Vue3 component usage - routing (Part 2)
1, Use of components
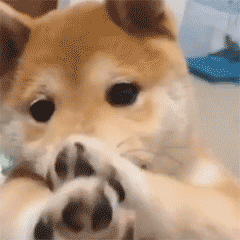
1. Reference component
1. Create a new component named header vue
Header.vue
<template> <h1>This is the head assembly</h1> </template>
2. On app Reference in Vue
Run it
2. Pass the contents of the parent component to the child component
Transfer variable
3. Transfer the contents of the child component to the parent component
2, Declare periodic function
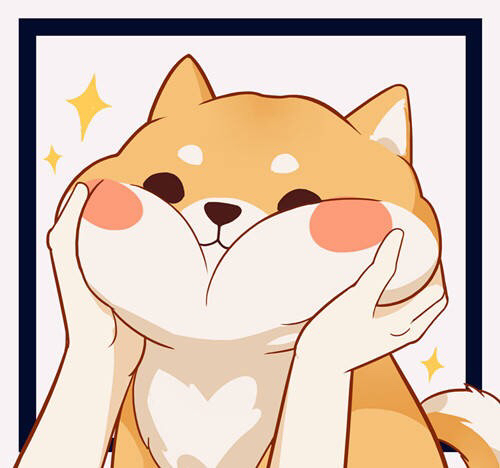
<template> <div class="content"> </div> </template> <script> //Declarative export default { name: 'App', data(){ return{ } } , beforeCreate(){ console.log("Before initializing data") }, created(){ console.log("After data initialization") }, beforeMount(){ console.log("Before mounting rendering") }, mounted(){ console.log("After mounting rendering") }, beforeUpdate(){ console.log("Before update") }, updated(){ console.log("After update") }, beforeUnmount(){ console.log("Before destruction") }, unmount(){ console.log("After destruction") } } </script>
3, Synthetic API
1. Initial experience of synthetic API
setup()
This is the entrance to using composition API;
Two parameters can be accepted: setup(props, context)
props: accept the parameters passed from the parent component;
Context: (execution context)
Using ref to declare functions is mutable
You must return from the setup to use the function
<template> <div class="content"> <h1 @click="changeNum">{{num}}</h1> <h2>full name:{{user.username}}</h2> <h2>Age:{{user.age}}</h2> <h1>{{username}}</h1> <h3>{{reverseType}}</h3> </div> </template> <script> //Declarative import {ref,reactive,toRefs,computed,watch,watchEffect} from 'vue' //Import ref reactive toref function export default { name: 'App', setup(){ //numerical value //Make a value or string responsive const num = ref(0); //object //Make an object responsive const user = reactive({ username : "cocoa", age:18, type:"Love movement", reverseType:computed(()=>{ return user.type.split('').reverse().join('') }) }) //method function changeNum(){ num.value += 10; } function changeType(){ user.type = "Super active" } watchEffect(()=>{ //The function that will determine the content to listen to console.log(user.type) console.log("user.type change") }) watch([num,user],(newnum,prevNum)=>{ console.log(newnum,prevNum); console.log("When num When changing, the execution function will be triggered") }) //Return variable method return {num,changeNum,user,...toRefs(user),changeType} } </script>
2. Detailed explanation of synthetic api
<template> <div class="content"> <user :username="username" :age="age"/> </div> </template> <script> //Declarative import user from './components/user.vue' import {ref,reactive,toRefs,computed,watch,watchEffect} from 'vue' //Import ref reactive toref function export default { name: 'App', components:{ user }, setup(){ //numerical value //Make a value or string responsive const num = ref(0); //object //Make an object responsive const user = reactive({ username : "cocoa", age:18, type:"Love movement", reverseType:computed(()=>{ return user.type.split('').reverse().join('') }) }) //method function changeNum(){ num.value += 10; } function changeType(){ user.type = "Super active" } watchEffect(()=>{ //The function that will determine the content to listen to console.log(user.type) console.log("user.type change") }) watch([num,user],(newnum,prevNum)=>{ console.log(newnum,prevNum); console.log("When num When changing, the execution function will be triggered") }) //Return variable method return {num,changeNum,user,...toRefs(user),changeType} } </script>
user.vue
<template> <div> <!-- Parameters passed from parent component to child component --> <h1>username:{{username}}</h1> <h1>age:{{age}}</h1> <h3>{{description}}</h3> </div> </template> <script> import {ref,reactive,toRefs,computed,watch,watchEffect} from 'vue' export default { props:['age','username'], //content can obtain the data passed from the parent element, such as the value of class attribute setup(props,content){ console.log(props); //Since setup is executed earlier and the this pointer has not been loaded, you cannot use this to pass values here const description = ref(props.username+"Age is"+props.age); return { description } } } </script>
3. Using lifecycle functions in setup
user.vue
