File class
effect
The File class can create an object location File: delete, obtain the information of the text itself, and so on(
package com.itliuxue.d2_recursion; public class RecursionDemoc03 { public static void main(String[] args) { System.out.println(f(1)); } public static int f(int n){ if(n == 10){ return 1; }else { return 2*(f(n+1) + 1); } } }
But you can't read or write the contents of the file)
summary
File class in package Java io. Under file, the file object (file, folder) representing the operating system
File class provides functions such as locating files, obtaining the information of the file itself, deleting files, creating files (folders) and so on
Create object with File class
1. Create a File object (specify the path of the File)
package com.itliuxue.d1_file; import java.io.File; public class FileDemo { public static void main(String[] args) { // File f = new File("C:/Users/LIUXUE/Desktop/wallhaven-z8dp1v.jpg"); // File f = new File("C:"+ File.separator +"Users"+ File.separator +"LIUXUE"+ File.separator +"Desktop"+ File.separator +"wallhaven-z8dp1v.jpg"); File f = new File("C:\\Users\\LIUXUE\\Desktop\\wallhaven-z8dp1v.jpg"); long length = f.length();//Byte size of the file System.out.println(length); } }
2.File creates objects and supports absolute paths and relative paths (key points)
Absolute path and relative path
package com.itliuxue.d1_file; import java.io.File; public class FileDemo { public static void main(String[] args) { //Absolute path File f1 = new File("C:\\Users\\LIUXUE\\Desktop\\wallhaven-z8dp1v.jpg"); System.out.println(f1.length()); //Relative path: generally locate the files in the module, relative to the project!!! File f2 = new File("file-io-app/src/date.txt"); long l2 = f2.length(); System.out.println(l2); } }
3.File creates objects, which can be files or folders
Common API of File class
1. Judge the file type and obtain the file information
2.File class creates file functions and deletes file functions
3.File traversal function
Results
listFiles precautions:
Returns null when the caller does not exist
Returns null when the caller is a file
Returns null when the caller is an empty folder
When the caller is a folder with contents, the paths of all the files and folders in it are returned in the File array
When the caller is a folder with hidden files, the paths of all the files and folders in it are returned in the File array, including the hidden contents
When the caller is a folder that requires permission to enter, null is returned
Method recursion
Forms and characteristics of recursion
The concept of method recursion
Methods that call themselves directly or indirectly are called recursion
As an algorithm, recursion is widely used in programming languages
Recursive form
Direct recursion: method calls itself
Indirect recursion: method calls other methods, and other methods call back their own methods
Existing problems: if the recursion is not terminated properly, a recursive loop will appear, resulting in memory overflow
Recursive algorithm flow, core elements
package com.itliuxue.d2_recursion; public class RecursionDemo02 { public static void main(String[] args) { System.out.println(f(5)); } public static int f(int n){ if(n == 1){ return 1; }else{ return f( n-1)*n; } } }
Common cases of recursion
package com.itliuxue.d2_recursion; public class RecursionDemo02 { public static void main(String[] args) { System.out.println(f(5)); } public static int f(int n){ if(n == 1){ return 1; }else{ return f( n-1) + n; } } }
Classic problem of recursion -- monkey eating peach problem
package com.itliuxue.d2_recursion; public class RecursionDemoc03 { public static void main(String[] args) { System.out.println(f(1)); } public static int f(int n){ if(n == 10){ return 1; }else { return 2*(f(n+1) + 1); } } }
Irregular recursive case -- file search, beer problem
