Because vite uses the modular function of the native browser, node cannot be used internally, so there is no require method. This section mainly introduces the common use of vite, including css, ts, env environment variables and import Meta and other functions.
CSS processing
Basic use
Index. Is added in src directory css
@import url('@styles/others.css') // For HTML,: root represents the < HTML > element, which is the same as the HTML selector except for its higher priority :root { --bg-color: pink; } body { background-color: var(--bg-color); }
vite recommended css variable
Configure path alias
// vite.config.js export default defineConfig({ ... resolve: { alias: { '@styles': "/src/styles" } } })
Using postcss
Postcss has been integrated in vite. If you want to use it, you can directly create a new postcss in the root directory config. js
module.exports = { plugins: [ require('@postcss-plugins/console') // Using the postcss plug-in ] }
Use (remember to modify the configuration file and restart the project)
body { background-color: var(--main-bg-color); @console.warn hello postcss // It will print on the command line, not on the browser console }
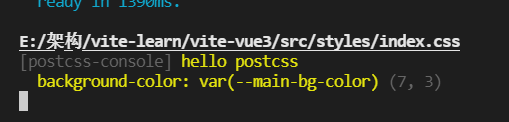
css modules
css modules can make your css use like a module. The internal class names have become object attributes. It is also convenient to use in vite. The file name is XXX module. css form. css modules learning
// styles/test.module.css .moduleTest { color: red; }
Used in vue files
<script setup> import classModule from '@styles/test.module.css' console.log(classModule) // Object form </script> Variable form use <template> <p :class="classModule.moduleTest">ppppppppppp</p> </template>
Preprocessor
The advantage of vite is that there is no need to configure loader. Many of them have been built-in. Here, take less as an example, yarn add less
// styles/test.less @bgColor: red; .root { background-color: @bgColor; }
The use of css in vite is introduced here. If you have any questions, you are welcome to leave a message for discussion.
ts use
tsc needs to be installed globally, and tsconfig needs to be created in the root path js
vite's attitude towards ts is that it only compiles and does not verify. It just processes ts into js for browsers, but ts syntax cannot do verification. We rely more on vscode and Volar plug-ins, which can be configured if packaged for verification
"scripts": { "build": "tsc --noEmit && vite build" }
Because ts does not take effect on vue files, add ts support for vue files, yarn add vue TSC, and modify the configuration
"scripts": { "build": "vue-tsc --noEmit && tsc --noEmit && vite build" }
We can configure "isolatedModules": true, because vite development environment cannot verify ts correctly,
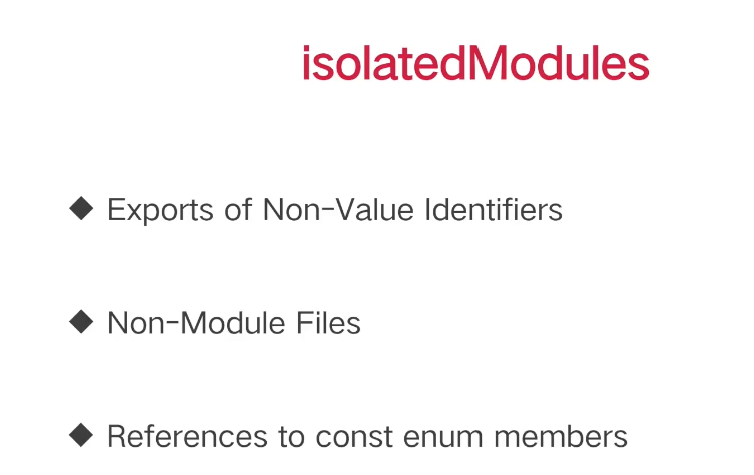
isolatedModules function
- exports of Non-Value Identifiers// a.ts export interface Test{} // b.ts import { Test } from './a' Export {test} / / an error is reported. Each file is exported without definition. Each file must be a module. Either import or export const enum Test{ a = 0, b = 1 } let test = { age: Test.a. / / an error will be reported. There is no test enumeration here, resulting in an error }