<template> <div> <!-- Parameters passed from parent component to child component --> <h1>username:{{username}}</h1> <h1>age:{{age}}</h1> <h3>{{description}}</h3> </div> </template> <script> import {ref,reactive,toRefs,computed,watch,watchEffect,onBeforeMount,onMounted,onBeforeUpdate,onBeforeUnmount,onUnmounted} from 'vue' export default { props:['age','username'], //content can obtain the data passed from the parent element, such as the value of class attribute setup(props,content){ console.log(props); //Since setup is executed earlier and the this pointer has not been loaded, you cannot use this to pass values here const description = ref(props.username+"Age is"+props.age); //It can be triggered multiple times. If the life cycle is required, import them in, and add an on in front onBeforeMount(()=>{ console.log("onBeforeMount") }) onBeforeMount(()=>{ console.log("onBeforeMount") }) return { description } } } </script>
4. Use provide to pass values from parent components to child components
<template> <div class="content"> <student/> </div> </template> <script> //Declarative import student from './components/student.vue' import {ref,reactive,toRefs,computed,watch,watchEffect,provide} from 'vue' //Import ref reactive toref function export default { name: 'App', components:{ student }, setup(){ const student = reactive({ name:"Xiao Ke", classname:"Class two of three years" }) //Provided name, provided content provide('student',student); return {} } } </script>
student.vue
<template> <div> <h1>student:{{name}}</h1> <h1>Age:{{classname}}</h1> </div> </template> <script> import {ref,reactive,toRefs,computed,watch,watchEffect,provide,inject} from 'vue' //To obtain the provided content, you need to inject the function inject export default { setup(props,content){ const student = inject('student') return{ ...student } } } </script>
4, Routing
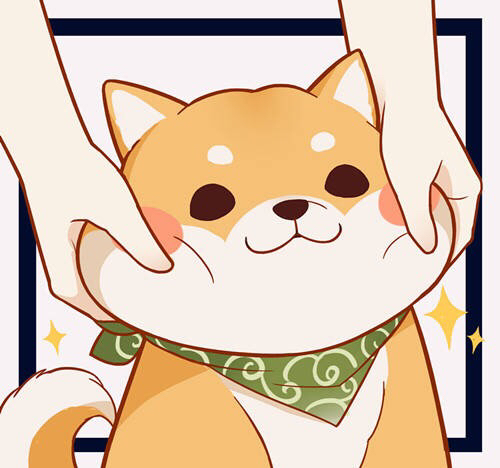
1. Basic concept of routing
There are three basic concepts in routing: route, routes and router
// Route is a route, which is a mapping relationship between path and component { path:'/', component: Home }
//routes It's a set of routes that put each route Routes are combined to form an array. routes:[ { path:'/', component: Home }, { path:'/list', component: List } ]
/*router It is a routing mechanism, equivalent to a manager, He manages the routing. Because routes only defines a group of routes, That is, the corresponding relationship between a group of paths and components. When the user clicks Button, change a path, and the router will handle different components */ var router = new VueRouter({ // Configure routing routes:[...] })
2. Install routing
Input in terminal
npm install vue-router@next
Vue3 routing usage official website
Index. Under router js
// 1. Define routing components // You can also import from other files const Home = { template: '<div>Home</div>' } const About = { template: '<div>About</div>' } // 2. Define some routes // Each route needs to be mapped to a component. // We will discuss nested routing later. const routes = [ { path: '/', component: Home }, { path: '/about', component: About }, ] // 3. Create a routing instance and pass the 'routes' configuration // You can enter more configurations here, but we are here // Keep it simple for the time being const router = VueRouter.createRouter({ // 4. The implementation of history mode is provided internally. For simplicity, we use the hash pattern here. history: VueRouter.createWebHashHistory(), routes, // `Routes: abbreviation for routes' })
main.js
Run it
npm run dev
Router link component description:
/* router-link It is a registered component of Vue router. You can use it directly router-link It is used to switch routes and jump to the specified route through the to attribute router-link When compiling, it will be automatically compiled into a tag. You can use the tag attribute to specify the tag you want to compile */
Router view component description:
/* router-view Used to specify the location where the components mapped by the current route are displayed router-view It can be understood as a placeholder for the components of route mapping. The components of different route mappings are displayed through replacement The effect is similar to that of dynamic components */
3. Dynamic routing and 404NotFound
Introduction: when different users log in to an interface, we will find that some of the URLs are the same, but the personal id values involved are different. This means that it is a component, assuming the user component. Different users (i.e. different user IDs) will navigate to the same user component. In this way, we can't write dead when configuring routing, so we introduce dynamic routing.
