catalogue
Specific functions of hook functions
Number of life cycle executions of the component
Component lifecycle execution sequence
preface
I've been learning vue and nodejs recently. I can't think about React for a long time. Don't forget. Write a blog to solve the pain point and life cycle when I first learned React.
Understanding of life cycle
- Components go through specific stages from creation to death.
- The React component contains a series of hook functions (life cycle callback functions), which will be called at a specific time.
- When defining components, we will do specific work in specific life cycle callback functions.
Three states of the lifecycle
- Mounting: real DOM inserted
- Updating: being re rendered
- Unmounting: the real DOM has been removed
Three phases of the life cycle (old)
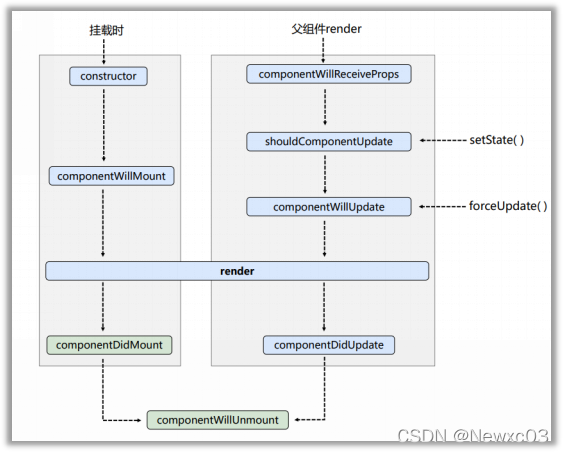
1. Initialization phase: by reactdom Render() trigger - first render
- constructor()
- componentWillMount()
- render()
- componentDidMount()
2. Update phase: this. By the component Setsate() or parent component re render trigger
- shouldComponentUpdate()
- componentWillUpdate()
- render()
- componentDidUpdate()
3. Uninstall components: by reactdom Unmountcomponentatnode() trigger
- componentWillUnmount()
Life cycle flowchart (New)
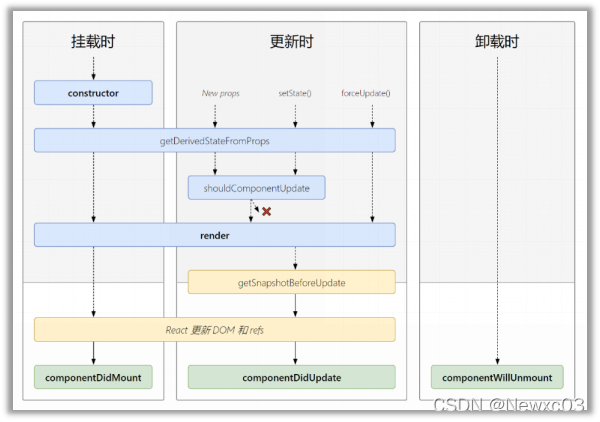
1. Initialization phase: by reactdom Render() trigger - first render
- constructor()
- getDerivedStateFromProps
- render()
- componentDidMount()
2. Update phase: this. By the component Setsate() or parent component re render trigger
- getDerivedStateFromProps
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate
- componentDidUpdate()
3. Uninstall components: by reactdom Unmountcomponentatnode() trigger
- componentWillUnmount()
Important hook
- render: call to initialize rendering or update rendering
- componentDidMount: enable listening and send ajax requests
- componentWillUnmount: do some finishing work, such as cleaning up the timer
Hook to be discarded
- componentWillMount
- componentWillReceiveProps
- componentWillUpdate
Specific functions of hook functions
1,constructor() Complete the initialization of React data.
2,componentWillMount() The logic executed when the component has finished initializing data but has not yet rendered the DOM is mainly used for server-side rendering.
3,componentDidMount() The logic executed when the component finishes rendering for the first time. At this time, the DOM node has been generated.
4,componentWillReceiveProps(nextProps) When receiving new props from the parent component, re render the logic executed by the component.
5,shouldComponentUpdate(nextProps, nextState) After setState, the state changes, and the component will enter the logic executed when re rendering. In this life cycle, return false can prevent component updates. It is mainly used for performance optimization.
6,componentWillUpdate(nextProps, nextState) After shouldComponentUpdate returns true, the logic executed when the component enters the re rendering process.
7,render() The render function compiles jsx into a function and generates a virtual DOM, then compares the old and new DOM trees before and after the update through its diff algorithm, and renders the changed nodes.
8,componentDidUpdate(prevProps, prevState) The logic executed after re rendering.
9,componentWillUnmount() The logic executed before uninstalling the component, such as "clear all timeouts, setintervals and other timers in the component" or "remove listeners in all components removeEventListener".
