Writer: character output stream base class
- Structure diagram
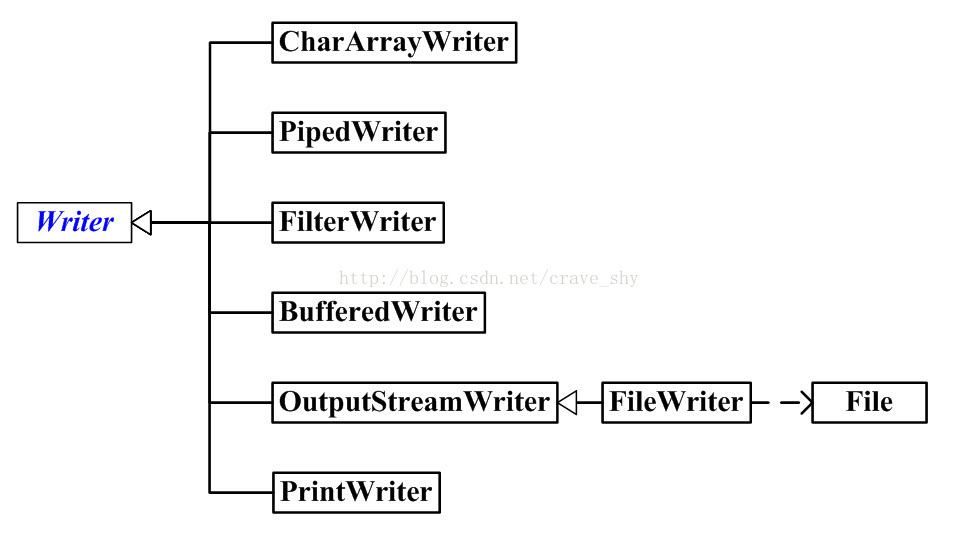
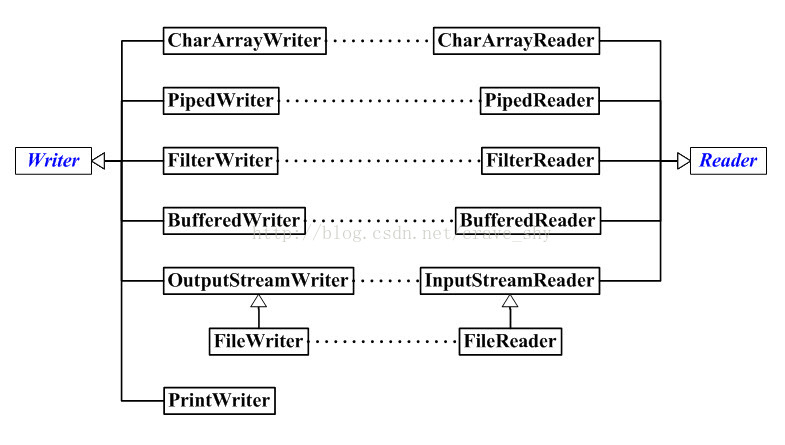
OutputStreamWriter character output stream
-
summary
Transform stream Java io. Outputstreamwriter is a subclass of Writer and a bridge from character stream to byte stream.
It encodes characters into bytes using the specified character set. The character set it uses can be specified by name or accept the default character set of the platform. -
Construction method
Construction method describe OutputStreamWriter(OutputStream out) Create an OutputStreamWriter that uses the default character encoding OutputStreamWriter(OutputStream out, String charsetName) Creates an OutputStreamWriter that uses the specified character set public class Demo { public static void main(String[] args) throws IOException { OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("1.txt"));//Default UTF-8 //Write data osw.write("Shirin "); //Close flow osw.close(); } } ---*--- Output result: Xilin is written to 1 in the directory of the current project.txt In the file
public class Demo { public static void main(String[] args) throws IOException { OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("1.txt"),"GBK"); //Write data osw.write("Shirin "); //Close flow osw.close(); } } ---*--- Output result: ���� 1 written to the directory of the current project.txt In the file
Note: this class converts characters into a series of bytes through the corresponding character set and stores them to the destination. When the two characters of "Celine" are stored in the file through GBK character set coding, the file needs to support GBK coding to display "Celine" normally, otherwise it displays garbled code.