path:'/students/:id',component:students
ps:
visit`/students/101`and`/students/102`The same route can be matched at all times,Take the same route processing function, We have to define/students/:id Routing
Index. Under router js
import {createRouter,createWebHashHistory} from 'vue-router' import News from '../src/components/News.vue' import Notfound from '../src/components/Notfound.vue' const Home = { template: '<div>Home</div>' } const About = { template: '<div>About</div>' } const routes = [ { path: '/', component: Home }, { path: '/about', component: About }, { path:'/News/:id',component:News}, {path:'/:path(.*)',component:Notfound //Set pathname, component name } ] const router = createRouter({ history: createWebHashHistory(), routes, }) export default router;
Notfound.vue
<template> <div> <p>404 not found page</p> </div> </template>
import {createRouter,createWebHashHistory} from 'vue-router' import News from '../src/components/News.vue' import Notfound from '../src/components/Notfound.vue' const Home = { template: '<div>Home</div>' } const About = { template: '<div>About</div>' } const routes = [ { path: '/', component: Home }, { path: '/about', component: About }, { path:'/News/:id',component:News}, { path:'/News/:id(//d+)',component:News}, { path:'/News/:id+',component:News},/* +At least one· ?Yes or no, can not be repeated *Yes or no*/ { path:'/News/:id*',component:News}, {path:'/:path(.*)',component:Notfound} ] const router = createRouter({ history: createWebHashHistory(), routes, }) export default router;
4. Nested routing
Index. Under router js
import { createRouter, createWebHashHistory } from "vue-router"; import User from "../src/components/User.vue"; import hengban from "../src/components/hengban.vue"; import shuban from "../src/components/shuban.vue"; const Home = { template: "<div>Home</div>" }; const About = { template: "<div>About</div>" }; const routes = [ { path: "/", component: Home }, { path: "/about", component: About }, { path: "/user", component: User, children:[ { path:'hengban', component:hengban },{ path:'shuban', component:shuban } ] } ]; const router = createRouter({ history: createWebHashHistory(), routes }); export default router;
Using req params. ID to get the data of different parts of the route
Index. Under router js
import { createRouter, createWebHashHistory } from "vue-router"; import User from "../src/components/User.vue"; import hengban from "../src/components/hengban.vue"; import shuban from "../src/components/shuban.vue"; const Home = { template: "<div>Home</div>" }; const About = { template: "<div>About</div>" }; const routes = [ { path: "/", component: Home }, { path: "/about", component: About }, { path: "/user/:id", component: User} ]; const router = createRouter({ history: createWebHashHistory(), routes }); export default router;
user.vue
<template> <div> <h1>User page</h1> <h2>{{$route.params.id}}</h2> <router-view></router-view> </div> </template>
5. Use js to jump to the page
$router.push()
page.vue
<template> <div> <button @click="toindexpage">Jump to home page</button> </div> </template> <script> export default { methods:{ toindexpage(){ console.log("Jump to about page") this.$router.push({path:'/about'}) //this.$router.push({path:'/mypage/123'}) //Carry parameters //this.$router.push({name:"mypage",params:{id:132}}) } } } </script>
Index. Under router js
import { createRouter, createWebHashHistory } from "vue-router"; import User from "../src/components/User.vue"; import page from "../src/components/page.vue"; const Home = { template: "<div>Home</div>" }; const About = { template: "<div>About</div>" }; const routes = [ { path: "/", component: Home }, { path: "/about", component: About }, {path:'/page/:id', name:'mypage',//alias component:page} ]; const router = createRouter({ history: createWebHashHistory(), routes }); export default router;
You can also jump with parameters
$router.replace()
this.$router.replace({path:'/page',query:{search:"cocoa"}})
$router.go(-1) returns to the previous page
$router.go(-1)
$router.go(1) go to the next page
$router.go(1)
code
