Realization idea
- Define student classes
- Add student code
- View student coding
- Delete student code
- Modify students' code writing
- Coding of main interface
Define student classes
- Student: Student
- Member variables: student number: ID, name, age, address: Address
- Construction method: nonparametric construction
- Member method: each member variable corresponds to get, set and toString methods
class Student2{
private int ID=001;
private static int COUNT=001;
private String name;
private String age;
private String address;
public Student2() {
ID = COUNT;
COUNT++;
}
public int getID() {
return ID;
}
public void setID(int iD) {
ID = iD;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return "Student2 [ID=" + ID + ", name=" + name + ", age=" + age + ", address=" + address + "]";
}
}
Add student code writing
- Define a method for adding students
- A prompt message is displayed to prompt what information to enter
- Enter the data required by the student object with the keyboard
- Create a student object and assign the data entered by the keyboard to the member variable of the student object
- Add student object to collection
- Give a prompt of successful addition
public void addStudnet(ArrayList<Student2> arrayList) {
System.out.println("Please enter student name:");
String name=scanner.nextLine();
System.out.println("Please enter student address:");
String address=scanner.nextLine();
System.out.println("Please enter student age:");
String age=scanner.nextLine();
Student2 s1=new Student2();
s1.setName(name);
s1.setAddress(address);
s1.setAge(age);
arrayList.add(s1);
System.out.println("Student added successfully");
}
View student code writing
- Define a method for viewing student information
- Judge whether there is student information
- If there is student information, take out the data in the set and display the student information in the corresponding format
public void findAllStudent(ArrayList<Student2> arrayList) {
if (arrayList.size()==0) {
System.out.println("No student information, please add data.");
return;
}
for (int i = 0; i < arrayList.size(); i++) {
Student2 s2=arrayList.get(i);
System.out.println(s2.toString());
}
}
Delete student code writing
- Define a method to delete student information
- Display prompt information
- Enter the student number to be deleted
- Determine whether the student exists
- If it exists, traverse the collection and delete the student object from the collection
- Prompt for successful deletion
public void deleteStudent(ArrayList<Student2> arrayList) {
System.out.println("Please enter the student to delete ID:");
int deleteId=scanner.nextInt();
boolean flag=false;
for (int i = 0; i < arrayList.size(); i++) {