-
Write data method
Method name describe void write(int c) Write a character void write(char[] cbuf) Write a character array void write(char[] cbuf, int off, int len) Write part of a character array void write(String s) Write a string void write(String s, int off, int len) Write part of a string public class Demo{ public static void main(String[] args) throws IOException { OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("1.txt"),"gbk"); //Write a character // osw.write(97); //Write a character array // char[] cs = {'a','b','c','d','e'}; // osw.write(cs); //Write part of a character array // char[] cs = {'a','b','c','d','e'}; // osw.write(cs,1,3); //Write a string // osw.write("abcde"); //Write part of a string // osw.write("abcde",1,3); //Refresh stream // osw.flush(); //Close flow osw.close(); } } ---*--- Output result: a abcde bcd abcde bcd
-
Refresh stream / close stream
Method name describe flush() Flush the buffer and continue to write data close() Close the flow and release resources, but a new flow will be brushed before closing. Once closed, data cannot be written
FileWriter is a convenient class for writing character files
-
summary
Because the name of the conversion stream is long, it is generally implemented according to the local default code. Therefore, in order to simplify writing, the conversion stream provides corresponding subclasses. -
Construction method
Construction method describe FileWriter(String fileName) Create a convenient class object for writing characters and point to the file FileWriter(File file) Create a convenient class object for writing characters and point to the file FileWriter(String fileName ,boolean append) Create a convenient class object for writing characters and point to the file. You can also select append write FileWriter(File file ,boolean append) Create a convenient class object for writing characters and point to the file. You can also select append write -
Copy file comparison
Use conversion flow directlypublic class Demo{ public static void main(String[] args) throws IOException { InputStreamReader isr = new InputStreamReader(new FileInputStream("idea_test\\src\\com\\file\\testFile.java")); OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("copy.java")); //Copy file char[] cs = new char[1024]; int len; while ((len=isr.read(cs))!=-1){ osw.write(cs); } //Close flow isr.close(); osw.close(); } } ---*--- Output result: File copied successfully
Easy to use class
public class Demo{ public static void main(String[] args) throws IOException { FileReader fr = new FileReader("idea_test\\src\\com\\file\\testFile.java"); FileWriter sw = new FileWriter("copy.java"); //Copy file char[] cs = new char[1024]; int c; while ((c= fr.read(cs))!=-1){ sw.write(cs); } //Close flow fr.close(); sw.close(); } } ---*--- Output result: File copied successfully
BufferedReader character buffered input stream
-
summary
Like the byte buffer stream, this class provides a buffer with a settable size for the character output stream. The data is written to the buffer first and then to memory again. Note: the real read data is still the character output stream. This class only provides a buffer. -
Construction method
Construction method describe BufferedReader(Reader in) Create a buffered character input stream that uses a default size input buffer BufferedReader(Reader in, int sz) Creates a buffered character input stream using an input buffer of the specified size -
Buffer character stream copy file
public class Demo{ public static void main(String[] args) throws IOException { BufferedReader br = new BufferedReader(new FileReader("idea_test\\src\\com\\file\\testpoker.java")); BufferedWriter bw = new BufferedWriter(new FileWriter("idea_test\\copy.java")); //Copy one character at a time // int c = 0; // while ((c=br.read())!=-1){ // bw.write(c); // } //Copy one character array at a time char[] cs = new char[1024]; int len; while ((len=br.read(cs))!=-1){ bw.write(cs); } //Close flow br.close(); bw.close(); } } ---*--- Output result: All files were copied successfully
Special function of character buffered output stream
-
void newLine(): write a line separator defined by the system attribute
public class Demo{ public static void main(String[] args) throws IOException { BufferedWriter bw = new BufferedWriter(new FileWriter("e:\\360sd\\2.txt")); BufferedReader br = new BufferedReader(new FileReader("e:360sd\\1.txt")); //Read and write one line of characters at a time String str; int len; while((str=br.readLine())!=null){//Read one line of characters (excluding line breaks) bw.write(str); //Write a string bw.newLine(); //Write a newline character of the current system bw.flush(); //Refresh stream System.out.println(str);//Used to view results } //Close flow br.close(); bw.close(); } } ---*--- Output result: 1 Hello world! 2 Hello world! 3 Hello world! 4 Hello world!
summary
1. Character stream is essentially byte stream. Character and byte conversion is realized through coding table.
2.Buffered flow can be regarded as enhanced convection, so buffered flow is recommended.
3. The character stream is recommended to read and write one line at a time.