1, Write a script to log in to the remote host. (both expect and shell scripts are used).
1.expect script
[root@centos7 ~]# cat ./expects/expect.sh #!/usr/bin/expect spawn ssh 192.168.181.137 expect { "yes/no" { send "yes\n";exp_continue } "password" { send "1\n" } } interact
results of enforcement
[root@centos7 ~]# ./expects/expect.sh spawn ssh 192.168.181.137 root@192.168.181.137's password: Last login: Sun Nov 28 16:34:25 2021 [root@centos7 ~]#
2.shell script
Prepare environment
[root@centos7 expects]# yum install -y sshpass Loaded plugins: fastestmirror, langpacks Loading mirror speeds from cached hostfile file:///mnt/cdrom/repodata/repomd.xml: [Errno 14] curl#37 - "Couldn't open file /mnt/cdrom/repodata/repomd.xml" Trying other mirror. epel | 4.7 kB 00:00 Resolving Dependencies --> Running transaction check ---> Package sshpass.x86_64 0:1.06-1.el7 will be installed --> Finished Dependency Resolution Dependencies Resolved ================================================================================ Package Arch Version Repository Size ================================================================================ Installing: sshpass x86_64 1.06-1.el7 epel 21 k Transaction Summary ================================================================================ Install 1 Package Total download size: 21 k Installed size: 38 k Downloading packages: sshpass-1.06-1.el7.x86_64.rpm | 21 kB 00:00 Running transaction check Running transaction test Transaction test succeeded Running transaction Installing : sshpass-1.06-1.el7.x86_64 1/1 Verifying : sshpass-1.06-1.el7.x86_64 1/1 Installed: sshpass.x86_64 0:1.06-1.el7 Complete!
Execute script
[root@centos7 expects]# cat shell1.sh #!/bin/bash PASSWORD="1" sshpass -p "$PASSWORD" ssh root@192.168.181.137 [root@centos7 expects]# chmod 777 shell1.sh [root@centos7 expects]# ./shell1.sh Last login: Sun Nov 28 22:02:41 2021 [root@centos7 ~]#
2, Generate 10 random numbers, save them in the array, and find out their maximum and minimum values
[root@centos7 expects]# vi max_min.sh [root@centos7 expects]# cat max_min.sh #!/bin/bash declare -i min max declare -a nums for ((i=0;i<10;i++));do nums[$i]=$RANDOM [ $i -eq 0 ] && min=${nums[$i]} && max=${nums[$i]}&& continue [ ${nums[$i]} -gt $max ] && max=${nums[$i]} [ ${nums[$i]} -lt $min ] && min=${nums[$i]} done echo "Numbers are ${nums[*]}" echo Max is $max echo Min is $min [root@centos7 expects]# chmod 777 max_min.sh [root@centos7 expects]# ./max_min.sh "Numbers are 29914 21507 13798 15550 5451 8132 11577 9773 1315 17704" Max is 29914 Min is 1315 [root@centos7 expects]#
3, Input several values into the array, and use the bubbling algorithm to sort in ascending or descending order
