Use of this tutorial angularjs Version: 1.5.3
AngularJs GitHub: https://github.com/angular/angular.js/
Angular Js Download Address: https://angularjs.org/
I. The Basis and Usage of controller
The Chinese name of controller in Angular JS is controller. It is a controller. JavaScript Function (type/class) to add additional functionality to the scope of the view ($scope). And each controller has its own scope. They can also share data within their father controller's scope.
Its functions are as follows:
(1) Setting the initial state of the scoped object
You can set the initial state of the initial scope by creating a model attribute. For example:
- var app = angular.module('myApp', []);
- app.controller('myController', function($scope) {
- $scope.inputValue = "Pin-Wen Lin Evankaka";
- });
Above we set up an inputValue. If you want to use it in html pages, you can use {inputValue} directly, as follows:var app = angular.module('myApp', []); app.controller('myController', function($scope) { $scope.inputValue = "Pin-Wen Lin Evankaka"; });
- <h1> The content you entered is: {inputValue}</h1>
<h1> The content you entered is: {inputValue}</h1>
- <input type="text" ng-model = "inputValue">
<input type="text" ng-model = "inputValue">
(2) Adding behavior to scoped objects
The behavior of Angular JS scoped objects is represented by scoped methods. These methods can be invoked in templates or views. These methods interact with application models and can change them.
As we said in the chapter on models, any object (or primitive type) that is assigned scope becomes a model. Any scoped method can be invoked in a template or view, and can be invoked through expressions or ng event instructions. As follows:
- var app = angular.module('myApp', []);
- app.controller('myController', function($scope) {
- $scope.myClick = function(){
- alert("click");
- }
- });
Then use on the page:var app = angular.module('myApp', []); app.controller('myController', function($scope) { $scope.myClick = function(){ alert("click"); } });
- <button ng-click= "myClick()" ></button>
This adds a click event to the button<button ng-click= "myClick()" ></button>
II. controller Inheritance
Inheritance here is generally referred to as scope data, because the scope of the sub-controller will prototype inherit the scope of the parent controller. So when you need to reuse functionality from the parent controller, all you need to do is add methods to the parent scope. In this way, the self-controller will obtain all the methods in the parent scope through the prototype of its scope.
The following examples are given:
- <!DOCTYPE html>
- <html lang="zh" ng-app="myApp">
- <head>
- <meta charset="UTF-8">
- <title>AngularJS Introductory Learning</title>
- <script type="text/javascript" src="./1.5.3/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller = "parentCtrl">
- <p><input type="text" ng-model = "value1">Please enter the content</p>
- <h1>The content you entered is:{{value1}}</h1>
- <div ng-controller = "childCtrl">
- <button ng-click = "gerParentValue()"></button>
- </div>
- </div>
- </body>
- <script type="text/javascript">
- var app = angular.module('myApp', []);//Get the html elements that affect the entire angular JS
- app.controller('parentCtrl',function($scope){
- $scope.value2 = "I'm Lin Bingwen.";
- });
- app.controller('childCtrl',function($scope){
- $scope.gerParentValue = function() {
- alert($scope.value1 + $scope.value2);
- }
- });
- </script>
- </html>
<!DOCTYPE html> <html lang="zh" ng-app="myApp"> <head> <meta charset="UTF-8"> <title>AngularJS Introductory Learning</title> <script type="text/javascript" src="./1.5.3/angular.min.js"></script> </head> <body> <div ng-controller = "parentCtrl"> <p><input type="text" ng-model = "value1">Please enter the content</p> <h1>The content you entered is:{{value1}}</h1> <div ng-controller = "childCtrl"> <button ng-click = "gerParentValue()"></button> </div> </div> </body> <script type="text/javascript"> var app = angular.module('myApp', []);//Get the html elements that affect the entire angular JS app.controller('parentCtrl',function($scope){ $scope.value2 = "I'm Lin Bingwen."; }); app.controller('childCtrl',function($scope){ $scope.gerParentValue = function() { alert($scope.value1 + $scope.value2); } }); </script> </html>
It should be noted here that the DVI of child Ctrl must be placed in the DVI of parent Ctrl before it takes effect! And if you need to call the method of the sub-controller from the parent controller, then using the above code will cause errors.
