- Intersection selector
- Union selector
- Descendant Selectors
- Child Selector
- Adjacent Sibling Selectors
- attribute selectors
Intersection selector
The intersection selector consists of two selectors directly connected. The first selector must be an element selector, and the second selector must be a class selector or ID selector. The two selectors must be written continuously without spaces.
The element selected by the intersection selector must be the element type specified by the first selector, which must contain the ID name or class name corresponding to the second selector. The style of the element selected by the intersection selector is three selector styles, that is, the first selector;
Syntax:
element selector . Class selector | #ID selector { Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; }
Syntax description: "class selector ID selector" means to use class selector or ID selector.
Example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title> Style using the intersection selector </title> <style> /* The element selector sets the border and bottom margin styles */ div { border: 5px solid red; margin-bottom:20px; } /* Sets the background color using the intersection selector */ div.txt { background:#33FFCC; } /* Set font format using class selector */ .txt { font-style:italic; } </style> </head> <body> <div> Element selector effect </div> <div class="txt"> Intersection selector effect </div> <span class="txt"> Class selector effect </p> </body> </html>
Union selector
Union selector is also called group selector or group selector. It is composed of two or more arbitrary selectors. Different selectors are separated by "," to realize the "collective declaration" of multiple selectors.
The characteristic of union selector is that the style set is effective for each selector in union selector.
The function of union selector is to extract the same styles of different selectors, and then put them in one place for one-time definition, so as to simplify the amount of CSS code.
Syntax:
Selector 1, Selector 2, Selector 3, { Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; }
Syntax description: the type of selector is arbitrary. It can be either a basic selector or a composite selector.
Example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title> Style using the union selector </title> <style> div { margin-bottom:10px; border:3px solid red; } span { font-size:26px; } p { font-style:italic; } /* Use the union selector to set the common style of the element */ span, .p1, #d1 { background:#CCC; } </style> </head> <body> <div id="d1"> This is DIV1</div> <div> This is DIV2</div> <p class="p1"> This is paragraph one </p> <p> This is paragraph two </p> <span> This is a SPAN</div> </body> </html>
Descendant Selectors
Descendant selectors, also known as include selectors, are used to select descendant elements of a specified element. Using descendant selectors can help us find the target element faster and more accurately.
Syntax:
Selector 1 selector 2 selector 3 { Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; }
Example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title> Style using the descendant selector </title> <style> #box1 .p1 {/ * descendant selector*/ background:#CCC; } #box2 p {/ * descendant selector*/ background:#CFC; } </style> </head> <body> <div id="box1"> <p class="p1"> Duan Luoyi </p> <p class="p2"> Paragraph 2 </p> </div> <div id="box2"> <p class="p1"> Duan Luosan </p> <p> Paragraph 4 </p> </div> <p class="p1"> Duan luowu </p> <p> Paragraph 6 </p> </body> </html>
Child Selector
The descendant selector can select all descendant elements of the specified type of an element. If you only want to select all child elements of an element, you need to use the child element selector.
Syntax:
Selector 1> Selector 2 { Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; }
Syntax description: ">" is called a left binder, and spaces can appear on the left and right sides. "Selector 1 > selector 2" means "select all the child elements specified by selector 2 as the element specified by selector 1"
Example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title> Application example of child element selector </title> <style> h1>span { color:red; } </style> </head> <body> <h1> This is very, very <span> important </span> And <span> crux </span> Next step.</h1> <h1> This is really very <em><span> important </span> And <span> crux </span></em> Next step.</h1> </body> </html>
Adjacent Sibling Selectors
If you need to select the element immediately after an element, and they have the same parent element, you can use the adjacent sibling selector.
Syntax:
Selector 1+ Selector 2 { Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; }
Example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title> Adjacent brother selector application example </title> <style> h1+p { color:red; font-weight:bold; margin-top:50px; } p+p{ color:blue; text-decoration:underline; } </style> </head> <body> <h1> This is a primary title </h1> <p> This is paragraph 1.</p> <p> This is paragraph 2.</p> <p> This is paragraph 3.</p> </body> </html>
attribute selectors
In CSS, we can also select elements according to their attributes and attribute values. The selector used at this time is called attribute selector. There are two main forms of using attribute selectors,
Syntax:
Property selector 1 property selector 2...{ Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; } Element selector attribute selector 1 attribute selector 2... { Attribute 1: Attribute value 1; Attribute 2: Attribute value 2; }
Syntax description: the attribute selector is written as [attribute expression], in which the attribute expression can be an attribute name or an expression such as "attribute = attribute value"
Application of attribute selector:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title> Application of attribute selector </title <style> [title] {/* Select the element with the title attribute */ color: #F6F; } a[href][title]{/* Select the a element with both href and title attributes */ font-size: 36px; } img[alt] {/* Select the img element with the alt attribute */ border: 3px #f00 solid; } p[align="center"] {/* Select the p element whose align attribute is equal to center */ color: red; font-weight: bolder; } </style> </head> <body> <h2> Apply attribute selector style:</h2> <h3 title="Helloworld">Helloworld</h3> <a title=" home page "href="#"> back to home < / a > < br / > < br / > <img src="miaov.jpg" alt="logo" /> <p align="center"> Duan Luoyi </p> <hr /> <h2> No attribute selector style applied <h3>Helloworld</h3> <a href="#"> back to home < / a > < br / > < br / > <img src="miaov.jpg"> <p align="right"> Paragraph 2 </p> </body> </html>
last
If you want to ask whether front-end development is difficult or not, I have to say a sentence often said in the computer field. This sentence is "difficult will not, will not be difficult". For people who are not familiar with the technology in a certain field, they have a sense of mystery because they do not understand it. The sense of mystery will make people feel very difficult, that is, "difficult will not"; When you learn this technology, you know what technology can do and what you can't do. It's just a matter of how long it takes to do it. It's not difficult, so it's "it's not difficult to do it".
I specially organize a set of front-end learning materials for beginners and share them for free, You can get it for free by poking here
### last If you want to ask whether front-end development is difficult or not, I have to say a sentence often said in the computer field, which is『**It won't be difficult. It won't be difficult**』,For people who are not familiar with the technology in a certain field, they have a sense of mystery because they do not understand it. The sense of mystery will make people feel very difficult, that is『Hard won't』;When you learn this technology, you know what technology can do and what you can't do. It's just a matter of how long it takes to do it. It's not difficult, so it's『Yes, it's not difficult』. **I specially organize a set of front-end learning materials for beginners and share them for free,[You can get it here for free](https://gitee.com/vip204888/web-p7)** [External chain picture transfer...(img-ughiRK52-1627009740134)] 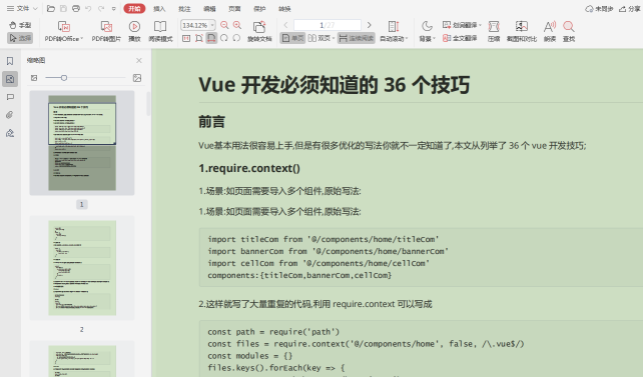