The previous learning section introduced the use of some form contents - the previous cases basically used input. Next, learn the difference between controlled forms and uncontrolled forms, and learn the knowledge points of uncontrolled components through uncontrolled forms.
Controlled forms refer to forms whose states are managed by React. Naturally, uncontrolled forms are forms whose states are not managed by React.
The learning case download resources are: Controlled form vs uncontrolled form - Case
Contents reviewed before:
- What is JSX and how to use it
- Element vs component
- State & state update & lifecycle approach
- event processing
Controlled form
Controlled forms refer to all forms whose state is managed by state. The state update is triggered through setState, such as the following controlled component:
class ControlledForm extends Component { constructor() { super(); this.state = { name: '', phone: '', }; this.onSubmit = this.onSubmit.bind(this); } updateInputHandler = (e, label) => { this.setState((prevState) => { return { ...prevState, [label]: e.target.value }; }); }; onSubmit = (e) => { for (const [key, val] of Object.entries(this.state)) { console.log(key, val); } // e.preventDefault(); Place page redirection e.preventDefault(); }; render() { return ( <form onSubmit={this.onSubmit}> <div> <label>name: </label> <input type="text" value={this.state.name} onChange={(e) => this.updateInputHandler(e, 'name')} /> </div> <div> <label>phone: </label> <input type="text" value={this.state.phone} onChange={(e) => this.updateInputHandler(e, 'phone')} /> </div> <div> <input type="submit" value="submit" /> </div> </form> ); } }
The results are as follows:

The event processing here can simplify the method of state update by receiving another parameter. This is achieved by using the special feature of calculating attribute names in ES6. By using obj[variable] syntax, you can dynamically update the value of the parameter (variable) by passing in the parameter (variable).
For a better understanding of the new features of ES6, please refer to: It's 2021. Don't you even know what ES6/ES2015 has been updated This note.
In addition, note that the writing of setstate here is different from that in the previous case, because the state updated by React cannot be guaranteed to be synchronized. In order to save efficiency, it will determine which setstates can be merged internally and update them in a batch job. So call this directly in setstate State to pass values is a very dangerous behavior. And through this setState(prevState => {//...}) Callback function, which can ensure that the state obtained in setstate is the state at the time of update.
According to the above illustration, this. is called in setState2. State, which may be greatly affected by setState4, resulting in an error in the updated state. This kind of merging and updating often occurs in large applications, so we must pay attention to this.
Indeed, it will be a bit troublesome to write controlled components, especially if you need to add other type checks later, there will be a lot of content in updateInputHandler, which is also the content subject of optimization / encapsulation in the next step.
refs
refs is actually a method that can access DOM nodes more directly in React or create React elements in the render method. Its appearance is aimed at the practical methods that may need to be used in the reaction of unconventional operation in the component.
Recall that the conventional method is that the components are managed through state, and the parent-child components pass information through props. Naturally, the unconventional method is to operate on components and / or elements without state or props.
In fact, React officials do not recommend using refs for DOM operation, because it will give up the benefits of Virtual DOM - that is, by comparing the Virtual DOM tree with the real DOM tree, only the updated DOM nodes will be re rendered, which can save a lot of meaningless repeated rendering.
The React team listed three scenarios for using refs:
- Manage focus, text selection, or media playback.
- Trigger forced animation.
- Integrate third-party DOM libraries.
Generally speaking, refs is not needed in normal business situations... At least I haven't used it yet. Now the method of creating and using refs is also simple:
class MyComponent extends React.Component { constructor(props) { super(props); // Create ref by createRef this.myRef = React.createRef(); } render() { // The ref instance}} created by ref={this. {can be associated with Ref return <div className="exposeRef" ref={this.myRef} />; } }
In this way, the div element with the class name exposeRef can operate this Myref method to modify. After all, refs can also be passed to other components through props, so that exposeRef can be operated directly in other components, usually subcomponents of MyComponent.