3. Sharing data between controller s
(1) Define scope in parent controller and share it with children
- <!DOCTYPE html>
- <html lang="zh" ng-app="myApp">
- <head>
- <meta charset="UTF-8">
- <title>AngularJS Introductory Learning</title>
- <script type="text/javascript" src="./1.5.3/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="paretnCtrl">
- <input type="text" ng-model="name" />
- <div ng-controller="childCtrl1">
- {{name}}
- <button ng-click="setName()">set name to jack jack jack</button>
- </div>
- <div ng-controller="childCtrl2">
- {{name}}
- <button ng-click="setName()">set name to tom tom tom</button>
- </div>
- </div>
- </body>
- <script type="text/javascript">
- var app = angular.module('myApp', []);
- app.controller('paretnCtrl', function($scope,$timeout) {
- $scope.name = "Pin-Wen Lin Evankaka";
- });
- app.controller('childCtrl1', function($scope,$timeout) {
- $scope.setName = function() {
- $scope.name = "set name to jack jack jack";
- };
- });
- app.controller('childCtrl2', function($scope,$timeout) {
- $scope.setName = function() {
- $scope.name = "set name to tom tom tom";
- };
- });
- </script>
- </html>
<!DOCTYPE html> <html lang="zh" ng-app="myApp"> <head> <meta charset="UTF-8"> <title>AngularJS Introductory Learning</title> <script type="text/javascript" src="./1.5.3/angular.min.js"></script> </head> <body> <div ng-controller="paretnCtrl"> <input type="text" ng-model="name" /> <div ng-controller="childCtrl1"> {{name}} <button ng-click="setName()">set name to jack jack jack</button> </div> <div ng-controller="childCtrl2"> {{name}} <button ng-click="setName()">set name to tom tom tom</button> </div> </div> </body> <script type="text/javascript"> var app = angular.module('myApp', []); app.controller('paretnCtrl', function($scope,$timeout) { $scope.name = "Pin-Wen Lin Evankaka"; }); app.controller('childCtrl1', function($scope,$timeout) { $scope.setName = function() { $scope.name = "set name to jack jack jack"; }; }); app.controller('childCtrl2', function($scope,$timeout) { $scope.setName = function() { $scope.name = "set name to tom tom tom"; }; }); </script> </html>
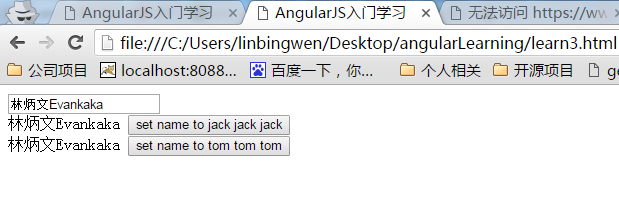
(2) Global sharing of data
Angular JS itself has two ways to set global variables, plus js There are three ways to set global variables. The function to achieve is that the global variables defined in ng-app can be used in different ng-controller s.
By defining global variable s directly through var, this pure js is the same.
Use angular JS value to set global variables.
Use angular JS constant to set global variables.
Here's how to use value
- <!DOCTYPE html>
- <html lang="zh" ng-app="myApp">
- <head>
- <meta charset="UTF-8">
- <title>AngularJS Introductory Learning</title>
- <script type="text/javascript" src="./1.5.3/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="childCtrl1">
- {{name}}
- <button ng-click="setName()">set name to jack jack jack</button>
- </div>
- <div ng-controller="childCtrl2">
- {{name}}
- <button ng-click="setName()">set name to tom tom tom</button>
- </div>
- </body>
- <script type="text/javascript">
- var app = angular.module('myApp', []);
- app.value('data',{'name':'I'm Lin Bingwen.'});
- app.controller('childCtrl1', function($scope,data) {
- $scope.name = data.name;
- $scope.setName = function() {
- $scope.name = "set name to jack jack jack";
- };
- });
- app.controller('childCtrl2', function($scope,data) {
- $scope.name = data.name;
- $scope.setName = function() {
- $scope.name = "set name to tom tom tom";
- };
- });
- </script>
- </html>
<!DOCTYPE html> <html lang="zh" ng-app="myApp"> <head> <meta charset="UTF-8"> <title>AngularJS Introductory Learning</title> <script type="text/javascript" src="./1.5.3/angular.min.js"></script> </head> <body> <div ng-controller="childCtrl1"> {{name}} <button ng-click="setName()">set name to jack jack jack</button> </div> <div ng-controller="childCtrl2"> {{name}} <button ng-click="setName()">set name to tom tom tom</button> </div> </body> <script type="text/javascript"> var app = angular.module('myApp', []); app.value('data',{'name':'I'm Lin Bingwen.'}); app.controller('childCtrl1', function($scope,data) { $scope.name = data.name; $scope.setName = function() { $scope.name = "set name to jack jack jack"; }; }); app.controller('childCtrl2', function($scope,data) { $scope.name = data.name; $scope.setName = function() { $scope.name = "set name to tom tom tom"; }; }); </script> </html>
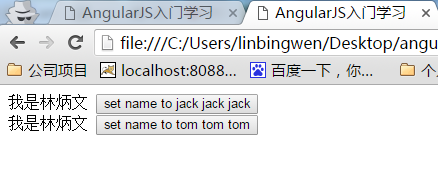
(3) service dependency injection
One of the most prominent features of angular JS is DI, i.e. injection, which uses service to inject data that needs to be shared into the required controller. This is the best way.