- Non-Modules Files
- References to const enum members
We know that node syntax cannot be used in vite, access path and environment variables need to use import Meta, we need it in tsconfig JS
{ "compilerOptions": { "target": "esnext", "module": "esnext", "moduleResolution": "node", "strict": true, "jsx": "preserve", "sourceMap": true, "resolveJsonModule": true, "esModuleInterop": true, "lib": [ "ESNext", "DOM" ], "types": [ "vite/client" // Use import with vite meta. xxx ], "isolatedModules": true, }, "include": [ // Where to check the ts file "src/**/*.ts", "src/**/*.d.ts" ] }
Static file processing
Basic use
<script setup> import logo from './assets/logo.png' </script> <template> <img :src="logo" alt=""> </template>
Self contained types
We splice url or raw after the imported file, and print the path and content of the file
import test from './test?url' console.log(test, 11) import test1 from './test?raw' console.log(test1, 22)
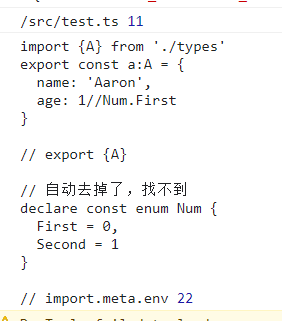
web worker
vite also has a built-in web worker for us. We can open a separate thread for time-consuming calculation
// main.js import Worker from './worker?worker' const worker = new Worker() worker.onmessage = function (e) { console.log(e) }
let i = 0 function timedCount() { i += 1 postMessage(i) setTimeout(timedCount, 1000) } timedCount()
Processing json files
vite helped us automatically handle the json format
import {version} from '../package.json' console.log(version)
webassembly
We need to generate wasm files in other languages. Here we use assemblyscript and install npm i assemblyscript
New assembly ts
export function fib(n: i32): i32 { var a = 0, b = 1 if (n > 0) { while (--n) { let t = a + b a = b b = t } return b } return a }
Execution/ node_modules/.bin/asc assembly --binaryFile fib.wasm
main.js
import init from './fib.wasm' init().then(m => { console.log(m.fib(10)) // 55 })
Integrated eslint pritter
eslint
New root directory eslintrc.js, install NPM I eslint config standard eslint plugin import eslint plugin promise eslint plugin node - D
module.exports = {
extends: 'standard',
globals: {
postMessage: true, // Prevent global undefined error reporting
},
}
### pritter New root directory `.prettierrc` Documents,
{
"semi": false, / / no semicolon
"singleQuote": true, / / single quotation mark
}
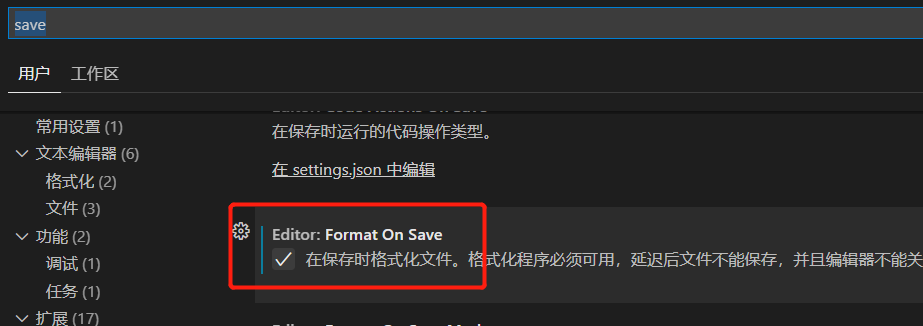 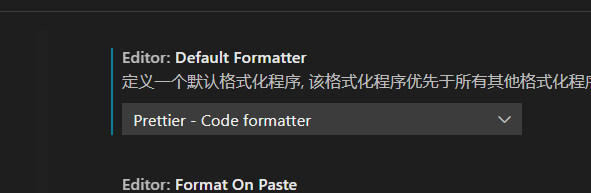 ### Configuration verification
"lint": "eslint --ext js src/"
If we want to `commit` Pre verification, used here `husky` Package, the root directory must have `.git` Directory, execute the following command to verify - npx husky install - npx husky add .husky/pre-commit "npm run lint" ## environment variable `vite` The specified environment variable does not exist `import.meta.env` Create a centralized environment file (file name) under the root path `VITE_APP=xxx` (form) 1. `.env` Variables in any environment will exist 2. `env.development` Test environment usage 3. `.env.development.local` `local` Local environment to `development` The packaged environment runs on other machines and can be distinguished,`local` High priority 4. `.env.production` Variables used in formal environment 5. `.env.test` Variables used by the test environment, configuration commands `vite --mode test` If you use `ts` Development, configurable `ts` type Root directory creation `vite-env.d.ts` ```ts
