1, Method of use
1.1 step 1: introduce components
- All code files in the component are a whole, and the splitting of a single code file is not supported, because many common methods are placed in one code file and reuse many codes.
- datehead is the header file and general data structure used by this component.
- datahelper is a general check column function and data export printing function.
- datacreat is used to create data in html format for exporting to pdf and printing data.
- datacsv is used to import and export file csv format.
- dataxls is the core of exporting data to xls.
- dataprint is data export to pdf and print data.
- Core_ Copy the entire dataout directory to the same level directory of your project folder.
- Open the pro file of your project and import components.
INCLUDEPATH += $$PWD/../core_dataout
include ($$PWD/../core_dataout/core_dataout.pri)
- At this point, you can see the core in the project tree view_ Dataout component code.
1.2 step 2: obtain data
- Whether exporting to xls, pdf or printing, the premise is to get the data set to be processed.
- There are basically two ways to get data. One is database query, such as QSqlTableModel, and the other is interface control, such as QTableView, QTableWidget and QStandardItemModel.
- QTableView generally has two data sources: QSqlTableModel and QStandardItemModel.
- The data set is based on one row of data, with English semicolons in the middle; Separate them, and finally put them into QStringList.
1.2.1 example QSqlTableModel
void frmDataOut2::on_btnLoad_clicked()
{
model->setTable("MsgInfo");
model->select();
ui->tableView->setModel(model);
for (int i = 0; i < columnCount; i++) {
model->setHeaderData(i, Qt::Horizontal, columnNames.at(i));
ui->tableView->setColumnWidth(i, columnWidths.at(i));
}
}
QStringList frmDataOut2::getContent()
{
QStringList content;
QString sql = QString("select * from MsgInfo limit %1").arg(100);
QSqlQuery query;
if (!query.exec(sql)) {
return content;
}
//Loop through data
while (query.next()) {
QStringList list;
for (int i = 0; i < column; i++) {
list << query.value(i).toString();
}
content << list.join(";");
}
return content;
}
1.2.2 example QStandardItemModel
void frmSimple::on_btnLoad_clicked()
{
//wipe data
model->clear();
//Set the number of columns, column title and column width
model->setColumnCount(column);
for (int i = 0; i < column; ++i) {
model->setHeaderData(i, Qt::Horizontal, columnNames.at(i));
ui->tableView->setColumnWidth(i, columnWidths.at(i));
}
//Loop add row data
for (int i = 0; i < row; ++i) {
//Loop to add a row of columns
QList<QStandardItem *> items;
for (int j = 0; j < column; ++j) {
QStandardItem *item = new QStandardItem;
item->setText(QString("that 's ok%1_column%2").arg(i + 1).arg(j + 1));
items << item;
}
model->appendRow(items);
}
ui->tableView->setModel(model);
}
QStringList frmSimple::getContent()
{
//Get data from QTableView
QStringList content;
for (int i = 0; i < row; ++i) {
QStringList list;
for (int j = 0; j < column; ++j) {
list << model->item(i, j)->text();
}
//Each line of data is stored as a whole string
content << list.join(";");
}
return content;
}
1.2.3 example QTableWidget
void frmDataOut1::on_btnLoad_clicked()
{
QStringList list;
list << "Defense zone Online" << "Zone offline" << "Zone bypass" << "Zone alarm" << "Zone failure";
ui->tableWidget->clearContents();
for (int i = 0; i < rowCount; i++) {
//Randomly generated alarm content
int index = rand() % 4;
QTableWidgetItem *item1 = new QTableWidgetItem(QString::number(i + 1));
QTableWidgetItem *item2 = new QTableWidgetItem("a sector" + QString::number(i + 1));
QTableWidgetItem *item3 = new QTableWidgetItem("Host reporting");
QTableWidgetItem *item4 = new QTableWidgetItem(list.at(index));
QTableWidgetItem *item5 = new QTableWidgetItem(DATETIME);
item5->setTextAlignment(Qt::AlignCenter);
ui->tableWidget->setItem(i, 0, item1);
ui->tableWidget->setItem(i, 1, item2);
ui->tableWidget->setItem(i, 2, item3);
ui->tableWidget->setItem(i, 3, item4);
ui->tableWidget->setItem(i, 4, item5);
}
}
QStringList frmDataOut1::getContent()
{
QStringList content;
for (int i = 0; i < row; i++) {
QStringList list;
for (int j = 0; j < column; j++) {
list << ui->tableWidget->item(i, j)->text();
}
content << list.join(";");
}
return content;
}
1.3 step 3: set data
- Whether exporting to xls, pdf or printing, you need to set the data structure and pass in the information such as column name, column width and data set.