- <!DOCTYPE html>
- <html lang="zh" ng-app="myApp">
- <head>
- <meta charset="UTF-8">
- <title>AngularJS Introductory Learning</title>
- <script type="text/javascript" src="./1.5.3/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="childCtrl1">
- {{name}}
- <button ng-click="setName()">set name to jack jack jack</button>
- </div>
- <div ng-controller="childCtrl2">
- {{name}}
- <button ng-click="setName()">set name to tom tom tom</button>
- </div>
- </body>
- <script type="text/javascript">
- var app = angular.module('myApp', []);
- app.factory('dataService', function() {
- var service = {
- name:'I'm Lin Bingwen.'
- };
- return service;
- });
- app.controller('childCtrl1', function($scope,dataService) {
- $scope.name = dataService.name;
- $scope.setName = function() {
- $scope.name = "set name to jack jack jack";
- };
- });
- app.controller('childCtrl2', function($scope,dataService) {
- $scope.name = dataService.name;
- $scope.setName = function() {
- $scope.name = "set name to tom tom tom";
- };
- });
- </script>
- </html>
<!DOCTYPE html> <html lang="zh" ng-app="myApp"> <head> <meta charset="UTF-8"> <title>AngularJS Introductory Learning</title> <script type="text/javascript" src="./1.5.3/angular.min.js"></script> </head> <body> <div ng-controller="childCtrl1"> {{name}} <button ng-click="setName()">set name to jack jack jack</button> </div> <div ng-controller="childCtrl2"> {{name}} <button ng-click="setName()">set name to tom tom tom</button> </div> </body> <script type="text/javascript"> var app = angular.module('myApp', []); app.factory('dataService', function() { var service = { name:'I'm Lin Bingwen.' }; return service; }); app.controller('childCtrl1', function($scope,dataService) { $scope.name = dataService.name; $scope.setName = function() { $scope.name = "set name to jack jack jack"; }; }); app.controller('childCtrl2', function($scope,dataService) { $scope.name = dataService.name; $scope.setName = function() { $scope.name = "set name to tom tom tom"; }; }); </script> </html>
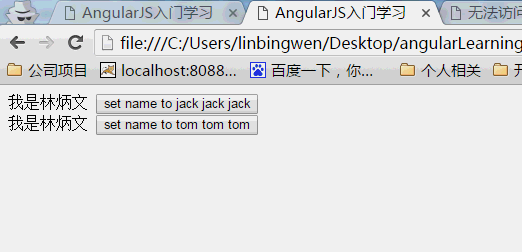
Communication between controller s
In general, the inheritance-based approach is sufficient for most cases, but it does not realize the communication between sibling controllers, so it leads to the way of events. In the event-based approach, we can implement the functions of $on,$emit,$boardcast, where $on means event listener and $emit means parent or higher.
Scope triggers events, $boardcast represents broadcast events to scopes below the child level.
