Part I knowledge review:
- What is Vue?
- Two principles and Realization of Vue
- Vue's six instructions
- Vue's filter
Summary of this article
- What is a watch listener?
- What are calculation properties?
- Use of Vue cli scaffold
1, watch listener
1. Introduction:
The watch listener allows developers to monitor the changes of data, so as to do specific operations for the changes of data
When we add a listener to a data object, once the value of the object changes, it will trigger our custom function on the listener to perform the operations defined in the function.
2. Method format listener
(1) Use:
Under the watch node of the Vue instance, define the function with the name of the data object as the method name.
There are two default parameters in the function:
1. New value newVal after the data object is changed
2. Old value oldVal before data object is changed
(2) Disadvantages:
1. It cannot be triggered automatically when you first enter the page
2. If the listener is an object, if the properties in the object change, the listener will not be triggered
(3) Syntax format:
<div id="app"> <input type="text" v-model="username"> </div> const vm = new Vue({ el: '#app', data: { username: 'admin' }, // All listeners should be defined under the watch node watch: { // The listener is essentially a function. To monitor which data changes, you can take the data name as the method name // The new value comes first and the old value comes last username(newVal, oldVal) { console.log(newVal) }) } } })
3. Object format listener
(1) Use
Under the watch node, create an object format listener with the name of the data object, and create a handler (newVal, oldVal) in the object
Method to respond to changes in the value of the data object.
(2) Benefits
1. The listener can be triggered automatically through the immediate option
2. You can use the deep option to let the listener deeply listen for the changes of each attribute in the object
(3) immediate option
By default, Vue components do not call the watch listener after initial loading. If you want the watch listener to be called immediately, you need to use the immediate option.
The example code is as follows
watch: { // Listener that defines the format of the object username: { // Listener handler handler(newVal, oldVal) { console.log(newVal, oldVal) }, // The default value of the immediate option is false // The function of immediate is to control whether the listener will automatically trigger once after the component is loaded! immediate: true } }
(4) deep option
If the watch is listening on an object, if the attribute value in the object changes, it cannot be listened.
At this time, you need to use the deep option and listen for the property changes of the object at the same time.
data: { // User's information object info: { username: 'admin' } }, watch: { info: { handler(newVal) { console.log(newVal.username) }, // Turn on deep listening. As long as any attribute in the object changes, the "object listener" will be triggered deep: true } }
You can also directly listen to a single attribute of the object
watch: { // If you want to listen for changes to the sub attributes of an object, you must wrap a layer of single quotes 'info.username'(newVal) { console.log(newVal) } }
2, Calculation properties
1. Introduction:
Calculating attributes refers to obtaining an attribute value after a series of operations.
The dynamically calculated attribute value can be used by the template structure or methods method.
The calculation attribute should be defined under the calculated node of the Vue instance and in the form of a method. At the end of the method, a string must be return ed
2. Characteristics of calculation attributes
① Although a calculated attribute is defined as a method when declared, the essence of a calculated attribute is an attribute
② The calculation attribute will cache the calculation results, and the operation will be restarted only when the data that the calculation attribute depends on changes
<div> <span>R: </span> <input type="text" v-model.number="r"> </div> <div> <span>G: </span> <input type="text" v-model.number="g"> </div> <div> <span>B: </span> <input type="text" v-model.number="b"> </div> <span>"The result is "+ {{ rgb }}</span> //Displays the value of the calculated property <script> // Create Vue instance and get ViewModel var vm = new Vue({ el: '#app', data: { // gules r: 0, // green g: 0, // blue b: 0 }, // All calculation attributes must be defined under the calculated node // When defining calculation attributes, they should be defined as "method format" computed: { // As a calculation attribute, rgb is defined as a method format, // Finally, in this method, a generated rgb(x,x,x) string is returned rgb() { return `rgb(${this.r}, ${this.g}, ${this.b})` } } }); </script>
3, Use of Vue cli
1. What is Vue cli?
Vue cli is Vue JS development standard tools. It simplifies the process of programmers creating engineering Vue projects based on webpack
So that programmers can focus on writing applications without having to spend days worrying about webpack configuration.
2. Installation and use
Vue cli is a global package on npm
(1) Use the npm install command to easily install it on your computer:
npm install -g @vue/cli
(2) Commands for quickly generating engineering Vue projects based on Vue cli:
vue create Name of the project
(3) Then select the package you need for your project, and Vue cli will automatically install it into the project for you
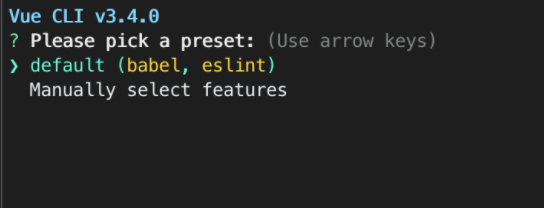
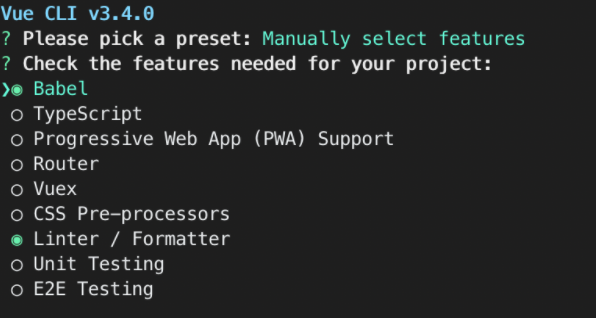
(4) Then the following folder structure will be generated under the project root directory:
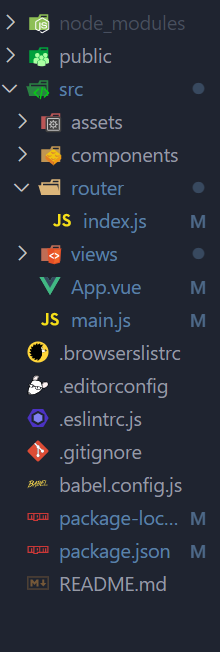
3. Introduction to some generated files
In an engineering project, vue only needs to pass main JS app vue render to index HTML in the specified area.
(after running npm run build, webpack will package the. Vue components in the project and generate dist folder in the root directory of the project. The content of index.html file is rendered by App.vue.)
Of which:
assets folder: stores static resource files used in the project, such as css style sheets and picture resources
Components folder: the reusable components encapsulated by programmers should be placed in the components directory
main.js is the entry file of the project. To run the whole project, execute main js
App.vue is the root component of the project
This will involve the knowledge related to webpack, because Vue cli itself is based on webpack to help us create and configure new engineering front-end projects. If you are still confused, you can see what I wrote before( Front end Engineering: detailed explanation of common configuration of Webpack (dry goods))
Summary:
I wonder if you will have a question after reading it. What is the Vue component I mentioned in this article? What does it do
In the next article, I will share with you the knowledge of Vue components and their life cycle