<template> <div> <button @click="toindexpage">Jump to home page</button> <button @click="replacePage">Replace page</button> <button @click="$router.go(1)">forward</button> <button @click="$router.go(-1)">back off</button> </div> </template> <script> export default { methods:{ toindexpage(){ console.log("Jump to about page") this.$router.push({path:'/mypage'}) }, replacePage(){ console.log("Replace page") //Can't go back to the previous page this.$router.replace({path:'/page',query:{search:"cocoa"}}) } } } </script>
6. Named view
You can set up multiple components for rendering in one page
import { createRouter, createWebHashHistory } from "vue-router"; import shop from '../src/components/shop.vue' import shopfoot from '../src/components/shopfoot.vue' import shopTop from '../src/components/shopTop.vue' const Home = { template: "<div>Home</div>" }; const About = { template: "<div>About</div>" }; const routes = [ { path: "/", component: Home }, { path: "/about", component: About }, {path:'/shop', components:{ default:shop,//The default page is shop shopfoot:shopfoot, shopTop:shopTop } } ]; const router = createRouter({ history: createWebHashHistory(), routes }); export default router;
<template> <div class="content"> <router-view></router-view> <router-view name="shopTop"></router-view> <router-view name="shopfoot"></router-view> <!-- If you encounter a with the same name, the content will be updated to the route --> </div> </template> <script> export default { name: "App", components: {}, setup() { return {}; } }; </script>
7. Redirection and alias
{path:'/mall', redirect:(to)=>{return{path:'/shop'}} }//Redirect to the shop page
//Alias. The name in the alias address bar will not be changed to shop, but still cocoshop. You can take multiple aliases alias:"/cocoshop",
8. Navigation guard
If you have permission, you can jump to the page
router.beforeEach((to,from)=>{ return false;//If false is returned, there is no permission and the page is not displayed })
router.beforeEach((to,from,next)=>{ console.log(to) next();//Call next() if you want to continue accessing })
import { createRouter, createWebHashHistory } from "vue-router"; import shop from '../src/components/shop.vue' import shopfoot from '../src/components/shopfoot.vue' import shopTop from '../src/components/shopTop.vue' import mypage from '../src/components/mypage.vue' const Home = { template: "<div>Home</div>" }; const About = { template: "<div>About</div>" }; const routes = [ { path: "/", component: Home }, { path: "/about", component: About }, {path:'/shop', //Alias. The name in the alias address bar will not be changed to shop, but still cocoshop. You can take multiple aliases alias:"/cocoshop", components:{ default:shop,//The default page is shop shopfoot:shopfoot, shopTop:shopTop }, //Enter a specific component page beforeEnter:(to,from)=>{ console.log("beforeEnter") //It will only be generated when you enter the page, which is often used } }, // {path:'/mall', // redirect:(to)=>{return{path:'/shop'}} // }, {path:'/mypage', component:mypage } ]; const router = createRouter({ history: createWebHashHistory(), routes }); //Execute before jump router.beforeEach((to,from,next)=>{ console.log(to) next(); //If return (false), do you have the right to jump to a page and cancel the jump //Safe navigation }) export default router;
Use options API
beforeRouteEnter(){ console.log("Route entry") }, beforeRouteUpdate(){ console.log("Route update component") }, beforeRouteLeave(){ console.log("Route away component") }
<template> <div> <h1>Shopping mall</h1> <button @click="topage"></button> </div> </template> <script> export default { methods:{ topage:function(){ this.$router.push({path:'/mypage'}) } }, beforeRouteEnter(){ console.log("Route entry") }, beforeRouteUpdate(){ console.log("Route update component") }, beforeRouteLeave(){ console.log("Route away component") } } </script>
9. Routing mode
There are two schemes for routing: one is through the hash value, and the other is through the history of H5 However, Vue routing uses hash mode by default
//hash routing # looks a little awkward It's like using / / history mode. How to set it
The vueroter configuration item provides a route to configure the route mapping table and a mode option to configure the route mode
import { createRouter, createWebHashHistory } from "vue-router"; const router = createRouter({ history: createWebHashHistory(), routes });
import { createRouter, createWebHistory } from 'vue-router' const router = createRouter({ history: createWebHistory(process.env.BASE_URL), routes })