$emit can only pass event and data to parent controller
$broadcast can only pass event and data to child controller
$on is used to receive event and data
Example 1:
- <!DOCTYPE html>
- <html lang="zh" ng-app="myApp">
- <head>
- <meta charset="UTF-8">
- <title>AngularJS Introductory Learning</title>
- <script type="text/javascript" src="./1.5.3/angular.min.js"></script>
- </head>
- <body>
- <div ng-app="app" ng-controller="parentCtr">
- <div ng-controller="childCtr1">childCtr1 name :
- <input ng-model="name" type="text" ng-change="change(name)" />
- </div>
- <div ng-controller="childCtr2">from childCtr1 name:
- <input ng-model="ctr1Name" />
- </div>
- </div>
- </body>
- <script type="text/javascript">
- var app = angular.module('myApp', []);
- app.controller("parentCtr",function ($scope) {
- $scope.$on("Ctr1NameChange",function (event, msg) {//Receive information from child Ctr1 and broadcast it to all child controller s.
- console.log("parent", msg);
- $scope.$broadcast("Ctr1NameChangeFromParrent", msg);//Broadcast to all sub-controller s
- });
- });
- app.controller("childCtr1", function ($scope) {
- $scope.change = function (name) {
- console.log("childCtr1", name);
- $scope.$emit("Ctr1NameChange", name);//Pass the information to the parent controller
- };
- }).controller("childCtr2", function ($scope) {
- $scope.$on("Ctr1NameChangeFromParrent",function (event, msg) { //Listen for information from father controller
- console.log("childCtr2", msg);
- $scope.ctr1Name = msg;
- });
- });
- </script>
- </html>
<!DOCTYPE html> <html lang="zh" ng-app="myApp"> <head> <meta charset="UTF-8"> <title>AngularJS Introductory Learning</title> <script type="text/javascript" src="./1.5.3/angular.min.js"></script> </head> <body> <div ng-app="app" ng-controller="parentCtr"> <div ng-controller="childCtr1">childCtr1 name : <input ng-model="name" type="text" ng-change="change(name)" /> </div> <div ng-controller="childCtr2">from childCtr1 name: <input ng-model="ctr1Name" /> </div> </div> </body> <script type="text/javascript"> var app = angular.module('myApp', []); app.controller("parentCtr",function ($scope) { $scope.$on("Ctr1NameChange",function (event, msg) {//Receive information from child Ctr1 and broadcast it to all child controller s console.log("parent", msg); $scope.$broadcast("Ctr1NameChangeFromParrent", msg);//Broadcast to all sub-controller s }); }); app.controller("childCtr1", function ($scope) { $scope.change = function (name) { console.log("childCtr1", name); $scope.$emit("Ctr1NameChange", name);//Pass information to parent controller }; }).controller("childCtr2", function ($scope) { $scope.$on("Ctr1NameChangeFromParrent",function (event, msg) { //Listening for information from father controller console.log("childCtr2", msg); $scope.ctr1Name = msg; }); }); </script> </html>
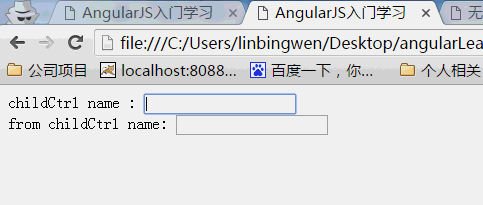
Example 2:
- <!DOCTYPE html>
- <html lang="zh" ng-app="myApp">
- <head>
- <meta charset="UTF-8">
- <title>AngularJS Introductory Learning</title>
- <script type="text/javascript" src="./1.5.3/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="ParentCtrl"> <!--Father level-->
- <div ng-controller="SelfCtrl"> <!--Own-->
- <button ng-click="click()">click me</button>
- <div ng-controller="ChildCtrl">
- </div> <!--Sub level-->
- </div>
- <div ng-controller="BroCtrl">
- </div> <!--Level-->
- </div>
- </body>
- <script type="text/javascript">
- var app = angular.module('myApp', []);
- app.controller('SelfCtrl', function($scope) {
- $scope.click = function () {
- $scope.$broadcast('to-child', 'child');
- $scope.$emit('to-parent', 'parent');
- }
- });
- app.controller('ParentCtrl', function($scope) {
- $scope.$on('to-parent', function(event,data) {
- console.log('ParentCtrl', data); //Parents get values.
- });
- $scope.$on('to-child', function(event,data) {
- console.log('ParentCtrl', data); //The sublevel does not get a value.