package com.itliuxue.d2_recursion; import java.io.File; public class RecursionDemo04 { public static void main(String[] args) { //Incoming file and file name search(new File("E:/"), "characters.vpp_pc"); } //Search all the files in a directory to find the problem we want //1dir: fileName of the searched source directory; Name of the file being searched public static void search(File dir, String fileName) { //3 determine whether dir is a folder if (dir != null && dir.isDirectory()) { //4. Extract the first level file object under the current directory File[] files = dir.listFiles(); //5. Judge whether there is a level-1 object. Only when it exists can it be traversed if(files != null&&files.length>0){ for (File file : files) { //6. Judge whether the first level file object currently traversed is a file or a directory if(file.isFile()){ //7. Whether it is the one found and output the path if(file.getName().contains(fileName)){ System.out.println("eureka:"+file.getAbsolutePath()); } }else{ //8. If it is a folder, search recursively again search(file,"characters.vpp_pc"); } } } } else { System.out.println("Sorry, the folder you are searching for is not"); } } }
package com.itliuxue.d2_recursion; public class RecursionDemo05 { public static int lastCoverNumber; public static int lastBottleNumber; public static int totalNumber; public static void main(String[] args) { buy(10); System.out.println(totalNumber); System.out.println(lastBottleNumber); System.out.println(lastCoverNumber); } public static void buy(int money){ int buyNumber = money / 2; totalNumber += buyNumber; //2. Convert the number of caps and bottles into money int coverNumber = lastCoverNumber + buyNumber; int bottleNumber = lastBottleNumber + buyNumber; int allMoney = 0; if(coverNumber >= 4){ allMoney += (coverNumber / 4) * 2; } lastCoverNumber = coverNumber % 4; if(bottleNumber >= 2){ allMoney += (bottleNumber / 2)*2; } lastBottleNumber = bottleNumber % 2; if(allMoney >= 2){ buy(allMoney); } } }
character set
Basic knowledge of character set
The bottom layer of the computer cannot store characters directly. The bottom layer of the computer can only store binary (0, 1)
Binary can be converted to decimal
Conclusion: the bottom layer of the computer can represent decimal number. The computer can number and store human characters. This set of numbering rules is the character set
ASCLL character set (American Standard Code for information interchange)
Including numbers, English and symbols.
ASCLL uses 1 byte to store a character. A byte is 8 bits. It can represent 128 character information in total. For English, numbers are enough
GBK:
The default code table of windows system. Compatible with ASCLL code table, it also contains tens of thousands of Chinese characters, and supports traditional Chinese characters and some Japanese and Korean characters
Note: GBK is the code table of China. A Chinese is stored in the form of two bytes. But it does not contain the words of all countries in the world
Unicode code table:
Unicode (also known as unified code, universal code, single code) is an industry character coding standard in the field of computer science
It contains all the common characters and symbols in most countries in the world
Analysis of Chinese character storage and display process
Summary
Character set encoding and decoding operation
package com.itliuxue.d3_charset; import java.io.UnsupportedEncodingException; import java.util.Arrays; public class Test { public static void main(String[] args) throws UnsupportedEncodingException { //1. Encoding: convert text into bytes (using the specified encoding) String name = "abc I love you China"; byte[] bytes = name.getBytes();//Encode with the current default character set (UTF-8) // byte[] bytes = name.getBytes("GBK"); System.out.println(bytes.length); System.out.println(Arrays.toString(bytes)); // 2. Decoding: convert the byte into the corresponding Chinese form (the character set before and after encoding must be consistent, otherwise it is garbled) String rs = new String(bytes);//Default UTF-8 // String rs1 = new String(bytes,"GBK"); System.out.println(rs); } }
