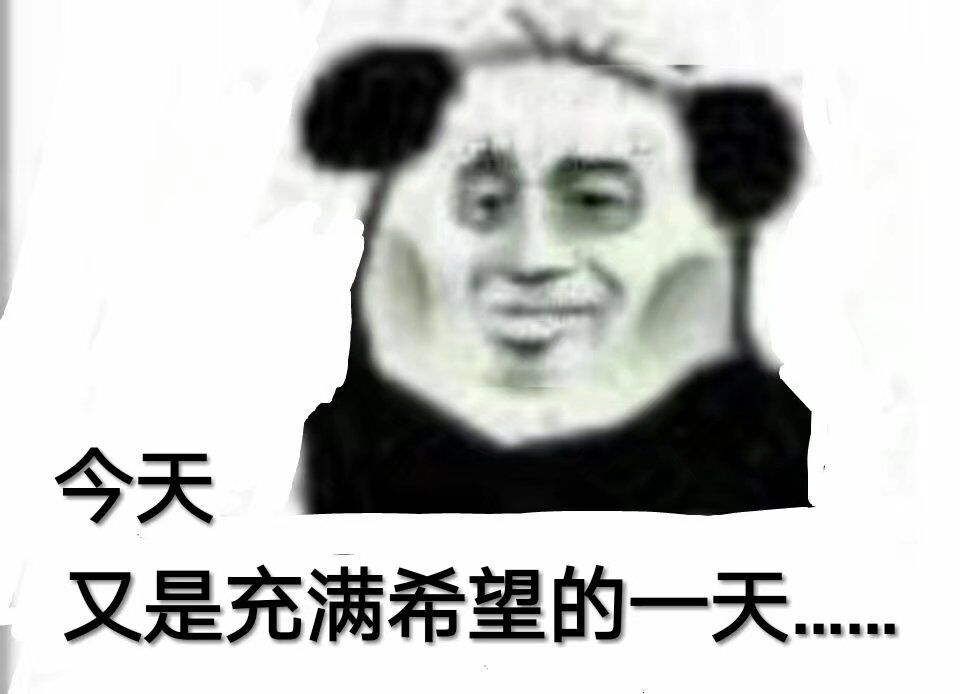
I can't remember what I used to refer to, so I can only turn back (what I learned will be updated)
vue basis interpolation expression
Declarative rendering / text interpolation
Syntax: {expression}}
<template> <div> <h1>{{ msg }}</h1> <h2>{{ obj.name }}</h2> <h3>{{ obj.age>=18 ? 'Grown up':'under age'}}</h3> </div> </template> <script> export default { data() { return{ msg:"hello,world", obj : { name:"zs", age:18 } } } } </script> <style> </style>
vue instruction - v-bind
Objectives:
Set the value of the vue variable for the label property
vue instruction, in essence, is a special html tag attribute, with the characteristics of starting with v -
Each instruction has its own function
Syntax:
v-bind: attribute name = "vue variable"
Abbreviation:
: attribute name = "vue variable"
<!-- vue instructions v-bind Attribute dynamic assignment --> <a v-bind:href="url">I am a label</a> <img src="imgsrc" alt="">
Summary:
Assigning the value of vue variable to dom attribute will affect the label display effect
vue instruction - v-on
Objectives:
Bind events to tags
Syntax:
v-on: event name = "a small amount of code to execute"
v-on: event name = "function in methods"
v-on: event name = "function (argument) in methods"
Abbreviation:
@Event name = "function in methods"
<template> <div> <p>The quantity of goods you want to buy:{{count}}</p> <button v-on:click="count=count+1">Add 1</button> <button v-on:click="addFn">Add 1</button> <button v-on:click="addcountFn(5)">Add 5</button> <button @click="subFn">reduce</button> </div> </template> <script> export default { data(){ return{ count:1, } }, methods:{ addFn(){ this.count++ }, addcountFn(num){ this.count += num }, subFn(){ this.count-- } } } </script> <style> </style>
Summary:
Common @ event names, binding events to dom tags, and = event handling functions on the right
vue instruction - v-on event object
Objectives:
In the vue event handler function, get the event object
Syntax:
No parameters, received directly through formal parameters
With parameters, it refers to the event object and passes it to the event handler function through $event
<template> <div> <a @click="one" href="http://Www.baidu.com "> stop Baidu</a> <a @click="two(10,$event)" href="http://Www.baidu.com "> stop going to Baidu</a> </div> </template> <script> export default { methods:{ one(e){ e.preventDefault(); }, two(num,e){ e.preventDefault(); } } } </script> <style> </style>
vue instruction - v-on modifier
Objectives:
After the event. Modifier name - brings more power to the event
Syntax:
@Event name. Modifier = "function in methods"
. stop - prevent event bubbling
. prevent - block default behavior
. once - the event handler is triggered only once during program operation
<template> <div @click="fatherFn"> <button @click.stop="btn">.syop Prevent event bubbling</button> <a href="https://Www.baidu.com "@ click. Prevent =" BTN ">. Prevent block default behavior</a> <button @click.once="btn">.once During program operation,The event handler is triggered only once</button> </div> </template> <script> export default { methods:{ fatherFn(){ console.log("father Triggered"); }, btn(){ console.log(1); } } } </script> <style> </style>
Summary:
Modifiers extend additional functionality to events
vue command - v-on key modifier
Objectives:
Add modifiers to keyboard events to enhance capabilities
Syntax:
@keyup.enter - monitors the Enter key
@keyup.esc - monitor return key
<template> <div> <input type="text" @keydown.enter="enterFn"> <hr> <input type="text" @keydown.esc="escFn"> </div> </template> <script> export default { methods:{ enterFn(){ console.log("enter Press enter"); }, escFn(){ console.log("esc Press the button"); } } } </script> <style> </style>
Summary:
Using more event modifiers can improve development efficiency and reduce your own judgment process
vue instruction v-model
Objectives:
Bind the value attribute and vue data variable together in two directions;
Syntax:
v-model = "vue data variable"
Bidirectional data binding:
Data change - > View auto synchronization
View change - > automatic data synchronization