- });
- });
- app.controller('ChildCtrl', function($scope){
- $scope.$on('to-child', function(event,data) {
- console.log('ChildCtrl', data); //Sublevel can get value.
- });
- $scope.$on('to-parent', function(event,data) {
- console.log('ChildCtrl', data); //Parents do not get values.
- });
- });
- app.controller('BroCtrl', function($scope){
- $scope.$on('to-parent', function(event,data) {
- console.log('BroCtrl', data); //Level is not valued.
- });
- $scope.$on('to-child', function(event,data) {
- console.log('BroCtrl', data); //Level is not valued.
- });
- });
- </script>
- </html>
Output results:<!DOCTYPE html> <html lang="zh" ng-app="myApp"> <head> <meta charset="UTF-8"> <title>AngularJS Introductory Learning</title> <script type="text/javascript" src="./1.5.3/angular.min.js"></script> </head> <body> <div ng-controller="ParentCtrl"> <!--Father level--> <div ng-controller="SelfCtrl"> <!--Own--> <button ng-click="click()">click me</button> <div ng-controller="ChildCtrl"> </div> <!--Sub level--> </div> <div ng-controller="BroCtrl"> </div> <!--Level--> </div> </body> <script type="text/javascript"> var app = angular.module('myApp', []); app.controller('SelfCtrl', function($scope) { $scope.click = function () { $scope.$broadcast('to-child', 'child'); $scope.$emit('to-parent', 'parent'); } }); app.controller('ParentCtrl', function($scope) { $scope.$on('to-parent', function(event,data) { console.log('ParentCtrl', data); //Parents can get values }); $scope.$on('to-child', function(event,data) { console.log('ParentCtrl', data); //The sublevel does not get a value }); }); app.controller('ChildCtrl', function($scope){ $scope.$on('to-child', function(event,data) { console.log('ChildCtrl', data); //Sublevel can get value }); $scope.$on('to-parent', function(event,data) { console.log('ChildCtrl', data); //Parents do not get values }); }); app.controller('BroCtrl', function($scope){ $scope.$on('to-parent', function(event,data) { console.log('BroCtrl', data); //Level can't get value }); $scope.$on('to-child', function(event,data) { console.log('BroCtrl', data); //Level can't get value }); }); </script> </html>
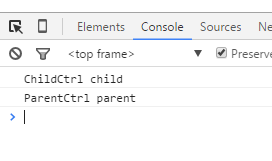
$emit and $broadcast can pass multiple parameters, and $on can receive multiple parameters.
The event event event parameter in the $on method has the following attributes and methods for the object
Event attributes | objective |
---|---|
event.targetScope | Scope of sending out or disseminating the original event |
event.currentScope | Scope of events currently being addressed |
event.name | Event name |
event.stopPropagation() | A function that prevents further propagation of events (bubbles/traps) (this applies only to events emitted using `emit') |
event.preventDefault() | This method doesn't actually do anything, but it sets `default Prevented'to true. It does not check the `defaultPrevented'value until the event listener implementer takes action. |
event.defaultPrevented | If called |
5. Some Suggestions for the controller Layer
1. controller layer does not involve too much business logic. It can extract common parts to Service layer.
2. service layer: mainly responsible for data interaction and data processing, processing logic in some business areas;
3. controller layer: It is mainly responsible for initializing the variables of $scope to pass to view layer, and handling some logic generated by page interaction.
4. When a function is to design remote API calls, data sets, business insights into complex logic, will be a large number of repetitive computing methods, you can consider injecting code into the controller layer in the form of service.
5. The $scope in the controller is the only source of page data. Do not modify DOM directly.
6. controller should not be global
Reference article:
http://www.jianshu.com/p/1e1aaf0fd30a
http://cnodejs.org/topic/54dd47fa7939ece1281aa54f
http://www.html-js.com/article/1847
http://blog.51yip.com/jsjquery/1601.html
http://www.cnblogs.com/CraryPrimitiveMan/p/3679552.html?utm_source=tuicool&utm_medium=referral
http://www.cnblogs.com/whitewolf/archive/2013/04/16/3024843.html
Reprinted from: http://blog.csdn.net/evankaka/article/details/51097039
For more knowledge, welcome to join 651308349, 627336556 technology development exchange group, there will be unexpected gains ~!!!