Article catalog
1, Concurrent tool class
1. Concurrency tool class - Hashtable
Reasons for the emergence of Hashtable: in collection classes, HashMap is a common collection object, but HashMap is thread unsafe (there may be problems in multi-threaded environment). In order to ensure the security of data, we can use Hashtable, but Hashtable is inefficient.
Code implementation:
package com.itheima.mymap; import java.util.HashMap; import java.util.Hashtable; public class MyHashtableDemo { public static void main(String[] args) throws InterruptedException { Hashtable<String, String> hm = new Hashtable<>(); Thread t1 = new Thread(() -> { for (int i = 0; i < 25; i++) { hm.put(i + "", i + ""); } }); Thread t2 = new Thread(() -> { for (int i = 25; i < 51; i++) { hm.put(i + "", i + ""); } }); t1.start(); t2.start(); System.out.println("----------------------------"); //In order to add all the data t1 and t2 Thread.sleep(1000); //0-0 1-1 ..... 50- 50 for (int i = 0; i < 51; i++) { System.out.println(hm.get(i + "")); }//0 1 2 3 .... 50 } }
2. Concurrency tool class - basic usage of ConcurrentHashMap
Reasons for the occurrence of ConcurrentHashMap: in collection classes, HashMap is a common collection object, but HashMap is thread unsafe (there may be problems in multi-threaded environment). In order to ensure the security of data, we can use Hashtable, but Hashtable is inefficient.
For the above two reasons, we can use jdk1 The ConcurrentHashMap provided after 5.
Architecture:
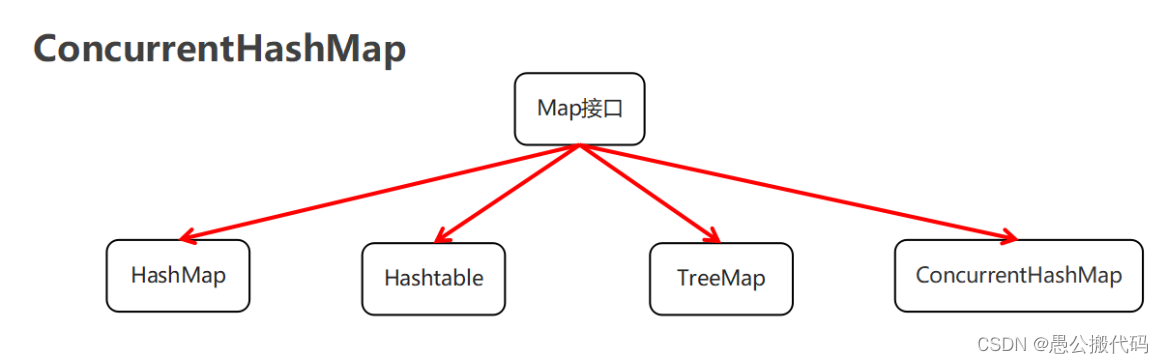
Summary:
1.HashMap is thread unsafe. There will be data security problems in multithreaded environment
2.Hashtable is thread safe, but it will lock the whole table, which is inefficient