[root@centos7 expects]# cat arry.sh read -p "Please enter the number of values:" COUNT declare -a nums for ((i=0;i<$COUNT;i++));do num[$i]=$RANDOM done echo "Original array:${num[@]}" declare -i n=$COUNT for (( i=0; i<n-1; i++ ));do for (( j=0; j<n-1-i; j++ ));do let x=$j+1 if (( ${num[$j]} < ${num[$x]} ));then #Arrange from large to small tmp=${num[$x]} num[$x]=${num[$j]} num[$j]=$tmp fi done done echo "After arrangement:${num[*]}" echo "the max integer is $num,the min integer is ${num[$((n-1))]}" [root@centos7 expects]# ./arry.sh Please enter the number of values:10 Original array:25138 26980 11797 4296 25516 25625 20062 15422 18409 25784 After arrangement:26980 25784 25625 25516 25138 20062 18409 15422 11797 4296 the max integer is 26980,the min integer is 4296 [root@centos7 expects]# vim arry.sh [root@centos7 expects]# ./arry.sh Please enter the number of values:10 Original array:10799 2623 13598 26123 4088 32235 22436 290 30159 32077 After arrangement:32235 32077 30159 26123 22436 13598 10799 4088 2623 290 the max integer is 32235,the min integer is 290 [root@centos7 expects]#
4, Summarize several commands for viewing the system load, and summarize the general meaning of the indicators of the top command (it is not required to write them all)
uptime: view the average system load
mpstat: percentage shows various indicators of CPU utilization
top and htop: view the real-time status of the process
free: view the usage status of memory space
pmap: view the memory map corresponding to the process. You can see the amount of memory occupied by the sub modules that the process depends on, which can be used to judge the OOM
vmstat: view the information of virtual memory, continuously refresh the status at user-defined intervals, and see the IO between memory, SWAP and disk;
iostat: you can see more IO performance status. You can customize the refresh interval to determine which hard disk has busy IO- With the x parameter, you can see the disk sector based IO, queue length, processing time, etc
iotop: monitor disk I/O in top mode, monitor in real time, and only display the reading and writing process, and provide many non interactive parameters;
iftop: display the usage of network bandwidth, view the real-time information of traffic accessing the current host, real-time connection, etc;
nload: you can only view the real-time throughput in the unit of interface. You can't see the connection information, but only the rate information;
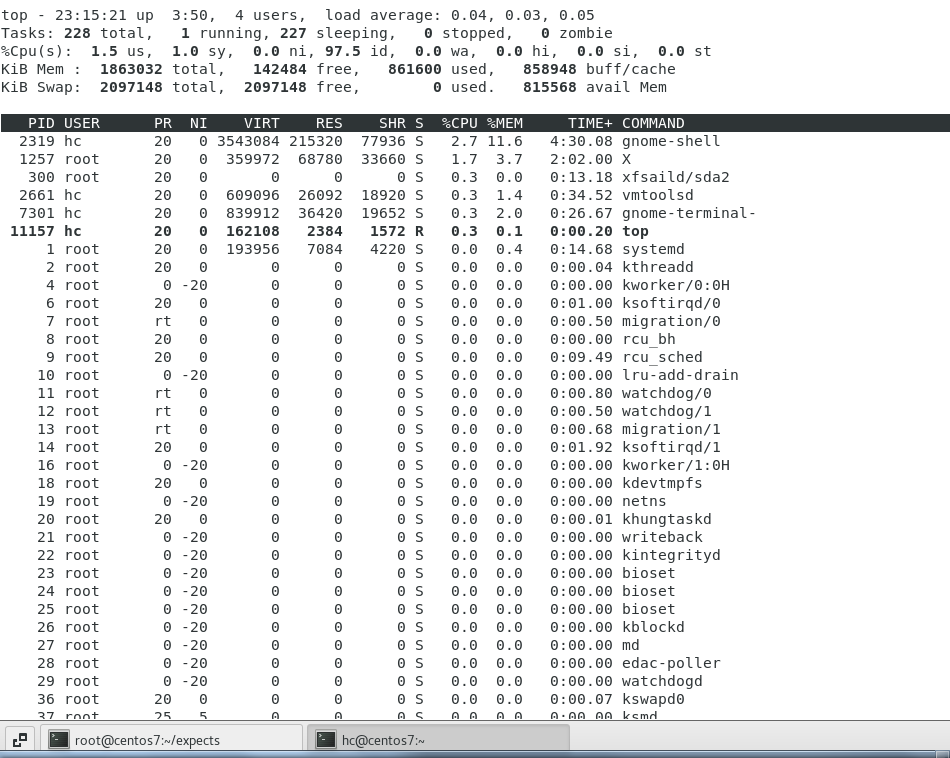
meaning:
top - 23:22:06 up 3:57, 4 users, load average: 0.00, 0.01, 0.05 This line represents the current time -- Startup time--Number of currently logged in users -- Average load -- 5 Minutes, 10 minutes, 15 minutes
Tasks: 228 total, 2 running, 226 sleeping, 0 stopped, 0 zombie This line represents:Total tasks -- Number of tasks currently running -- Number of tasks in hibernation -- Number of tasks in stopped state -- Number of zombie processes
%Cpu(s): 3.6 us, 4.1 sy, 0.0 ni, 92.4 id, 0.0 wa, 0.0 hi, 0.0 si, 0.0 st Within the interval since the last refresh CPU Percentage of status; It's multi-core CPU The average value of can be toggled and displayed as independent by pressing 1 CPU Statistics; us: No in user space nice The running time of the prioritized process sy: Time the kernel space process is running ni: Adjusted nice User space process runtime id: free time wa: wait for IO Time hi,Hard interrupt time si,Soft interrupt (mode switching) st,Time stolen by the virtual machine (time of process execution in the virtual machine)