- If you are exporting to xls, you also need to set the file name and table name.
- If you are exporting to pdf, you need to set the file name.
- You do not need to set the file name and table name for printing.
void frmSimple::on_btnXls_clicked()
{
//Set structure data
DataContent dataContent;
//Fill content
dataContent.content = getContent();
//Set column name and width
dataContent.columnNames = columnNames;
dataContent.columnWidths = columnWidths;
//Set file name
dataContent.fileName = "d:/0.xls";
//set a table name
dataContent.sheetName = "Test information";
//Call static function to save
DataXls::saveXls(dataContent);
//Open the file you just exported
QUIHelper::openFile(dataContent.fileName, "Export test information");
}
void frmSimple::on_btnPdf_clicked()
{
//Set structure data
DataContent dataContent;
//Fill content
dataContent.content = getContent();
//Set column name and width
dataContent.columnNames = columnNames;
dataContent.columnWidths = columnWidths;
//Set file name
dataContent.fileName = "d:/0.pdf";
//Call static function to save
DataPrint::savePdf(dataContent);
//Open the file you just exported
QUIHelper::openFile(dataContent.fileName, "Export test information");
}
void frmSimple::on_btnPrint_clicked()
{
//Set structure data
DataContent dataContent;
//Fill content
dataContent.content = getContent();
//Set column name and width
dataContent.columnNames = columnNames;
dataContent.columnWidths = columnWidths;
//Call static function to print
DataPrint::print(dataContent);
}
1.4 step 4: execute operation
- The data import and export component dataout provides a unified data structure for setting data and other parameters.
- It encapsulates the unified static function DataXls::saveXls to export to xls, DataPrint::savePdf to export to pdf and DataPrint::print to print data.
- It supports various detailed and complex parameter settings and multi-threaded operation of massive data export. For details, see the detailed usage demo.
//Call static function and save to xls
DataXls::saveXls(dataContent);
//Call static function and save to pdf
DataPrint::savePdf(dataContent);
//Call static function to print data
DataPrint::print(dataContent);
2, Functional features
- The component integrates exporting data to csv, xls, pdf and printing data at the same time.
- All operations are provided with static methods without new. Structural data is adopted for parameter settings such as data and attributes, which is very convenient.
- It also supports QTableView, QTableWidget, QStandardItemModel, QSqlTableModel and other data sources.
- Provide static methods to directly pass in QTableView and QTableWidget controls to automatically identify column name, column width and data content.
- Each group of functions provides a separate complete example with detailed comments, which is very suitable for Qter programmers at all stages.
- The original data export mechanism does not rely on any third-party libraries such as office components or operating systems, and supports embedded linux.
- The speed is super fast. It takes only 2 seconds to complete 100000 rows of data in 9 fields.
- It only takes four steps to quickly export massive data, such as 100W records to Excel.
- At the same time, it provides direct write data interface and multi-threaded write data interface without card main interface.
- You can set the title, subtitle and table name.
- You can set the field name, column name and column width of the exported data.
- You can set the automatic stretching and filling of the end column, and the default stretching is more beautiful.
- You can set whether to enable verification filtering data. After enabling, the data that meets the rules will be displayed in special colors.
- Check columns, check rules, check values, and check value data types can be specified.
