Article catalog
preface
- What is an API API (Application Programming Interface): application programming interface
- API in java It refers to Java classes with various functions provided in the JDK. These classes encapsulate the underlying implementation. We don't need to care about how these classes are implemented. We just need to learn how to use these classes. We can learn how to use these API s through help documents.
Tip: the following is the main content of this article. The following cases can be used for reference
1, Common API
1.Math
- 1. Math class overview
- Math contains methods for performing basic numeric operations
- 2. Method calling method in Math
- If there is no constructor in Math class, but the internal methods are static, you can use the class name Make a call
- 3. Common methods of Math class

2.System
- Common methods of System class

Sample code
Requirement: output 1-10000 on the console and calculate how many milliseconds this code has been executed
public class SystemDemo { public static void main(String[] args) { // Get start time node long start = System.currentTimeMillis(); for (int i = 1; i <= 10000; i++) { System.out.println(i); } // Get the time node after the code runs long end = System.currentTimeMillis(); System.out.println("Total time:" + (end - start) + "millisecond"); } }
3. toString method of object class
Object class overview
Object is the root of the class hierarchy. Each class can use object as a superclass. All classes directly or indirectly inherit from this class. In other words, all classes will have a copy of the methods of this class
How to view the method source code
Select the method and press Ctrl + B
How to override the toString method
Alt + Insert select toString
In the blank area of the class, right-click - > generate - > select toString
toString method:
It is more convenient to display the attribute values in the object in a good format
Example code:
class Student extends Object { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "Student{" + "name='" + name + '\'' + ", age=" + age + '}'; } } public class ObjectDemo { public static void main(String[] args) { Student s = new Student(); s.setName("Kaka, Kaka"); s.setAge(30); System.out.println(s); System.out.println(s.toString()); } }
Operation results:
Student{name='Kaka, Kaka', age=30} Student{name='Kaka, Kaka', age=30}
4. equals method of object class
Function of equals method
It is used for comparison between objects and returns true and false results
Example: S1 equals(s2); S1 and S2 are two objects
Scenario of overriding the equals method
When you don't want to compare the address value of the object and want to compare it with the object attribute.
How to override the equals method
alt + insert select equals() and hashCode(), IntelliJ Default, next and finish all the way
In the blank area of the class, right-click - > generate - > select equals() and hashCode(), and the following is the same as above.
Example code:
class Student { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public boolean equals(Object o) { //this -- s1 //o -- s2 if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; Student student = (Student) o; //student -- s2 if (age != student.age) return false; return name != null ? name.equals(student.name) : student.name == null; } } public class ObjectDemo { public static void main(String[] args) { Student s1 = new Student(); s1.setName("Lin Qingxia"); s1.setAge(30); Student s2 = new Student(); s2.setName("Lin Qingxia"); s2.setAge(30); //Requirement: compare whether the contents of two objects are the same System.out.println(s1.equals(s2)); } } Interview questions // Look at the program and analyze the results String s ="abc"; StringBuilder sb = new StringBuilder("abc"); s.equals(sb); sb.equals(s); public class InterviewTest { public static void main(String[] args) { String s1 = "abc"; StringBuilder sb = new StringBuilder("abc"); //1. The equals method in the String class is called at this time //Ensure that the parameter is also a string, otherwise the attribute value will not be compared and false will be returned directly //System.out.println(s1.equals(sb)); // false //The equals method is not overridden in the StringBuilder class, but in the Object class System.out.println(sb.equals(s1)); // false } }
5.Objects
- common method
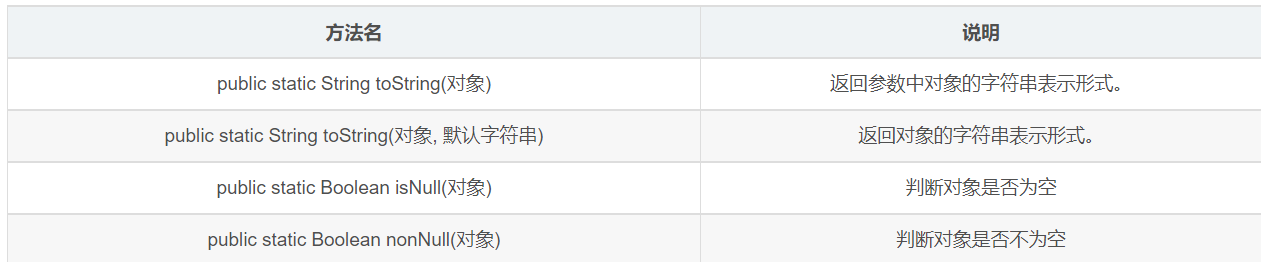
Sample code
Student class
class Student { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "Student{" + "name='" + name + '\'' + ", age=" + age + '}'; } }
Test class
public class MyObjectsDemo { public static void main(String[] args) { // Public static string toString (object): returns the string representation of the object in the parameter. // Student s = new Student("Xiao Luo", 50); // String result = Objects.toString(s); // System.out.println(result); // System.out.println(s); // Public static string toString (object, default string): returns the string representation of the object. If the object is empty, the second parameter is returned //Student s = new Student("Xiaohua", 23); // Student s = null; // String result = Objects.toString(s, "write any one"); // System.out.println(result); // Public static Boolean isnull (object): judge whether the object is empty //Student s = null; // Student s = new Student(); // boolean result = Objects.isNull(s); // System.out.println(result); // Public static Boolean nonnull (object): judge whether the object is not empty //Student s = new Student(); Student s = null; boolean result = Objects.nonNull(s); System.out.println(result); } }
6.BigDecimal
- effect It can be used for accurate calculation
- Construction method
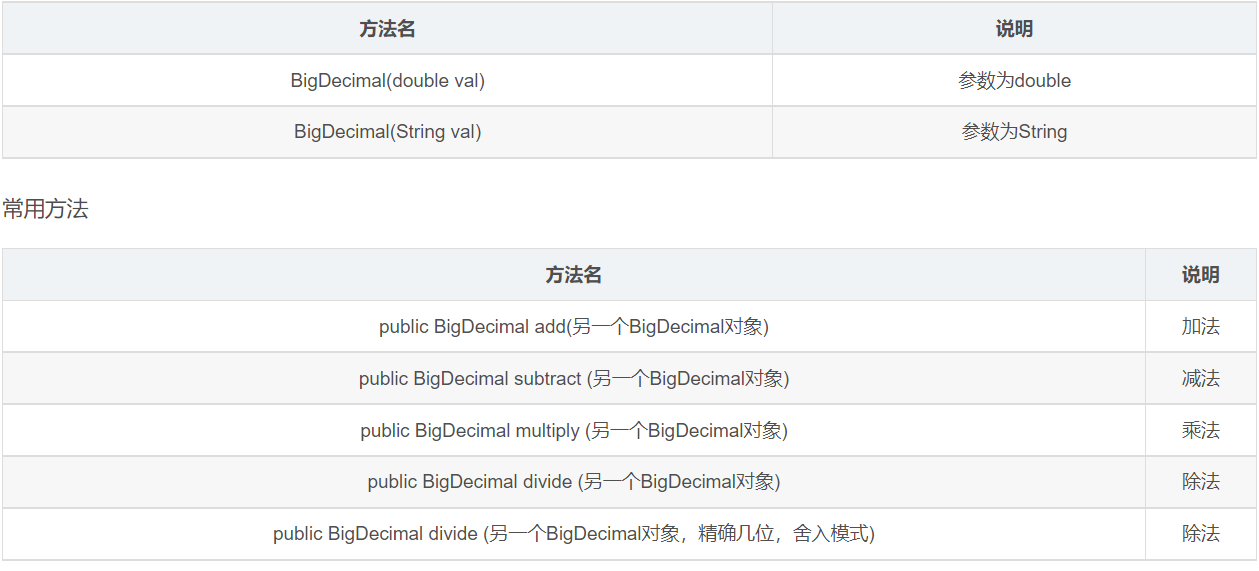
Economic object) Division
public BigDecimal divide (another BigDecimal object, exact number of bits, rounding mode) Division
summary
BigDecimal is used for accurate calculation
Create a BigDecimal object, and the constructor uses a string with the parameter type.
The division in the four operations. If the division is not complete, please use the method of three parameters of divide.
Code example:
BigDecimal divide = bd1.divide(Objects participating in the operation,To what number of decimal places,rounding mode ); Parameter 1 indicates the number of participants in the operation BigDecimal Object. Parameter 2 indicates the number of decimal places after the decimal point Parameter 3, rounding mode BigDecimal.ROUND_UP Progressive method BigDecimal.ROUND_FLOOR Tailless method BigDecimal.ROUND_HALF_UP rounding