/// <reference types="vite/client" />
interface ImportMetaEnv {
VITE_TITLE: string
}
## hmr The implementation of hot update is based on `ws`,I won't introduce it in detail here. If we build a basic template, the basic logic is as follows ```js
// main.js
document.querySelector("#app").innerHTML = `
<h1>Hello Vite!</h1>
<a href="https://vitejs.dev/guide/features.html" target="_blank">Documentation</a>
`;
}
If we change the text content inside, we find that the page is refreshed, not hot update. If we want to realize hot update, we need to modify it to: ```js
//Must export
export function render() {
document.querySelector("#app").innerHTML = `
<h1>Hello Vite!</h1>
<a href="https://vitejs.dev/guide/features.html" target="_blank">Documentation</a>
`;
}
render()
//Hot may not exist, but only works in the development environment. vite build does not have hot
if (import.meta.hot) {
//Files can accept their own hot updates
import.meta.hot.accept((newModule) => {
newModule.render()
// The render of the new module is used here. If you call render directly, the page will not be refreshed, and the original render will be called,
})
}
## glob import We are `vite` Used in `import.meta.xxx` Because `vite` Used in `fast-glob` Third party packages, we can catalog files 1. A set of rules is introduced in a regular way js File, not a `src/glob` folder, `a.js`, `b.js`, `a.json`, `b.json` ```js
const globModules = import.meta.glob('./glob/*')
console.log(globModules)
Object.entries(globModules).forEach((k, v) => {
v().then((m) => console.log(k, m.default))
})
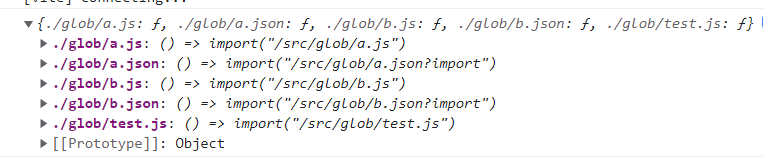 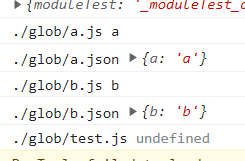 In practical work, for example, multilingual support requires multiple languages `js` Configuration files, you can obtain files in batch Function from [fast-glob](https://github.com/mrmlnc/fast-glob) ## precompile The purpose of precompiling is to turn files that are not recognized by the browser into `esm` File, handle the cache of third-party packages,`vite` Each file is not cached. As long as it is requested, it must be the latest content. 1. `vite` The first use will be slightly slower, because the files to be processed will become recognized by the browser, and the processed packages will exist slowly `node_modules/.vite` Yes. To process files that are not recognized by the browser, for example `.vue` Documents,`commonjs` File to esm file [reference resources](https://juejin.cn/post/7032991450665058311) 2. If we use `lodash` Bag, you can know `lodash` There are many different files under the. The files are combined and packaged together to avoid separation `import`,This will cause the browser to send multiple requests 3. We can configure `vite` Precompiled ```js
export default defineConfig({
//Packages that need to be precompiled can be configured by themselves
optimizeDeps: {
include: [],
exclude: []
}
})
This section introduces the common usage of vite. The next section will introduce the usage of rollup. If you have any questions, please leave a message. Thank you for reading!