- The verification rule supports accurate equal to = =, greater than >, greater than or equal to > =, less than <, less than or equal to < =, not equal to! = contains.
- The data type of the check value supports integer int, floating point float and double, and the default text string type.
- You can set the random background color and the column set that needs the random background color.
- It supports grouping and outputting data, such as grouping and outputting data according to equipment, which is convenient for viewing.
- You can set csv separator, line content separator and sub content separator.
- You can set the border width and auto fill data type. The auto data type is on by default.
- You can set whether to turn on the data cell style. It is not turned on by default, which can save about 30% of the file volume.
- You can set horizontal layout, paper margin, etc., such as exporting to pdf and printing data.
- Support mixed arrangement of pictures and texts, export data to pdf and print data, and automatic paging.
- Super flexible, free to change the source code, set the alignment, text color, background color, etc.
- Support any excel form software, including but not limited to excel 2003-2021, wps, openoffice, etc.
- Written in pure Qt, it supports any Qt version + any compiler + any system.
3, Experience address
- Experience address: https://pan.baidu.com/s/1ZxG-oyUKe286LPMPxOrO2A Extraction code: o05q file name: bin_dataout.zip
- Domestic sites: https://gitee.com/feiyangqingyun
- International sites: https://github.com/feiyangqingyun
- Personal homepage: https://blog.csdn.net/feiyangqingyun
- Zhihu homepage: https://www.zhihu.com/people/feiyangqingyun/
4, Renderings
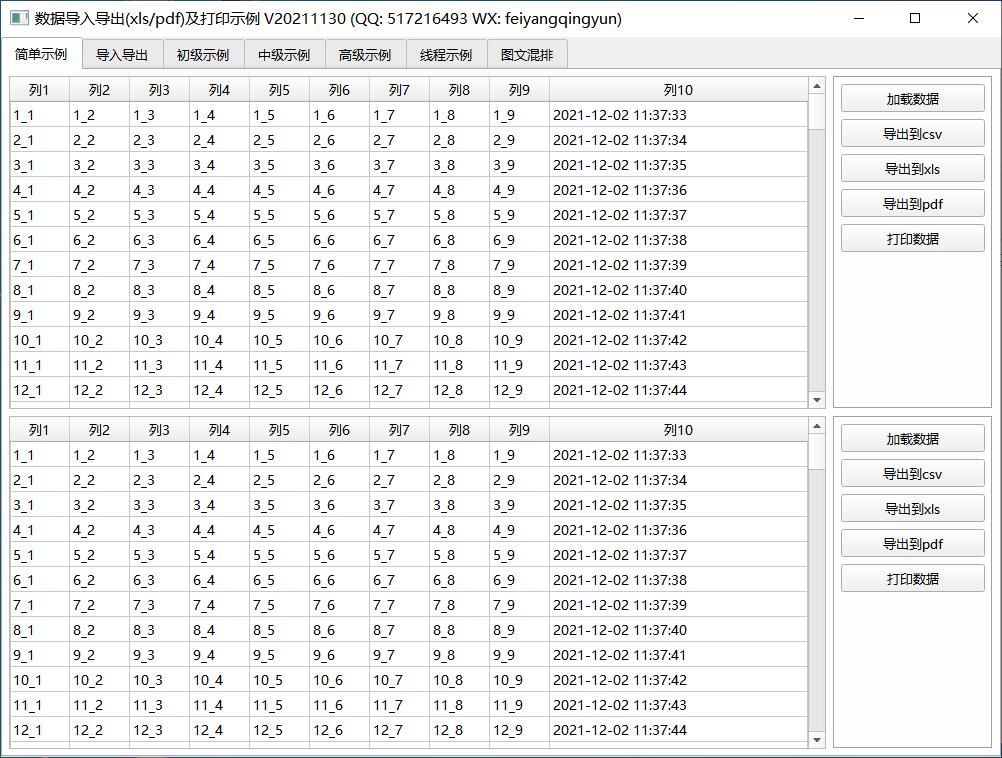