See my blog for the original text: IQA (image quality assessment)
Full name: Image Quality Assessment
Baidu Encyclopedia IQA: https://baike.baidu.com/item/IQA/19453034?fr=aladdin
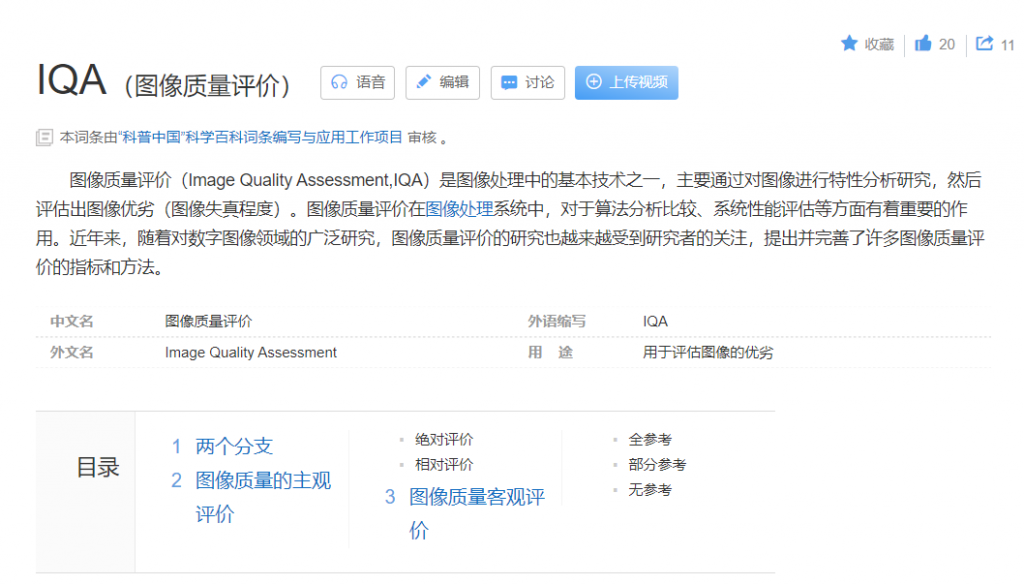
subjective evaluation
Subjective evaluation methods can be divided into two kinds: absolute evaluation and relative evaluation.
However, there are difficulties in implementation, which will not be considered here.
objective evaluation
Full reference
Image based pixel statistics
- Peak signal-to-noise ratio (PSNR): the greater the PSNR value, the smaller the distortion between the image to be evaluated and the reference image, and the better the image quality
- Mean square error (MSE): the smaller the MSE value, the better the image quality
Code example
import cv2 as cv import numpy as np import math # Mean square error (MSE): the smaller the MSE value, the better the image quality def get_mse(img1, img2): return np.mean((img1 / 255. - img2 / 255.) ** 2) # Peak signal-to-noise ratio (PSNR): the greater the PSNR value, the smaller the distortion between the image to be evaluated and the reference image, and the better the image quality # The benchmark is 30dB, and less than 30 is inferior def get_psnr(img1, img2): mse = get_mse(img1, img2) if mse < 1.0e-10: return 100 pixel_max = 1 return 20 * math.log10(pixel_max / math.sqrt(mse)) if __name__ == '__main__': origin_img = cv.imread('img.png', 1) new_img = cv.imread('denoised_img_noise.png', 1) print('MSE:', get_mse(origin_img, new_img)) print('PSNR:', get_psnr(origin_img, new_img))
result
MSE: 0.0004777804926332579
PSNR: 33.20771586094474
It can be seen from the results that the image quality after noise reduction used in the above test is still acceptable
Based on information theory
Based on information entropy in information theory, mutual information is widely used to evaluate image quality. In recent years, Sheikh and Bovik proposed two algorithms: information fidelity criterion (IFC) and visual information fidelity (VIF). They measure the quality of the image to be evaluated by calculating the mutual information between the image to be evaluated and the reference image. These two methods have certain theoretical support and expand the relationship between image and human eye in terms of information fidelity, but these methods do not respond to the structural information of image.
With regard to the two algorithms of information fidelity criterion (IFC) and visual information fidelity (VIF), it is difficult to find appropriate data on the Internet. Even if you can find individual ones, they are all matlab. The IFC method has not been realized yet. Here is the Vif method.
