1. Overview of component life cycle
- Significance: the life cycle of components is helpful to understand the operation mode of components, complete more complex component functions, analyze the causes of component errors, etc.
- **Component life cycle: * * the process from component creation to mounting to running on the page, and then to component uninstallation when not in use
- Each stage of the life cycle is always accompanied by some method calls, which are the hook functions of the life cycle
- Hook function: it provides an opportunity for developers to operate components at different stages.
- Only class components have a lifecycle
2. Three stages of the life cycle
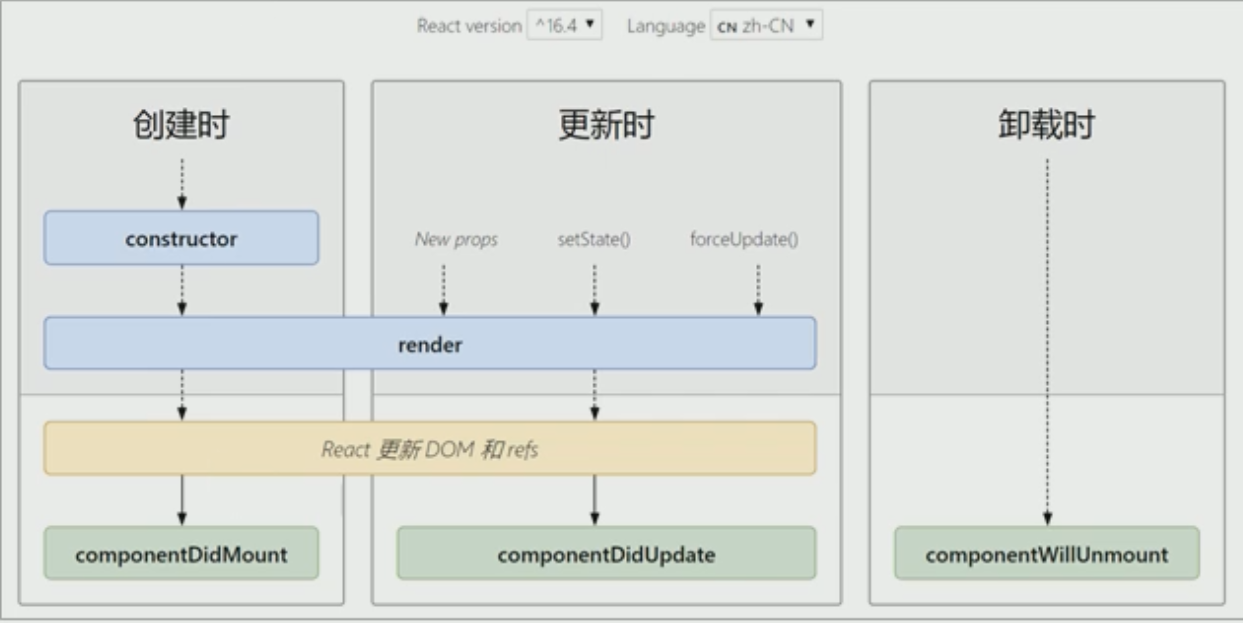
1. When creating (Mount phase)
Execution time: when the component is created (when the page is loaded)
Execution sequence:
constructor()-> render()-> compoDidMount()
Hook function | Trigger timing | effect |
---|
constructor | When creating a component, execute first | 1. Initialize state 2 Bind this for the event handler |
rende r | Triggered each time a component is rendered | Rendering UI (Note: cannot call setState(), which will cause recursive update) |
componDidMount | After the component is mounted (DOM rendering is completed) | 1. Send ajax request to obtain remote data 2 Perform DOM operations |
/*
Component lifecycle
*/
class App extends React.Component {
constructor(props) {
super(props)
// Initialize state
this.state = {
count: 0
}
// Handle this pointing problem
console.warn('Lifecycle hook function: constructor')
}
// 1 perform DOM operation
// 2 send ajax request to get remote data
componentDidMount() {
// axios.get('http://api.....')
// const title = document.getElementById('title')
// console.log(title)
console.warn('Lifecycle hook function: componentDidMount')
}
render() {
// Error demo!!! Do not call setState() in render.
