Part I knowledge review:
- What are Vue components?
- What stages will Vue components go through from creation to destruction?
- How do Vue components share data?
Summary of this article
- How to use dynamic components?
- How to use slots to reserve custom content for users?
- Use of Vue router in SPA project under Vue framework
1, Dynamic component
1. What is a dynamic component
Dynamic component refers to dynamically switching the display and hiding of components.
2. Use of dynamic components
Example:
<button @click="comName = 'Left'">Exhibition Left</button> <button @click="comName = 'Right'">Exhibition Right</button> <div class="box"> <!-- Render Left Components and Right assembly --> <keep-alive include="Right,Left"> <component :is="comName"></component> </keep-alive> </div> import Left from '@/components/Left.vue' import Right from '@/components/Right.vue' export default { components: { // If you do not specify a name for the component when "declaring the component", the name of the component is "the name at the time of registration" by default Left, Right }, data() { return { // comName indicates the name of the component to be displayed comName: 'Left' } } }
(1) < component > label
vue's built-in label only serves as a placeholder for components
(2) is attribute
The value of the is attribute indicates the < component > location and the name of the component to be rendered
Note: the component name should be the registered name of the component under the components node
(3) < keep alive > tag
When component a displayed by < component > is hidden and component B is to be displayed, component A is usually destroyed directly. Recreate component a the next time you show it. Frequent switching of components a and B will cause certain losses.
At this time, you can use the keep alive tag and use its include and exclude attributes to cache the specified components, which can be used later without re creation.
-The tag caches the component specified by the include attribute instead of destroying it directly
When a component is cached, the deactivated lifecycle function of the component is automatically triggered.
-You can also specify which components do not need to be cached through the exclude attribute;
When a component is activated, the activated lifecycle function of the component is automatically triggered
Note: do not use both include and exclude attributes at the same time
2, Slot
1. What is a slot
A Slot is the ability vue provides for the packager of a component. It allows developers to define uncertain parts that want to be specified by users as slots when packaging components.
The slot can be regarded as a placeholder for the content reserved for users during component packaging. An example is as follows
<!-- Left assembly --> <div class="left-container"> <h3>Left assembly</h3> <!-- Declare a slot area --> <!-- vue Official regulations: every slot Slots, there must be one name name --> <!-- If omitted slot of name Property, there is a default name called default --> <slot> <!-- When the user does not specify the content for the slot, the backup content of the slot will be displayed --> <h6>This is default Backup content of slot</h6> </slot> </div>
The slot to which a name is assigned is called a named slot
On app The Left component is invoked in Vue and the contents are specified for the slot.
<div class="box" style="display: none;"> <!-- Render Left assembly --> <Left> <template #default> <p>This is in Left Content area of the component, declared p label</p> </template> </Left> </div>
By default, when using a component, the content provided will be filled into the slot named default in the component by default
If you want to fill the slot with the specified name, you need to use the v-slot: instruction, which is abbreviated as#
be careful:
1. v-slot: follow the slot name
2. v-slot: the instruction cannot be directly used on the element, but must be used on the template label
3. The tag template is a virtual tag, which only plays the role of wrapping. However, it will not be rendered as any substantive html element
When there are multiple slots in the component to be used, fill in # slot name in each template tag attribute, and Vue framework can determine which slot the content in the template is placed in.
2. Scope slot
In the process of packaging components, props data can be bound for reserved < slot > slots
This < slot > with props data is called "scope slot"
The example code is as follows
<h3 v-color="'red'">Article assembly</h3> <!-- Content of the article --> <div class="content-box"> <!-- It is reserved when packaging components <slot> Provide the value corresponding to the attribute --> <slot name="content" msg="hello vue.js" :user="userinfo"></slot> </div>
How to get the bound props value in the parent component? Examples are as follows:
<Article> <template #content="scope"> <p>{{ scope.msg }}</p> <p>{{ scope.user }}</p> </template> <!-- You can also directly obtain attributes by deconstruction #content="{{ msg, user }}" --> </Article>
3, Routing
1. What is routing
In front-end projects, routing is the corresponding relationship, that is, the corresponding relationship between Hash address and components
2. Working principle
① The user clicks the route link on the page ② causes the Hash value in the URL address bar to change ③ the front-end route monitors the change of the Hash address ④ the front-end route renders the components corresponding to the current Hash address to the specified position of the current page
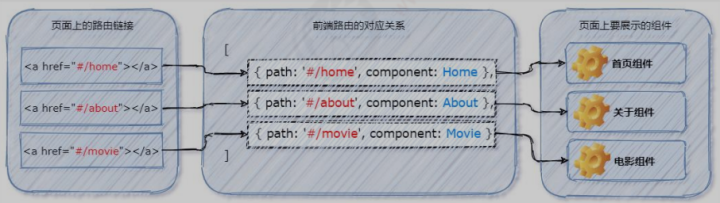
The routing function in vue is actually realized by dynamic components at the bottom, but the framework has been encapsulated for us to use directly
3. Single page application (SPA) and front-end routing
SPA means that a web site has only one HTML page, and the display and switching of all components are completed in this unique page.
At this time, the switching between different components needs to be realized through the front-end routing.
Therefore, in the SPA project, the switching between different functions depends on the front-end routing
4. vue-router
1. Concept:
vue router is vue JS official routing solution. It can only be used in combination with vue project, and can easily manage the switching of components in SPA project.
2. Steps of Vue router installation and configuration
① Install Vue router package
npm i vue-router@3.5.2 -S
② In the src source code directory, create a router / index JS routing module and initialize the following code:
import Vue from 'vue' import VueRouter from 'vue-router' // Import required components // Install vueroter as a plug-in for Vue projects Vue.use(VueRouter) // Create an instance object for routing const router = new VueRouter({ }) export default router
③ In Src / main JS entry file, import and mount the routing module.

