There is only one abstract method in the interface:
Modifier interface Interface name { public abstract Return value type method name(Optional parameter information); // Other non abstract method content }
Since the public abstract keywords in the interface can be omitted, the above functional interface can be written in the following form:
Modifier interface Interface name { Return value type method name(Optional parameter information); // Other non abstract method content }
Abstract method definition: for methods without method body (without braces), abstract methods must be modified with abstract. Before JDK1.8, all methods in the interface have no method body and are public, so methods before JDK1.8 will not write public and abstract keywords.
2.2 @FunctionalInterface annotation
If we define an interface as a functional interface, we must ensure that there is only one abstract method. At this time, if a new method (generally abstract method) is added to the interface by others, it will break the original design intention of the original functional interface and lead to problems in the running process of the program.
In view of this situation, a new annotation @ FunctionalInterface is specially introduced for functional interfaces in java, which means that if an interface is annotated with @ FunctionalInterface and there are multiple abstract methods in the interface, errors will be reported during compilation, which prevents the possibility of tampering with functional interfaces.
For example, the following code has two abstract methods: method1();method2() is compiled:
@FunctionalInterface public interface MyFunctionalInterface { void method1(); void method2(); }
2.3 customize a functional interface
We now customize a functional interface. In order not to destroy the functional interface, we usually add @ functional interface annotation on the functional interface.
@FunctionalInterface public interface MyFunctionInterface { void method1(); } /** * Test execution functional interface */ public class DemoMyFunctionalInterface { private static void execFunctionMethod(MyFunctionInterface inter) { inter.method1(); } public static void main(String[] args) { execFunctionMethod(() -> { System.out.println("Execute functional interface method!"); }); } }
The execution result of the above code is: execute the functional interface method!, Again, the @ functional interface annotation can be removed, but it is recommended to add this annotation to all functional interfaces.
3. Common functional interfaces
JDK provides a large number of commonly used functional interfaces, which are mainly in the java.util.function package. Next, we will demonstrate several commonly used functional interfaces.
Supplier interface
The Supplier interface is defined in JDK as follows:
@FunctionalInterface public interface Supplier<T> { /** * Gets a result. * * @return a result */ T get(); }
The java.util.function.Supplier interface only contains a parameterless method T get(). If this method is called, an object with generic type T will be returned. The specific generation of the object is provided by the corresponding lambda expression.
Let's take an example of obtaining the maximum value from an integer array using the Supplier interface:
public class DemoSupplier { public static int max(Supplier<Integer> sup) { return sup.get(); } public static void main(String[] args) { int arr[] = {5, 7, 338, 12}; // Call getMax method and pass Lambda as parameter int maxNum = max(() -> { // Calculate the maximum value of the array int max = arr[0]; for (int i : arr) { if (i > max) { max = i; } } return max; }); System.out.println(maxNum); } }
The code execution result is 338. The code logic is to define a method whose max parameter is the Supplier interface, and the logic to obtain the maximum value of data is written in the lambda expression.
Consumer interface
The Consumer interface is defined in the JDK as follows:
@FunctionalInterface public interface Consumer<T> { /** * Performs this operation on the given argument. * @param t the input argument */ void accept(T t); /** * */ default Consumer<T> andThen(Consumer<? super T> after) { Objects.requireNonNull(after); return (T t) -> { accept(t); after.accept(t); }; ## last Here I specially compiled a copy<**Android Notes on developing core knowledge points**>,It contains customization View Related content 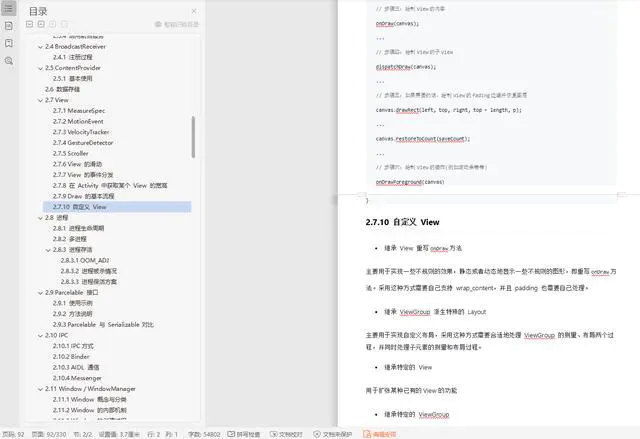 In addition to this note, I'll share it with you **Android study PDF+Architecture video+Interview documents+Source notes**,Advanced architecture technology, advanced brain map Android Develop interview materials and advanced architecture materials. It is very suitable for friends who have recent interviews and want to continue to improve on the technical road. **[CodeChina Open source projects:< Android Summary of study notes+Mobile architecture video+Real interview questions for large factories+Project practice source code](https://codechina.csdn.net/m0_60958482/android_p7)** 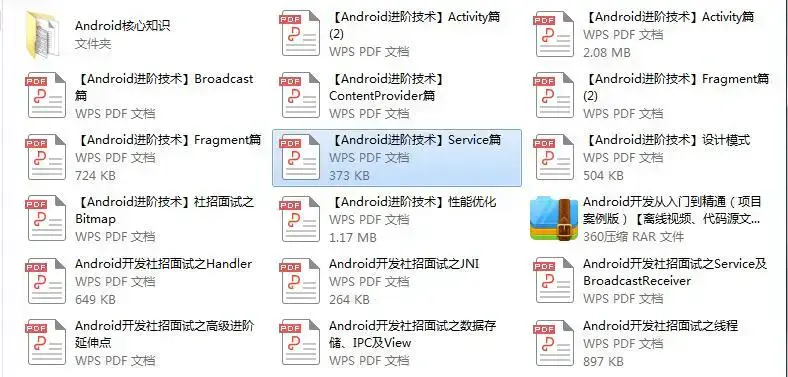 Share the above resources, hoping to help you improve,**If you find them useful, you might as well recommend them to your friends~** s://codechina.csdn.net/m0_60958482/android_p7)** [External chain picture transfer...(img-07gEfib0-1630671346910)] Share the above resources, hoping to help you improve,**If you find them useful, you might as well recommend them to your friends~** > If you like this article, give me a little praise, leave a message in the comment area or forward it for support~