// this.setState({
// count: 1
// })
console.warn('Lifecycle hook function: render')
return (
<div>
<h1 id="title">Count the number of times Doudou was beaten:</h1>
<button id="btn">Beat beans</button>
</div>
)
}
}
2. When updating (update phase)
- Execution time: 1 setState() 2. forceUpdate()3. Component received new props
- Note: if any of the above three changes, the component will be re rendered
- Execution order: render() – > componentdidupdate()
Hook function | Trigger timing | effect |
---|
render | Triggered each time a component is rendered | Render UI (same redner as Mount phase) |
componDidUpdate | After the component is mounted (DOM rendering is completed) | 1. Send ajax request to obtain remote data 2 Note: if you want to use the DOM, setState () must be placed in an if condition |
class App extends React.Component {
constructor(props) {
super(props)
// Initialize state
this.state = {
count: 0
}
}
// Beat beans
handleClick = () => {
// render() is triggered when the setState method is triggered
// this.setState({
// count: this.state.count + 1
// })
// render() is triggered when a forced update occurs
this.forceUpdate()
}
render() {
console.warn('Lifecycle hook function: render')
return (
<div>
<Counter count={this.state.count} />
<button onClick={this.handleClick}>Beat beans</button>
</div>
)
}
}
// Subcomponent computer
class Counter extends React.Component {
render() {
console.warn('--Subcomponents--Lifecycle hook function: render')
return <h1>Count the number of times Doudou was beaten:{this.props.count}</h1>
}
}
3. During unloading (unloading phase)
- Execution phase: the component disappears from the page
Hook function | Trigger timing | effect |
---|
componentWillUnmount | Component uninstall (disappear from page) | Perform cleaning work (e.g. cleaning timer, etc.) |
class App extends React.Component {
constructor(props) {
super(props)
// Initialize state
this.state = {
count: 0
}
}
// Beat beans
handleClick = () => {
this.setState({
count: this.state.count + 1
})
}
render() {
return (
<div>
{this.state.count > 3 ? (
<p>Doudou was killed~</p>
) : (
<Counter count={this.state.count} />
)}
<button onClick={this.handleClick}>Beat beans</button>
</div>
)
}
}
class Counter extends React.Component {
componentDidMount() {
// Turn on the timer
this.timerId = setInterval(() => {
console.log('The timer is executing~')
}, 500)
}
render() {
return <h1>Count the number of times Doudou was beaten:{this.props.count}</h1>
}
componentWillUnmount() {
console.warn('Lifecycle hook function: componentWillUnmount')
// Remember to clean the timer manually ~ otherwise it may cause problems such as memory leakage
clearInterval(this.timerId)
}
}
Introduction to uncommon hook functions
New full lifecycle hook function (understand):
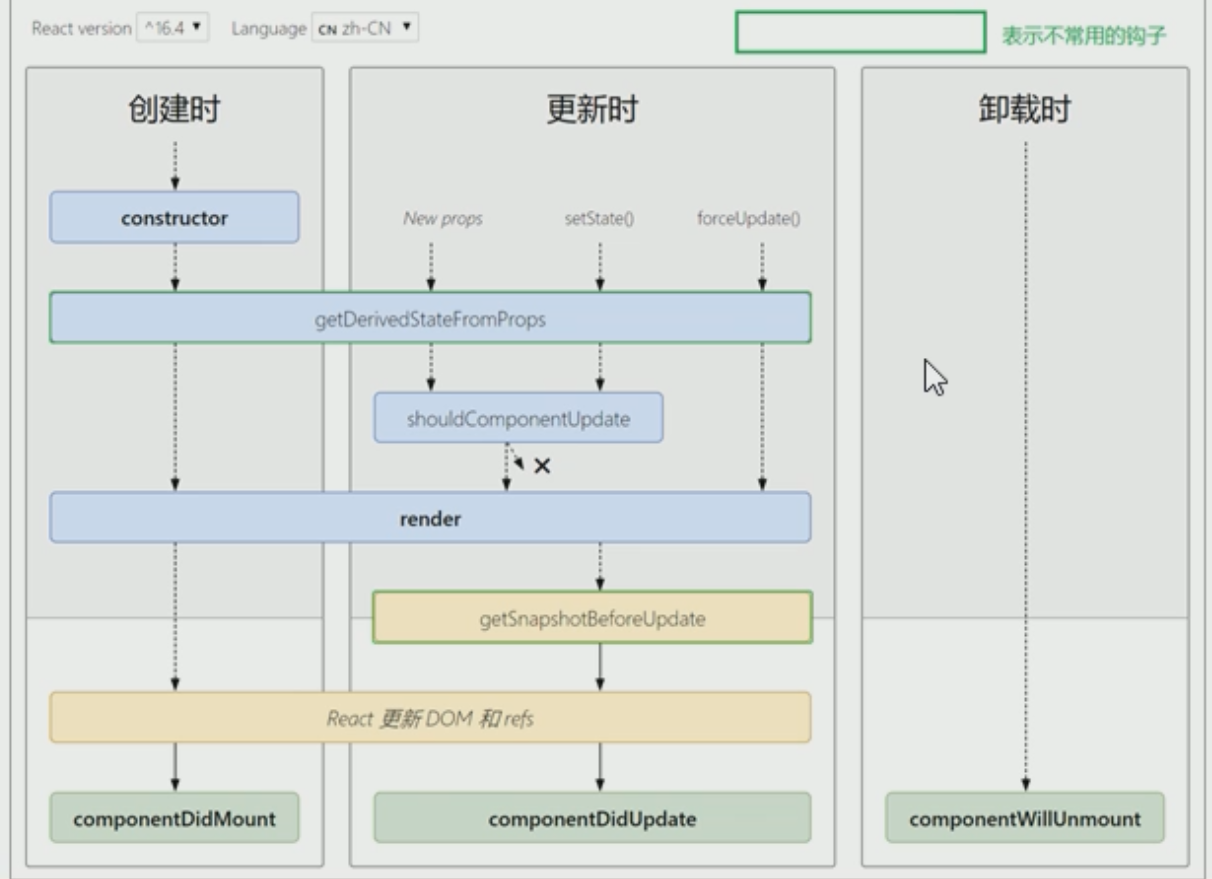