTION_DOWN: matrix.set(view.getImageMatrix()); savedMatrix.set(matrix); startPoint.set(event.getX(), event.getY()); mode = DRAG; break; // Double fingers case MotionEvent.ACTION_POINTER_DOWN: oriDis = distance(event); if (oriDis > 10f) { savedMatrix.set(matrix); midPoint = middle(event); mode = ZOOM; } break; // Finger release case MotionEvent.ACTION_UP: case MotionEvent.ACTION_POINTER_UP: mode = NONE; break; // Single finger sliding event case MotionEvent.ACTION_MOVE: if (mode == DRAG) { // It's a finger drag. matrix.set(savedMatrix); matrix.postTranslate(event.getX() - startPoint.x, event.getY() - startPoint.y); } else if (mode == ZOOM) { // Two fingers sliding float newDist = distance(event); if (newDist > 10f) { matrix.set(savedMatrix); float scale = newDist / oriDis; matrix.postScale(scale, scale, midPoint.x, midPoint.y); } } break; } // Setting up Matrix for ImageView view.setImageMatrix(matrix); return true; } // Calculate the distance between two touchpoints private float distance(MotionEvent event) { float x = event.getX(0) - event.getX(1); float y = event.getY(0) - event.getY(1); return FloatMath.sqrt(x * x + y * y); } // Calculate the midpoint of two touchpoints private PointF middle(MotionEvent event) { float x = event.getX(0) + event.getX(1); float y = event.getY(0) + event.getY(1); return new PointF(x / 2, y / 2); } }