Previous remarks
If you only use Vue's most basic declarative rendering capabilities, you can use Vue as a template engine.This article details the Vue template content
Summary
Vue.js uses HTML-based template syntax, which allows DOM to be declaratively bound to the data of the underlying Vue instance.All Vue.js templates are legitimate HTML, so they can be parsed by standard-compliant browsers and HTML parsers
On the underlying implementation, Vue compiles the template into a virtual DOM rendering function.Combined with response systems, Vue can intelligently calculate the minimum cost of re-rendering components and apply them to DOM operations when applying state changes
In general, template content includes text content and element attributes
Text Rendering
[Text Interpolation]
The most common form of text rendering is to use double brace syntax for text interpolation. The message below is equivalent to a variable or placeholder and will eventually be represented as real text content
<div id="app"> {{ message }} </div>
<script> new Vue({ el: '#app', data:{ 'message': '<span>Test Content</span>' } }) </script>
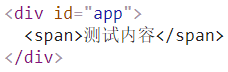
[Expression Interpolation]
{{ number + 1 }} {{ ok ? 'YES' : 'NO' }} {{ message.split('').reverse().join('') }}
These expressions are parsed as JS under the data scope of the Vue instance to which they belong.The limitation is that each binding can only contain a single expression, so the following example will not work
Template expressions are sandboxed and can only access a whitelist of global variables, such as Math and Date.User-defined global variables should not be attempted in template expressions
<!-- This is a statement, not an expression --> {{ var a = 1 }} <!-- Flow control will not work either, please use a ternary expression --> {{ if (ok) { return message } }}
[Note] About the differences between expressions and statements Move Here
<div id="app"> {{ num + 1 }} </div>
<script> new Vue({ el: '#app', data:{ 'num': -1 } }) </script>
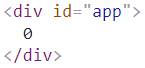
[v-text]
Another way to achieve interpolation-like effects is to use the v-text directive, which updates an element's innerText.If you want to update part of innerText, you need to use template interpolation
[Note] v-text priority is higher than template interpolation priority
<div id="app" v-text="message"> </div>
<script> new Vue({ el: '#app', data:{ message:"This is a <i>simple</i> document" } }) </script>

[v-html]
If you want to output true HTML, you need to use the v-html directive, which updates the element's innerHTML
[Note] Dynamically rendering any HTML on a website is dangerous because it can easily lead to XSS attacks.Use v-html only on trusted content, not on user-submitted content
<div id="app" v-html="message"> </div>
<script> new Vue({ el: '#app', data:{ message:"This is a <i>simple</i> document" } }) </script>