Uncontrolled form
If the content of an uncontrolled form cannot be controlled by state, the value cannot be obtained through state. In this case, it can only be handled by refs, such as:
class Form extends Component { constructor() { super(); this.name = React.createRef(); this.phone = React.createRef(); this.onSubmit = this.onSubmit.bind(this); } onSubmit(e) { console.log('submit form'); console.log(this.name.current.value, this.phone.current.value); // e.preventDefault(); Place page redirection e.preventDefault(); } render() { return ( <form onSubmit={this.onSubmit}> <div> <input type="text" ref={this.name} /> </div> <div> <input type="text" ref={this.phone} /> </div> <div> <input type="submit" value="submit" /> </div> </form> ); } }
The use effect is:
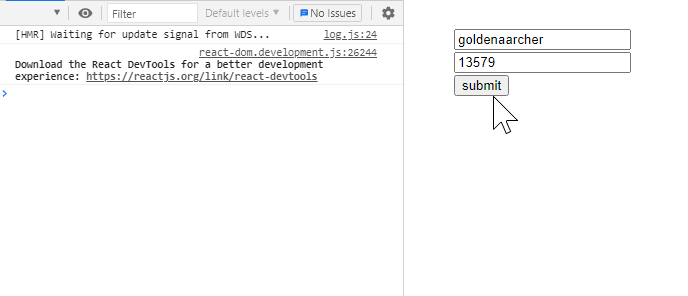
You can see that the first line of the submit form and the second line also successfully output the values in the two input input boxes.
If you only need the value of the form and do not need to verify the content of the form, it will be more convenient to use the uncontrolled form. By comparing the same code, you can see that the amount of code of the uncontrolled component is less and the implementation is simpler.
Relatively speaking, the application scope of this business is also relatively small.
Attention, remember State & state update & lifecycle approach Error reporting information mentioned in:
The solution of changing the value in input to defaultValue is mainly for uncontrolled components. In uncontrolled components, you can set the default value by adding the property defaultValue.
Uncontrolled components
Uncontrolled form is an uncontrolled component, but uncontrolled components have a wider range of applications.
Compared with controlled groups, uncontrolled components are rarely used. In most cases, third-party libraries are used to manage div. for example, some visual processing libraries are used. In this case, the componentWillUnmount method is also required to clean up the elements managed by the third-party library.
For example How to properly use componentWillUnmount() in ReactJs The answer adopted in the uses a case:
import React from 'react'; import { Component } from 'react'; import ReactDom from 'react-dom'; import d3Chart from './d3charts'; export default class Chart extends Component { static propTypes = { data: React.PropTypes.array, domain: React.PropTypes.object, }; constructor(props) { super(props); } componentDidMount() { let el = ReactDom.findDOMNode(this); d3Chart.create( el, { width: '100%', height: '300px', }, this.getChartState() ); } componentDidUpdate() { let el = ReactDom.findDOMNode(this); d3Chart.update(el, this.getChartState()); } getChartState() { return { data: this.props.data, domain: this.props.domain, }; } componentWillUnmount() { let el = ReactDom.findDOMNode(this); d3Chart.destroy(el); } render() { return <div className="Chart"></div>; } }
The Chart component in this case does not contain any state. Any operation on the image is managed by d3Chart itself.
In addition, the function findDOMNode is in strict mode, that is < react Strictmode > < other components / > < / react Strictmode > has been abandoned, and refs is officially recommended to replace findDOMNode.
When using third-party components, you must remember that if a new object is created by binding ref/findDOMNode in componentDidMount, you must pay attention to destroying the created object in componentWillUnmount to prevent memory leakage.
summary
Here, we mainly have a certain degree of in-depth understanding of the content of the form, and learned about the use of refs for uncontrolled forms, as well as the key points that should be paid attention to in integrating third-party libraries in React to manage uncontrolled components.
The knowledge points are summarized as follows:
-
Controlled forms are managed through state
The knowledge points in the controlled form include:
-
Calculation properties of ES6
By calculating properties, you can quickly update the value of a given parameter of an object
-
Use setState correctly
This refers to the content lost to update the status of multiple key value pairs.
In order to improve performance, React compresses setstates, and then uses batch update to update multiple setstates, so as to avoid frequent UI updates. At this time, it is necessary to adopt another writing method to update etState, instead of directly calling this State method to get value
-
-
Uncontrolled form, let the page handle the problem of value update
Update and obtain values through refs
-
Uncontrolled components, including uncontrolled forms, have a wider range of applications
A case of StackOverflow is used to learn the application of uncontrolled components