KiB Mem : 1863032 total, 142340 free, 861596 used, 859096 buff/cache KiB Swap: 2097148 total, 2097148 free, 0 used. 815512 avail Mem Memory and swap partition usage: total: Total size free: Free size, plus buff/cache After that is the total free space that can really be used used: Used size buff/cache: Size occupied by data cache
PID USER PR NI VIRT RES SHR S %CPU %MEM TIME+ COMMAND PID: process ID %MEM: Memory usage percentage VIRT: The amount of virtual memory used, including page tables that have been mapped but not yet used RES: Physical memory size used CODE: The amount of physical memory that can be used to execute code DATA: The private memory space reserved by the process may not have been mapped to physical memory, but has been counted into virtual memory space SHR: Shared memory space, which can be shared by other processes PR: top Command display priority 0-39 Between, the smaller NI: nice priority %CPU: CPU Utilization rate TIME+: Occupied after process startup CPU Time is the accumulation of time slices COMMAND: The name of the process associated program, or the command to start the process
5, Write a script and use for and while to realize whether the address in the 192.168.0.0/24 network segment can be pinged. If pinging is OK, output "success!" and if pinging is not OK, output "fail!"
for loop implementation
[root@centos7 expects]# vi for.sh [root@centos7 expects]# cat for.sh #!/bin/bash for ip in `seq 254`;do echo -n "ping 192.168.0.$ip ...." ping 192.168.0.$ip -c 1 -w 1 &> /dev/null if [ $? == 0 ]; then echo "success!" else echo "fail!" fi done [root@centos7 expects]# chmod 777 for.sh [root@centos7 expects]# ./for.sh ping 192.168.0.1 ....fail! ping 192.168.0.2 ....fail! ping 192.168.0.3 ....fail! ping 192.168.0.4 ....fail! ping 192.168.0.5 ....fail! ping 192.168.0.6 ....fail! ping 192.168.0.7 ....fail! ping 192.168.0.8 ....fail! ping 192.168.0.9 ....fail! ping 192.168.0.10 ....fail! ping 192.168.0.11 ....fail! ping 192.168.0.12 ....fail! ping 192.168.0.13 ....fail! ping 192.168.0.14 ....fail! ping 192.168.0.15 ....fail! ping 192.168.0.16 ....fail!
while implementation:
[root@centos7 expects]# chmod 777 while.sh [root@centos7 expects]# ./while.sh ping 192.168.0.1 ....fail! ping 192.168.0.2 ....fail! ping 192.168.0.3 ....fail! ping 192.168.0.4 ....fail! ping 192.168.0.5 ....fail! ping 192.168.0.6 ....fail! ping 192.168.0.7 ....fail! ping 192.168.0.8 ....fail! ping 192.168.0.9 ....fail! ping 192.168.0.10 ....fail! ping 192.168.0.11 ....fail! ping 192.168.0.12 ....fail!
6, At 1:30 every working day, back up / etc to the / backup directory. The saved file name format is "etcbak-yyyy-mm-dd-HH.tar.xz", where the date is the time of the previous day
[root@centos7 expects]# vim etcbak.sh [root@centos7 expects]# cat etcbak.sh #!/bin/bash #take/etc Backup to/backup In the directory, the format of the saved file name is“ etcbak-yyyy-mm-dd-HH.tar.xz",Where the date is the time of the previous day #If/backup Create if path does not exist backup folder [ -d /backup ] || mkdir /backup 30 1 * * 1-5 /usr/bin/tar -zcf etcbak-date -d "-1 day" +%Y-%m-%d-%H.tar.xz /etc &> /dev/null [root@centos7 expects]# chmod 777 etcbak.sh [root@centos7 expects]# ./etcbak.sh [root@centos7 expects]# ll /backup/ total 0 [root@centos7 expects]#