Static Interpolation
Template interpolation is described above. Generally, template interpolation is dynamic.That is, whenever the placeholder content on the bound data object changes, the content at the interpolation is updated
<div id="app"> {{ message }}</div>
<script> var vm = new Vue({ el: '#app', data:{ 'message': 'Test Content' } }) </script>
As shown in the following figure, the contents of vm.message have changed and the elements in the DOM structure have been updated accordingly
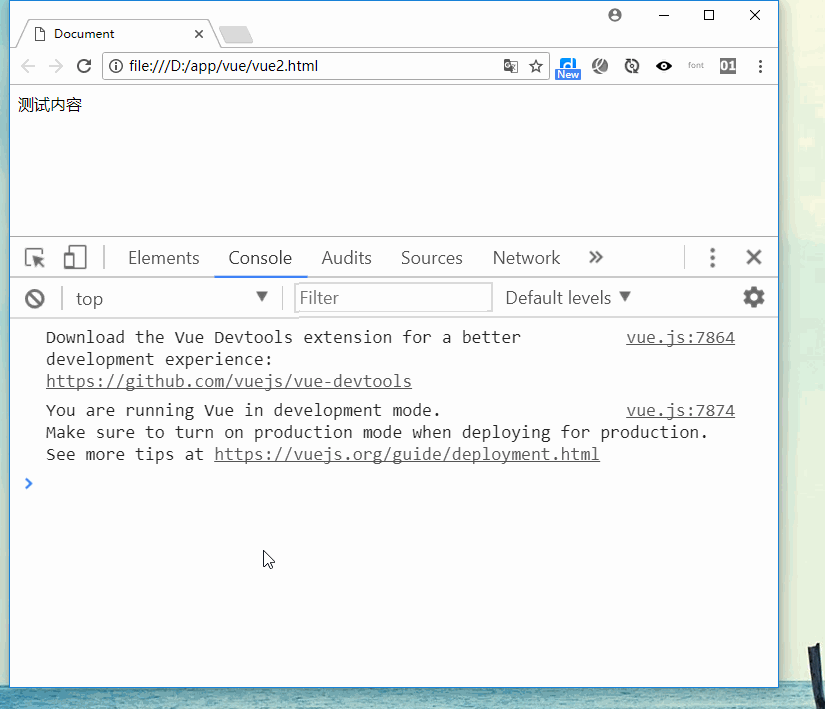
[Static Interpolation]
If static interpolation is to be implemented, i.e., one-off interpolation, the contents of the interpolation will not be updated when the data changes, requiring the v-once instruction
<div id="app" v-once>{{ message }}</div>
<script> var vm = new Vue({ el: '#app', data:{ 'message': 'Test Content' } }) </script>
As shown in the figure below, when vm.message is changed to 123, the element content in the DOM structure is still "test content"
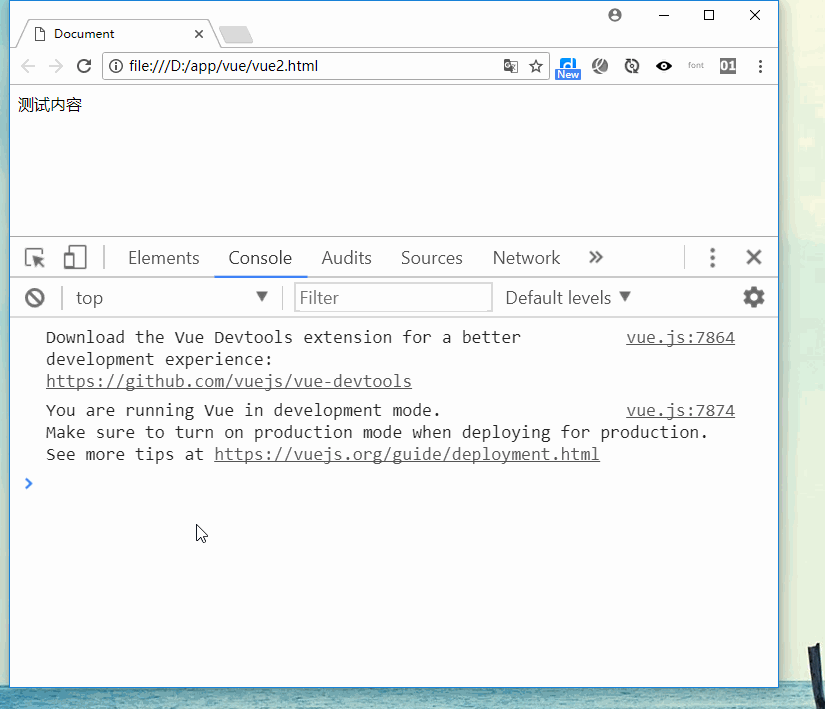
Feature Rendering
HTML There are 16 Global Properties Vue.js supports dynamic rendering of the content of a feature (or feature)
[Note] Differences between object properties and element attributes Move Here
You cannot use double brace syntax when rendering attributes
<div id="app" title={{my-title}}></div>
<script> var vm = new Vue({ el: '#app', data:{ 'my-title': 'Test Content' } }) </script>
When using the above code, the console will display the following errors

[v-bind]
As mentioned in the error prompt above, the v-bind directive should be used to dynamically bind one or more attributes
The title here is a parameter that tells the v-bind directive to bind the title attribute of the element to the value of the expression message
<div id="app" v-bind:title="message"></div>
Since the v-bind directive is very common, it can be abbreviated as follows
<div id="app" :title="message"></div>
<script> new Vue({ el: '#app', data:{ message:"I'm a small match" } }) </script>