3. Concurrent HashMap is also thread safe and efficient. In JDK7 and JDK8, the underlying principle is different.
Code implementation:
package com.itheima.mymap; import java.util.Hashtable; import java.util.concurrent.ConcurrentHashMap; public class MyConcurrentHashMapDemo { public static void main(String[] args) throws InterruptedException { ConcurrentHashMap<String, String> hm = new ConcurrentHashMap<>(100); Thread t1 = new Thread(() -> { for (int i = 0; i < 25; i++) { hm.put(i + "", i + ""); } }); Thread t2 = new Thread(() -> { for (int i = 25; i < 51; i++) { hm.put(i + "", i + ""); } }); t1.start(); t2.start(); System.out.println("----------------------------"); //In order to add all the data t1 and t2 Thread.sleep(1000); //0-0 1-1 ..... 50- 50 for (int i = 0; i < 51; i++) { System.out.println(hm.get(i + "")); }//0 1 2 3 .... 50 } }
3. Concurrency tool class - concurrenthashmap1 7 principle
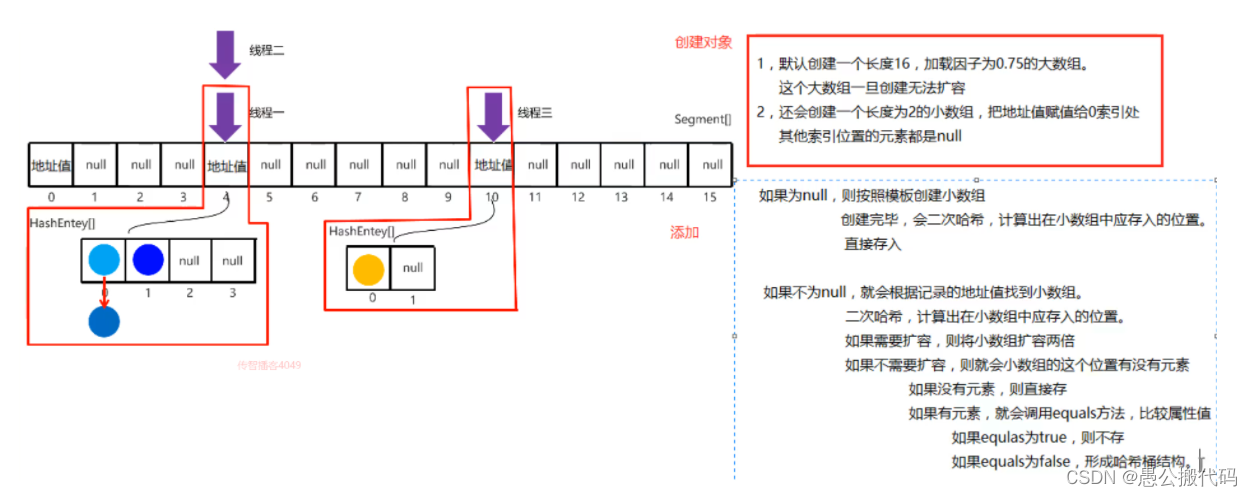
4. Concurrency tool class - concurrenthashmap1 8 principle
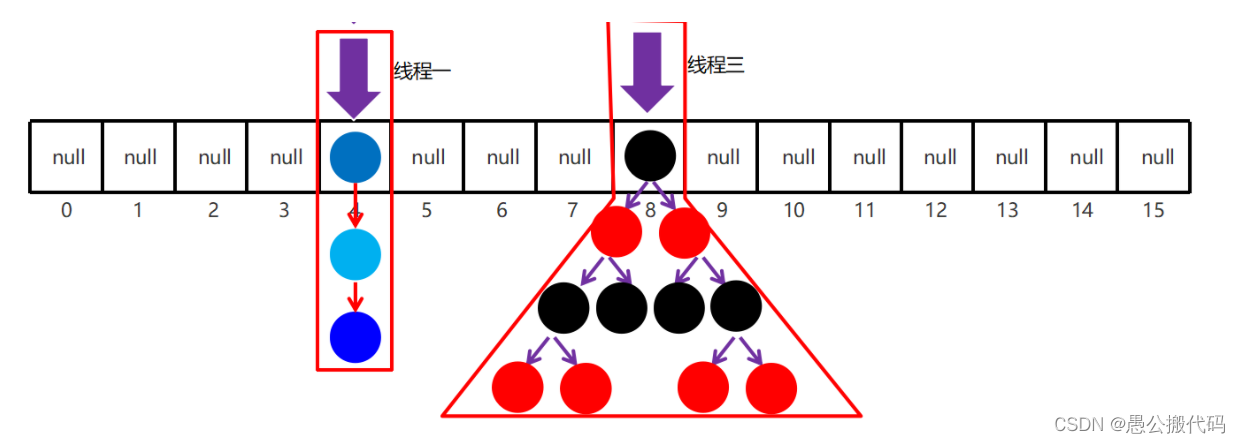
Summary:
1. If you create a ConcurrentHashMap object using an empty parameter construct, you do nothing. Create a hash table the first time you add an element
2. Calculates the index into which the current element should be stored.
3. If the index position is null, the cas algorithm is used to add the node to the array.
4. If the index location is not null, the volatile keyword is used to obtain the latest node address of the current location, which is hung under it and becomes a linked list.
