A Brief Introduction to Hibernate
In summary, Hibernate is a lightweight persistence framework for ORM that addresses the mismatch between tables in object and relational databases (impedance mismatch) and the advantage that development code does not inherit hibernate classes or interfaces (non-intrusive).The implementation of the Hibernate framework allows developers to avoid repeatedly writing javajdbc code and using object-oriented thinking to manipulate relational databases.
2. Two ways to create a hibernate instance using myeclipse (taking hibernate 3.5.2 and mysql as examples)
a) Manually write hibernate.cfg.xml and *.hbm.xml files
1. Create a new java project and import the required jar packages (all jar packages + mysql corresponding jar packages + hibernate3.jar packages in the lib\required folder) into the new lib file created under the java project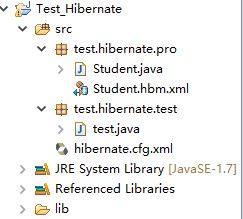
1 package test.hibernate.pro; 2 3 public class Student { 4 int id; 5 int age; 6 String name; 7 int classid; 8 public int getId() { 9 return id; 10 } 11 public void setId(int id) { 12 this.id = id; 13 } 14 public int getAge() { 15 return age; 16 } 17 public void setAge(int age) { 18 this.age = age; 19 } 20 public String getName() { 21 return name; 22 } 23 public void setName(String name) { 24 this.name = name; 25 } 26 public int getClassid() { 27 return classid; 28 } 29 public void setClassid(int classid) { 30 this.classid = classid; 31 } 32 }
3. Use the MysqlGUI tool or the cmd window to build the table (or use hibernate's SchemaExport method to automatically generate the table structure).Here, using the cmd window, enter mysql - hlocalhost - u root - p into the lib directory under the mysql folder, and Enter Password enters the database create to create the table
4. Build the *.hbm.xml file under the pro package to import the header file and complete the mapping relationship between the object and the table structure
1 <?xml version="1.0" encoding="utf-8" ?> 2 <!DOCTYPE hibernate-mapping PUBLIC 3 "-//Hibernate/Hibernate Mapping DTD 3.0//EN" 4 "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> 5 <hibernate-mapping> 6 <class name="test.hibernate.pro.Student" table="student"> 7 <!-- name Value is the object property name, column Values correspond to column names and correspond to each other --> 8 <id name="id" column="stu_id"> 9 <!-- Primary Key Generation Policy --> 10 <generator class="native"></generator> 11 </id> 12 <!-- Entity Class Properties --> 13 <property name="age" column="stu_age"></property> 14 <property name="name" column="stu_name"></property> 15 <property name="classid" column="stu_classid"></property> 16 </class> 17 </hibernate-mapping>
5. Configure the hibernate.cfg.xml file
1 <?xml version="1.0" encoding="utf-8" ?> 2 <!DOCTYPE hibernate-configuration PUBLIC 3 "-//Hibernate/Hibernate Configuration DTD 3.0//EN" 4 "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> 5 6 <hibernate-configuration> 7 <session-factory> 8 <!-- Configuration Resource Information --> 9 <!-- mysql Default port number is 3306 --> 10 <property name="myeclipse.connection.profile">com.mysql.jdbc.Driver</property> 11 <property name="connection.driver_class">com.mysql.jdbc.Driver</property> 12 <property name="connection.url">jdbc:mysql://localhost:3306/hibernate</property> 13 <property name="connection.username">root</property> 14 <property name="connection.password">123456</property> 15 <property name="format_sql">true</property> 16 <property name="show_sql">true</property> 17 18 <!-- Database Dialect --> 19 <property name="dialect">org.hibernate.dialect.MySQLDialect</property> 20 21 <!-- Register Call*. hbm.xml file --> 22 <mapping resource="test/hibernate/pro/Student.hbm.xml"></mapping> 23 </session-factory> 24 </hibernate-configuration>
6. Run the test.java test under the test package
1 package test.hibernate.test; 2 3 import org.hibernate.Session; 4 import org.hibernate.SessionFactory; 5 import org.hibernate.Transaction; 6 import org.hibernate.cfg.Configuration; 7 8 import test.hibernate.pro.Student; 9 10 public class test { 11 public static void main(String[] args) { 12 Configuration cfg = new Configuration().configure(); 13 14 // hibernate3.5 Writing 15 SessionFactory sf = cfg.buildSessionFactory(); 16 // hibernate4.0 -hibernate4.2 Writing 17 // ServiceRegistry reg = new 18 // ServiceRegistryBuilder().applySettings(cfg.getProperties()) 19 // .buildServiceRegistry(); 20 // hibernate4.3 Writing 21 // ServiceRegistry reg = new 22 // StandardServiceRegistryBuilder().applySettings(cfg.getProperties()) 23 // .build(); 24 // SessionFactory sf = cfg.buildSessionFactory(reg); 25 26 Session session = null; 27 Transaction ta = null; 28 try { 29 session = sf.openSession(); 30 ta = session.beginTransaction(); 31 32 Student stu = new Student(); 33 stu.setAge(17); 34 stu.setName("Neo"); 35 stu.setClassid(2); 36 session.save(stu); 37 38 ta.commit(); 39 } catch (Exception e) { 40 e.printStackTrace(); 41 if (ta != null) { 42 ta.rollback(); 43 } 44 } finally { 45 if (session != null && session.isOpen()) { 46 session.close(); 47 } 48 } 49 } 50 }
b) Automatically generate hibernate.cfg.xml and *.hbm.xml files using myeclipse
1. Create a new java web project with pro,factory,dao,test packages in the src directory
2. Open the taskbar window -> Show View -> DB Browser and import the mysqljar package to create a new mysql jdbc Driver.Right-click item - > MyEclipse -> ProjectFacets -> Install Hibernate Facet to create hibernate.cfg.xml and SessionFactory files named SF.java
3. Right-click table structure Hibernate Reverse Engineering in DB Browser reverse generates entity classes to pro packages, chooses three ticks, chooses primary key growth strategy, and chooses native (default is native) in the instance
4.Create a dao layer class in a dao package
1 package test.hibernate.dao; 2 3 import org.hibernate.Session; 4 import org.hibernate.Transaction; 5 6 import test.hibernate.factory.SF; 7 import test.hibernate.pro.Student; 8 9 public class StudentDao { 10 Session session = null; 11 Transaction ta = null; 12 13 public void addStudent(Student stu) { 14 try { 15 session = SF.getSession(); 16 ta = session.beginTransaction(); 17 session.save(stu); 18 ta.commit(); 19 } catch (Exception e) { 20 e.printStackTrace(); 21 if (ta != null) 22 ta.rollback(); 23 } finally { 24 if (session != null) 25 session.close(); 26 } 27 } 28 }
5. Create a test test class test in the test package
1 package test.hibernate.test; 2 3 import test.hibernate.dao.StudentDao; 4 import test.hibernate.pro.Student; 5 6 public class Test { 7 public static void main(String[] args) { 8 StudentDao sd = new StudentDao(); 9 10 Student stu = new Student(); 11 stu.setStuAge(19); 12 stu.setStuName("Mike"); 13 stu.setStuClassid(1); 14 15 sd.addStudent(stu); 16 } 17 }