The Boolean property is also valid -- if the condition evaluates to false, the property is removed
<button id="app" :disabled="isButtonDisabled">Button</button>
<script> var vm = new Vue({ el: '#app', data:{ 'isButtonDisabled': true } }) </script>
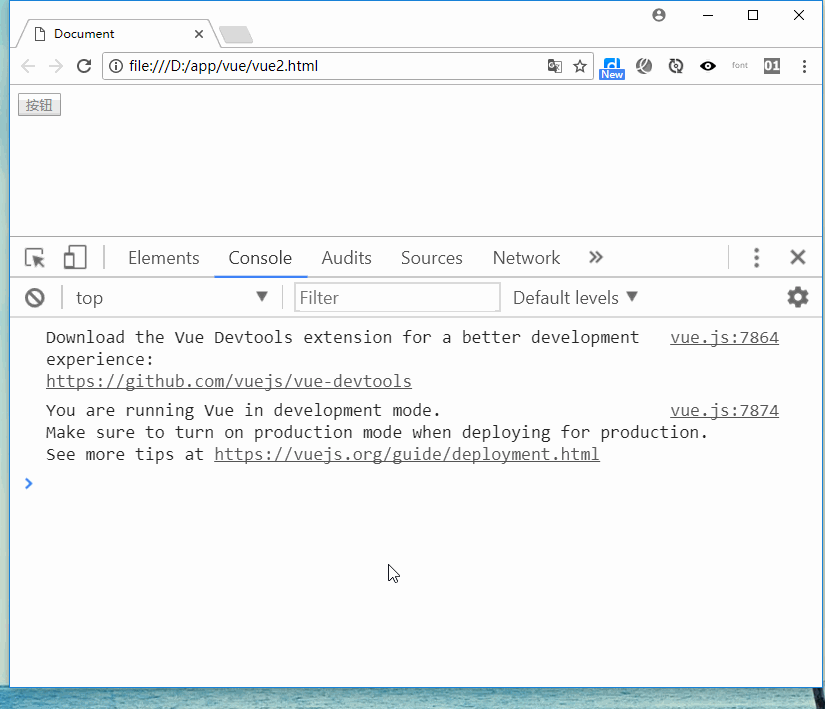
class binding
A common requirement for data binding is the class list of operation elements and its inline style.Because they are attributes, they can be handled with v-bind: you only need to calculate the final string of the expression.However, string splicing is cumbersome and error-prone.Therefore, when v-bind is used for classes and styles, Vue.js specifically enhances it.The result type of an expression can be an object or an array in addition to a string
Binding class es include object syntax, array syntax, and component binding
[Object Grammar]
Can be passed to a v-bind:class object to dynamically switch classes
<div v-bind:class="{ active: isActive }"></div>
The syntax above indicates that updates to class active will depend on whether the data attribute isActive is true or not.
More attributes can be passed in the object to dynamically switch multiple classes.v-bind:class directives can coexist with common class attributes
<div id="app" class="static" v-bind:class="{ active: isActive, 'text-danger': hasError }"> </div>
<script> var app = new Vue({ el: '#app', data:{ isActive:true, hasError:false } }) </script>

When isActive or hasError changes, the class list updates accordingly.For example, if hasError has a value of true, the class list becomes "static active text-danger"
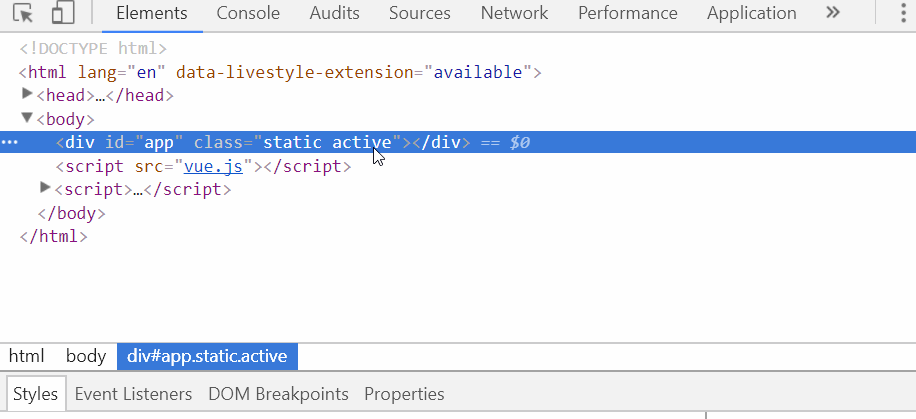
Or you can directly bind an object in your data
<div id="app" :class="classObject"></div>
<script> var app = new Vue({ el: '#app', data:{ classObject: { active: true, 'text-danger': false } } }) </script>

You can also bind the calculated properties of the returned object here.This is a common and powerful pattern
<div id="app" :class="classObject"></div>
<script> var app = new Vue({ el: '#app', data: { isActive: true, error: null }, computed: { classObject: function () { return { active: this.isActive && !this.error, 'text-danger': this.error && this.error.type === 'fatal', } } } }) </script>

[Array Grammar]
You can pass an array to v-bind:class to apply a class list
<div id="app" :class="[activeClass, errorClass]"></div>
<script> var app = new Vue({ el: '#app', data: { activeClass: 'active', errorClass: 'text-danger' } }) </script>