<template> <div> <!-- v-model:Is to achieve vuejs Variables and form labels value attribute,Bidirectional bound instructions --> <div> <span>user</span> <input type="text" v-model="username"> </div> <div> <span>password</span> <input type="text" v-model="pass"> </div> <div> <span>From:</span> <!-- Drop down menu to bind to select upper --> <select v-model="from"> <option value="Beijing">Beijing</option> <option value="Nanjing City">Nanjing</option> <option value="Tianjin">Tianjin</option> </select> </div> <div> <!-- (important) Check box encountered, v-model Variable value of Non array - Associated is the of the check box checked attribute array - Associated is the of the check box value attribute --> <span>hobby: </span> <input type="checkbox" v-model="hobby" value="draw">draw <input type="checkbox" v-model="hobby" value="listen to the music">listen to the music <input type="checkbox" v-model="hobby" value="watch comic and animation">watch comic and animation </div> <div> <span>Gender:</span> <input type="radio" value="male" name="sex" v-model="gender">male <input type="radio" value="female" name="sex" v-model="gender">female </div> <div> <span>self-introduction</span> <textarea v-model="intro"></textarea> </div> </div> </template> <script> export default { data(){ return{ username:"", pass:"", from: "", hobby: [], sex: "", intro: "", }; // Summary: // Pay special attention to: v-model, which is in the multi checkbox state of input[checkbox] // If the variable is not an array, the checked attribute is bound (true/false) - commonly used for: single binding // If the variable is an array, the value in their value attribute is bound - commonly used to collect which values are checked } } </script> <style> </style>
Summary:
At this stage, v-model can only be used on form elements. After learning the components, we will talk about the advanced usage of v-model
vue instruction v-model modifier
Objectives:
Let v-model have more powerful functions;
Syntax:
v-model. Modifier = "vue data variable"
. number is converted to numeric type with parseFloat
. trim removes leading and trailing white space characters
. lazy is triggered at change instead of input
<template> <div> <div> <span>Age:</span> <!-- .number Modifier -Put value parseFloat Reprint the value and give it to v-model Corresponding variable --> <input type="text" v-model.number="age"> </div> <div> <span>Life motto:</span> <!-- .trim modification -Remove the leading and trailing spaces --> <input type="text" v-model.trim="motto"> </div> <div> <span>self-introduction:</span> <!-- .lazy Modifier -Loss of focus when content changes(onchange event),Sync content to v-model Variable of --> <textarea v-model.lazy="intro"></textarea> </div> </div> </template> <script> export default { data() { return { age: "", motto: "", intro: "" } } } </script> <style> </style>
Summary:
v-model modifier, which can preprocess the value, is very efficient and easy to use
vue instructions v-text and v-html
Objectives:
Update innerText / innerHTML of DOM object
Syntax:
v-text = "vue data variable"
v-html = "vue data variable"
be careful:
The interpolation expression is overwritten
<template> <div> <!-- v-text Display the value as an ordinary string --> <p v-text="str"></p> <!-- v-html Take the value as html analysis --> <p v-html="str"></p> </div> </template> <script> export default { data(){ return{ str:"<span>I am a span label</span>" } } } </script> <style> </style>
Summary:
v-text displays the value as an ordinary string, and v-html parses the value as HTML
vue instructions v-show and v-if
Objectives:
Controls the hiding or appearance of labels
Syntax:
v-show = "vue variable"
v-if = "vue variable"
Principle:
display:none hidden for v-show (frequently switched)
v-if is removed directly from the DOM tree
Advanced:
v-else use
<template> <div> <!-- v-show perhaps v-if v-show="vue variable" v-if="vue variable" --> <h1 v-show="isOk">v-show My box</h1> <h1 v-if="isOk">v-if My box</h1> <!-- v-show hide: use display:none //Frequent switching v-if hide: Adopt from DOM Tree direct removal //remove --> <div> <p v-if="age">I'm an adult</p> <p v-else>You have to eat more</p> </div> </div> </template> <script> export default { data(){ return{ isOk:true, age:20 } } } </script> <style> </style>