④ In Src / APP In the Vue component, route links and placeholders are declared using < router link > and < router View > provided by Vue router

3. Declare matching rules for routes
Common usage:
(1) Route redirection
When accessing address A, the user is forced to jump to address C to display A specific component page. By specifying A new routing address through the redirect attribute of the routing rule, you can easily set the redirection of the route
// Create an instance object for routing const router = new VueRouter({ // routes is an array that defines the correspondence between "hash address" and "component" routes: [ // Redirected routing rules { path: '/', redirect: '/home' }, // Routing rules { path: '/home', component: Home }, })
(2) Dynamic route matching
The variable part of the Hash address is defined as a parameter item, so as to improve the reusability of routing rules. In Vue router, the English colon: is used to define the parameter items of the route. The example code is as follows:
// Requirement: you want to display the detailed information of the corresponding movie according to the id value // You can enable props parameter transmission for routing rules, so as to easily get the value of dynamic parameters { path: '/movie/:mid', component: Movie, props: true },
You can also use this in a component$ route. params. Mid gets the value, but props is easier to use
(3) Nested routing
Usage scenario:
Click the parent routing link to display the corresponding component content ① there are child routing links in the component content ② click the child routing link to display the component content corresponding to the child

import Vue from 'vue' import VueRouter from 'vue-router' // Import required components import Home from '@/components/Home.vue' import Movie from '@/components/Movie.vue' import About from '@/components/About.vue' import Tab1 from '@/components/tabs/Tab1.vue' import Tab2 from '@/components/tabs/Tab2.vue' Vue.use(VueRouter) // Create an instance object for routing const router = new VueRouter({ // routes is an array that defines the correspondence between "hash address" and "component" routes: [ { path: '/about', component: About, children: [ // Sub routing rule // Default sub route: if the path value of a routing rule in the children array is an empty string, this routing rule is called "default sub route" { path: '', component: Tab1 }, { path: 'tab2', component: Tab2 } ] }, }) export default router
4. Programming navigation API
The way to navigate by clicking on links is called declarative navigation. For example:
⚫ Clicking < a > link in ordinary web pages and < router link > in vue projects are declarative navigation
The way to call API methods to realize navigation is called programmatic navigation. For example:
⚫ Calling location. in ordinary web pages Href the way to jump to a new page is programmatic navigation
Vue router also provides AIP for programmed navigation
① this.$router.push('hash address')
⚫ Jump to the specified hash address and add a history
this.$router.push('/home')
② this.$router.replace('hash address')
⚫ Jump to the specified hash address and replace the current history
this.$router.replace('/home')
③ this.$ router. Go (value n)
⚫ Realize navigation history forward and backward
The following API can be used to simplify
$router.back() in the history, go back to the previous page $router Forward() advances to the next page in the history
5. Navigation guard
The navigation guard can control the access permission of the route and play the role of interception
For example, judge whether there is a token in the local storage. If it does not exist, it means that the user has not logged in, and force the user to jump to the login page
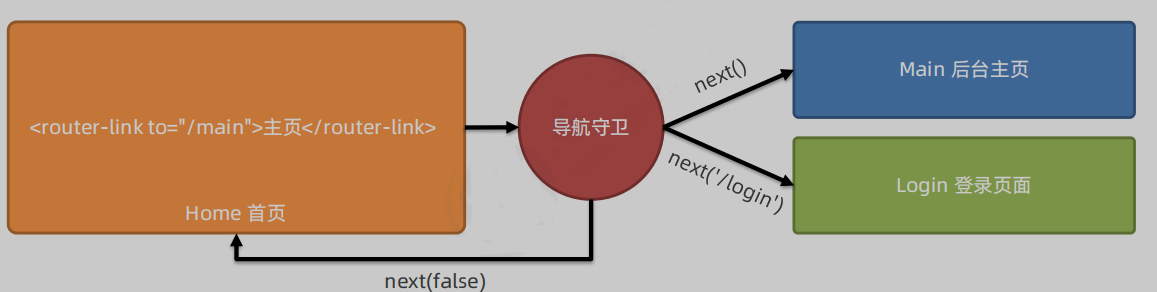
In Src / router / index JS file, write the following code
// Declare the global pre navigation guard for the router instance object // As long as a route jump occurs, the function callback function specified by beforeEach will be triggered router.beforeEach(function(to, from, next) { // To represents the information object of the route to be accessed // from indicates the information object of the route to leave // The next() function means release if (to.path === '/main') { // To access the background home page, you need to judge whether there is a token const token = localStorage.getItem('token') if (token) { next() } else { // No login, forced jump to login page next('/login') } } else { next() //Otherwise release } })
summary
Front end routing is an important dependence of Vue framework to realize SPA project. Although there are many knowledge points, only those methods are frequently used in actual development. The navigation guard can realize the control of access rights. Vue router can be said to be very comprehensive.
Making good use of Vue slots can sometimes bring great convenience to development, such as:
In this case, middleware A and B can only share data by using middleware A and B. in this case, component A and B can only share data.
However, if a slot is placed in component B, when component a references component B on the page, it only needs to put component C into the slot from component B in the label of component B. at this time, component A and component C also become a parent-child relationship. By passing values in the label properties of component C, it simplifies a large part of the code compared with using EventBus.