The difference between the three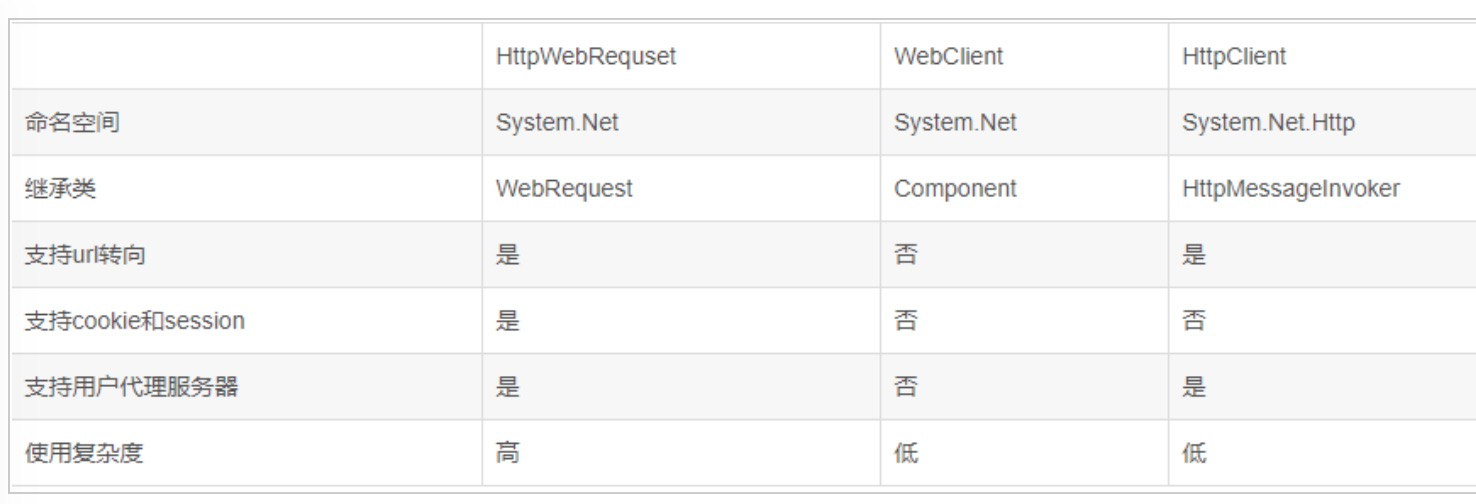
HttpWebRequest
Namespace: system NET, this is NET creators originally developed standard classes for using HTTP requests. Using HttpWebRequest allows developers to control all aspects of the request / response process, such as timeouts, cookies, headers and protocols. Another advantage is that the HttpWebRequest class does not block the UI thread. For example, when you download a large file from a slow response API server, your application's UI does not stop responding. HttpWebRequest is usually used together with WebResponse. One sends a request and the other obtains data. HttpWebRquest is more low-level and can have an intuitive understanding of the whole access process, but it is also more complex.
//POST method public static string HttpPost(string Url, string postDataStr) { HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url); request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded"; Encoding encoding = Encoding.UTF8; byte[] postData = encoding.GetBytes(postDataStr); request.ContentLength = postData.Length; Stream myRequestStream = request.GetRequestStream(); myRequestStream.Write(postData, 0, postData.Length); myRequestStream.Close(); HttpWebResponse response = (HttpWebResponse)request.GetResponse(); Stream myResponseStream = response.GetResponseStream(); StreamReader myStreamReader = new StreamReader(myResponseStream, encoding); string retString = myStreamReader.ReadToEnd(); myStreamReader.Close(); myResponseStream.Close(); return retString; } //GET method public static string HttpGet(string Url, string postDataStr) { HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url + (postDataStr == "" ? "" : "?") + postDataStr); request.Method = "GET"; request.ContentType = "text/html;charset=UTF-8"; HttpWebResponse response = (HttpWebResponse)request.GetResponse(); Stream myResponseStream = response.GetResponseStream(); StreamReader myStreamReader = new StreamReader(myResponseStream, Encoding.GetEncoding("utf-8")); string retString = myStreamReader.ReadToEnd(); myStreamReader.Close(); myResponseStream.Close(); return retString; }
WebClient
Namespace system Net, webclient is a higher-level abstraction created by Httpwebrequest to simplify the most common tasks. In the process of use, you will find that it lacks basic header and timeout settings, but these can be realized by inheriting Httpwebrequest. Relatively speaking, webclient is simpler than WebRequest. It is equivalent to encapsulating the request and response methods. However, it should be noted that webclient and WebRequest inherit different classes, and they have no relationship in inheritance. The number of milliseconds is much simpler than that of webclient, but the number of milliseconds used directly is much less than that of webclient.