Summary:
Using v-show, v-if and v-else instructions, it is convenient to control the appearance / hiding of a set of labels through variables
vue instruction - v-for
Objectives:
List rendering, where the label structure is, is generated circularly according to the amount of data
Syntax:
v-for = "(value, index) in target structure"
v-for = "value in target structure"
Target structure:
Can traverse array / object / number / string (traversable structure)
be careful:
The temporary variable name of v-for cannot be used outside the range of v-for
<template> <div id="app"> <div id="app"> <!-- v-for Put a set of data, Render as a group DOM --> <!-- Pithy formula: Who will generate the loop, v-for On who --> <p>Student name</p> <ul> <li v-for="(item,index) in arr" :key="item"> {{index}}---{{item}} </li> </ul> <p>Student details</p> <ul> <li v-for="obj in stuArr" :key="obj.id"> <span>{{ obj.name }}</span> <span>{{ obj.sex }}</span> <span>{{ obj.hobby }}</span> </li> </ul> <!-- v-for Traversal object(understand) --> <p>Teacher information</p> <div v-for="(value, key) in tObj" :key="value"> {{ key }} -- {{ value }} </div> <!-- v-for Ergodic integer(understand) - Start with 1 --> <p>Serial number</p> <div v-for="i in count" :key="i">{{ i }}</div> </div> </div> </template> <script> export default { data() { return { arr: ["Xiao Ming", "Xiao Huanhuan", "chinese rhubarb"], stuArr: [ { id: 1001, name: "Sun WuKong", sex: "male", hobby: "Eat peaches", }, { id: 1002, name: "Zhu Bajie", sex: "male", hobby: "Back daughter-in-law", }, ], tObj: { name: "Xiao Hei", age: 18, class: "1 stage", }, count: 10, }; }, } </script> <style> </style>
Summary:
vue is the most commonly used instruction. It lays a sharp tool for pages and quickly assigns data to the same dom structure for cyclic generation
vue basic v-for update monitoring
Objectives:
When the target structure of v-for traversal changes, Vue triggers v-for update
Case 1: array flip
Case 2: array interception
Case 3: update value
Pithy formula:
Changing the array method will lead to v-for update and page update
If the array is not changed, returning a new array will not cause v-for update. You can overwrite the array or this.$set()
<template> <div> <ul> <li v-for="(val,index) in arr" :key="index "> {{val}}</li> </ul> <button @click="revBtn">Array flip</button> <button @click="sliceBtn">Intercept the first 3</button> <button @click="updateBtn">Update first element value</button> </div> </template> <script> export default { data(){ return{ arr:[5,3,9,2,1] } }, methods:{ revBtn(){ // 1. Array flipping allows v-for to update this.arr.reverse() }, sliceBtn(){ // 2. Array slice method will not cause v-for update // slice does not change the original array // this.arr.slice(0, 3) // Resolve v-for update - overwrite original array let newArr = this.arr.slice(0,3) this.arr = newArr }, updateBtn(){ // 3. When updating a value, v-for cannot be monitored // this.arr[0] = 1000; // Solve - this.$set() // Parameter 1: update target structure // Parameter 2: update location // Parameter 3: update value this.$set(this.arr,0,1000) } } } </script> <style> </style>
These methods will trigger array changes, and v-for will monitor and update the page:
push()
pop()
shift()
unshift()
splice()
sort()
reverse()
These methods do not trigger v-for updates:
slice()
filter()
concat()
be careful:
vue cannot be updated by monitoring the assignment action in the array. If necessary, please use Vue.set() or this.$set(), or overwrite the entire array
Summary:
Change the method of the original array to make v-for update
vue basic dynamic class
Objectives:
Use v-bind to set the dynamic value of the label class
Syntax:
: class = "{class name: Boolean}"
<template> <div> <!-- grammar: :class="{Class name: Boolean value}" Usage scenario: vue Variable controls whether the tag should have a class name --> <p :class="{red_str:bool}">dynamic class</p> </div> </template> <script> export default { data(){ return{ bool:true } } } </script> <style> .red_str{ color: red; } </style>
Summary:
Is to save the class name in the vue variable and assign it to the tag