Number of life cycle executions of the component
Execute only once:
- constructor
- componentWillMount
- componentDidMount
Execute multiple times:
- render
- componentWillReceiveProps of subcomponents
- componentWillUpdate
- componentDidUpdate
Conditional execution:
- componentWillUnmount (when the page leaves and the component is destroyed)
Not executed:
- componentWillReceiveProps of the root component (the component of ReactDOM.render on the DOM) (because there is no parent component to pass props)
Component lifecycle execution sequence
Suppose the component nesting relationship is that there is a parent component in the App and a child component in the parent component.
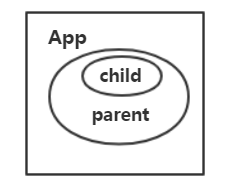
If the setState update is not involved, the order of the first rendering is as follows:
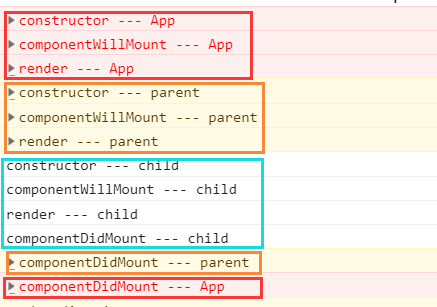
App: constructor --> componentWillMount --> render --> parent: constructor --> componentWillMount --> render --> child: constructor --> componentWillMount --> render --> componentDidMount (child) --> componentDidMount (parent) --> componentDidMount (App)
The App's setState event is triggered at this time
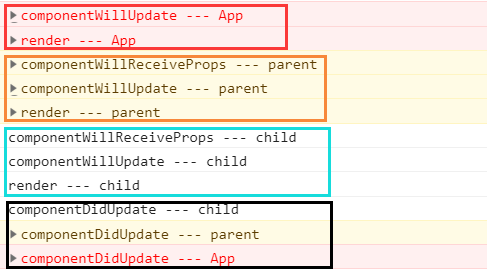
App: componentWillUpdate --> render --> parent: componentWillReceiveProps --> componentWillUpdate --> render --> child: componentWillReceiveProps --> componentWillUpdate --> render --> componentDidUpdate (child) --> componentDidUpdate (parent) --> componentDidUpdate (App
What if it is the setState that triggers the parent?
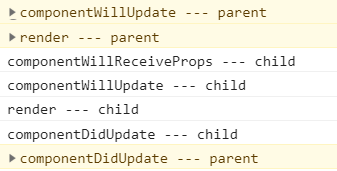
parent: componentWillUpdate --> render --> child: componentWillReceiveProps --> componentWillUpdate --> render --> componentDidUpdate (child) --> componentDidUpdate (parent)
What if it just triggers the setState of the child component itself?

child: componentWillUpdate --> render --> componentDidUpdate (child)
Conclusion:
- As shown in the figure: the sequence before completion is from root to sub part, and from sub part to root when completion. (similar to event mechanism)
- During the red line (including initial and update) life cycle of each component, the lower level red line life cycle will be executed after one brain is executed.
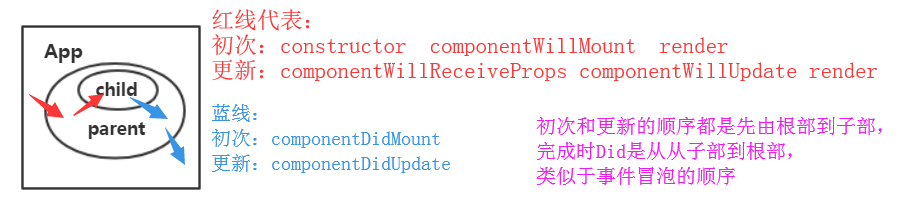
- The first level component setState cannot trigger the life cycle update function of its parent component, but can only trigger the life cycle update function of a lower level. The summary is as follows:
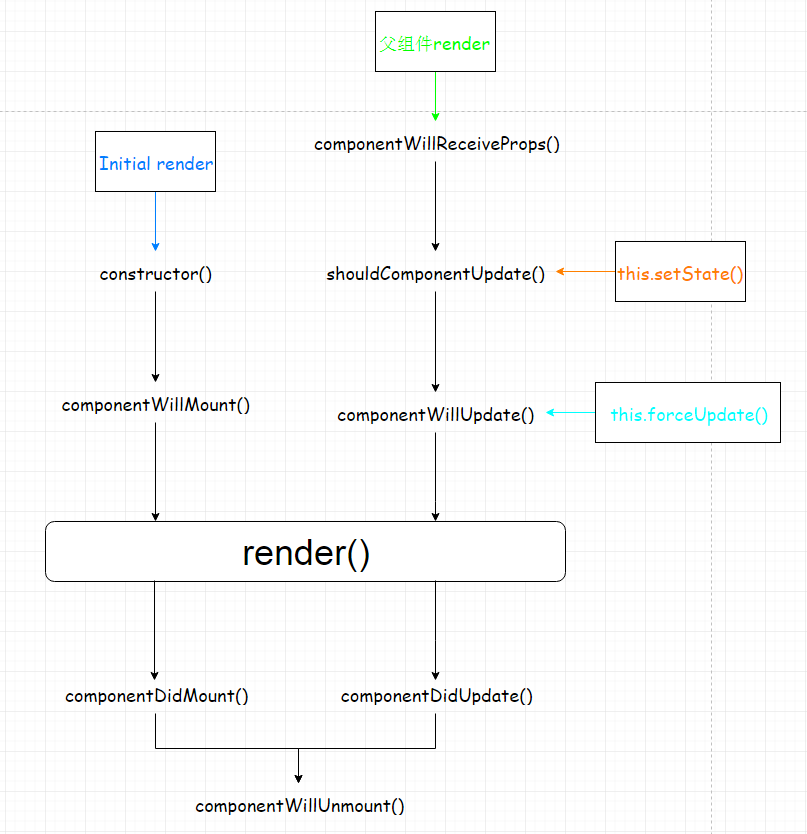
Small example
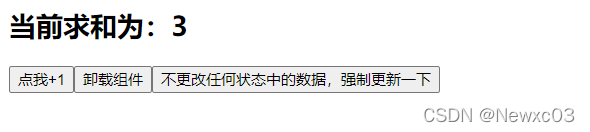