IO stream
summary
IO streams, also known as input and output streams, are used to read and write data
I stands for input, which is the process of reading data from hard disk file to memory. It is called input and is responsible for reading
O stands for output, which is the process from memory to writing out the data of the memory program to the hard disk file. It is called output and is responsible for writing
Classification of IO streams
Classification of summary flow
Byte input stream: Based on memory, the stream in which data from disk file / network is read into memory in the form of bytes is called byte input stream
Byte output stream: Based on memory, the stream that writes the data in memory to disk file or network in bytes is called byte output stream
Character input stream: Based on memory, the stream in which data from disk file / network is read into memory in the form of characters is called byte input stream
Character output stream: Based on memory, the stream that writes the data in memory to disk file or network as characters is called byte output stream
Summary of IO flows
Use of byte stream
File byte input stream: FileInputStream
Function: take the memory as the benchmark to read the data in the disk file into the memory in the form of bytes
package com.itliuxue.d4_byte_stream; import java.io.*; public class FileInputStreamDemo01 { public static void main(String[] args) throws IOException { //Create a file input stream pipeline to connect with the source file // InputStream is = new FileInputStream(new File("file-io-app\\src\\date.txt")); InputStream is = new FileInputStream("D:\\IDEA\\file-io-app\\src\\date.txt"); //Define a variable to record the bytes read each time int b; while((b = is.read()) != -1){ System.out.println((char)b); } } }
Summary
File Bytes input stream: one byte array is read at a time
package com.itliuxue.d4_byte_stream; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.InputStream; public class FileInputDemo02 { public static void main(String[] args) throws Exception { //Create a file byte input stream pipeline to connect with the source file InputStream is = new FileInputStream("D:\\IDEA\\file-io-app\\src\\date.txt"); byte[] buffer = new byte[3]; int len; while((len = is.read(buffer)) != -1) { System.out.println("Read a few bytes" + len); String rs = new String(buffer, 0, len); System.out.println(rs); } } }
Summary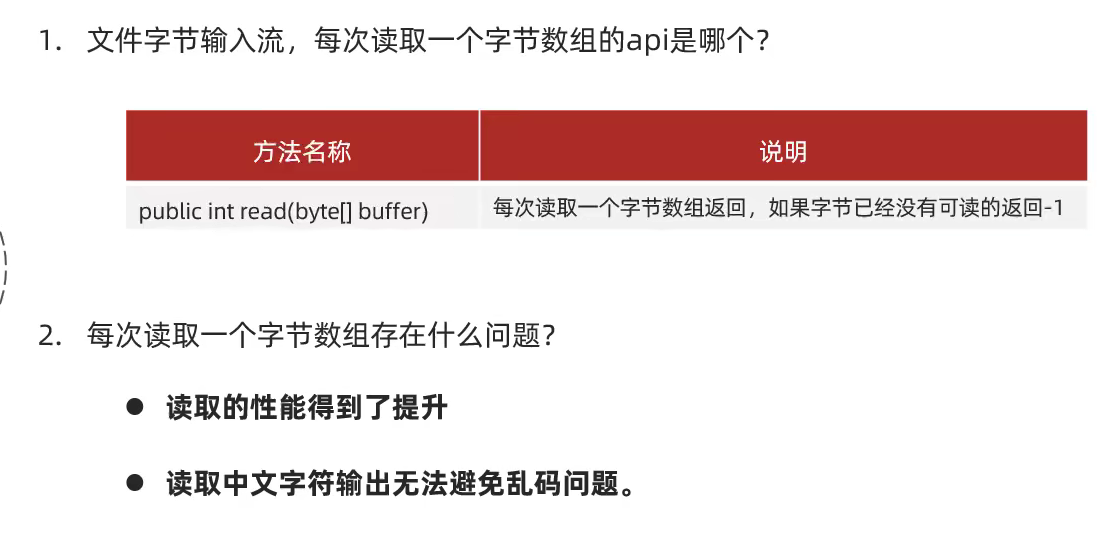
File byte input stream: read all bytes at one time
How to use byte input stream to read Chinese content and output without garbled code
Define a byte array as large as the file to read all the bytes of the file at one time
Directly reading all the file data into a byte array can avoid garbled code. Is there a problem
If the file is too large, the byte array may cause memory overflow
package com.itliuxue.d4_byte_stream; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.InputStream; public class FileInputStreamDemo03 { public static void main(String[] args) throws Exception { File f = new File("D:\\IDEA\\file-io-app\\src\\date02"); InputStream is = new FileInputStream(f); //Define a byte array just as large as the file size byte[] buffer = new byte[(int) f.length()]; int len = is.read(buffer); System.out.println("How many bytes were read"+len); System.out.println("file size"+f.length()); System.out.println(new String(buffer));, } }
File byte output stream: write byte data to file
OutputStream os = new FileOutputStream("D:\\IDEA\\file-io-app\\src/out01.txt",true);
Append data pipeline (add data without deleting the data in the previous file)