If you want to switch class es in the list according to a condition, you can use a ternary expression
<div id="app" :class="[isActive ? activeClass : '', errorClass]"></div>
This example always adds errorClass, but only if isActive is true
This is tedious when there are multiple conditional class es.You can use object syntax in array syntax
<div id="app" :class="[{ active: isActive }, errorClass]"></div>
[Component Binding]
When class attributes are used on a custom component, these classes are added to the root element, and classes that already exist on this element are not overwritten
<div id="app" class="test"> <my-component class="baz boo"></my-component> </div>
<script> Vue.component('my-component', { template: '<p class="foo bar">Hi</p>' }) var app = new Vue({ el: '#app' }) </script>
The HTML will eventually be rendered as follows

The same applies to binding HTML class es
<div id="app" class="test"> <my-component :class="{ active: isActive }"></my-component> </div>
<script> Vue.component('my-component', { template: '<p class="foo bar">Hi</p>' }) var app = new Vue({ el: '#app', data:{ isActive:true } }) </script>
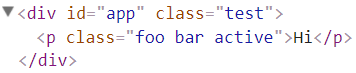
style binding
[Object Grammar]
The object syntax of v-bind:style is intuitive -- it looks very like CSS, but it's actually a JS object.CSS attribute names can be named as camelCase or kebab-case separated by short dashes (in quotation marks)
<div id="app" :style="{ color: activeColor, fontSize: fontSize + 'px' }"></div>
<script> var app = new Vue({ el: '#app', data: { activeColor: 'red', fontSize: 30 } }) </script>

It is usually better to bind directly to a style object to make the template clearer
<div id="app" :style="styleObject"></div>
<script> var app = new Vue({ el: '#app', data: { styleObject: { color: 'red', fontSize: '13px' } } }) </script>
[Array Grammar]
Array syntax for v-bind:style can apply multiple style objects to an element
<div id="app" :style="[baseStyles, overridingStyles]"></div>
<script> var app = new Vue({ el: '#app', data: { baseStyles: { color: 'red', fontSize: '13px' }, overridingStyles:{ height:'100px', width:'100px' } } }) </script>

[prefix]
When v-bind:style uses CSS attributes that require a specific prefix, such as transform, Vue.js automatically detects and adds the appropriate prefix
You can provide an array of multiple values for attributes in a style binding, often used to provide multiple prefixed values
<div :style="{ display: ['-webkit-box', '-ms-flexbox', 'flex'] }">
This renders the last browser-supported value in the array.In this example, if the browser supports flexbox without a browser prefix, the rendering result will be display: flex
Filter
Vue.js allows for custom filters and can be used as some common text formatting.Filters can be used in two places: template interpolation and v-bind expressions.The filter should be added at the end of the JS expression, indicated by the "pipe" symbol
{{ message | capitalize }} <div v-bind:id="rawId | formatId"></div>
Filters are designed for text conversion.For more complex data transformations in other instructions, you should use Computing attributes
The filter function always accepts the value of the expression (the result of the previous chain of operations) as the first parameter.In this example, the capitalize filter function will receive the value of message as the first parameter
<div id="app"> {{ message}} {{ message | capitalize }} </div>
<script> var app = new Vue({ el: '#app', data:{ message: 'Small matches' }, filters: { capitalize: function (value) { if (!value) return '' value = value.toString() return value.split('').reverse().join('') } } }) </script>
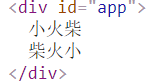
Filters can be in series
{{ message | filterA | filterB }}
In this example, filterA has a single parameter, it receives the value of the message, then calls filterB, and the processing result of filterA is passed in as a single parameter of filterB
<div id="app"> {{ message}} {{ message | filterA | filterB }} </div>
<script> var app = new Vue({ el: '#app', data:{ message: 'Small matches' }, filters: { filterA: function (value) { return value.split('').reverse().join('') }, filterB: function(value){ return value.length } } }) </script>
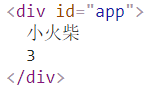
The filter is a JS function and therefore can accept parameters
{{ message | filterA('arg1', arg2) }}
Here, filterA is a function with three parameters.The value of message will be passed in as the first parameter.The string'arg1'is passed to filterA as the second parameter and the value of the expression arg2 as the third parameter
<div id="app"> {{ message}} {{ message | filterA('arg1', arg) }} </div>
<script> var app = new Vue({ el: '#app', data:{ message: 'Small matches', arg: 'abc' }, filters: { filterA: function (value,arg1,arg2) { return value + arg1 + arg2 } } }) </script>
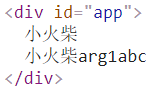
The following is an example of the use of filters in v-bind expressions
<div id="app" :class="raw | format"></div>
<script> var app = new Vue({ el: '#app', data:{ raw: 'active' }, filters: { format: function (value) { return value.split('').reverse().join('') } } }) </script>