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>3_react life cycle(new)</title> </head> <body> <!-- Prepare a "container" --> <div id="test"></div> <!-- introduce react Core library --> <script type="text/javascript" src="../js/17.0.1/react.development.js"></script> <!-- introduce react-dom,Used to support react operation DOM --> <script type="text/javascript" src="../js/17.0.1/react-dom.development.js"></script> <!-- introduce babel,Used to jsx Turn into js --> <script type="text/javascript" src="../js/17.0.1/babel.min.js"></script> <script type="text/babel"> //Create component class Count extends React.Component{ /* 1. Initialization phase: by reactdom Render() trigger - first render 1. constructor() 2. getDerivedStateFromProps 3. render() 4. componentDidMount() =====> Commonly used Generally, initialization is done in this hook, such as starting the timer, sending network requests, and subscribing to messages 2. Update phase: this. By the component Setsate() or parent component re render trigger 1. getDerivedStateFromProps 2. shouldComponentUpdate() 3. render() 4. getSnapshotBeforeUpdate 5. componentDidUpdate() 3. Uninstall components: by reactdom Unmountcomponentatnode() trigger 1. componentWillUnmount() =====> Commonly used Generally, you can do some closing things in this hook, such as closing the timer and unsubscribing messages */ //constructor constructor(props){ console.log('Count---constructor'); super(props) //Initialization status this.state = {count:0} } //Add 1 button callback add = ()=>{ //Get original status const {count} = this.state //Update status this.setState({count:count+1}) } //Callback for unload component button death = ()=>{ ReactDOM.unmountComponentAtNode(document.getElementById('test')) } //Callback for force Update button force = ()=>{ this.forceUpdate() } //If the value of state depends on props at any time, you can use getDerivedStateFromProps static getDerivedStateFromProps(props,state){ console.log('getDerivedStateFromProps',props,state); return null } //Take a snapshot before updating getSnapshotBeforeUpdate(){ console.log('getSnapshotBeforeUpdate'); return 'atguigu' } //Hook after component mounting componentDidMount(){ console.log('Count---componentDidMount'); } //The hook that the component will unload componentWillUnmount(){ console.log('Count---componentWillUnmount'); } //Control component updated "valve" shouldComponentUpdate(){ console.log('Count---shouldComponentUpdate'); return true } //Hook after component update componentDidUpdate(preProps,preState,snapshotValue){ console.log('Count---componentDidUpdate',preProps,preState,snapshotValue); } render(){ console.log('Count---render'); const {count} = this.state return( <div> <h2>The current summation is:{count}</h2> <button onClick={this.add}>Point me+1</button> <button onClick={this.death}>Uninstall components</button> <button onClick={this.force}>Do not change the data in any status, and force an update</button> </div> ) } } //Rendering Components ReactDOM.render(<Count count={199}/>,document.getElementById('test')) </script> </body> </html>