Student2 s2=arrayList.get(i);
if (s2.getID()==deleteId) {
arrayList.remove(i);
System.out.println("Delete succeeded");
flag=true;
break;
}
}
if (flag==false)
System.out.println("The information does not exist");
}
Modify student code writing
- Define a method for modifying student information
- Display prompt information
- Enter the student number to be modified on the keyboard
- Determine whether the student exists
- If it exists, enter the student information to be modified with the keyboard
- Traverse the set and modify the corresponding student information
- Prompt for successful modification
public void updateStudent(ArrayList<Student2> arrayList) {
System.out.println("Please enter the student to modify ID:");
int updateId=scanner.nextInt();
boolean flag=false;
for (int i = 0; i < arrayList.size(); i++) {
if (arrayList.get(i).getID()==updateId) {
System.out.println("Please enter the new student name:");
scanner.nextLine();
String name=scanner.nextLine();
System.out.println("Please enter the new age of the student:");
String age=scanner.nextLine();
System.out.println("Please enter the student's new address:");
String address=scanner.nextLine();
Student2 s1=new Student2();
s1.setID(updateId);
s1.setName(name);
s1.setAddress(address);
s1.setAge(age);
arrayList.set(i, s1);
System.out.println("Update succeeded");
flag=true;
break;
}
}
if (flag==false)
System.out.println("The information does not exist");
}
Main interface code writing
- Write the main interface with output statements
- Using Scanner to realize the process of keyboard entry
- Complete the selection of operation with switch statement
- Complete the cycle and return to the main interface again
public static void main(String[] args) {
StudentManager sm=new StudentManager();
ArrayList<Student2> arrayList=new ArrayList<Student2>();
while (true) {
System.out.println("----Welcome to the student management system---");
System.out.println("1. Add student");
System.out.println("2. Delete student");
System.out.println("3. Modify student");
System.out.println("4. View all students");
System.out.println("5. sign out");
System.out.println("Please enter your choice:");
Scanner scanner=new Scanner(System.in);
int x=scanner.nextInt();
switch (x) {
case 1:
sm.addStudnet(arrayList);
break;
case 2:
sm.deleteStudent(arrayList);
break;
case 3:
sm.updateStudent(arrayList);
break;
case 4:
sm.findAllStudent(arrayList);
break;
case 5:
System.out.println("Thank you for using");
System.exit(0);//jvm exit
}
}
}
test
Add information
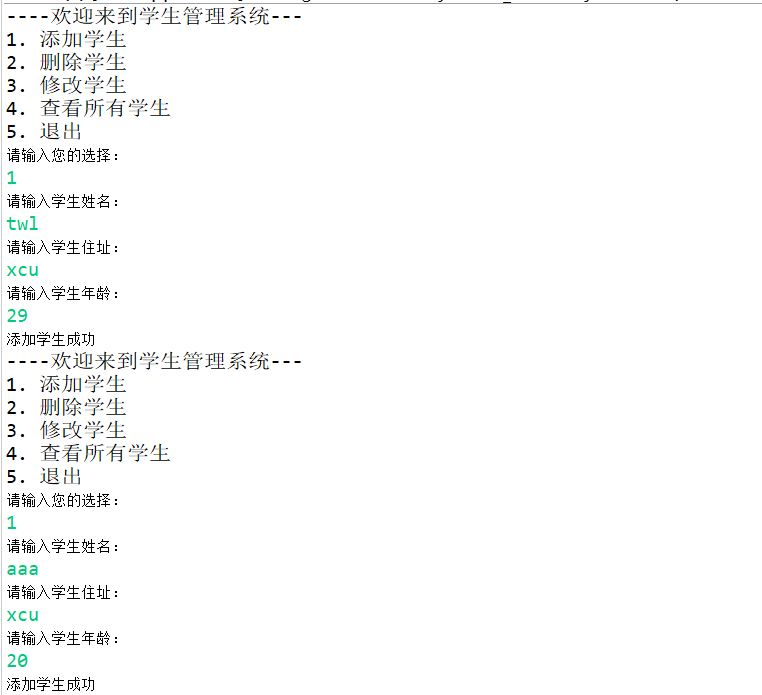
see information

Delete information
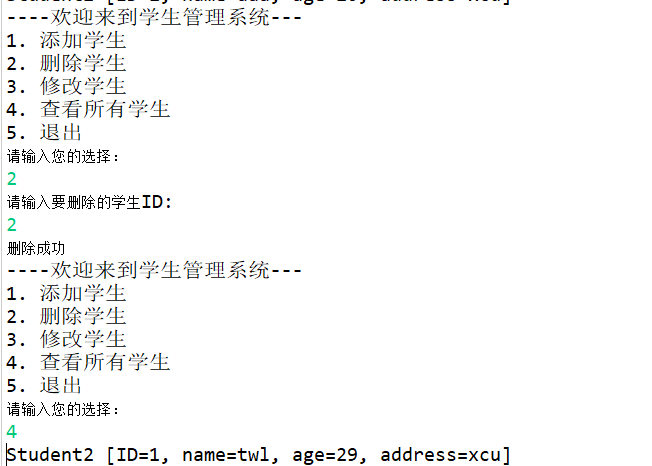
Modify information
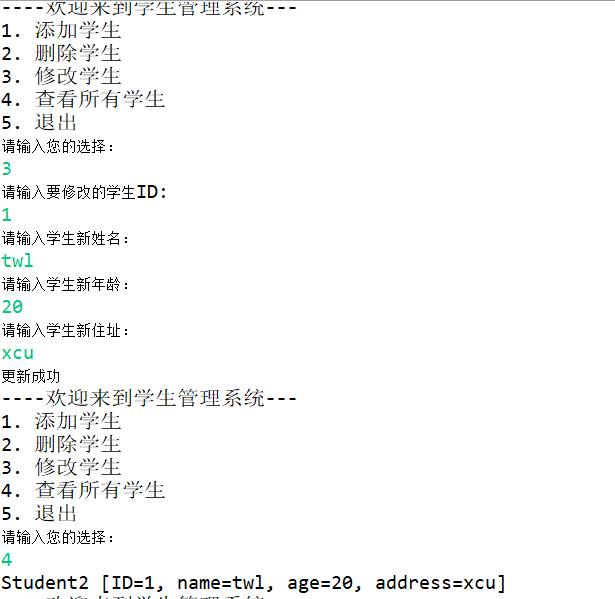