public class WebClientHelper { public static string DownloadString(string url) { WebClient wc = new WebClient(); //wc.BaseAddress = url; // Set root directory wc.Encoding = Encoding.UTF8; //Set the access code. If this line is not added, the Chinese character string obtained will be garbled string str = wc.DownloadString(url); return str; } public static string DownloadStreamString(string url) { WebClient wc = new WebClient(); wc.Headers.Add("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/76.0.3809.132 Safari/537.36"); Stream objStream = wc.OpenRead(url); StreamReader _read = new StreamReader(objStream, Encoding.UTF8); //Create a new read stream and read it with the specified code. Here is utf-8 string str = _read.ReadToEnd(); objStream.Close(); _read.Close(); return str; } public static void DownloadFile(string url, string filename) { WebClient wc = new WebClient(); wc.DownloadFile(url, filename); //Download File } public static void DownloadData(string url, string filename) { WebClient wc = new WebClient(); byte [] bytes = wc.DownloadData(url); //Download to byte array FileStream fs = new FileStream(filename, FileMode.Create); fs.Write(bytes, 0, bytes.Length); fs.Flush(); fs.Close(); } public static void DownloadFileAsync(string url, string filename) { WebClient wc = new WebClient(); wc.DownloadFileCompleted += DownCompletedEventHandler; wc.DownloadFileAsync(new Uri(url), filename); Console.WriteLine("Downloading..."); } private static void DownCompletedEventHandler(object sender, AsyncCompletedEventArgs e) { Console.WriteLine(sender.ToString()); //The object that triggered the event Console.WriteLine(e.UserState); Console.WriteLine(e.Cancelled); Console.WriteLine("Asynchronous download completed!"); } public static void DownloadFileAsync2(string url, string filename) { WebClient wc = new WebClient(); wc.DownloadFileCompleted += (sender, e) => { Console.WriteLine("Download complete!"); Console.WriteLine(sender.ToString()); Console.WriteLine(e.UserState); Console.WriteLine(e.Cancelled); }; wc.DownloadFileAsync(new Uri(url), filename); Console.WriteLine("Downloading..."); } }
HttpClient
HttpClient is NET4.5 introduces an HTTP client library with the namespace system Net. Http ,. Before NET 4.5, we may use WebClient and HttpWebRequest to achieve the same purpose. HttpClient takes advantage of the latest task-oriented mode, which makes it very easy to process asynchronous requests. It is suitable for multiple request operations. Generally, after setting the default header, you can make repeated requests. Basically, you can submit any HTTP request with one instance. HttpClient has a warm-up mechanism. It is slow to access for the first time, so you should not use HttpClient. Just use new. You should use singleton or other methods to obtain the instance of HttpClient
public class HttpClientHelper { private static readonly object LockObj = new object(); private static HttpClient client = null; public HttpClientHelper() { GetInstance(); } public static HttpClient GetInstance() { if (client == null) { lock (LockObj) { if (client == null) { client = new HttpClient(); } } } return client; } public async Task<string> PostAsync(string url, string strJson)//post asynchronous request method { try { HttpContent content = new StringContent(strJson); content.Headers.ContentType = new System.Net.Http.Headers.MediaTypeHeaderValue("application/json"); //Asynchronous Post request from HttpClient HttpResponseMessage res = await client.PostAsync(url, content); if (res.StatusCode == System.Net.HttpStatusCode.OK) { string str = res.Content.ReadAsStringAsync().Result; return str; } else return null; } catch (Exception ex) { return null; } } public string Post(string url, string strJson)//post synchronization request method { try { HttpContent content = new StringContent(strJson); content.Headers.ContentType = new System.Net.Http.Headers.MediaTypeHeaderValue("application/json"); //client.DefaultRequestHeaders.Connection.Add("keep-alive"); //Post request from HttpClient Task<HttpResponseMessage> res = client.PostAsync(url, content); if (res.Result.StatusCode == System.Net.HttpStatusCode.OK) { string str = res.Result.Content.ReadAsStringAsync().Result; return str; } else return null; } catch (Exception ex) { return null; } } public string Get(string url) { try { var responseString = client.GetStringAsync(url); return responseString.Result; } catch (Exception ex) { return null; } } }
HttpClient has a preheating mechanism, and the first request is slow; It can be solved by sending a head request before initialization:
_httpClient = new HttpClient() { BaseAddress = new Uri(BASE_ADDRESS) }; //Warm up HttpClient _httpClient.SendAsync(new HttpRequestMessage { Method = new HttpMethod("HEAD"), RequestUri = new Uri(BASE_ADDRESS + "/") }) .Result.EnsureSuccessStatusCode();