Code example
import numpy import scipy.signal import scipy.ndimage __all__ = ['compare_vifp'] def compare_vifp(ref, dist): sigma_nsq = 2 eps = 1e-10 num = 0.0 den = 0.0 for scale in range(1, 5): N = 2 ** (4 - scale + 1) + 1 sd = N / 5.0 if scale > 1: ref = scipy.ndimage.gaussian_filter(ref, sd) dist = scipy.ndimage.gaussian_filter(dist, sd) ref = ref[::2, ::2] dist = dist[::2, ::2] mu1 = scipy.ndimage.gaussian_filter(ref, sd) mu2 = scipy.ndimage.gaussian_filter(dist, sd) mu1_sq = mu1 * mu1 mu2_sq = mu2 * mu2 mu1_mu2 = mu1 * mu2 sigma1_sq = scipy.ndimage.gaussian_filter(ref * ref, sd) - mu1_sq sigma2_sq = scipy.ndimage.gaussian_filter(dist * dist, sd) - mu2_sq sigma12 = scipy.ndimage.gaussian_filter(ref * dist, sd) - mu1_mu2 sigma1_sq[sigma1_sq < 0] = 0 sigma2_sq[sigma2_sq < 0] = 0 g = sigma12 / (sigma1_sq + eps) sv_sq = sigma2_sq - g * sigma12 g[sigma1_sq < eps] = 0 sv_sq[sigma1_sq < eps] = sigma2_sq[sigma1_sq < eps] sigma1_sq[sigma1_sq < eps] = 0 g[sigma2_sq < eps] = 0 sv_sq[sigma2_sq < eps] = 0 sv_sq[g < 0] = sigma2_sq[g < 0] g[g < 0] = 0 sv_sq[sv_sq <= eps] = eps num += numpy.sum(numpy.log10(1 + g * g * sigma1_sq / (sv_sq + sigma_nsq))) den += numpy.sum(numpy.log10(1 + sigma1_sq / sigma_nsq)) vifp = num / den if numpy.isnan(vifp): return 1.0 else: return vifp
result
Using the same test picture, the results can be obtained:
VIF: 0.9758460769325894
Structure based information base
- SSIM: the structural similarity between the reference image and the image to be evaluated is constructed according to the correlation between image pixels. The larger the SSIM value, the better the image quality
PS: so far, find a more reasonable code method
Code example
import cv2 as cv import numpy as np import math from skimage.metrics import mean_squared_error from skimage.metrics import peak_signal_noise_ratio from skimage.metrics import structural_similarity # Mean square error (MSE): the smaller the MSE value, the better the image quality def get_mse(img1, img2): return np.mean((img1 / 255. - img2 / 255.) ** 2) if __name__ == '__main__': origin_img = cv.imread('img.png', 1) new_img = cv.imread('denoised_img_noise.png', 1) # Mean square error (MSE): the smaller the MSE value, the better the image quality mse = get_mse(origin_img, new_img) # Peak signal-to-noise ratio (PSNR): the greater the PSNR value, the smaller the distortion between the image to be evaluated and the reference image, and the better the image quality # The benchmark is 30dB, and less than 30 is inferior psnr = peak_signal_noise_ratio(origin_img, new_img) # Structural similarity (SSIM). The larger the SSIM value, the better the image quality ssim = structural_similarity(origin_img, new_img, multichannel=True) print('MSE:', mse) print('PSNR:', psnr) print('SSIM:', ssim)
result
MSE: 0.0004777804926332579
PSNR: 33.20771586094474
SSIM: 0.9570612590498769
Existing problems
There is a line in my code: from skimage.metrics import mean_squared_error
However, mean is not used below_ squared_ Error is a function because mean is used in the test_ squared_ The result (30 +) obtained by the error function is the same as the get written by yourself_ The result of MSE function (0 +) is quite different, and most of the resources on the Internet are similar to get_mse method, while mean_squared_error method is rarely mentioned, and from the results, the value of MSE algorithm should be as small as possible, so I don't use mean for the time being_ squared_ Error function
Partial reference
Partial reference also becomes semi reference. It takes part of the feature information of the ideal image as a reference, compares and analyzes the images to be evaluated, and obtains the image quality evaluation results. Because the reference information is the feature extracted from the image, it must first extract part of the feature information of the image to be evaluated and the ideal image, and evaluate the quality of the image to be evaluated by comparing the extracted part of the information. Some reference methods can be divided into methods based on original image features, methods based on digital watermarking and methods based on Wavelet domain statistical model. Because part of the reference quality evaluation depends on some features of the image, compared with the whole image, the amount of data decreases a lot. At present, the application is more concentrated in the image transmission system.
There is no clue about this part for the time being. Let go first
No reference
The non reference method is also called the first evaluation method. Because the general ideal image is difficult to obtain, this quality evaluation method which is completely independent of the dependence on the ideal reference image is widely used. No reference methods are generally based on image statistical characteristics.
- mean value
- standard deviation
- Average gradient
- entropy
- . . . . . .
I understand
The so-called no reference method is to analyze only the existing processed pictures without comparing with the original picture. The evaluation method is actually whether it conforms to the characteristics of the ideal image (similar to the way of looking for noise: the pixels that conform to the noise characteristics will be judged as noise).
Personally, this method is more suitable for the situation that the original image cannot be obtained or there is non negligible noise in the original image.