package com.itliuxue.d4_byte_stream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.OutputStream; public class FileOutputStreamDemo01 { public static void main(String[] args) throws Exception { //Clear the previous data first and enter after writing data // OutputStream os = new FileOutputStream("D:\\IDEA\\file-io-app\\src/out01.txt"); OutputStream os = new FileOutputStream("D:\\IDEA\\file-io-app\\src/out01.txt",true); os.write('a'); os.write(98); os.write("\r\n".getBytes());//Line feed byte[] buffer = {'a',97,98,99}; os.write(buffer); os.write("\r\n".getBytes());//Line feed byte[] buffer2 = "I am Chinese,".getBytes(); os.write(buffer2); os.write("\r\n".getBytes());//Line feed byte[] buffer3 = {'a',97,98,99}; os.write(buffer3,0,2);//Write a part of a byte out //When writing data, you must refresh the data, and you can continue to use the stream // os.flush(); os.close();//Release resources, including refresh. After closing, the stream cannot be used } }
summary
File copy
package com.itliuxue.d4_byte_stream; import java.io.*; //Objectives; Learn to use byte stream to copy files public class CopyDemo { public static void main(String[] args) throws Exception { try { // 1. Create a byte input stream pipeline to connect with the original file InputStream is = new FileInputStream("C:\\Users\\LIUXUE\\Desktop\\wallhaven-z8dp1v.jpg"); //Create a byte output stream pipeline to connect with the target file OutputStream os = new FileOutputStream("C:\\Users\\LIUXUE\\Desktop\\Copy.jpg"); //Define a byte array to transfer data byte[] buffer = new byte[1024]; int len;//Defines the number of bytes read each time while ((len = is.read(buffer)) != -1){ os.write(buffer,0,len); } System.out.println("Copy succeeded!"); //Close flow os.close(); is.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } } }
summary
Resource release mode
try-catch-finally
Finally: provide a finally block during exception handling to perform all clear operations, such as releasing resources in the IO stream
Features: finally controlled statements will be executed eventually unless the JVM exits
Exception handling standard format: try catch... finally
Try catch finally format
Note: even if the return statement is executed on the code, it must be returned to finally
summary
try-with-resource
Code above
Use of character stream
File character input stream - read one character at a time
package com.itliuxue.d6_char_stream; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.Reader; public class FileReaderDemo01 { public static void main(String[] args) throws Exception { Reader fr = new FileReader("D:\\IDEA\\file-io-app\\src\\filereader01"); int code; while ((code = fr.read()) != -1) { System.out.print((char) code); } }}
summary
File character input stream -- read one character array at a time
package com.itliuxue.d6_char_stream; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.Reader; public class FileReaderDemo03 { public static void main(String[] args) throws Exception { Reader fr = new FileReader("D:\\IDEA\\file-io-app\\src\\filereader02"); int len; char[] buffer = new char[1024];//1k characters while ((len = fr.read(buffer)) != -1){ String rs = new String(buffer,0,len); System.out.print(rs); } } }
Summary
File character output stream
package com.itliuxue.d6_char_stream; import java.io.FileWriter; import java.io.IOException; import java.io.Writer; public class FileWriteDemo01 { public static void main(String[] args) throws Exception { //Overwrite the pipeline. Each startup will overwrite the previous data Writer w = new FileWriter("D:\\IDEA\\file-io-app\\src\\filewriter01"); w.write('a'); w.write("\r\n"); w.write('Liu'); w.write("\r\n"); w.write("abc,I am Chinese,"); w.write("abc,I am Chinese,",0,3); // w.flush();// After refreshing, the stream can continue to be used w.close();//Closing includes refreshing. After closing, the flow cannot be used } }
summary