5. When the length of the linked list is greater than or equal to 8, it is automatically converted to red black tree 6. The linked list or red black tree head node is used as the lock object, combined with pessimistic lock to ensure the data security of multi-threaded operation set
5. Concurrency tool class - CountDownLatch
CountDownLatch class:
method | explain |
---|---|
public CountDownLatch(int count) | Parameter number of passing threads, indicating the number of waiting threads |
public void await() | Let the thread wait |
public void countDown() | The current thread has finished executing |
Usage scenario: let a thread wait for other threads to execute before executing
Code implementation:
package com.itheima.mycountdownlatch; import java.util.concurrent.CountDownLatch; public class ChileThread1 extends Thread { private CountDownLatch countDownLatch; public ChileThread1(CountDownLatch countDownLatch) { this.countDownLatch = countDownLatch; } @Override public void run() { //1. Eat dumplings for (int i = 1; i <= 10; i++) { System.out.println(getName() + "Eating the first" + i + "A dumpling"); } //2. Say it after eating //Every time the countDown method is, the counter is set to - 1 countDownLatch.countDown(); } }
package com.itheima.mycountdownlatch; import java.util.concurrent.CountDownLatch; public class ChileThread2 extends Thread { private CountDownLatch countDownLatch; public ChileThread2(CountDownLatch countDownLatch) { this.countDownLatch = countDownLatch; } @Override public void run() { //1. Eat dumplings for (int i = 1; i <= 15; i++) { System.out.println(getName() + "Eating the first" + i + "A dumpling"); } //2. Say it after eating //Every time the countDown method is, the counter is set to - 1 countDownLatch.countDown(); } }
package com.itheima.mycountdownlatch; import java.util.concurrent.CountDownLatch; public class ChileThread3 extends Thread { private CountDownLatch countDownLatch; public ChileThread3(CountDownLatch countDownLatch) { this.countDownLatch = countDownLatch; } @Override public void run() { //1. Eat dumplings for (int i = 1; i <= 20; i++) { System.out.println(getName() + "Eating the first" + i + "A dumpling"); } //2. Say it after eating //Every time the countDown method is, the counter is set to - 1 countDownLatch.countDown(); } }
package com.itheima.mycountdownlatch; import java.util.concurrent.CountDownLatch; public class MotherThread extends Thread { private CountDownLatch countDownLatch; public MotherThread(CountDownLatch countDownLatch) { this.countDownLatch = countDownLatch; } @Override public void run() { //1. Wait try { //When the counter becomes 0, it will automatically wake up the waiting thread here. countDownLatch.await(); } catch (InterruptedException e) { e.printStackTrace(); } //2. Clean up the dishes and chopsticks System.out.println("Mother is cleaning up the dishes"); } }
package com.itheima.mycountdownlatch; import java.util.concurrent.CountDownLatch; public class MyCountDownLatchDemo { public static void main(String[] args) { //1. To create an object of CountDownLatch, you need to pass it to four threads. //A counter is defined at the bottom, and the value of the counter is 3 CountDownLatch countDownLatch = new CountDownLatch(3); //2. Create four thread objects and open them. MotherThread motherThread = new MotherThread(countDownLatch); motherThread.start(); ChileThread1 t1 = new ChileThread1(countDownLatch); t1.setName("Xiao Ming"); ChileThread2 t2 = new ChileThread2(countDownLatch); t2.setName("Xiao Hong"); ChileThread3 t3 = new ChileThread3(countDownLatch); t3.setName("Xiao Gang"); t1.start(); t2.start(); t3.start(); } }
Summary:
1. CountDownLatch(int count): parameter the number of write wait threads. A counter is defined.
2. await(): let the thread wait. When the counter is 0, the waiting thread will wake up
3. countDown(): called when the thread finishes executing, the counter will be - 1.
6. Concurrency tool class Semaphore
Usage scenario:
You can control the number of threads accessing a particular resource.
Implementation steps:
1. Someone needs to manage this channel
2. When a car comes in, issue a pass
3. When the car goes out, withdraw the pass
4. If the permit is issued, other vehicles can only wait
Code implementation:
package com.itheima.mysemaphore; import java.util.concurrent.Semaphore; public class MyRunnable implements Runnable { //1. Obtain the administrator object, private Semaphore semaphore = new Semaphore(2); @Override public void run() { //2. Obtain a pass try { semaphore.acquire(); //3. Start driving System.out.println("Get a pass and start driving"); Thread.sleep(2000); System.out.println("Return the pass"); //4. Return of pass semaphore.release(); } catch (InterruptedException e) { e.printStackTrace(); } } }
package com.itheima.mysemaphore; public class MySemaphoreDemo { public static void main(String[] args) { MyRunnable mr = new MyRunnable(); for (int i = 0; i < 100; i++) { new Thread(mr).start(); } } }